In Python, you can read a JSON file by using the json.load()
function.
Here is a quick example in case you’re in a hurry:
import json with open('PATH/TO/YOUR/FILE.json') as file: data = json.load(file)
I’m sure this quick example might already be a great enough answer for you.
But in case you’re not too familiar with JSON or Python yet, I highly recommend reading along. This is a comprehensive guide to reading JSON files in Python.
If you’re completely unfamiliar with JSON, make sure to read my complete guide to JSON data.
Example of Reading a JSON File in Python
Let’s see a more complete example of reading a JSON file. Suppose you have a JSON file called employee.json
:
{ "employee": { "name": "Charlie", "salary": 3200, "married": true, "address": "Imaginary Street 149" } }
And let’s say this file is located in the same folder as your Python program file, like so:
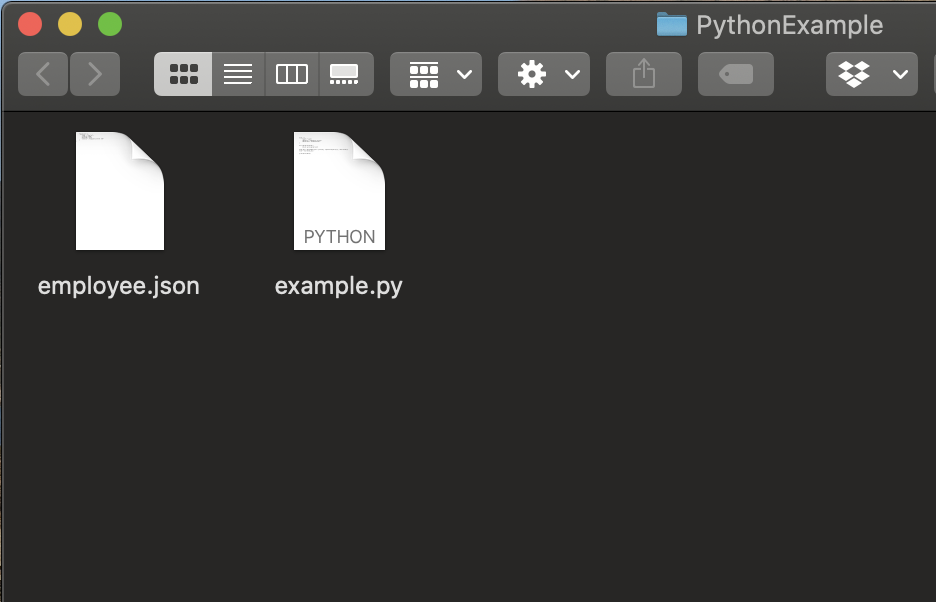
Because both files are in the same folder, you can now load the JSON by using the name of the JSON file as the path to it:
import json with open('employee.json') as file: data = json.load(file) print(data)
Remember, if the file is not in the same folder, you need to figure out the correct path to the file.
Anyway, here is the output of the above program:
{'employee': {'name': 'Charlie', 'salary': 3200, 'married': True, 'address': 'Imaginary Street 149'}}
In short, the above sample code:
-
Opens a file using the
with
statement. - Loads the JSON content from that file using the
json.load()
method. This turns the JSON data into a Python dictionary. - Finally, it prints the JSON data dictionary into the console.
Now you can do whatever you want with the JSON data dictionary in your Python program. As an example, let’s print the address
of the employee that comes with the data
:
address = data["employee"]["address"] print(address)
Output:
Imaginary Street 149
So this is how easy it really is to read a JSON file into your Python program.
Next, let’s make JSON printing look nicer by using a technique called pretty printing.
Make Your JSON Data Readable with Pretty-Printing
JSON is a compact data format that is commonly used in transmitting data over the internet. To make JSON data as compact as possible, it’s usually not formatted particularly nicely. For example, usually, the JSON is just a one-liner mess that is uneasy to get the hang of.
This is where you can use what is called pretty-printing.
Luckily, you can pretty-print JSON to make it look more appealing for you. To pretty-print the JSON data, you can use the json.dumps()
method. Don’t forget to specify the number of spaces you want for indentations!
print(json.dumps(data, indent=4))
Let’s apply this knowledge to the example in the previous section to see what happens.
Example of Pretty-Printing a JSON File in Python
Let’s go back to the previous example with employee.json
file.
Let’s read the JSON file the same way you already saw. But instead of directly printing the JSON data, let’s pretty-print it to make it readable:
import json with open("employee.json") as file: data = json.load(file) print(json.dumps(data, indent=4))
Output:
{ "employee": { "name": "Charlie", "salary": 3200, "married": true, "address": "Imaginary Street 149" } }
Now the output is way easier to understand than in the previous example as it’s now printed in a clear format. Now there is a clear structure to the JSON data where you can easily see what part belongs to where.
Pretty-printing JSON this way makes it easy to visualize the JSON data better. Especially when there is a whole bunch of JSON data, understanding the structure can be tricky. To efficiently work with JSON, you want to see it in as structured and clear a format as possible.
When reading JSON, I highly recommend you pretty-print it to make it easier for you to work with.
TIP: Feel free to copy-paste JSON data into a JSON formatter online. This can help identify the paths to particular JSON labels much easier.
Conclusion
To read JSON data into your Python program, use json.dumps()
method. Before you do that, you need to:
- Have a JSON file (and know the path to it).
- Import the JSON module into your Python program.
For example, if you have a JSON file employee.json
in the same folder as your Python program, you can read it with:
import json with open('employee.json') as file: data = json.load(file)
Also, don’t forget to pretty-print the JSON data to make it readable.
Thanks for reading. Happy coding!
Further Reading
- You commonly need to deal with JSON data when using an API. Make sure you check out a comprehensive guide on what is an API.