To copy a file in Python, use the shutil module’s copyfile() function.
from shutil import copyfile copyfile(src, dst)
This piece of code copies the contents of the file src to a destination file dst.
Both src and dst are strings that represent the names of the files (or rather, paths to the files).
If a file dst does not exist, a new file is created. If it does, the file behind that path is overridden.
Real-Life Example of How to Copy a File with Python
To understand how to copy a file in Python, you need to see an example.
Let’s start with a setup like this, where you have two files Example1.txt and example.py in the same folder on your computer:
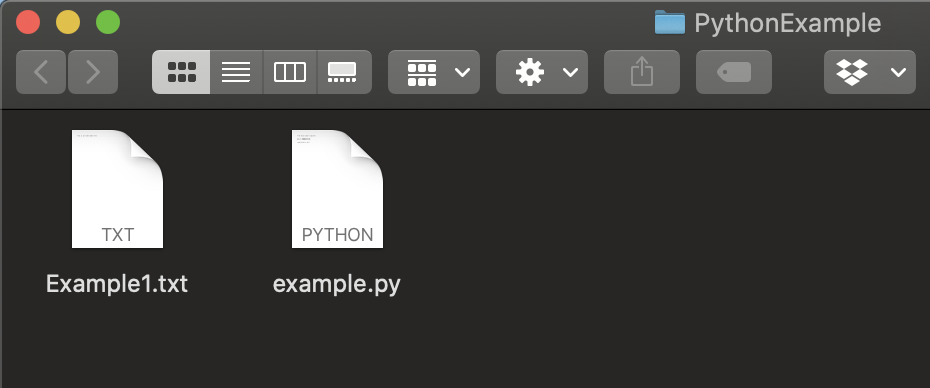
The example.py is a program that copies the file called Example1.txt to a new file called Example2.txt.
Because you are working in the same folder, you do not need to worry about the full paths of the files.
All you need is the names of the files.
Here is the code:
from shutil import copyfile src = 'Example1.txt' dst = 'Example2.txt' copyfile(src, dst)
After running this piece of code, you should see a file called Example2.txt appear in the same folder:
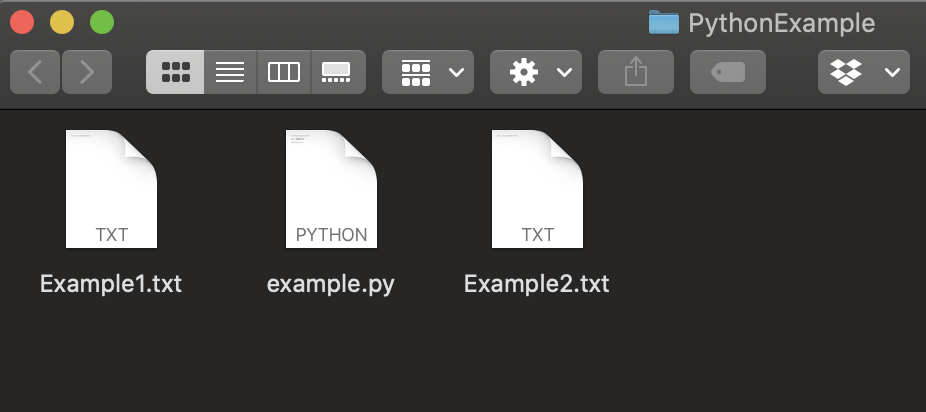
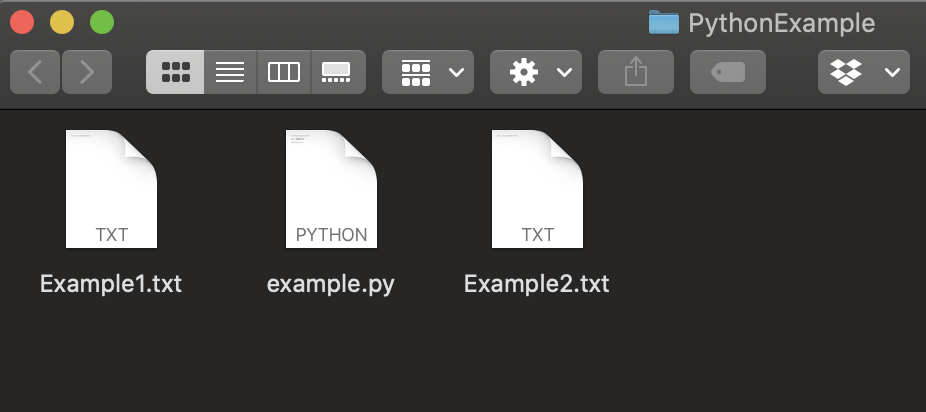
This is how easy it is to copy a file using Python.
How to Copy a File to Different Folder
More often than not your files are not going to be in the same folder.
If this is the case, accessing the files with their names is not possible.
If you want to copy a file from one folder to another, you need to specify the path of the source and the destination.
The path obviously depends entirely on your file setup.
Let’s see a simple and demonstrative example.
