To efficiently deal with dataframes and lists in R, you need to be able to access the columns or values in an easy and readable way. This is where you can use the dollar sign operator ($). This operator retrieves the value or column by a name after which you can easily read, modify, or remove the accessed objects.
This is a comprehensive guide to using the dollar sign operator $ in R. You will learn what the $ operator does and what are its main use cases for both lists and dataframes.
What Is $ in R?
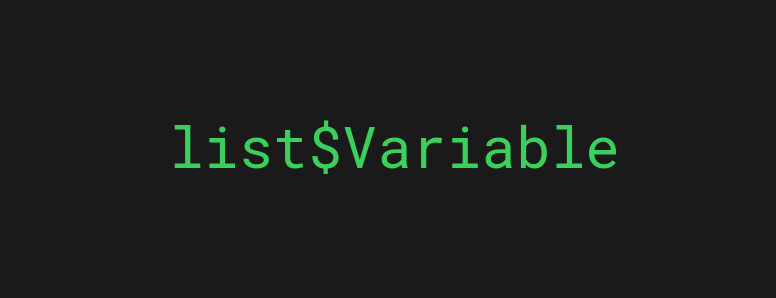
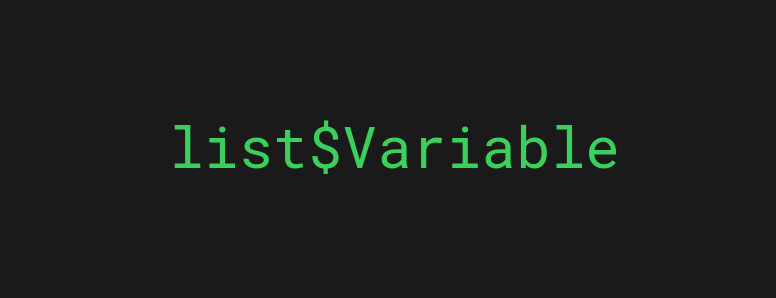
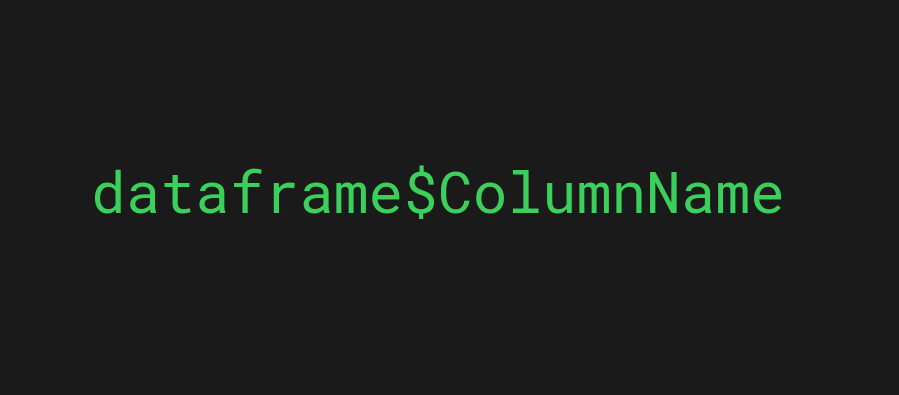
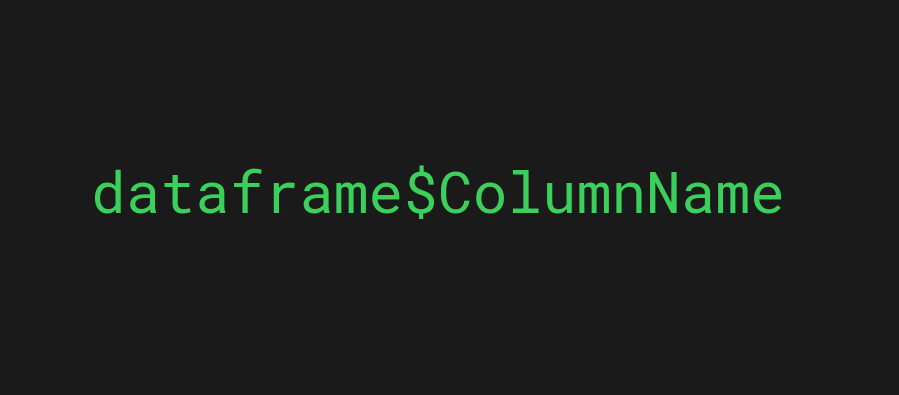
In R, one of the fundamental operators is the dollar sign operator ($). With the $ operator, you can access a specific part of data. The most notable use cases for the $ operator are related to lists and dataframes:
- With lists, you can use the $ operator to access a particular variable of the list.
