To convert a Python list into a string, use the join()
method of a string:
words = ["This", "is", "a", "test"] sentence = " ".join(words) print(sentence)
Output:
This is a test
This is a complete guide to converting a list to a string in Python. This guide shows you different approaches to making the conversion. Besides, you learn what to do if the list doesn’t have strings, to begin with.
If The Original List Doesn’t Contain Strings
If a list does not contain strings, you need to convert the elements to a string before merging them into a single string. You can do this by using a list comprehension, for example.
For instance, let’s convert a list of numbers to a string by using list comprehension and the join()
method:
numbers = [1, 2, 3, 4, 5] numbers_str = " ".join([str(number) for number in numbers]) print(numbers_str)
Output:
1 2 3 4 5
Convert List to a String in Python
There are 4 main ways you can use to convert a list into a string in Python.
- Use
string.join()
method for a list of strings - Use a list comprehension for a list of non-string elements
- Use the built-in
map()
function - Use a regular for loop to convert the list into a string
Let’s take a closer look at each of these approaches.
1. Merge a List of Strings to One String with the join() Method
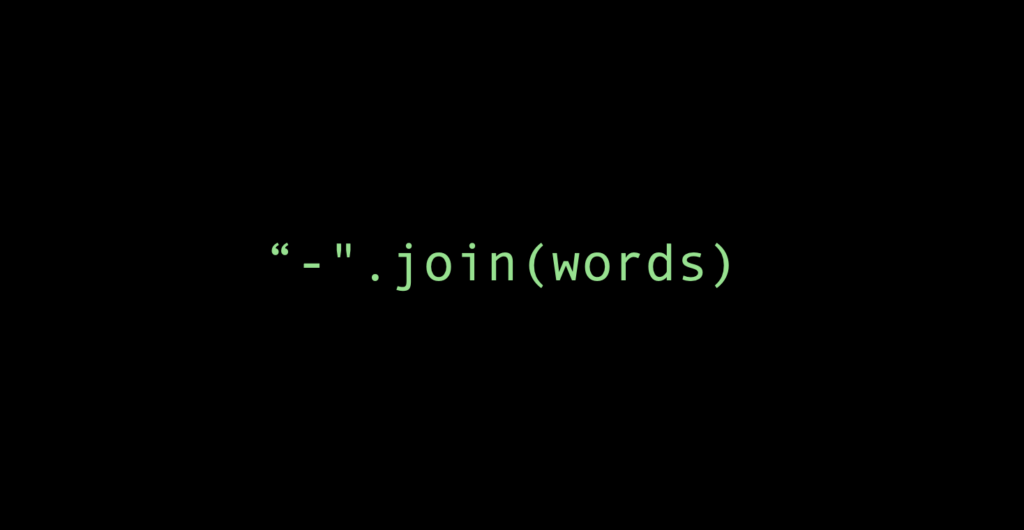
This approach works if you want to merge a list of strings into one long string.
The string type in Python has a built-in method called join()
. You can call join on any string. When you call join on a string with a list of strings, the list of strings is merged together by using the main string as a separator.
For instance, if you want to create a string where each string is on its own line, use a new line string as a separator. In other words, call join()
on the string "\n"
which represents a line break.
For example:
words = ["This", "is", "a", "test"] sentence = "\n".join(words) print(sentence)
This gives you an output:
This is a test
Now if you want to form a sentence based on a list of strings, call the join()
method on an empty space. This way it joins the list of strings and separates them by an empty space.
For example:
words = ["This", "is", "a", "test"] sentence = " ".join(words) print(sentence)
Result:
This is a test
2. Use a List Comprehension


If you have a list that does not contain strings and want to create a string from it, use comprehension.
If you have not heard about list comprehension, I highly recommend checking this article.
A list comprehension is a shorthand for a for loop. It transforms one list into another. In this case, list comprehension converts a list of non-string elements to a list of strings.
