Frozen sets in Python are sets that cannot be changed after creation. In addition, they cannot contain duplicate values. In this sense, a frozen set has the properties of a tuple and set.
To create a frozen set, wrap a set around a frozenset()
call:
constants = frozenset({3.141, 2.712, 1.414})
Let’s take a bit closer look at the frozen sets in Python and what problem they solve.
What Problem Do Frozen Sets Solve
Python’s frozen set is like a combination of a tuple and a set.
To fully understand the problem frozenset
solves, you need to first learn what is a set and what is a tuple in Python.
What Is a Set in Python
In Python, a set is an unordered, mutable collection of unique items.
A set has the following characteristics:
- Unique values. You cannot have duplicates in a set.
- Unordered collection. There is no order in the set.
- Mutable. You can modify the set by adding and removing values.
- Supports mathematical set operations, such as
union
,difference
,intersection
.
Here is some basic usage of a set.
>>> nums = {1, 7, 3, 2, 2, 2, 2} >>> nums.add(10) >>> nums {1, 2, 3, 7, 10} >>> nums.remove(7) >>> nums {1, 2, 3, 10} >>> len(nums) 4
Generally, you should use a set to carry out mathematical set operations in Python.
To review other features of sets, check out this article.
What Is a Tuple in Python
A tuple is an ordered, immutable data type. This means:
- You cannot modify a tuple after creation.
- The items are in an order.
- You can have duplicate values.
Here is an example of how to create a set and do some basic operations with it:
>>> nums = (1, 3, 7, 10, 10) >>> nums (1, 3, 7, 10, 10) >>> len(nums) 5 >>> nums[0] 1
Generally, you should use a tuple if you want a group of values that are not supposed to change.
For a more comprehensive guide on tuples in Python, check out this article.
Tuple + Set = Frozenset
A frozen set in Python is like a combination of tuples and sets. A frozen set cannot be modified and does not accept duplicates. It can be used to carry out mathematical set operations.
A frozen set has the following characteristics:
- Unique collection. No duplicate values are allowed.
- Unordered. The notion of order is meaningless in a frozen set.
- Immutable. You cannot change a frozen set after creation.
- Supports (immutable) set operations, such as
union
,difference
,intersection
.
There is no special syntax for creating a frozen set. Instead, you need to wrap a set around a frozenset()
function call.
For example:
names = frozenset({"Alice", "Bob", "Charlie"})
Frozen Set Methods in Python
The frozen set in Python supports the basic set operations.
1. difference
You can compute the difference between two frozen sets using the difference()
method.
Here is an illustration:
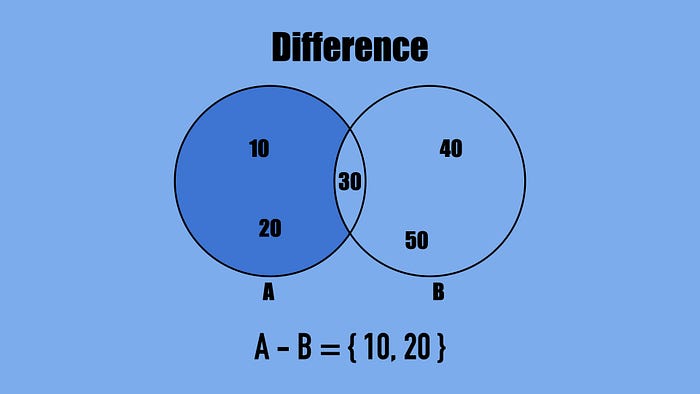
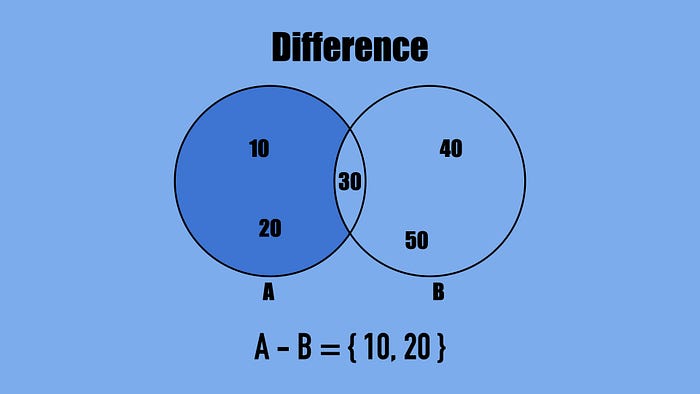
And here is the code:
A = frozenset({10, 20, 30}) B = frozenset({30, 40, 50}) print(A.difference(B))
Output:
frozenset({10, 20})
2. intersection
You can compute the intersection between two frozen sets using the intersection()
method.
Here is an illustration:


Here is an example:
A = frozenset({10, 20, 30}) B = frozenset({30, 40, 50}) print(A.intersection(B))
Output:
frozenset({30})
3. union
You can compute the union between two frozen sets using the union()
method.
Here is an illustration:


And here is the code:
A = frozenset({10, 20, 30}) B = frozenset({30, 40, 50}) print(A.union(B))
Output:
frozenset({50, 20, 40, 10, 30})
4. symmetric_difference
The symmetric difference between two sets is the opposite of the intersection between them.
In other words, the symmetric difference is the set with values in either set A or B, but not in both.
For example:
A = frozenset({30, 40, 50}) B = frozenset({30, 40, 10}) print(A.symmetric_difference(B))
Output:
frozenset({10, 50})
5. isdisjoint
Two (frozen) sets are disjoint if they have no common elements.
To check if two frozen sets are disjoint, use the isdisjoint()
method.
For instance:
A = frozenset({10, 20, 30}) B = frozenset({30, 40, 50}) print(A.isdisjoint(B))
Output:
False
These frozen sets are not disjoint as they have one common element—the number 30.
6. issubset
A (frozen) set is a subset of another (frozen) set if all the elements of the set are present in another.
For example:
A = frozenset({30, 40}) B = frozenset({30, 40, 50}) print(A.issubset(B))
Output:
True
The result is True
because all the elements of set A are found in set B.
7. issuperset
To check if a set has all the elements of another, use the issuperset(
) method.
For example:
A = frozenset({30, 40, 50}) B = frozenset({30, 40}) print(A.issuperset(B))
Output:
True
The result is True
because all the elements of set B are found in set A.
8. copy
To create a copy of a frozen set, use the copy()
method.
For example:
A = frozenset({30, 40, 50}) B = A.copy() print(B)
Output:
frozenset({40, 50, 30})
Length of a Frozenset
You can use the built-in len()
function to check how many elements there are in a frozen set.
For example:
A = frozenset({30, 40, 50}) print(len(A))
Output:
3
How to Loop Through a Frozenset in Python
A frozenset
is an iterable object in Python. This means it is possible to loop through it with a for loop. This looks similar to how you would loop through a set or a list in Python.
For instance:
A = frozenset({1, 2, 3, 6}) for number in A: print(number)
Output:
1 2 3 6
When Use Frozen Sets in Python
As mentioned earlier, a frozen set is like a combination of a tuple and a set.
You can use it if you need to perform set operations but don’t want to modify the contents of the set.
One benefit of using a frozenset
is to make your intention clear for other developers—this set of values should not be touched!
Conclusion
A frozen set in Python is a set of unique and unchangeable values.
A frozen set:
- Cannot be modified after creation.
- Cannot contain duplicate values.
- Can be used to perform mathematical set operations.
A frozen set is like a combination of a tuple and a set.
You can create one by calling frozenset()
on a set:
constants = frozenset({3.141, 2.712, 1.414})
Thanks for reading. I hope you liked it.