- With dataframes, you can use the $ operator to access a specific column.
The $ operator in R gives you easy access to a subset of data. This makes it easy to access, modify, and remove parts of your data.
Let’s take a look at the most common use cases and examples for the $ operator.
6 Use Cases for $ in R
The main use cases for the $ in R are associated with lists and dataframes. This section is split into two parts:
-
Using the $ operator with lists
- Select a variable from a list
- Add a new variable to a list
- Select a variable with white spaces
-
Using the $ operator with dataframes
- Select a column in a dataframe
- Add a new column to a dataframe
- Remove a column from a dataframe
Let’s jump into it!
Using $ on Lists
Lists are a common data type in R. You can use the $ operator to access, modify, and remove list values.
1. Select a Variable on a List
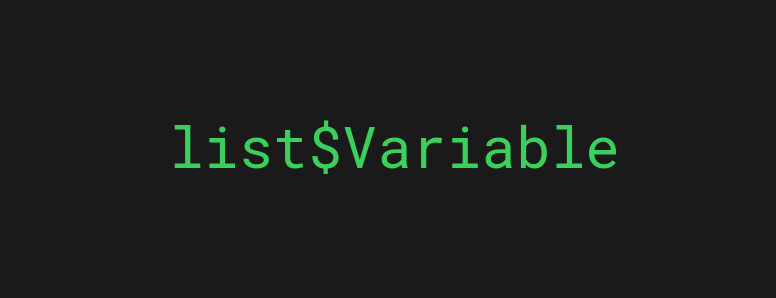
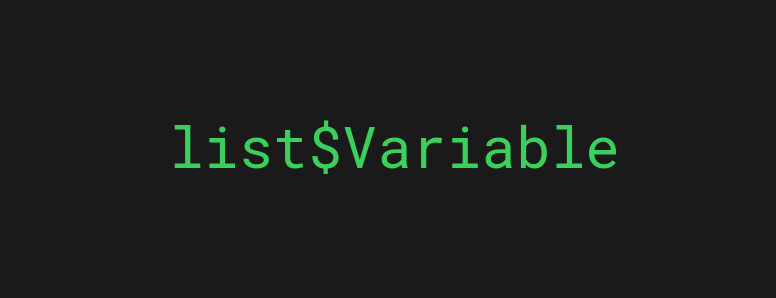
The most basic usage of the $ operator in R is to access a variable in a list. To do this, add a $ after the list name and specify the variable name you’re interested in.
list$Variable
For example, given a list of names associated with ages, let’s access the age of “Bob”:
ages <- list('Alice'=30, 'Bob'=25) ages$Bob
Output:
[1] 25
2. Add New Variable to a List
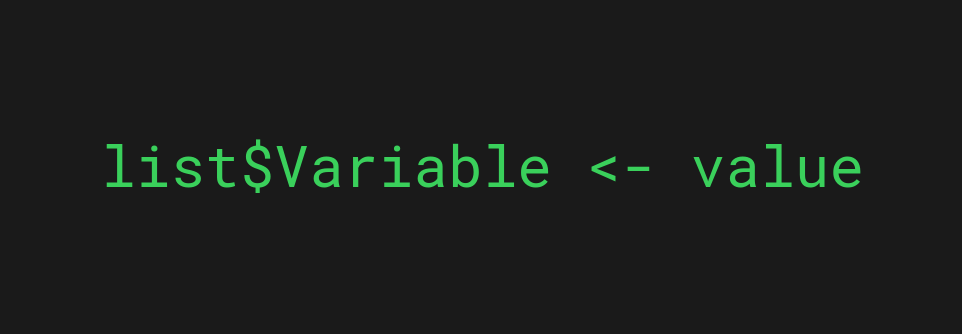
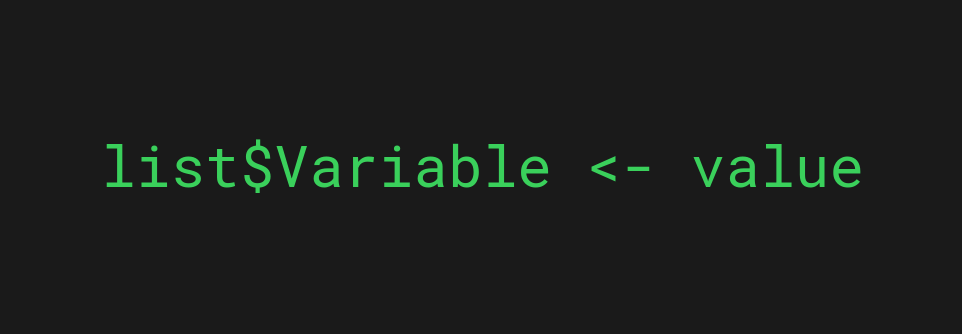
In the previous section, you learned how to access a variable in a list using the $ operator.
