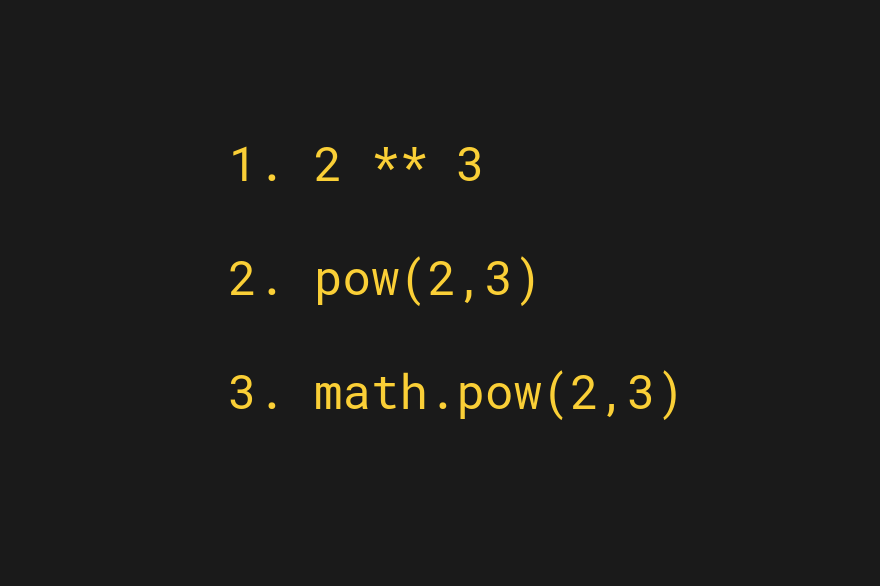
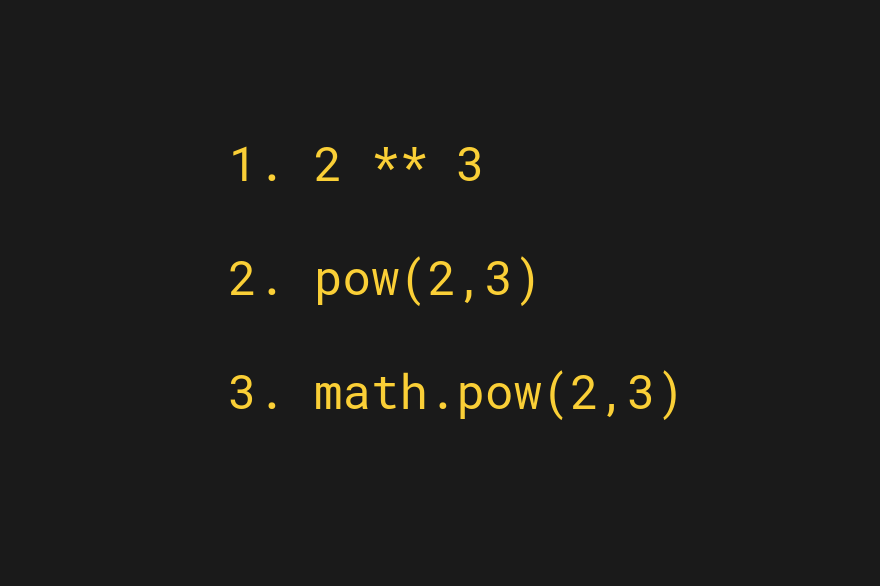
To raise a number to a power in Python, use the Python exponent ** operator.
For example, 23 is calculated by:
2 ** 3
And generally n to the power of m by:
n ** m
Python exponent is also related to another similar topic. The exponent notation is a way to express big or small numbers with loads of zeros. You can use the exponent notation e
or E
to replace the powers of ten.
For example, a billion (1 000 000 000) is 109. This means it can be written with an exponential notation 1e09 using the letter e or E followed by the number of zeros:
1000000000 # Hard to read the zeros 1e09 # Easier to read
This is a comprehensive guide to calculating exponents in Python. You will learn different operations you can use to raise a number to a power. Besides, you will learn how the exponent notation helps write big numbers in a more compact format. Of course, you’ll also learn why there are many ways to calculate exponents and which one you should use.
