One of the main benefits of writing computer programs is to automate tasks based on inputs and conditions. Adding logic to code is possible through decision-making if…else statements.
For example, a square root function should only compute the square root of an input if its value is positive. Otherwise, the function should warn about false input.
This type of decision-making logic is possible in R by using the if…else statements. And by the way, if you’re new to programming, if…else statements are present in all other programming languages too.
This is a comprehensive guide to if…else statements in R.
The guide teaches you theory about if…else statements, nested if…else statements, if…else’s with multiple conditions, and more. All the theory is backed up by great and illustrative examples.
Let’s jump into it!
if…else Statements in R
One of the programming fundamentals is decision-making. Based on the input, the program should choose which path to choose. In R, you can implement decision-making logic to your code by using the if…else statements. Before showing the syntax, here’s the flowchart of an if…else statement:
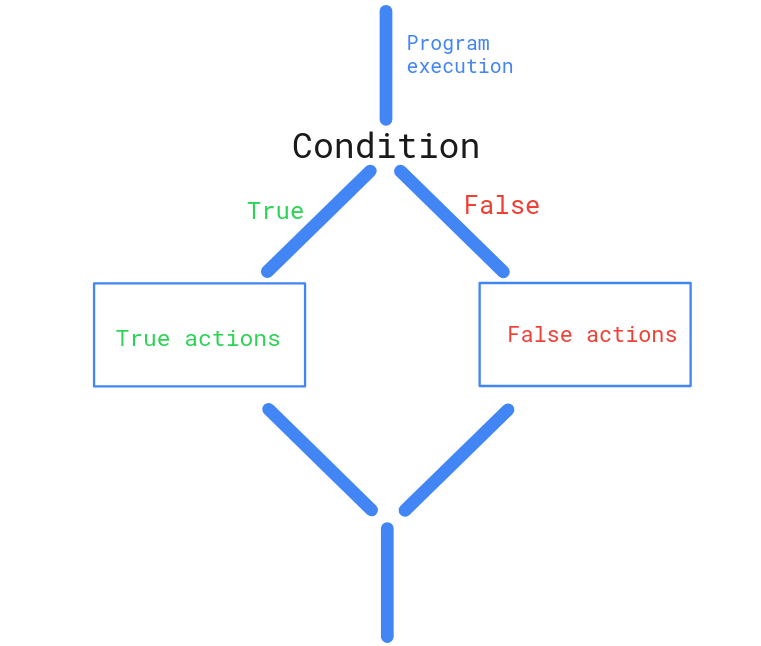
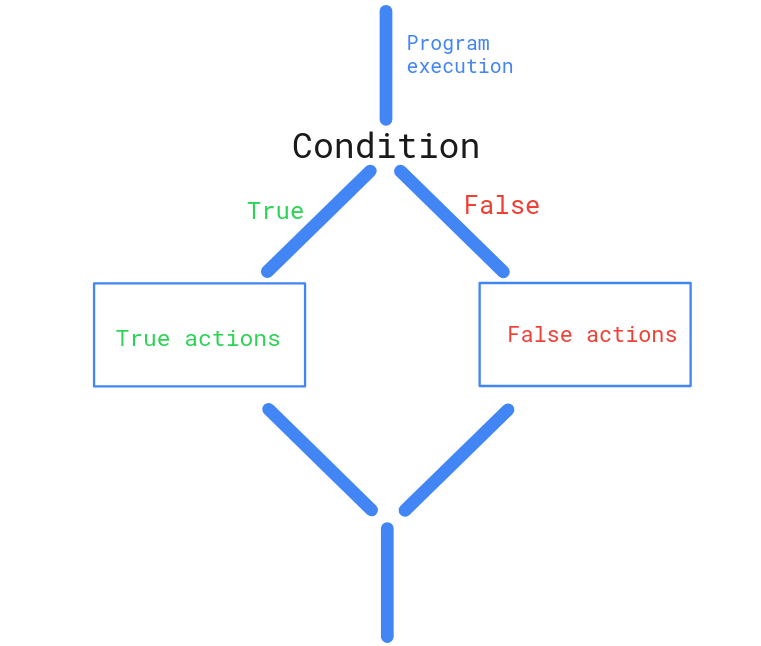
The idea is straightforward. Once an R program encounters an if…else statement, the program checks if a condition is TRUE or FALSE.
- If the condition is TRUE, the program takes actions associated with a true condition.
- If the condition is FALSE, the program runs different actions associated with a false value.