Adding a new variable to a list happens in a similar fashion. But instead of accessing a variable, you need to specify the name of the new variable after the dollar sign.
For example, let’s add a new variable Charlie=32 to the list of names and ages:
ages <- list('Alice'=30, 'Bob'=25) ages$Charlie <- 32 ages
Output:
$Alice [1] 30 $Bob [1] 25 $Charlie [1] 32
Now there are three names each associated with age in the list.
Notice that you can also update an existing value in a list with the $ operator.
For example, let’s update the age of “Alice”:
ages <- list('Alice'=30, 'Bob'=25) ages$Alice <- 60 ages
Output:
$Alice [1] 60 $Bob [1] 25
3. Select an Object with White Spaces
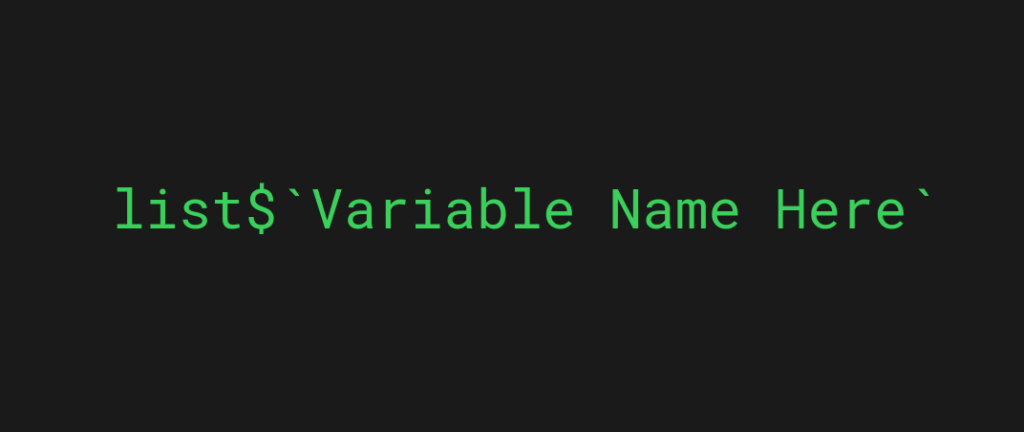
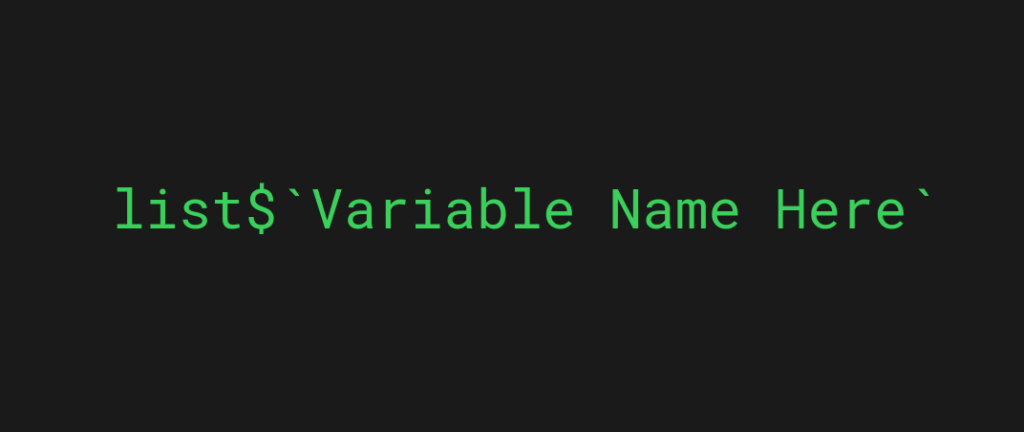
Accessing a list variable with names that contain blank spaces is a bit trickier. This is because blank spaces are a no-go in R (similar to other programming languages) as they mess up the compiler.