What Is an Exponent in Maths
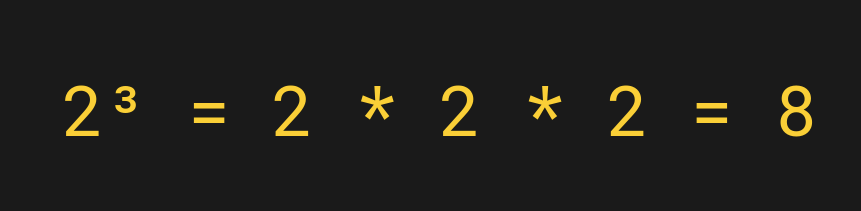
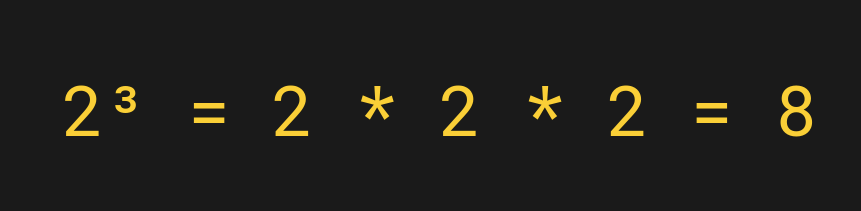
An exponent is the number of times the number is multiplied by itself. In maths, the exponent is denoted with a number as a superscript, such as 23.
The operation involving exponents is called raising a number to a power.
This means number 2 is multiplied by itself 3 times. This gives: 23 = 2 * 2 * 2 = 8
That’s it for the maths part. Let’s see how to calculate exponents in Python.
Let’s also get to know the exponent notation, which can help you represent large and small numbers.
How to Raise a Number to a Power in Python
There are three ways you can raise a number to a power in Python:
- The
**
operator - The built-in
pow()
function - The
math
module’smath.pow()
function
Here’s a table that summarizes each way to calculate exponents in Python:
Method | Description | Example | Result |
---|---|---|---|
1. a ** b | Raises a to the power of b | 2 ** 4 | 16 |
2. pow(a,b) | Raises a to the power of b | pow(3,4) | 81 |
3. math.pow(a,b) | Raises a to the power of b | math.pow(5,2) | 25 |
Let’s go through each of these with examples. We are also going to discuss the subtle differences between these methods.
1. The Double Asterisk (**) Operator
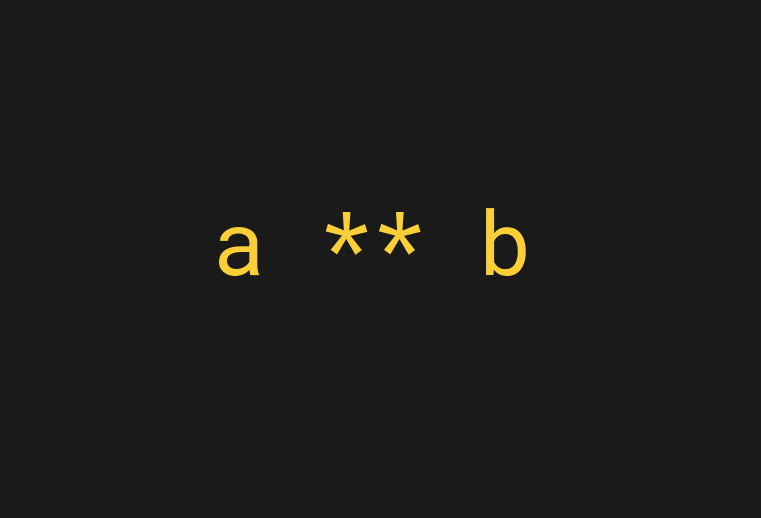
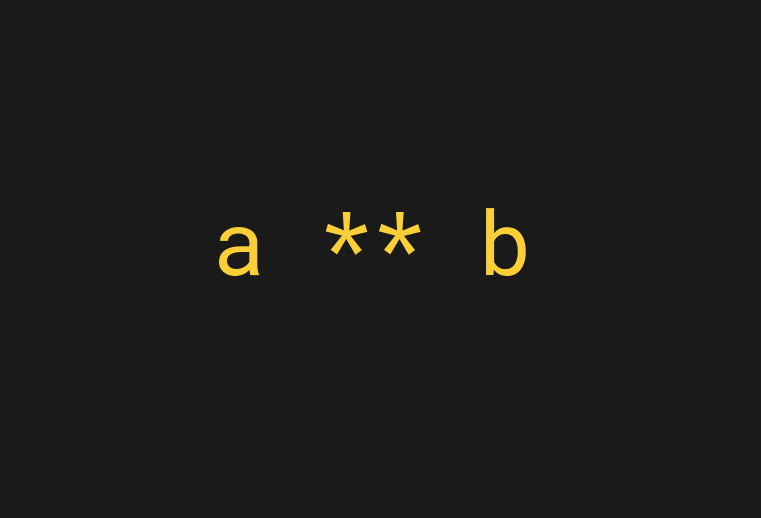
You can use the double-asterisk operator to raise a number to a power in Python.