For instance, let’s create a list of numbers and transform it into a list of strings:
numbers = [1, 2, 3, 4, 5] num_strings = [str(number) for number in numbers] print(num_strings)
Output:
['1', '2', '3', '4', '5']
Now you know how to use comprehension to convert a list to a list of strings. To merge this list into one string, use the join()
method as you saw earlier:
numbers = [1, 2, 3, 4, 5] numbers_str = " ".join([str(number) for number in numbers]) print(numbers_str)
3. Use the Built-In map() Function
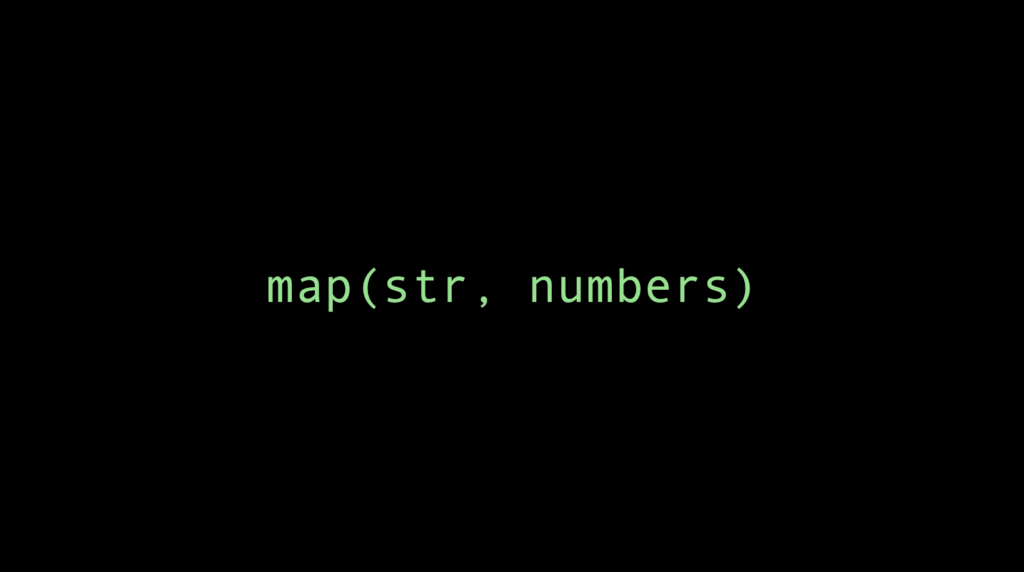
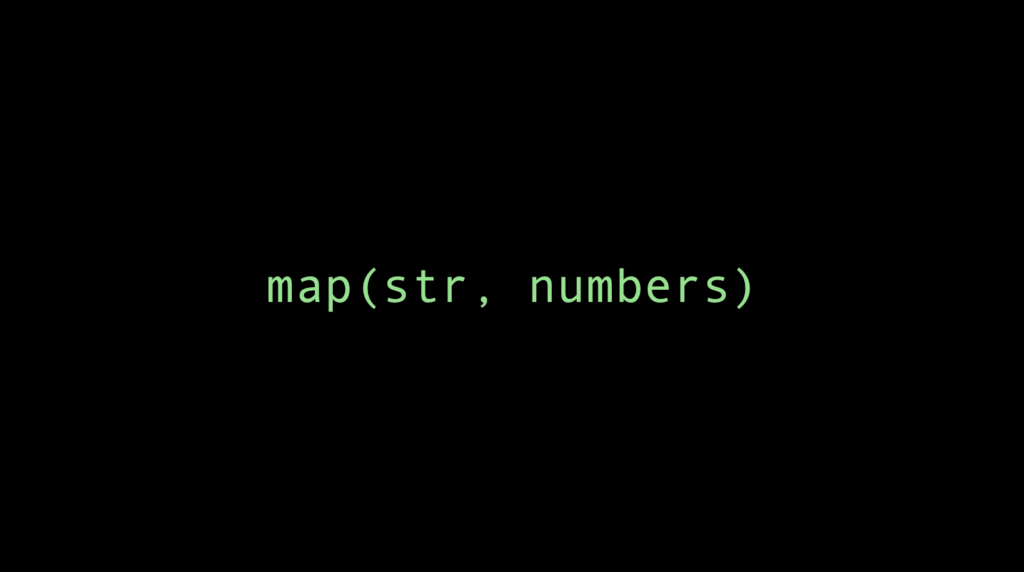
To convert a list of non-string elements into one string, you need to transform the list into a list of strings.
In the previous section, you saw how you can do it using list comprehension.
In this section, you are going to learn how you can use the built-in map()
function to do the same. In case you are unfamiliar with mapping in Python, check out this article for further details.
In short, the map()
function takes an operation and applies it to a collection of items, such as a list. The result is a new collection as a map object. The syntax for using map()
is as follows:
map(operation, collection)
And under the hood, all it does is it loops through the collection
and calls operation()
on each element.
To demonstrate, let’s create a list of numbers and turn it into a list of strings using the map()
function:
numbers = [1, 2, 3, 4, 5] num_strings = map(str, numbers) print(list(num_strings))
Output:
['1', '2', '3', '4', '5']
As described, this works such that the map()
function calls str()
on each element of a list. Then it returns a new map object that contains all the elements as a string. To convert this map object to a list, we use the built-in list()
function.
Let’s now apply this to our original problem on how to transform a list into a string.