For example:
ages <- list('Alice Smith'=30, 'Bob Jones'=25) ages$Bob Jones
Output:
[1] Error: unexpected symbol in "ages$Bob Jones" Execution halted
Here the blank space between Bob Jones caused the R compiler to think that Jones is an invalid and unrelated symbol that is there by accident.
But because the list has a variable with the name Bob Jones, this is not a mistake. To fix the issue with variables that have blank spaces, wrap the name around backticks after the $ operator.
For example:
ages <- list('Alice Smith'=30, 'Bob Jones'=25) ages$`Bob Jones`
Output:
[1] 25
Now the compiler successfully knows that it should look for a multi-part variable name in the list.
Notice that a better approach is to name the columns of the table so that there would be no blank spaces! If your table has columns with blank spaces, to begin with, you can replace the blank spaces with something else, such as underscores.
Using $ on Dataframes
Another main use case for the $ operator is when dealing with dataframes. Similar to lists, the $ operator makes it easy to access, modify, and remove columns in the dataframe.
This section shows you how to use the $ operator on data frames.
In the following sections, you are going to work with the following data frame:
shopping_list = data.frame(PRODUCT_GROUP = c("Fruit","Fruit","Fruit","Fruit","Fruit","Vegetable","Vegetable","Vegetable","Vegetable","Dairy","Dairy"), PRODUCT_NAME = c("Banana","Apple","Mango","Orange","Papaya","Carrot","Potato","Cucumber","Tomato","Milk","Yogurt"), Price = c(1,0.8,0.7,0.9,0.7,0.6,0.8,0.75,0.15,0.3,1.1), Tax = c(NA,NA,24,3,20,30,NA,10,NA,12,15))
This dataframe is a table of products that looks like this when printed out:
PRODUCT_GROUP PRODUCT_NAME Price Tax 1 Fruit Banana 1.00 NA 2 Fruit Apple 0.80 NA 3 Fruit Mango 0.70 24 4 Fruit Orange 0.90 3 5 Fruit Papaya 0.70 20 6 Vegetable Carrot 0.60 30 7 Vegetable Potato 0.80 NA 8 Vegetable Cucumber 0.75 10 9 Vegetable Tomato 0.15 NA 10 Dairy Milk 0.30 12 11 Dairy Yogurt 1.10 15
Let’s jump into it!
4. Select a Column
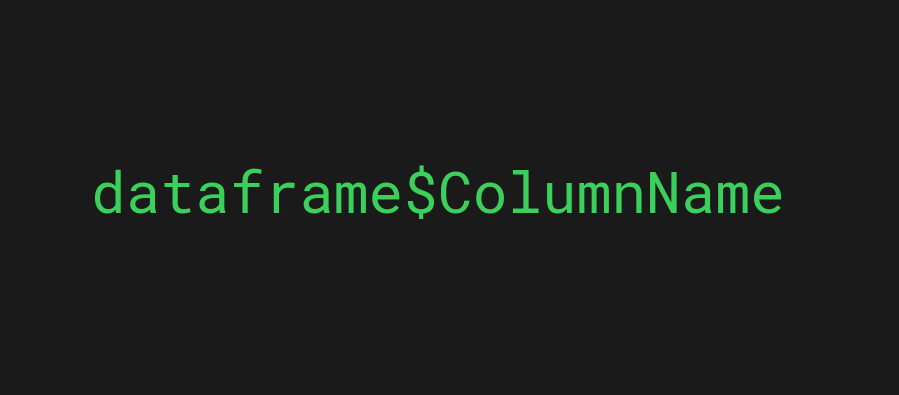
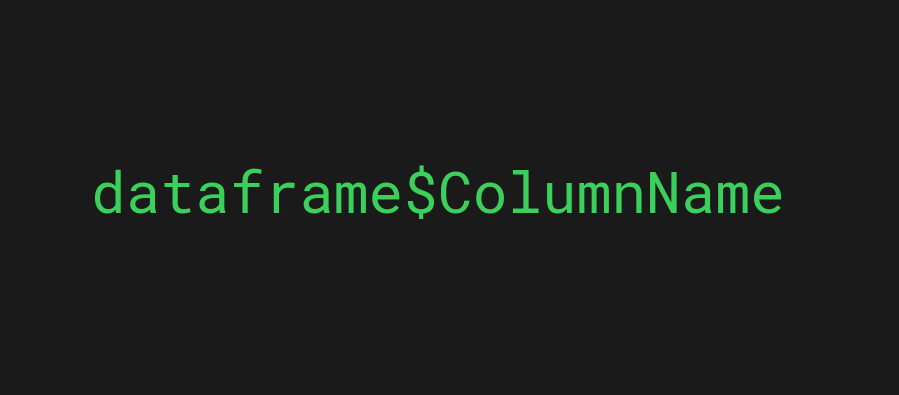
Selecting a particular column in a dataframe is easy with the $ operator. All you need to do is specify the name of the dataframe, followed by the $ operator and the name of the specific column you’d like to access.