Let’s say you have a folder called Project. Inside this folder, you have three subfolders called Scripts, Images, and Favorites.
- Inside the Scripts folder, you have a file called code.py. This is a program file that copies an image called zebra.png from Images to Favorites.
Here is an illustration of the folder structure:
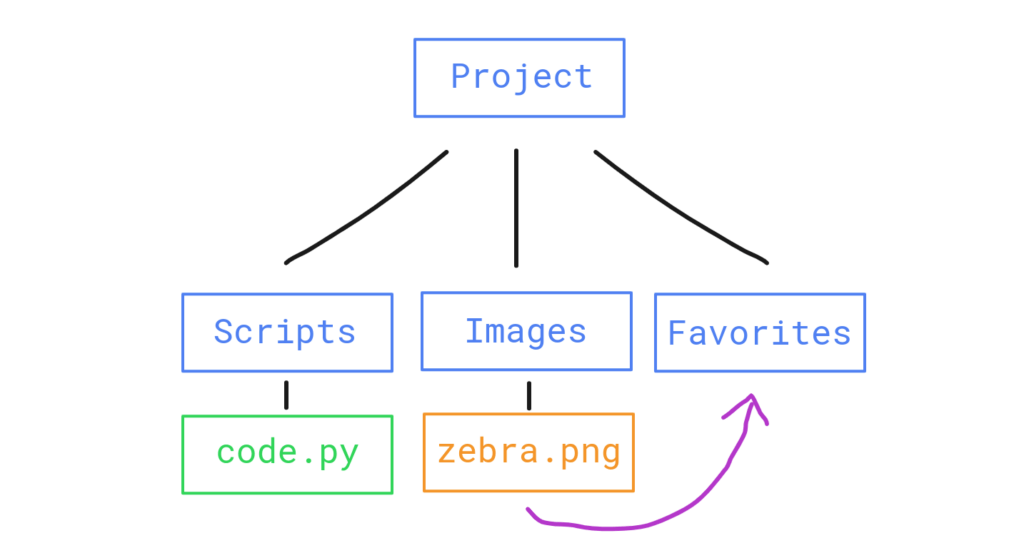
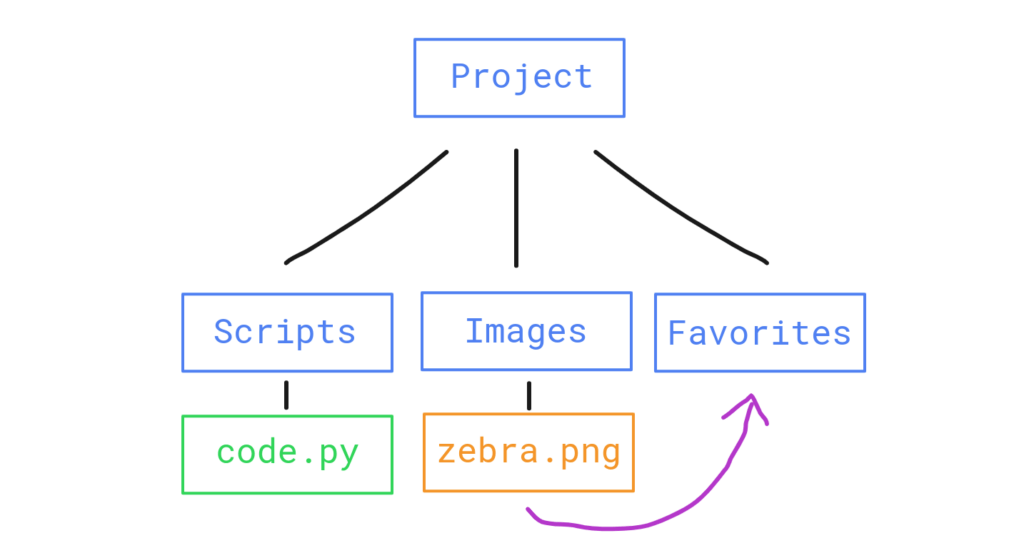
Because the code file, the source file, and the destination all lie in different folders, you need to work with paths instead of file names.
Here is how the code.py file looks in the Scripts folder
from shutil import copyfile src = '../Images/zebra.png' dst = '../Favorites/zebra.png' copyfile(src, dst)
Let’s examine the paths to understand how they work.
The ../ refers to the parent folder. In the case of the Scripts folder, this refers to the Project folder in which the Images and Favorites folders live.
The parent folder has to be specified because otherwise, the code.py script would search inside the Scripts folder which has no other files except for the code file.
Inside the Project folder, accessing the subfolders Images and Favorites is trivial:
- Images/zebra.png is the path to the image.
- Favorites/zebra.png is the desired path to the copied file.
Conclusion
Today you learned how to copy a file in Python using the shutil module’s copyfile() function:
from shutil import copyfile copyfile(src, dst)
Where:
- src is the path of the file to be copied as a string.
- dst is the destination file’s path as a string.
In case the source file and the destination file lie in the same folder, it is enough to use the names of the files.
Thanks for reading. Happy coding!