You can use a semicolon in Python to put multiple statements on one line. The semicolon terminates the line of code and starts a new one.
Remember, you shouldn’t use semicolons in your Python code even though it’s possible!
Here’s an example of an expression where three separate lines of code are placed on the same line separated by semicolons.
print("Hello."); print("It is me."); print("How do you do?")
Output:
Hello. It is me. How do you do?
In Python, the semicolon acts as a statement delimiter. The Python interpreter knows that the statement ends after the semicolon and a new one begins.
When Use Semicolon in Python?
Semicolons offer a smooth landing for those with backgrounds in C or C++. This is because you can freely add semicolons at the end of each line in Python. But remember that Python doesn’t require using semicolons and you almost never see it in use.
Later on, you’ll learn why using semicolons is bad and creates hard-to-read code that is tricky to manage and understand. Before that, let’s take a look at some rare scenarios in which you might need a semicolon in Python.
Use Case 1: Running Shell Scripts
A great use case for semicolons in Python is when you need to run a short Python script from the shell.
For example, you can open up your command line window and run the following command:
$ python -c 'for i in range (4): print ("Hi") ; print(f"The number is {i}")'
This results in the following:
Hi This is the number 0 Hi This is the number 1 Hi This is the number 2 Hi This is the number 3
As you might expect, adding the statements in the same line is the only easy way to run a Python script like this on the shell window. This is why it’s handy to have a semicolon in Python as an option.
Use Case 2: Suppressing Output in Jupyter Notebook
If you work with an environment like Jupyter Notebook, you may have noticed that the last expression of your script prints the return value. Sometimes, this can be annoying if you don’t need to see the return value.
%matplotlib inline import random import pandas as pd dat = [random.gauss(10, 2) for i in range(150) ] df = pd.DataFrame( { 'C': dat} ) axis = df.C.hist() axis.set_title('Histogram', size=10)
Output:
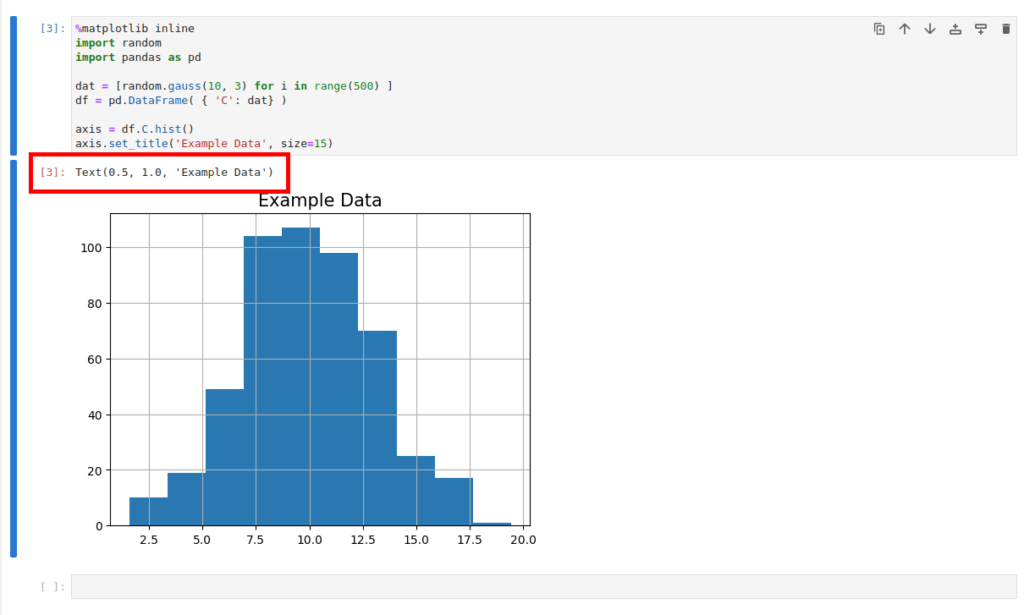
Notice how it shows a print Text(0.5, 1.0, ‘Example Data’) before the example histogram. This is the return value of the axis.set_title(‘Example data’, size=15) function call at the last line. If you don’t want to see this, you need to suppress the function call with a semicolon.
axis.set_title('Example Data', size=15);
Now the result looks less annoying as there are no random prints before the plot.
