In Python, the difference between the @staticmethod
and @classmethod
is that:
-
@staticmethod
is usually a helper method that behaves the same way no matter what instance you call it on. The@staticmethod
knows nothing about the class or its instances. -
@classmethod
is a method that takes the class as an argument. You can use it as an alternative constructor to the class. (E.g.MyClass.from_string()
)
This is a complete guide to understanding the differences between @staticmethod and @classmethod in Python. You will learn when to use and when not to use these methods. All the theory is backed up with illustrative examples.
What Is a @staticmethod in Python?
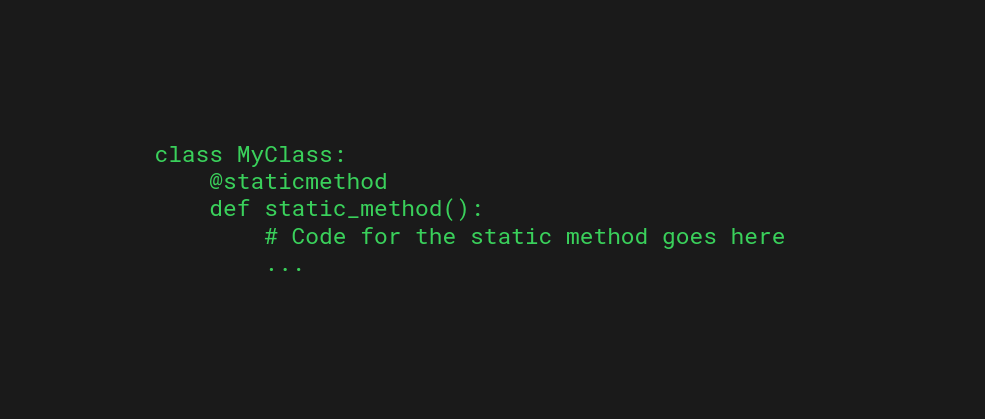
In Python, a
is a method that belongs to a class rather than an instance of the class.@staticmethod
This means that a
is a method that is shared among all instances of a class. It is called on the class itself, rather than on an instance of the class.@staticmethod
Here is an example of a class that defines a static method:
class MyClass: @staticmethod def static_method(): # Code for the static method goes here ...
To call a static method, you would do the following:
MyClass.static_method()
Note that you do not need to create an instance of the class in order to call the static method. This is because the static method belongs to the class itself, rather than to individual instances of the class. You can also see this by looking at the static method’s arguments. It takes neither self
nor cls
as the first argument.
What Is a @classmethod in Python?
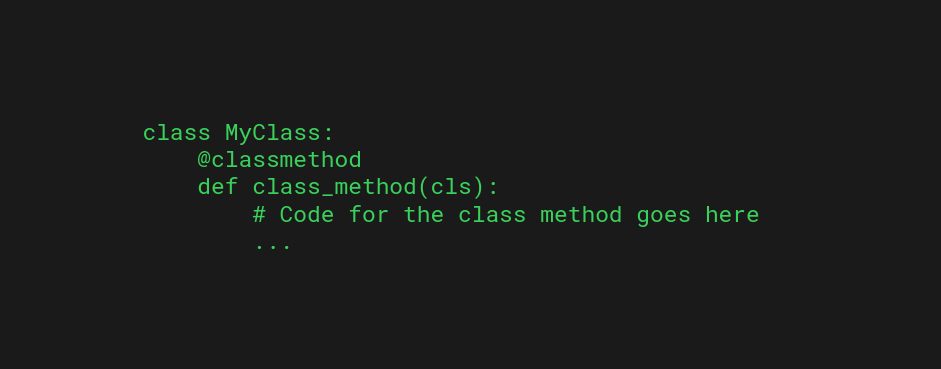
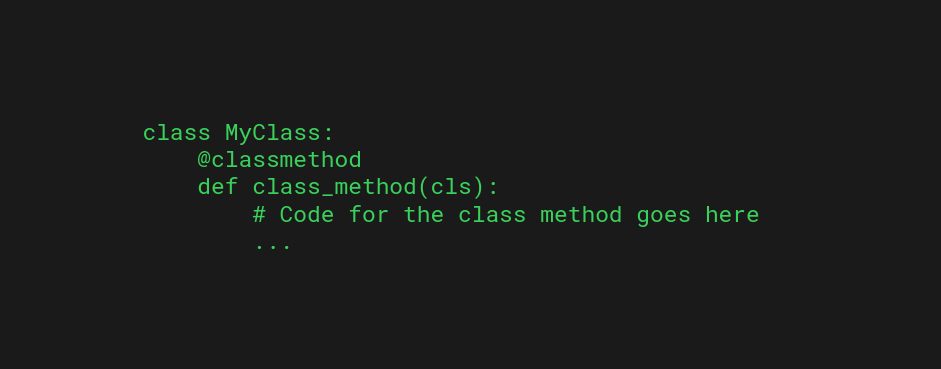
In Python, a
is a method you can call for both the instances of the class as well as the class itself. A class method takes the class itself (@classmethod
cls
) as the first argument, instead of an instance of the class (self
).