For example, let’s access the PRODUCT_NAME column in the shopping_list dataframe:
shopping_list$PRODUCT_NAME
Output:
[1] "Banana" "Apple" "Mango" "Orange" "Papaya" "Carrot" [7] "Potato" "Cucumber" "Tomato" "Milk" "Yogurt"
The result is the names of the individual products.
Notice how the idea of accessing a column in a dataframe is exactly the same as accessing a variable in a list.
5. Add a New Column to a Dataframe
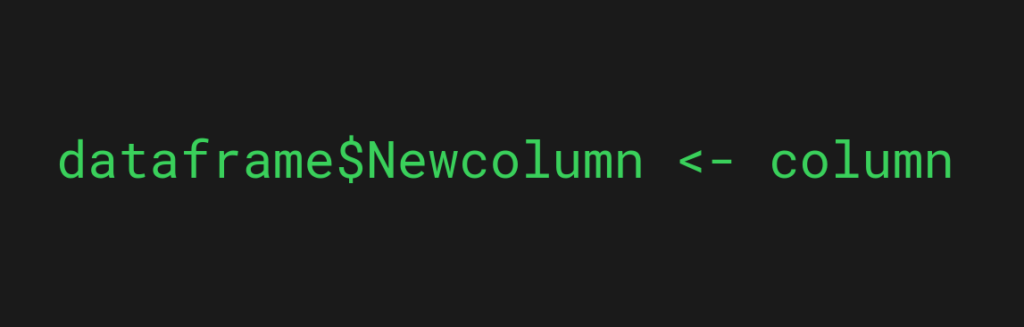
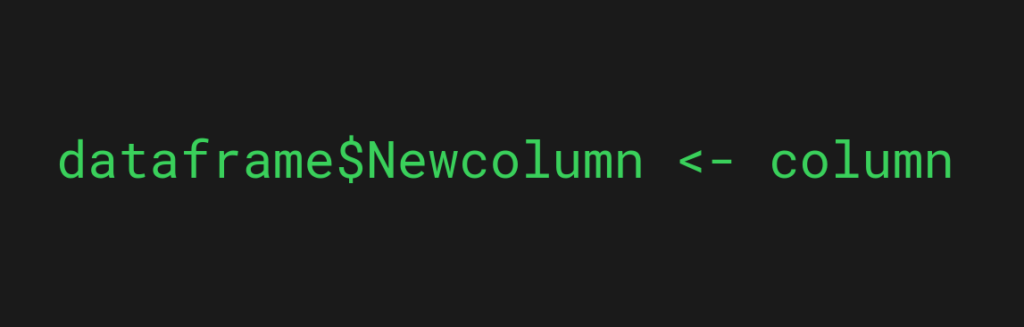
To add a new column to a dataframe, use the $ operator as an accessing operator by specifying the new column name to it.
The idea is the same as adding a new variable to a list with the $ operator.