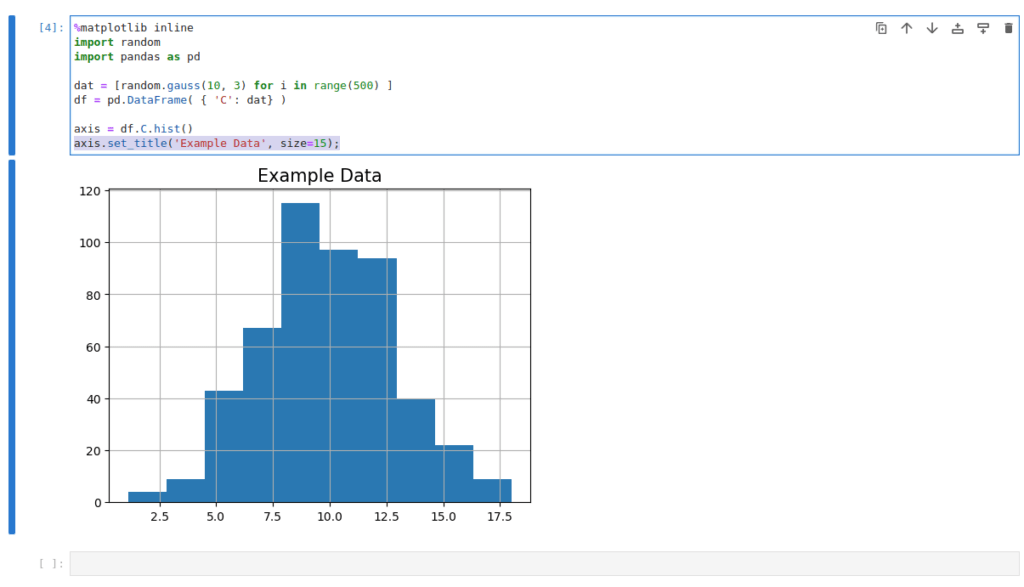
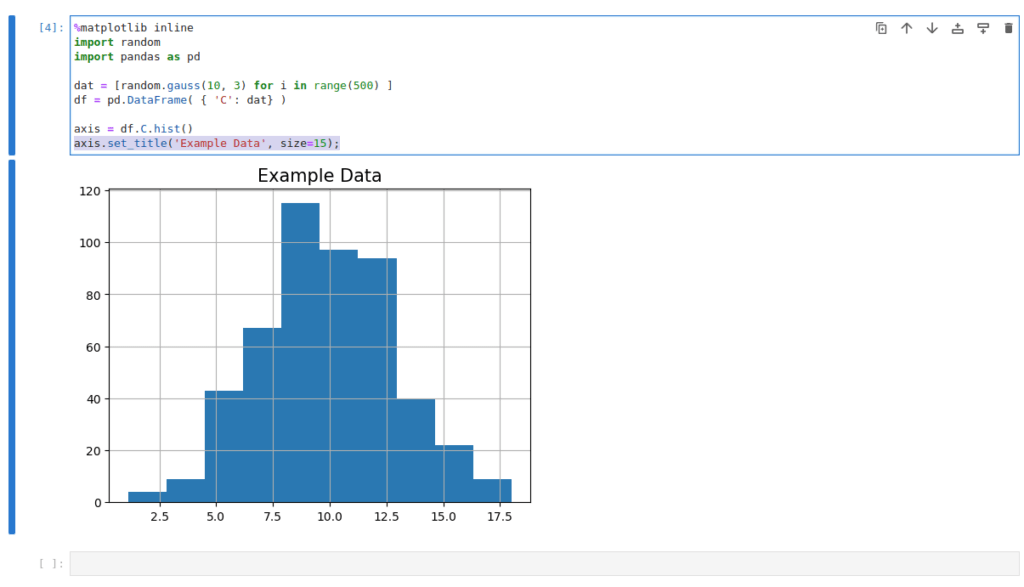
Stop Using Semicolons in Python
If you have a background in JavaScript, C++, or C, you might be familiar with adding semicolons to each line of code to terminate statements.
In Python, you also have the option to terminate code lines with semicolons. However, this is not mandatory like in C or C++. As a matter of fact, you should stay away from using semicolons in Python.
The reason why semicolons are bad in Python is that they are not Pythonic.
If you force multiple statements on the same line, it only makes the code harder to read.
For example, let’s create a simple piece of code with some variables and prints:
temperature = 45 cold = False if temperature < 50: cold = True print('Its a cold day') print('I need more clothes') print(f'cold={cold}') print('Status changed')
This code expands to multiple lines and is readable (even though it doesn’t do anything particularly smart).
Now, let’s delete some line breaks with semicolons in the above example:
temperature = 45; cold = False if temperature < 50: cold = True; print('Its a cold day'); print('I need more clothes') print(f'cold={cold}');print('Status changed')
This piece of code looks terrible and unreadable.
To develop programs at scale, you need to write code that is easy to read. Combining expressions that belong to their own lines into the same line makes no sense. It only saves you lines but makes the code so much harder to read and maintain.
Conclusion
Today you learned what a semicolon does in python.
The semicolon delimits statements. This makes it possible to add multiple statements in one line by semicolon-separating them.
But using semicolons to add multiple statements on the same line is highly against best practices. Adding multiple expressions in the same line makes the code less readable and harder to maintain.
Unless you’re suppressing Jupyter Notebook prints or running a shell script with multiple expressions, you should stay away from the semi-colon in Python.
Thanks for reading. I hope you enjoy it.
Happy coding!