There are 33 reserved keywords in Python in total. These keywords serve a useful purpose and are written as non-usable words in the Python programming language.
For example, ‘if’ is a reserved keyword. It allows you to introduce if-else logic in your code. You can’t name your variable as ‘if’ in Python.
Here’s a complete list of all the reserved keywords in Python. This guide shows you the use of each of these keywords.
and | else | in | return |
as | except | is | True |
assert | finally | lambda | try |
break | false | nonlocal | with |
class | for | None | while |
continue | from | not | yield |
def | global | or | |
del | if | pass | |
elif | import | raise |
Let’s go through each of these reserved keywords in more detail.
Feel free to use this list as a cheat sheet for Python’s reserved keywords.
1. and
The and operator is one of Python’s logical operators.
The and operator returns True if both statements surrounding it evaluate True.
Here is the truth table of the and operator in Python:
A | B | A and B |
False | False | False |
False | True | False |
True | False | False |
True | True | True |
For example:
is_sunny = True is_warm = True is_good_day = is_sunny and is_warm print(is_good_day)
Output:
True
2. as
The as statement in Python re-assigns a returned object to a new identifier.
There are two cases when you commonly use as statement in Python:
- Import a module with an alias name.
- Open a file with a context manager.
For example, you can create an alias for an imported module called datetime:
import datetime as dt today = dt.date.today() print(today)
Creating aliases is useful when you have a long module (or module member) name that you have to repeat over and over again.
As another example, here is how opening a file looks with a context manager:
with open("example.txt", "r") as file: lines = file.read()
3. assert
The assert statement in Python is used to continue execution given a condition that evaluates True. If the condition is False, an AssertionError is thrown.
You can include an error message in the assert call. This will be displayed as an error message with the AssertionError.
For example, let’s make sure no divisions are made by 0.
def divide(a, b): assert b != 0, "Division by 0 is not possible" return a / b # Example call with a faulty input: divide(10, 0)
Output:
Traceback (most recent call last): File "<string>", line 5, in <module> File "<string>", line 2, in divide AssertionError: Division by 0 is not possible
4. break
The break keyword in Python is a control flow statement. It is used to jump out of a loop. The execution resumes at the next statement.
The break statement is used to terminate loops that have no good reason to continue.
For example, let’s create a list of numbers and a target number. When we find the target number from the list of numbers, there is no reason to continue looping.
nums = [3, 2, 9, 2, 4, 3, 2, 1, 8] target = 9 for number in nums: print(f"Checking {number}...") if number == target: print("Target found!") break
Output:
Checking 3... Checking 2... Checking 9... Target found!
5. class
The class keyword in Python is used to define classes.
A class is a blueprint from which objects are created in Python. Classes bundle data and functionality together. Writing a class creates a new object type in your project. You can then create new instances of that custom type in your project.
For example, let’s create a Fruit class. With this class, you can create Fruit objects with different names in your code.
class Fruit: def __init__(self, name): self.name = name banana = Fruit("Banana")
Learn more about Python classes here.
6. continue
The continue statement is one of the control flow statements in Python. It is used to stop the current iteration in a loop.
Unlike break, the continue statement does not exit the loop.
The continue statement is useful when there is no reason to continue the current iteration any further.
For example, given a list of numbers and a target number, we are going to search for the target in the list.
To do this, we create a for loop where we check each number. If the number is not the target number, we continue to the next number. When the target is found, we break the loop.
nums = [3, 2, 9, 2, 4, 3, 2, 1, 8] target = 9 for number in nums: print(f"Checking {number}...") if number != target: continue print("The target number is found!") break
Output:
Checking 3... Checking 2... Checking 9... The target number is found!
7. def
The def statement in Python is used to declare a function or a method.
A function in Python is a block of reusable code that can be called to perform a certain action.
A function can also take arguments that it can use to perform an action.
For example, let’s create a function with a name as its argument. Calling this function with a name greets a person with that name:
def greet(name): print(f"Hello, {name}. How are you?") # Example call greet("Alice")
Output:
Hello, Alice. How are you?
8. del
The del statement in Python is used to delete an object.
For instance, let’s delete the first element of a list of numbers:
numbers = [1, 2, 3] del numbers[0] print(numbers)
Output:
[2, 3]
9. elif
The elif statement is used in conjunction with an if statement to check if a condition holds.
Elif is a shorthand for “else if”. The elif statement checks if some other condition holds when the condition in the if statement does not.