For example, let’s add a new column, “Available” to the shopping_list and make it default to the value “Yes“.
shopping_list$Available <- rep('Yes', length(shopping_list$PRODUCT_GROUP)) shopping_list
Output:
PRODUCT_GROUP PRODUCT_NAME Price Tax Available 1 Fruit Banana 1.00 NA Yes 2 Fruit Apple 0.80 NA Yes 3 Fruit Mango 0.70 24 Yes 4 Fruit Orange 0.90 3 Yes 5 Fruit Papaya 0.70 20 Yes 6 Vegetable Carrot 0.60 30 Yes 7 Vegetable Potato 0.80 NA Yes 8 Vegetable Cucumber 0.75 10 Yes 9 Vegetable Tomato 0.15 NA Yes 10 Dairy Milk 0.30 12 Yes 11 Dairy Yogurt 1.10 15 Yes
If you’re wondering what this part does: rep(‘Yes’, length(shopping_list$PRODUCT_GROUP)), it’s just to make the new column as long as the PRODUCT_GROUP column in the table.
6. Delete a Column
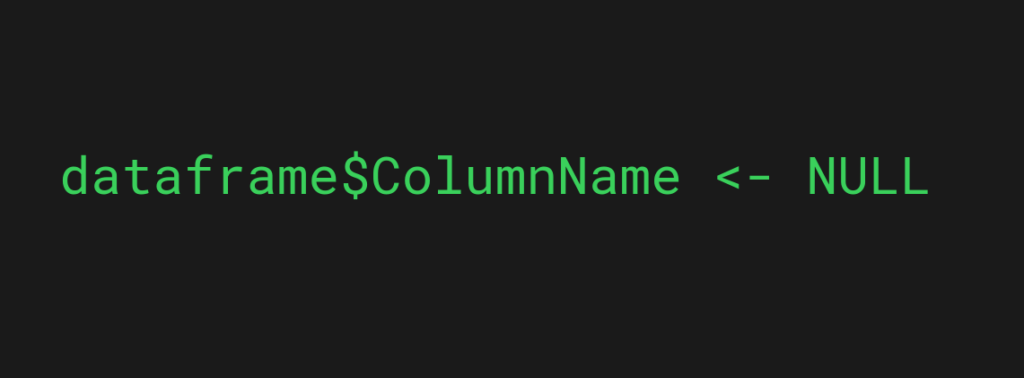
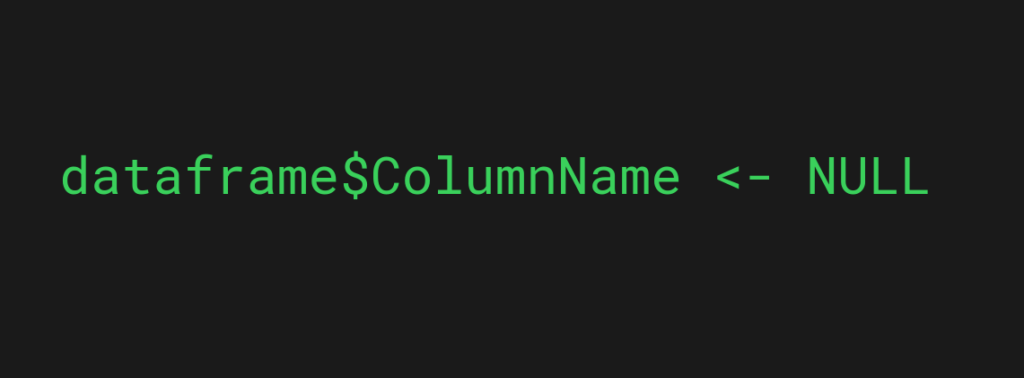
Deleting a specific column from a dataframe is possible by using the $ operator and the NULL object. To remove a column, access it with the $ operator and assign a NULL value to it.
For example, let’s remove the “Tax” column from the shopping_list table:
shopping_list$Tax <- NULL shopping_list
Output:
PRODUCT_GROUP PRODUCT_NAME Price 1 Fruit Banana 1.00 2 Fruit Apple 0.80 3 Fruit Mango 0.70 4 Fruit Orange 0.90 5 Fruit Papaya 0.70 6 Vegetable Carrot 0.60 7 Vegetable Potato 0.80 8 Vegetable Cucumber 0.75 9 Vegetable Tomato 0.15 10 Dairy Milk 0.30 11 Dairy Yogurt 1.10
Summary
Today you learned how to use the $ operator in R.
To recap, you can use the $ operator in R to access columns of dataframes or variables of a list. The $ operator makes it easy to access, modify, and delete values from dataframes and lists.
Thanks for reading. Happy coding!