For example:
2 ** 3 # -> 8
This is a clear and efficient way to compute powers in Python. Most of the time, this approach is the fastest to compute power in Python. More on efficiency later.
2. Pow() Function
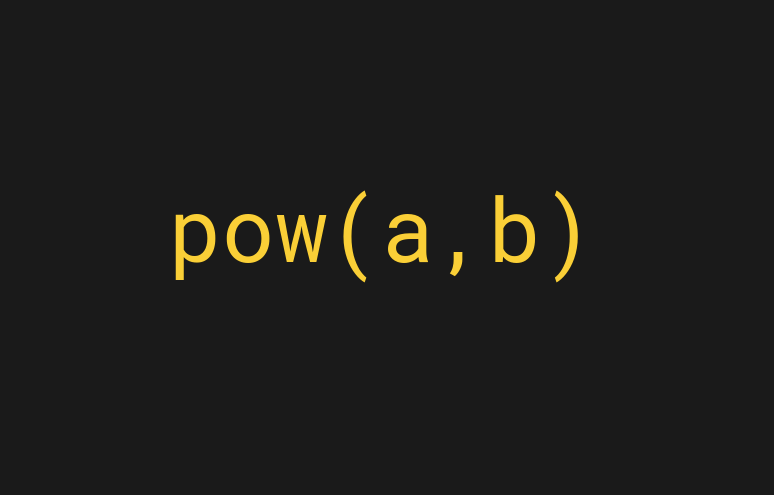
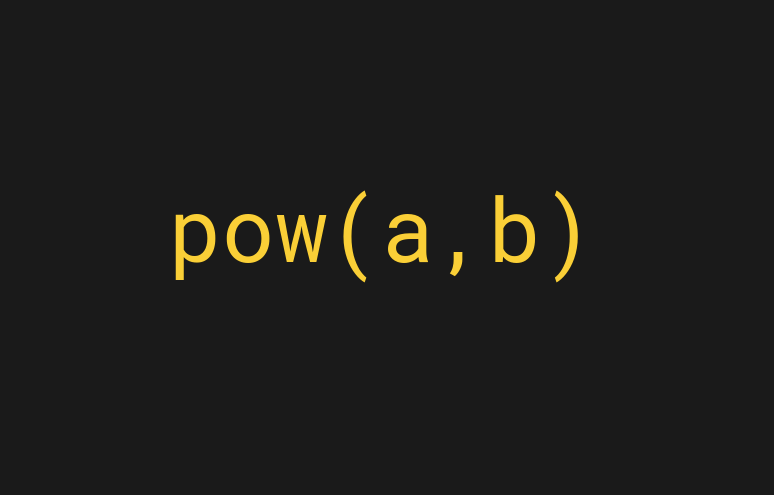
You can also use the built-in pow() function to raise a number to power.