Here is an example of a class that defines a class method:
class MyClass: @classmethod def class_method(cls): # Code for the class method goes here ...
To call a class method, you would do the following:
MyClass.class_method()
Note that you do not need to create an instance of the class in order to call the class method. This is because the class method belongs to the class itself, rather than to individual instances of the class.
Also note that the first argument to the class method is the class itself, which is passed in automatically. In the example above, this argument is named cls
, but it could be named anything else.
@staticmethod vs @classmethod in Python
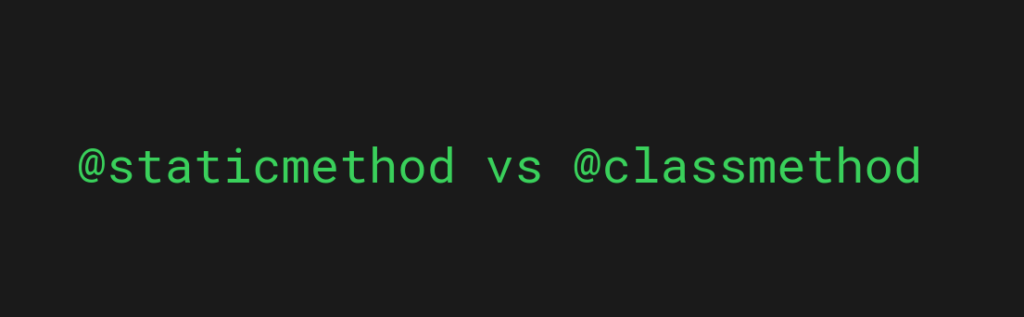
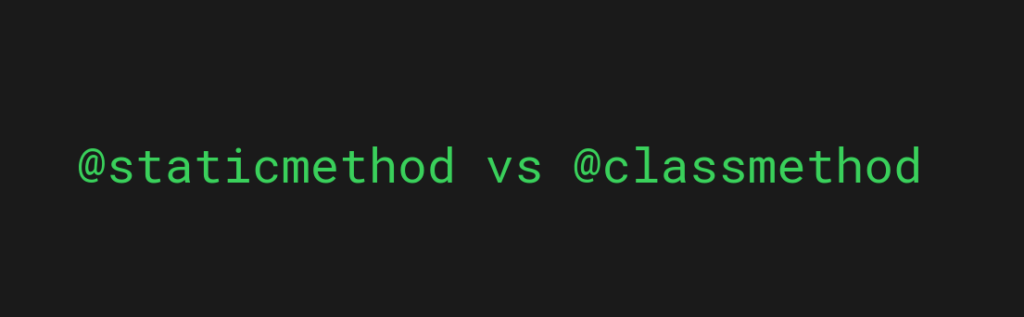
In Python, a
is a method that belongs to a class rather than an instance of the class. This means that a @staticmethod
is a method that is shared among all instances of a class. It is called on the class itself, rather than on an instance of the class. A static method knows nothing about the class or instance you’re calling it on.@staticmethod
On the other hand, a @classmethod
is a method that is called on a class, rather than on an instance of the class. It takes the class as the first argument, rather than an instance of it. This behavior is useful if you want to create an alternative constructor method that initializes the class objects from different parameters.