As we are dealing with a list of numbers, we need to convert it to a list of strings. Then we can use the join()
method.
numbers = [1, 2, 3, 4, 5] num_string = " ".join(map(str, numbers)) print(num_string) print(f"The type of number_string is {type(num_string)}")
Output:
1 2 3 4 5 The type of number_string is <class 'str'>
4. Use a For Loop to Convert a List into a String
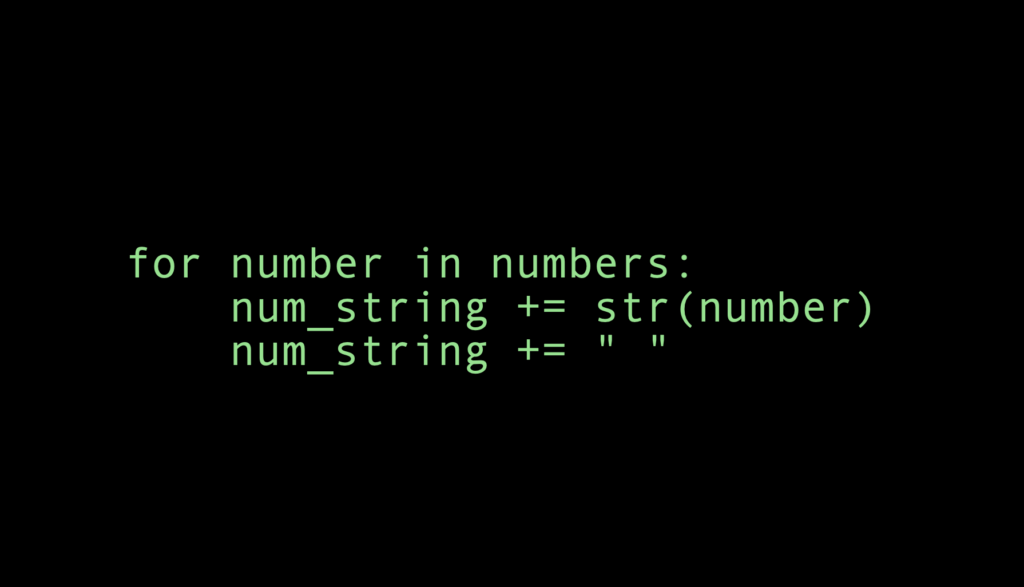
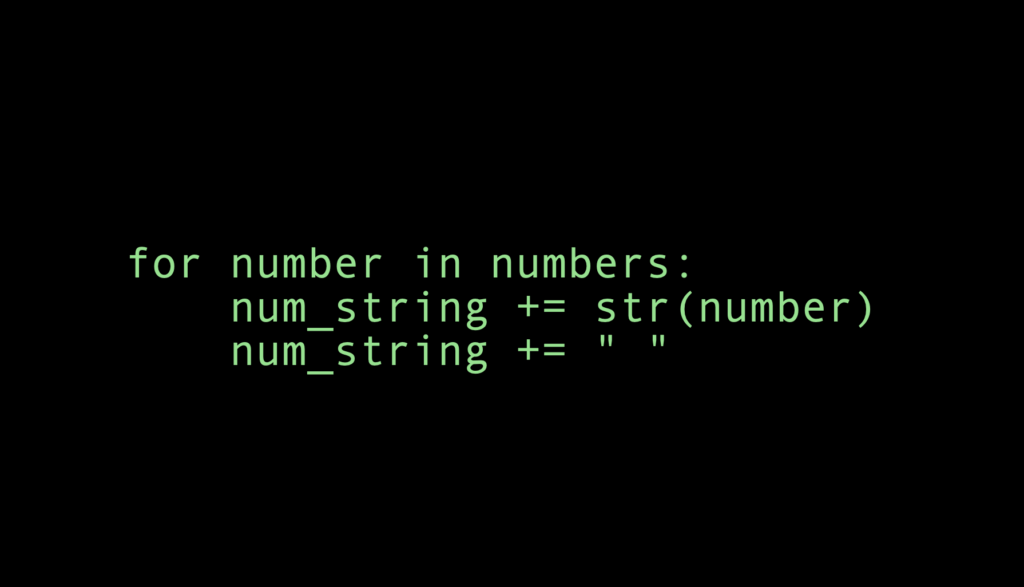
Last but not least, you can always use a regular for loop to convert a list into a string.
This approach takes quite a few lines of code, but it is readable and straightforward.
This loop takes each number from a list of numbers, transforms it into a string, and adds it to the end of a string.
numbers = [1, 2, 3, 4, 5] num_string = "" for number in numbers: num_string += str(number) num_string += " " print(num_string) print(f"The type of number_string is {type(num_string)}")
Output:
1 2 3 4 5 The type of number_string is <class 'str'>
Conclusion
Today you learned how to convert a Python list to a string using these four ways:
-
String.join()
method. - List comprehension +
string.join()
. - The built-in
map()
function +string.join()
. - For loop to convert the list into a string.
Thanks for reading. I hope you enjoy it. Happy coding!