For example:
age = 17 if age >= 18: print("You can drive") elif age == 17: print("You can go to driving school") else: print("You cannot drive yet")
Output:
You can go to driving school
10. else
In an if-elif-else statement the else statement is used to perform actions that happen if no previous condition was True.
For example:
age = 10 if age >= 18: print("You can drive") elif age == 17: print("You can go to driving school") else: print("You cannot drive yet")
Output:
You cannot drive yet
You can also use else block in Python loops. This way you can execute a piece of code right after the loop terminates.
For example, here is else statement after a for loop:
numbers = [1, 2, 3] for number in numbers: print(number) else: print("All numbers printed")
Output:
1 2 3 All numbers printed
Here is an else statement after a while loop.
numbers = [1, 2, 3] i = 0 while i < len(numbers): print(numbers[i]) i += 1 else: print("All numbers printed")
Output:
1 2 3 All numbers printed
The else block can also be used in error handling to run a piece of code if no errors were found.
try: print("Success") except: print("Failure") else: print("No errors")
Output:
Success No errors
11. except
The except statement is part of the try-except error handling structure in Python.
In error handling, the try block tries to run a piece of code. If the piece of code throws an error, the except block catches it.
Error handling in Python is useful as the program does not crash because the error is handled in the code.
For example, let’s use error handling to try to divide 10 by 0:
a = 10 b = 0 try: result = a / b except ZeroDivisionError: print("Cannot divide by 0!")
Output:
Cannot divide by 0!
Here the except block catches a ZeroDivisionError that is thrown when trying to divide a number by 0.
Learn more about error handling in Python.
12. finally
The finally block is an optional part of the try-except error handling structure.
The finally statement always executes the code regardless of whether an error was thrown or not. You can use finally statement to run some cleanup code, such as to close a file.
For example, let’s try to divide by zero:
a = 10 b = 0 try: result = a / b except ZeroDivisionError: print("Cannot divide by 0!") finally: print("Error handling complete.")
Output:
Cannot divide by 0! Error handling complete.
Here you can see that the code inside the finally block was executed even though an error was thrown.
13. False
In boolean logic, false represents not true.
In Python’s boolean logic there are two values True and False.
False represents something not being True.
For example:
print(1==2)
Output:
False
14. for
The for keyword in Python is used to create a for loop.
A for loop iterates over a sequence of values, such as a list, dictionary, or tuple.
Using for loop, you can execute statements for each item in the sequence.
For instance, let’s square a list of numbers using a for loop:
numbers = [1, 2, 3] squared = [] for number in numbers: squared.append(number ** 2) print(squared)
Output:
[1, 4, 9]
A Python for loop can alternatively be written as a comprehension.
For example, here is the above example as a one-liner shorthand:
numbers = [1, 2, 3] squared = [number ** 2 for number in numbers] print(squared)
Output:
[1, 4, 9]
Learn more about looping in Python.
15. from
To include a specific member from a Python module, use from statement in the import statement.
For instance, let’s import the sqrt() function from the math module:
from math import sqrt print(sqrt(16))
Output:
4.0
Learn what is the difference between from and from import in Python.
16. global
In Python, you can access global variables just like any other variable.
But to modify a global variable in Python, you need to use global keyword.
For example:
foo = 1 def change(): global foo foo = 3 change() print(foo)
Output:
3
Here is a more illustrative example:
foo = 1 # A global variable def temp_change(): foo = 2 # new local foo variable print(f"In temp_change() function, foo is {foo}") temp_change() print(f"After calling temp_change(), foo is still globally {foo}") def change(): global foo foo = 3 # changes the value of the global foo print(f"In change() function, foo becomes {foo}") change() print(f"After calling change(), foo is also globally {foo}")
Output:
In temp_change() function, foo is 2 After calling temp_change(), foo is still globally 1 In change() function, foo becomes 3 After calling change(), foo is also globally 3
17. if
In Python, an if statement is used to check if a condition evaluates True. If it does, then some action is performed.
if condition: # some action
Usually, if statements are coupled with elif statements and an else statement.
For instance:
age = 50 if age >= 18: print("You can drive") elif age == 17: print("You can go to driving school") else: print("You cannot drive yet")
Output:
You can drive
18. import
To include a Python module in your project, use the import statement.
For example, let’s import the math module to bring in some useful mathematical tools:
import math num = 16 square_root = math.sqrt(num) print(square_root)
Output:
4.0
19. in
The in statement in Python can be used to check if an element exists in an iterable. It returns True if it finds one and False if it does not.