For example, the dict.fromkeys()
method is a class method that initializes a dictionary from keys.
Now that you understand the main difference between the class methods and static methods, let’s take a look at why and when you should use these method types.
Why Use @staticmethod?
There are several reasons why you might want to use a
rather than defining a regular function outside of a class in Python:@staticmethod
- A
@staticmethod
provides a clear indication that the method belongs to the class, rather than to individual instances of the class. This can make the code easier to read and understand since the purpose and behavior of the method are more clearly defined. - A
can be used to define helper functions that are related to the class, but that do not depend on any instance-specific state. This can make the code more modular and reusable since the@staticmethod
can be called from multiple places within the class, as well as from outside of the class.@staticmethod
- A
can be overridden in subclasses. This allows subclasses to provide their own implementation of@staticmethod
@staticmethod
, which can be useful in certain scenarios.
Overall, using a
instead of a regular function can make the code more organized, reusable, and readable, especially when dealing with classes and subclasses in Python.@staticmethod
When Use @staticmethod in Python?
Use a @staticmethod
in Python when you have a method that belongs to a class, rather than to individual instances of the class.
A
is typically used to define helper functions that are related to the class, but that does not depend on any instance-specific state. This makes the code modular and reusable as the @staticmethod
can be called from multiple places within the class, as well as from outside of the class.@staticmethod
Notice that if you have a function that does not belong to a class, and that does not depend on any class-specific state, just define a regular function outside of the class. A regular function is more flexible and can be called from anywhere, without being tied to classes so unless the behavior is related to a class it’s better to leave it out of the class.
Python @classmethod as an Alternative Constructor
In Python, a classmethod
can be used as an alternative constructor for a class. This means that you can use a classmethod
to define a method that can be used to create and return instances of the class, in addition to the regular __init__
method that is used as the default constructor.
Here is an example of how you might use a @classmethod
as an alternative constructor for a class:
class MyClass: def __init__(self, param1, param2): # Code for the regular constructor goes here ... @classmethod def from_string(cls, string): # Code for the classmethod constructor goes here ...
In the example above, the __init__
method is the regular constructor for the MyClass
class, which is called when you create an instance of the class using the MyClass()
syntax.
The from_string
method is a
that can be used as an alternative constructor for the class. In other words, if you want to initialize a @classmethod
MyClass
object from a string, you can call the from_string
method instead of the default initializer.
To use the from_string
method as an alternative constructor, you can simply do the following:
my_object = MyClass.from_string('some string')
In this example, the from_string
method is called on the MyClass
class, rather than on an instance of the class. It is passed the string 'some string'
as the argument, and it returns an instance of the MyClass
class, which is then assigned to the my_object
variable.
Overall, using a
as an alternative constructor can provide a more convenient and flexible way to create instances of a class in Python. It allows you to define multiple ways of creating instances of a class, and to choose the most appropriate method based on the specific needs of your application.@classmethod
Summary
In Python, a class method is a method that belongs to a class rather than a particular object. It is marked with the @classmethod
decorator. A class method receives the class as an implicit first argument, just like an instance method receives the instance.
A static method is a method that belongs to a class rather than a particular object. It is marked with the @staticmethod
decorator. A static method does not receive any additional arguments; it behaves like a regular function but belongs to the class.
Here is an example of how to use these decorators in a Python class:
class MyClass: @classmethod def class_method(cls): # ... @staticmethod def static_method(): # ...
The key difference between a class method and a static method is that a class method can access or modify the class state, while a static method cannot.
Thanks for reading. Happy coding!