After this, the program continues execution normally.
In R, the if…else statement syntax is as follows:
if (condition) { statements1 } else { statements2 }
Let’s take a closer look at the parts of this statement:
- The condition is a boolean value that is either TRUE or FALSE.
- If the condition evaluates TRUE, statements1 will be executed. There can be as many lines of code inside the if…else statement.
- If the condition evaluates FALSE, statements2 will be executed.
Notice that the condition can be any valid R expression that produces a boolean value. For example 1 < 2.
The best way to learn how the if…else statements work in R is via examples.
Let’s see a basic example of a program that checks if a person’s age is enough to drive:
age = "19" if (age < 18) { print("You cannot drive") } else { print("You can drive") }
Output:
[1] "You can drive"
The above program first checks if the age is less than 18. Because age < 18 evaluates to FALSE, the if…else statement executes the code in the else branch, which prints out “You can drive”.
Make sure to play with the above code and change the condition or age to get a feel for how the if…else statements behave in R.
Now you have a basic understanding of how the if…else statements work in R.
if…else Statements with Multiple Conditions
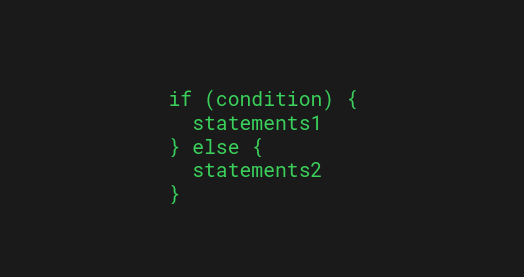
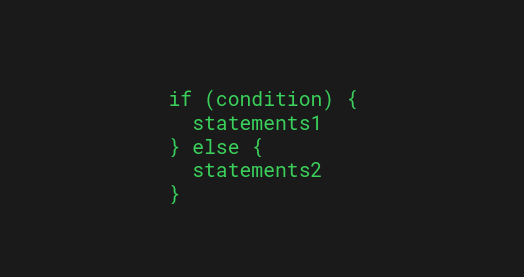
More often than not, an if…else statement has to check whether multiple conditions hold or not.
This is when you can use logical operators (&& for AND, || for OR) to combine multiple conditions.
For example, let’s only allow a person to drive a car if they are older than 18 and have a car:
age = "19" has_car = TRUE if (age > 18 && has_car) { print("You can drive") } else { print("You cannot drive") }
Output:
[1] "You can drive"
Here the condition age > 18 && has_car has two parts; age > 18 and has_car. This entire condition can only be TRUE if both age > 18 and has_car evaluate TRUE.
if…elseif…else Statements in R
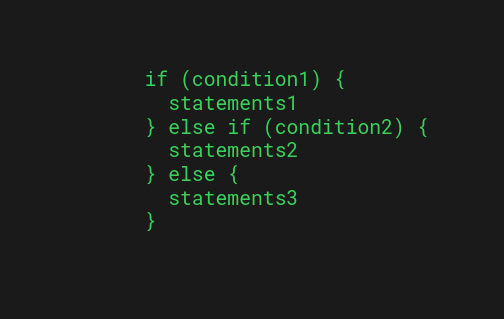
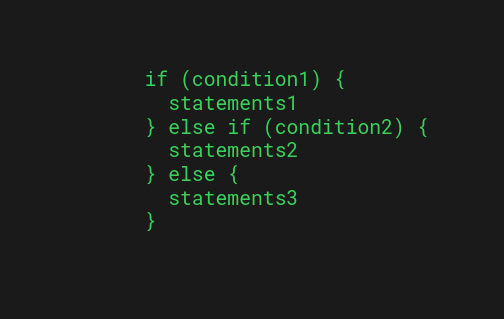
It’s quite common for a program to have more than two actions to run in a decision-making context. For example, if a game program rolls dice, there are six different outcomes and for each outcome, there is a different action.
With a traditional if…else statement, you can only choose between two actions based on the condition. But to add more actions, you need to add more “condition checks” in the code.
This is possible by using the if…elseif..else statements.
Here’s the basic syntax for the if…elseif…else statement in R:
if (condition1) { statements1 } else if (condition2) { statements2 } else { statements3 }
The above statement works exactly like the if…else statement you learned earlier, but this time there is one more condition to check (and action to run).
If the first condition1 is FALSE, the program checks if condition2 is TRUE. If both condition1 and condition2 are FALSE, then the statements3 in the else block gets executed.