For instance:
pow(2, 3) # -> 8
3. Math.pow() Function
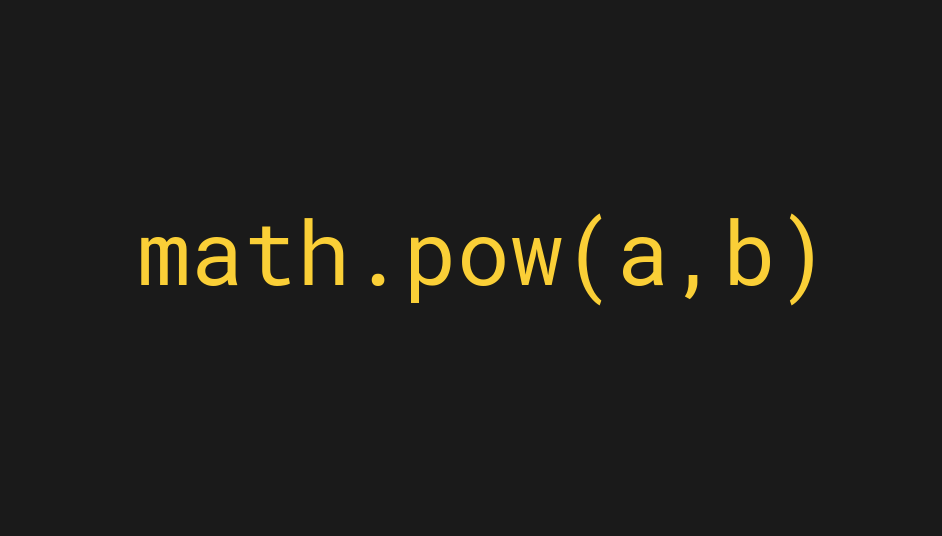
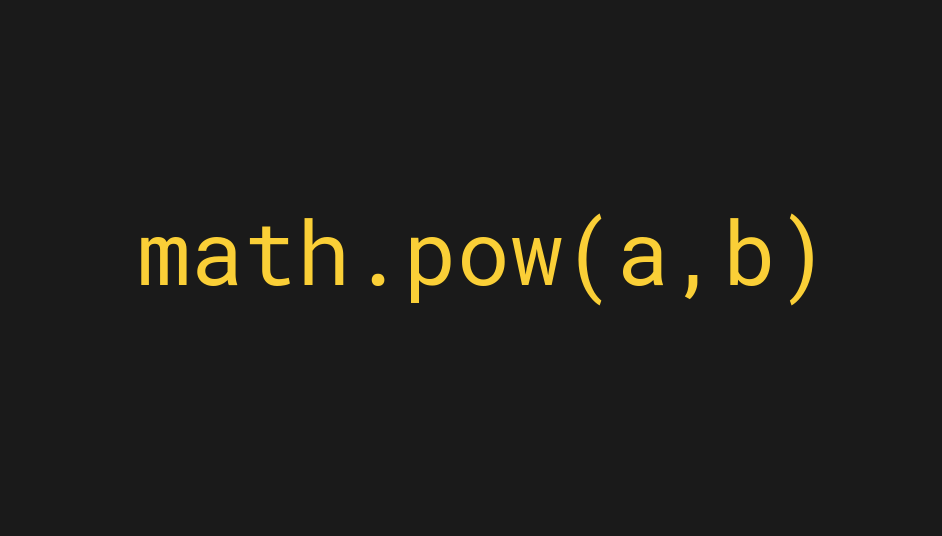
Finally, you may also use math.pow() function to raise a number to a power. Just remember to import the math module into your project.
For example:
import math math.pow(2, 3) # -> 8.0
This function does the same thing as the two earlier power-calculating approaches. But it is most efficient with floats.
Pow() vs math.pow() vs ** Operator
There are three main ways to raise a number to power in Python. Let’s discuss the differences between them.
All three approaches work almost identically with one another. But there are some slight differences you may be interested to learn.
- ** is generally faster.
- Math.pow() uses floats only.
- Math.pow() does not allow imaginary numbers.
- The built-in pow() function accepts a third argument.
Let’s go through each of these main differences in a bit more detail.
1. The ** Operator Is Faster
The double-asterisk approach is slightly faster than pow() or math.pow(). This is because it does not involve a separate function call.
For example:
from timeit import timeit asterisk_time = timeit('70. ** i', setup='i = 10') pow_time = timeit('pow(70., i)', setup='i = 10') math_pow_time = timeit('math.pow(70, i)', setup='import math; i = 10') print(f" **: {asterisk_time} \n pow: {pow_time} \n math.pow: {math_pow_time}")
Output:
**: 0.066251788 pow: 0.080007215 math.pow: 0.10603947999999999
2. Math.pow() Only Uses Floats
Math.pow() handles its arguments differently compared to the built-in pow() function or **
operator. Math.pow() converts the arguments to floats and returns the result as a float. In comparison, the built-in pow() and the ** return the result as an integer with integer inputs.
math.pow(4, 2) # 8.0 pow(4, 2) # 8 4 ** 2 # 8
If you want to raise a number to a power and have the result as a float, you can use math.pow(). This way you don’t have to separately convert the result to float yourself. This is very subtle, but the difference is there.