For example, you can check if a number is found in a numbers list. Or you can test if a character is found in a string.
Here is a code example:
numbers = [1, 2, 3] one_exists = 1 in numbers print(one_exists)
Output:
True
20. is
The is statement in Python is used to check if two objects are the same objects. In other words, if the two objects point to the same memory address.
For example:
a = [1, 2, 3] b = a print(a is b)
Output:
True
Here a is b because b points to the same memory address as a.
Let’s see another example where is statement returns False even though the objects look identical:
a = [1, 2, 3] b = [1, 2, 3] print(a is b)
Output:
False
The reason why this evaluates False is that even though the objects look the same, they are not the same under the hood.
You can verify it by checking the IDs of the objects:
a = [1, 2, 3] b = [1, 2, 3] print(id(a)) print(id(b))
Output:
4389559616 4389860736
In fact, the is statement checks if the IDs of the objects match to determine whether they are the same object or not.
Learn more about the is operator, id() function, and copying items in Python here.
21. lambda
A lambda function in Python is an anonymous function. It can take any number of arguments but only have a single expression.
You can always replace a lambda function with a regular function, but not the other way around.
Lambdas are useful when you only need the functionality once and don’t need a separate method to get the job done.
A good use case for lambdas is mapping a list of numbers.
- The lambda function is applied for each element in the list.
- When the mapping completes, there is no trace of the lambda.
Here is how it looks in code:
numbers = [1, 2, 3] squared_nums = map(lambda x: x ** 2, numbers) print(list(squared_nums))
Output:
[1, 4, 9]
Notice how you could do the exact same thing with a regular function:
numbers = [1, 2, 3] def square(x): return x ** 2 squared_nums = map(square, numbers) print(list(squared_nums))
Output:
[1, 4, 9]
The map function takes the square() function and applies it to each element in the list.
Compared to the lambda approach, the downside is that now you have a function square() that you will never use again.
Learn more about lambdas in Python.
22. nonlocal
The nonlocal keyword is used with functions inside functions. It makes the variable not belong only to the inner function.
Here is an example of using a non-local variable:
def outer(): x = "Hello" def inner(): nonlocal x x = "World" inner() return x print(outer())
Output:
World
In the inner() function the nonlocal keyword means “Variable x is not only in the inner() function’s scope. Instead, it refers to the outer function’s variable x too”. This is why changing the variable inside the inner() function also changes it outside of it.
If you run the above code without the nonlocal declaration, you get a different result:
def outer(): x = "Hello" def inner(): x = "World" inner() return x print(outer())
Output:
Hello
This is because the variable x in the inner() function only belongs to the scope of that function. It thus does not affect the variable x in the outer function.
23. None
You may have heard about null values in programming.
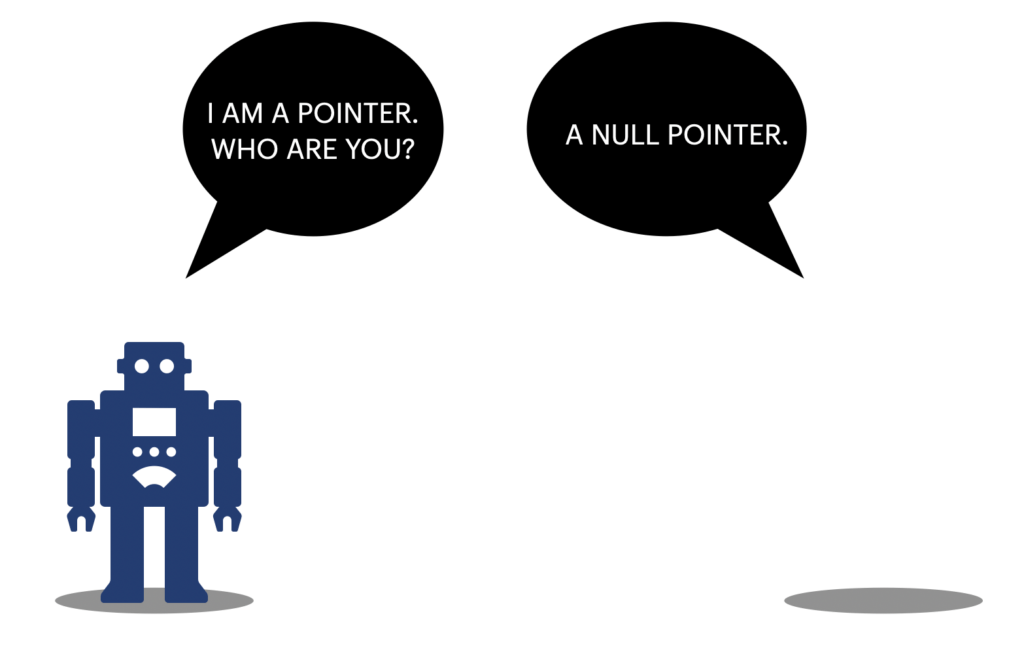
In Python, there is no null value. Instead, there is None.