You can add as many consecutive else if blocks into an if…else statement as you want to.
Once again, the best way to learn how to use the if…elseif…else statement is by seeing some examples.
For instance, let’s build a simple R program that checks if you own a car and if you are old enough to drive so that:
- If you are 18 or older and have a car –> You can drive.
- If you are 18 or older but don’t have a car –> You could drive if you had a car.
- If you are less than 18 years old and have a car –> You could drive if you were old enough.
- Otherwise (younger than 18 and no car) –> You cannot drive.
Here’s how it looks in the code:
age = "19" has_car = FALSE if (age >= 18 && has_car) { print("You can drive") } else if (age >= 18 && !has_car) { print("You could drive but don't have a car") } else if (age < 18 && has_car) { print("You could drive if you were old enough") } else { print("You cannot drive") }
Output:
[1] "You could drive but don't have a car"
Once again, make sure to play with the above code and change conditions and the age and the has_car variables to see how it affects the output.
‘if’ Statements without ‘else’ in R
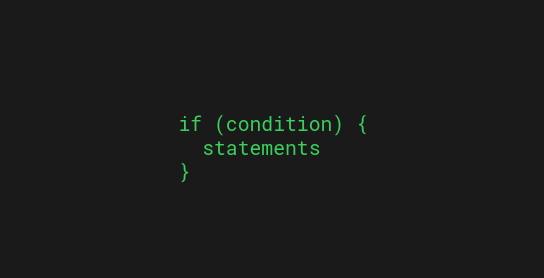
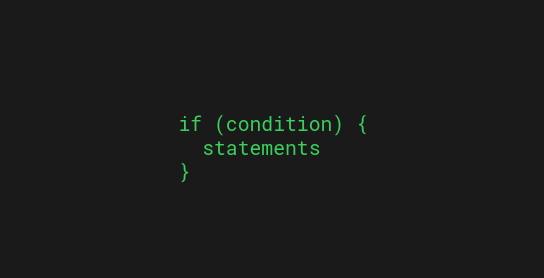
Sometimes you might only need to check if a condition holds and do nothing if it doesn’t.
In this case, you can use the if statement without the else part.
if (condition) { statements }
When R encounters an if statement, it only performs the code inside the if block if the condition is TRUE. If it’s not, the code continues running without taking any action.
Once again, let’s take a look at an example.
For example, let’s create a program that asks a person to leave a coat in the lobby in case they’re wearing one.
has_coat = TRUE if(has_coat){ print("You can leave your coat in the lobby.") } print("Welcome to the restaurant!")
Output:
[1] "You can leave your coat in the lobby." [1] "Welcome to the restaurant!"
If the person entering the restaurant doesn’t have a coat, they will only receive a warm welcome. But if they do have a coat, the waiter asks them to leave it in the lobby.
Let’s see another example where a function only sums up positive values. Notice that you need to be familiar with functions in R to understand this one!
sum_positives <- function(a, b){ if (a < 0 || b < 0) { return("Invalid input") } a + b } res1 = sum_positives(1, 5) res1 res2 = sum_positives(-1, 14) res2
Output:
[1] 6 [1] "Invalid input"
The first call succeeds because both input arguments are positive. But the second call fails because one of the arguments is negative.
This is a great example of using an if statement in R. If the input isn’t valid, there’s no reason to run the computation.
Calling if…else for Vectors in R
A typical R program operates on vectors. You have an input vector and a function that spits out an output vector.
To perform if…else checks for each vector element in R, you would need to combine if…else statement and a for loop, which is slow and inconvenient.
This is why there’s a built-in ifelse function in R. This function makes it convenient to call if…else for each vector element.
For example, here’s how you can use the ifelse function to check whether the elements of a vector are odd or even.
a = c(1, 2, 3, 4, 5, 6, 7) ifelse(a %% 2 == 0, "Even", "Odd")
To learn more about the ifelse function in R, make sure to read my complete guide.
Summary
Today you learned how if…else statements work in R.
As a recap, adding logic to your R code is possible through if…else statements. With the introduction of if…else, you can add logic to your code to perform specific actions with particular criteria.
There are some variations of if…else statements in R:
- The if…else statements for taking actions based on whether a condition is TRUE or FALSE.
- The if…elseif…else statements for taking more than two actions in different scenarios.
- The if statement without else for checking a single condition and takes action only if it’s TRUE.
Thanks for reading! Happy coding!