3. Math.pow() Does Not Accept Imaginary Numbers
The built-in pow() function and the ** operator support imaginary numbers. But math.pow() does not.
For instance:
pow(2, 1 + 0.5j) # 1.8810842093664877+0.679354250205337j 2 ** 1 + 0.5j # 1.8810842093664877+0.679354250205337j math.pow(2, 1 + 0.5j) # TypeError: can't convert complex to float
The math.pow() throws an error with imaginary units. So if you want to deal with imaginary numbers with powers, use pow() or **.
4. Pow() Takes a Third Argument
The built-in pow() function has a special use case for computing ab mod c. To do this, pass a third argument to pow() call.
For example, let’s compute 32 mod 4:
pow(3, 2, 4) # returns 1
It turns out this approach is faster than using the ** operator for doing the same:
(3 ** 2) % 4
Let’s make a comparison by using the timeit module and let’s use some big numbers:
import timeit start = timeit.default_timer() pow(3000000000, 2000, 4000) stop = timeit.default_timer() pow_time = stop - start print('Time using pow: ', pow_time) start = timeit.default_timer() (3000000000 ** 2000) % 4000 stop = timeit.default_timer() asterisk_time = stop - start print('Time using **: ', asterisk_time) print(f"The pow was {asterisk_time / pow_time} times faster")
Output:
Time using pow: 4.804000000000613e-06 Time using **: 0.0005024339999999995 The pow was 104.58659450456607 times faster
All in all, the differences between pow(), math.pow(), and ** are subtle but they exist. If you are a beginner, it does not really matter which approach you use as long as it works.
So far you’ve learned how to raise a number to a power in Python with exponents. But there’s another important use case for the exponents in Python that helps you express big and small numbers.
Python Exponent Notation—Get Rid of Zeros
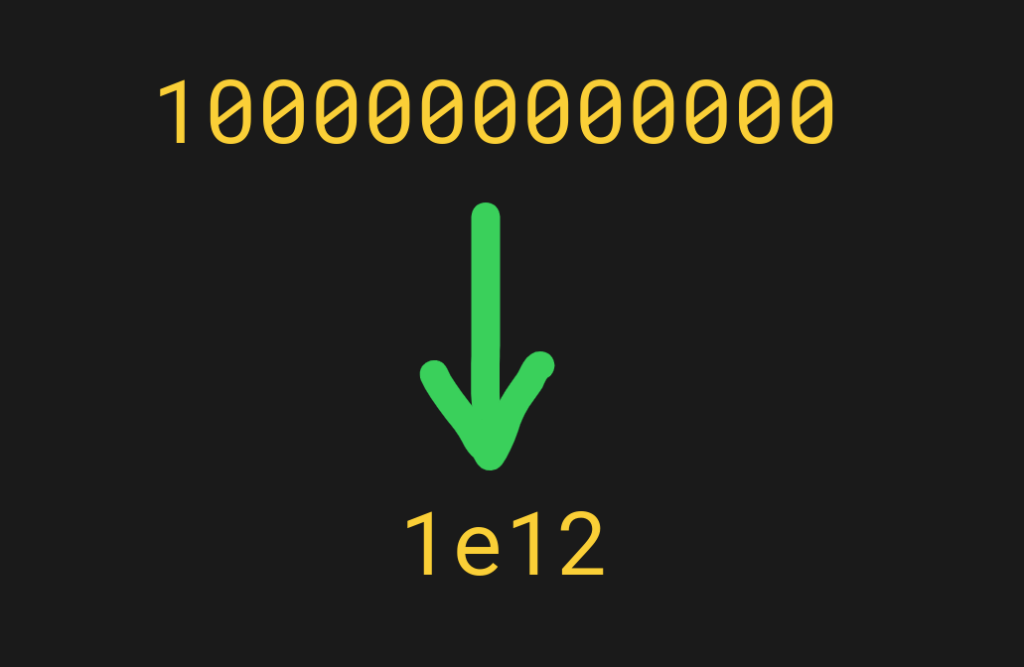
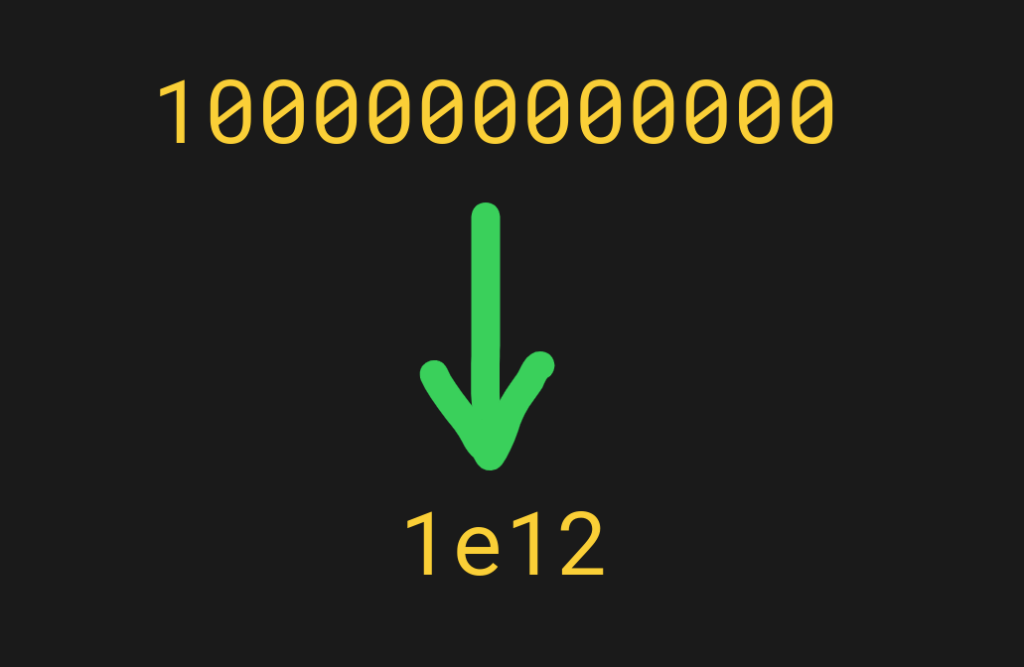
In Python, the exponent notation is used to express huge numbers or tiny numbers. The exponent notation becomes handy with numbers that have a lot of digits. With the exponent notation, the number has fewer digits and is easier to read and understand.
