JSON doesn’t support comments by design. This means you cannot use traditional code comments (//, /* .. */, or #) to add information as a comment in your JSON data.
The only workaround for adding comments in JSON is by adding a JSON element with a key such as “_comment” and a value that is the comment.
This quick guide teaches you why code comments are not possible in JSON and how to add comments in JSON with a simple workaround.
Why JSON Doesn’t Support Comments?
As a software developer, you know how valuable code comments can sometimes be.
Even though you should always write code that expresses itself well enough so that code comments aren’t needed, sometimes they are a must! For example, if a piece of code is a bit out-of-sort but necessary for project-specific needs, it might need some clarification.
Make sure to read my complete guide on how to comment code.
To your surprise, the JSON data format doesn’t allow comments by design. But there’s a great reason why this is not the case. As a matter of fact, in the past, JSON supported comments.
Here’s what the creator of JSON, Douglas Crockford, had to say about why JSON doesn’t support JSON:
I removed comments from JSON because I saw people were using them to hold parsing directives, a practice which would have destroyed interoperability.
Let’s have a moment to clarify what this means.
In the earlier days of JSON, developers stored parser-specific commands called parsing directives into JSON files as comments.
A parsing directive changes the behavior of the JSON file based on the parser being used. This could lead to differences in the behavior of a JSON file based on the browser being used.
The creator of JSON thought adding these parsing directives as a comment in JSON is a bad idea and a practice that might hurt JSON interoperability. This is the reason why he decided to remove comments in JSON.
Keep in mind that even though adding parsing directives isn’t possible in the form of JSON comments anymore, they can still be added to JSON data as string literals, for example.
This is why removing the JSON comments was a controversial decision. Some argue removing comments only makes JSON worse because developers use hacks to still be able to insert comments into JSON data.
How to Add a Comment in JSON?
Meanwhile adding actual comments in JSON is not possible, there are two main workarounds.
- Add a “_comment” field in the JSON data.
- Use JSON minifier to strip comments with JavaScript code.
1. Add a “_comment” Field
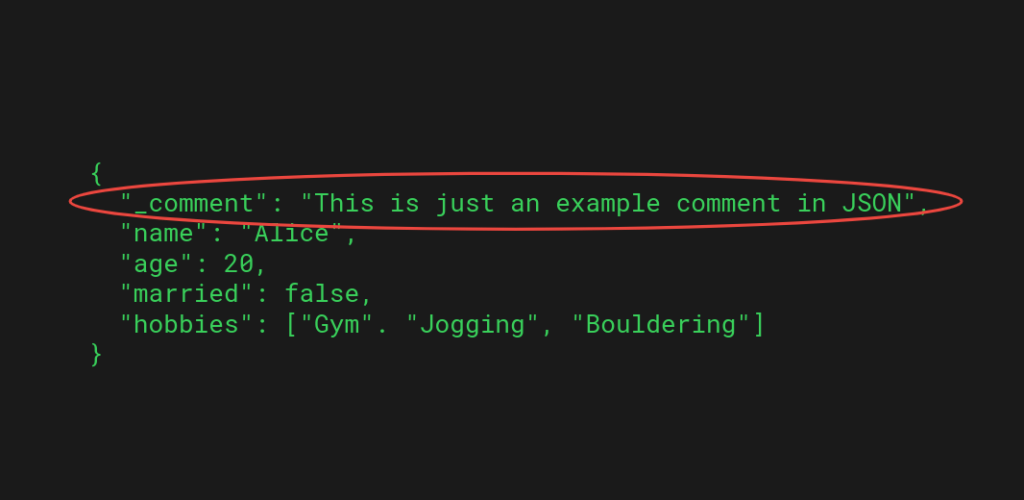
The most obvious workaround to add a comment in JSON is by adding a designated comment element in the JSON data.
For example:
{ "_comment": "This is just an example comment in JSON", "name": "Alice", "age": 20, "married": false, "hobbies": ["Gym". "Jogging", "Bouldering"] }
Here the comment is associated with a key “_comment“.
