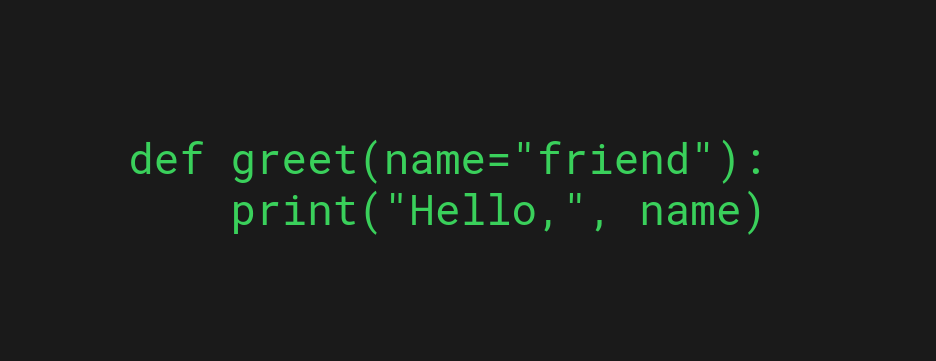
In almost every Python program, you are dealing with functions that take input, perform an action, and spit out an output. More often than not, it can be useful to include a default argument value in a function. If an input argument isn’t provided, the default value is used instead. And if the input value is provided, that’s going to be used in the function.
You can add default function parameters in Python by giving a default value for a parameter in the function definition using the assignment operator (=).
For example:
def greet(name="friend"): print("Hello,", name)
Now the default value for the name is “friend“.
If you call this function without a parameter:
greet()
You get the default greeting:
Hello friend
But if you call it with a name argument:
greet("Jon")
You get a personalized greeting using the name:
Hello Jon
This is a complete guide to default values in Python.
You will learn what is a default argument and how to specify multiple default arguments in a function. Besides, you learn that you can use both regular arguments and default arguments in the same function.
Multiple Default Parameters in Python
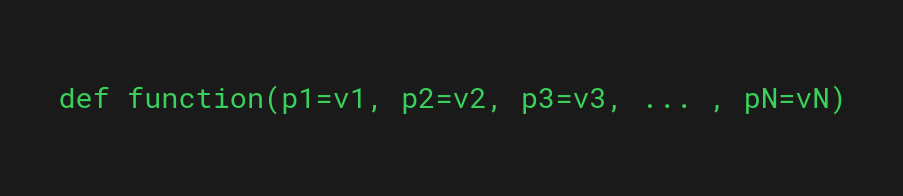
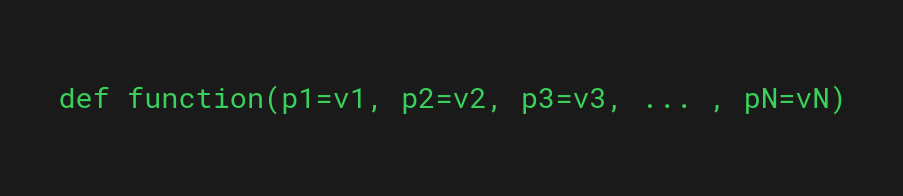
You can implement Python functions with multiple default parameters. This works similarly to how you can have functions with a number of regular parameters:
- Specify the parameter name and assign it a default value.
- Then add a comma and specify the next parameter.
def function(param1=value1, param2=value2, param3=value3, ...):
For instance, let’s create a function that asks for first name and last name. If these are not given, the default values are used instead:
def greet(firstname="Mr.", lastname="Who"): print("Hello,", firstname, lastname)
Here are some example calls for this function:
greet() greet("Jack") greet(lastname="Jones") greet("Matt", "Gibson")
Outputs:
Hello, Mr. Who Hello, Jack Who Hello, Mr. Jones Hello, Matt Gibson
Next, let’s take a look at how to add both default and regular parameters into a function call.
Mix Up Default Parameters and Regular Parameters
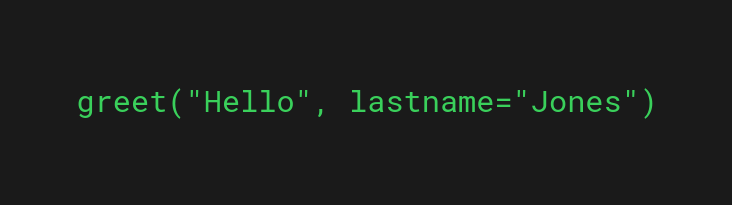
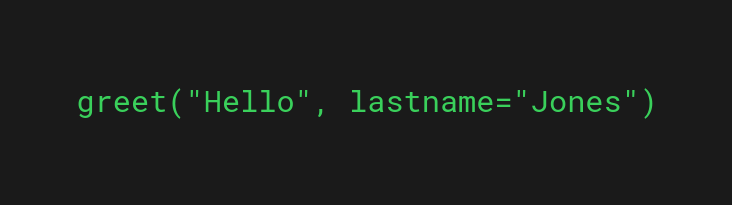
You can define a function with both default parameters and regular parameters in Python. When you call a function like this, the Python interpreter determines which variable is which based on whether it has the argument label or not.
For example:
def greet(greeting_word, firstname="Mr.", lastname="Who"): print(greeting_word, firstname, lastname) # Example calls: greet("Hi") greet("Hi", "Jack") greet("Hello", lastname="Jones") greet("Howdy", "Matt", "Gibson")
Output:
Hi Mr. Who Hi Jack Who Hello Mr. Jones Howdy Matt Gibson
Last but not least, let’s take a look at how default parameters are denoted in Python documentation.
Default Parameters in Python Documentation
If you read the Python documentation, you sometimes see functions that have default parameters in the function syntax.
For example, let’s take a look at the enumerate function documentation:


In this function, there is start=0 in the arguments.
This means that the enumerate function has a default argument start that is 0 by default. In other words, you can call the enumerate() function by specifying:
- iterable parameter only.
- start parameter to be something else than 0.
Conclusion
Today you learned about Python function default parameters.
You can provide default values for function parameters in Python. This can simplify your function calls and code.
Here is an example of a function with a default parameter value:
def greet(name="friend"): print("Hello,", name)
When you call this function without a parameter, it defaults to saying “Hello, friend”. When you call it with a name argument, it greets the person with that name.
Thanks for reading. I hope you enjoy it. Happy coding!