For example, a big number like one billion (1 000 000 000) can be hard to read in Python. This is where the exponent notation helps. It lets you replace powers of ten with e
or E
.
As you may know, a billion is 109. So you can replace that in Python with a shorthand of 1e09. (This reads 1 multiplied 10 to the power of 9, which is billion.)
# Hard to read 1000000000 # Better (possible for Python 3.6 <) 1_000_000_000 # Even better especially for the mathematically oriented 1e09 # or 1E09
The same goes for tiny numbers. Small numbers such as 0.7 or 0.03 are easy to read. But when numbers get really small, they become hard to read due to the leading zeros. For example, 0.000000001.
To overcome the readability issues, you can denote small numbers with the exponent notation e or E as well.
Let’s take a look at that number 0.000000001. Based on the number of zeros, it appears to be one billionth.
To denote this number in an exponent form, you can use the e notation. This time, however, you need to use a negative exponent (as the number is less than 1). This means billion becomes 1e-09.
# Hard to read 0.000000001 # Better 1e-09
Here are some random examples of representing numbers in the exponential form:
10 1e01 0.1 1e-01 2000 2e03 0.000342 3.42e-04 # Or just as well 342e-06 # The exponent notation does not always make things more readable, though. # For example: 12000.15 1.200015e04
Conclusion
Python exponent refers to two things:
- Raising numbers to a power.
- Denoting large or small numbers with e or E to reduce zeros.
To raise a number to a power in Python, you can use the double-asterisk operator **:
2 ** 3
Or you can use the built-in pow() function:
pow(2, 3)
Or you can use the math.pow() function especially when dealing with floats:
import math math.pow(2, 3)
To denote a number in the exponent form, replace powers of 10 with e or E:
# Hard to read 1000000000 # Better (possible for Python versions of 3.6+) 1_000_000_000 # Even better especially for the mathematically oriented 1e09 # or 1E09
Thanks for reading. I hope you enjoy it. Happy coding!