The None is an object that represents the absence of a value. It is like an empty object.
For example, you can assign None to a variable:
name = None if name is None: print("None found") else: print(name)
Output:
None found
In Python, a function that does not have the return statement always returns None.
For example:
def example(): pass print(example())
Output:
None
Learn more about None in Python.
24. not
The not operator is a logical operator in Python. It returns True when a value is False and vice versa.
Here is the truth table of the not operator:
A | Not A |
True | False |
False | True |
For example:
sunny = False if not sunny: print("Cloudy")
Output:
Cloudy
25. or
The or operator in Python is a logical operator. You can check if either one of two conditions is True with or operator.
Here is the truth table of or operator in Python:
A | B | A or B |
False | False | False |
False | True | True |
True | False | True |
True | True | True |
For example:
value = 1 if value == 1 or value == 2: print("You won")
Output:
You won
26. pass
In Python, you cannot have empty implementations.
For example, you cannot declare a function without implementation.
To omit the implementation “after colon” in Python, use the pass statement.
For example:
def add(a, b): pass class Fruit: pass for i in range(10): pass
27. raise
In Python, you can raise errors with the raise statement. These errors will be visible in the traceback and they will cancel the execution of your program if not handled properly.
For example, let’s raise a TypeError with a custom message if the variable x is not an integer:
x = "Hello world" if not type(x) is int: raise TypeError("Only integers allowed!")
Output:
Traceback (most recent call last): File "example.py", line 4, in <module> raise TypeError("Only integers allowed!") TypeError: Only integers allowed!
28. return
The return keyword is used to send a result of a function or method call back to the caller.
For example, a function that adds two values should return the result:
def sum(a, b): return a + b print(sum(1, 2))
Output:
3
29. True
In boolean logic, true represents something being true.
In Python’s boolean logic, there are two values True and False.
True represents something being True.
For example:
print(1==1)
Output:
True
30. try
In Python’s error try-except error handling scheme, the try statement starts a block of code that is tried to execute. If it fails, the except block catches the error.
For example, let’s try to divide a number by zero:
a = 10 b = 0 try: result = a / b except ZeroDivisionError: print("Cannot divide by 0!")
Output:
Cannot divide by 0!
Here the code in the try block throws an exception. This is caught by the except block. Instead of crashing the program, the except block handles the error.
Read more about error handling in Python.
31. with
The with statement in Python is used for resource management and error handling.
The with statement replaces commonly used try/finally error-handling statements.
You see with statement commonly being used when working with files in Python. The with statement makes sure that a file process doesn’t block other processes if an error is thrown. The with statement makes sure that the file being processed is closed after processing.
with open("example.txt", "r") as file: lines = file.read()
This piece of code reads the lines from a text file called “example.txt”.
Learn more about the with statement in Python.
32. while
The while statement in Python is used to start a while loop.
A while loop is a looping structure you can use to iterate over values in Python.
The while loop continues iteration until a condition no longer holds.
For example, you can loop through a list of numbers using a while loop:
numbers = [1, 2, 3] i = 0 while i < len(numbers): print(numbers[i]) i += 1
Output:
1 2 3
Read more about looping in Python.
33. yield
The yield statement in Python means a function returns an iterator.
In Python, yield returns from a function without destroying its variables. The yield statement pauses the execution of the function. When the function is invoked again, the execution continues from the last yield statement.
To better understand what yield does, you need to understand what are generators, iterators, and iterables in Python. I highly recommend checking this article.
For example, here is a generator function that yields positive numbers from zero to infinity. Warning: This creates an endless loop:
def all_positive_nums(): i = 0 while True: yield i i += 1 for num in all_positive_nums(): print(num)
Output is an endless loop:
1 2 3 4 5 6 . . .
Syntactically it looks as if all_positive_nums() returned an infinite number of positive numbers. In reality, it generates the values on-demand and only stores a single value.
This is the whole point of generators. You can loop through a stream of items without storing them in memory all at once while syntactically it looks as if you did.
I hope you find it useful. Thanks for reading!