Notice that this type of comment is not an actual comment, but valid JSON data like any other key-value pair in the data.
Also, to avoid potential confusion, make sure to add a noticeable prefix to the comment field. In the above example, I used “_comment” instead of “comment“. This is because if there ever was a real JSON field named “comment“, you wouldn’t accidentally overwrite its meaning.
2. Use a JSON Minifier with JavaScript
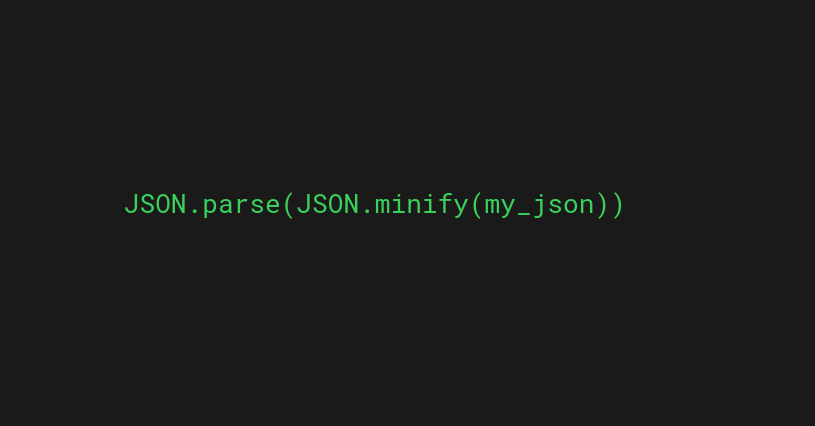
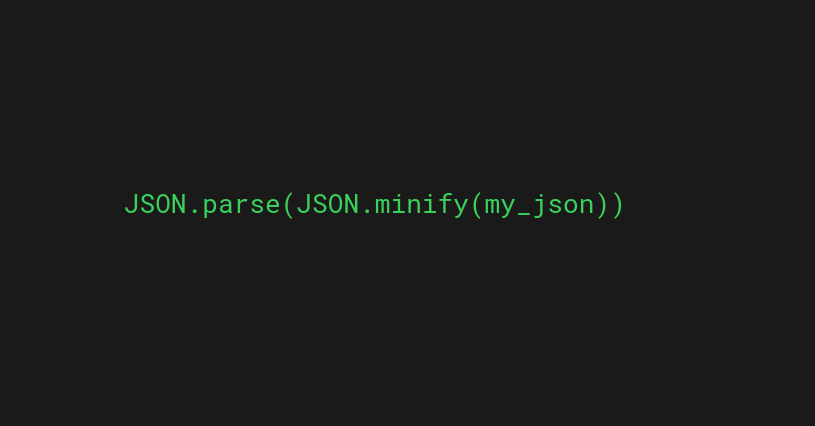
Another way to add a JSON comment is by adding comments but using a JSON minifier to remove the comments before using the JSON.
For example, here’s an external JavaScript library, JSON.minify() that strips JSON from comments and white spaces.
As an example, here’s the input JSON:
const my_json = { /* This is an example JSON comment with multiple lines of text that is going to be removed by a JSON minifier */ "name": "Alice", "age": 20, "married": false, "hobbies": ["Gym". "Jogging", "Bouldering"] }
Now, let’s run the JSON through the minifier:
JSON.parse(JSON.minify(my_json))
Output:
{ "name": "Alice", "age": 20, "married": false, "hobbies": ["Gym". "Jogging", "Bouldering"] }
This removes the comments in the JSON which makes it valid JSON data.
Summary
You cannot add comments in JSON by design. But there are some workarounds that still make it possible. The most notable workaround is by adding a “_comment” field (or similar) in which you place the comment. But the downside of this is that the comment becomes part of the JSON data and not an ignorable comment like in coding languages.
Another way to add comments in JSON is by using an external code library, such as JSON.minify() in JavaScript. This library strips the comments away from the JSON data before it’s used. So when you send the commented JSON, the comments are left out and the recipient never sees them!
Thanks for reading. Happy coding!