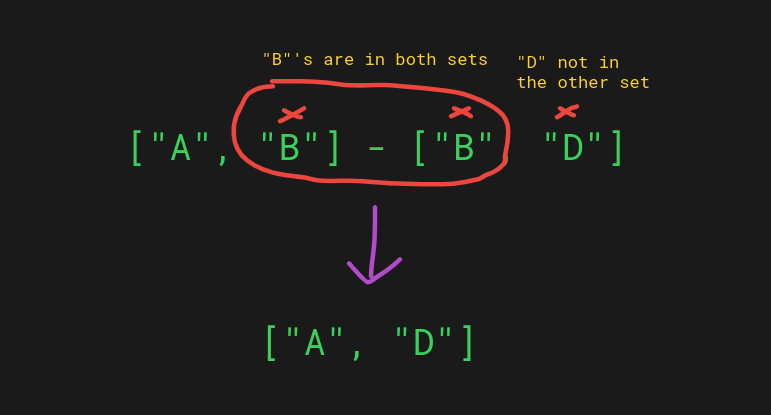
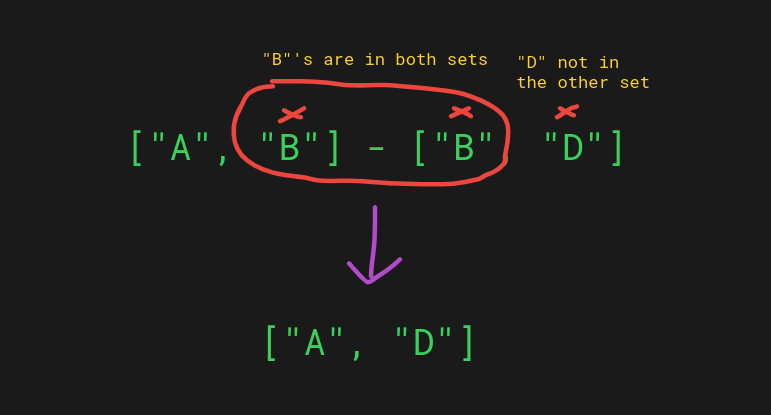
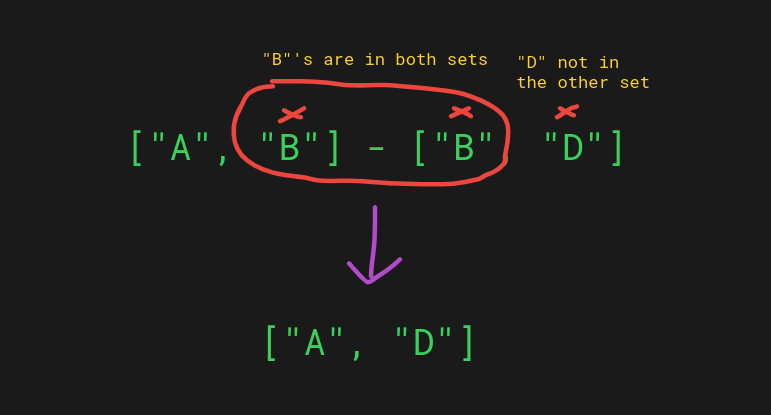
To find the asymmetric difference between two lists in Python:
- Convert the lists to sets.
- Subtract the sets from one another to find the difference.
- Convert the result back to a list.
Here is an example:
names1 = ["Alice", "Bob", "Charlie", "David"] names2 = ["Bob", "Charlie"] difference = list(set(names1) - set(names2)) print(difference)
Output:
['David', 'Alice']
Asymmetric Difference between Lists in Python
The asymmetric difference in set theory means that given sets A and B, return the elements that are in set A but not in set B. But do not return the elements that are in set B but not in A.
This image illustrates the idea:
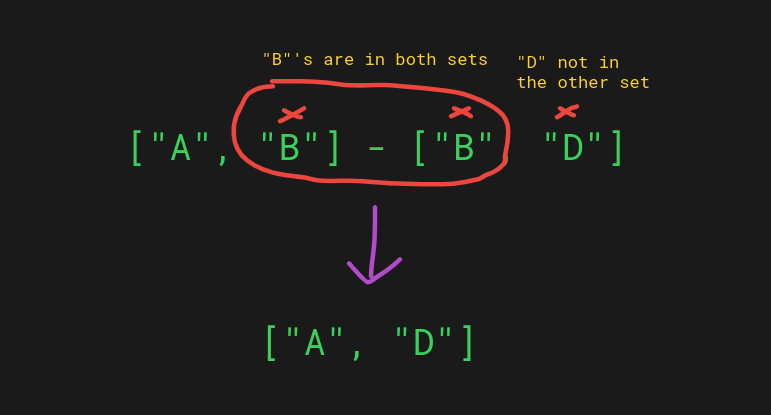
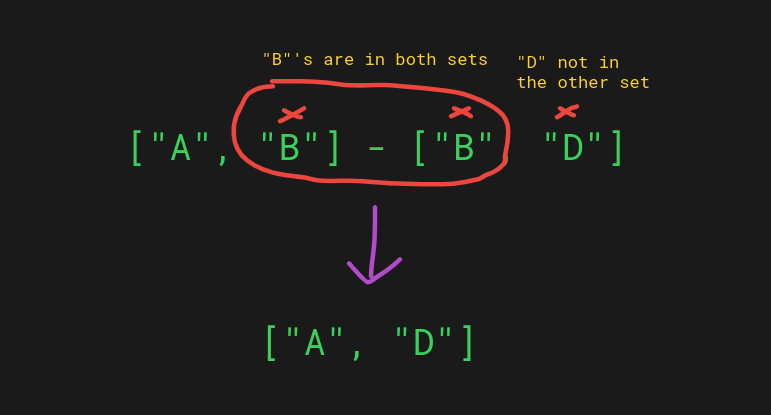
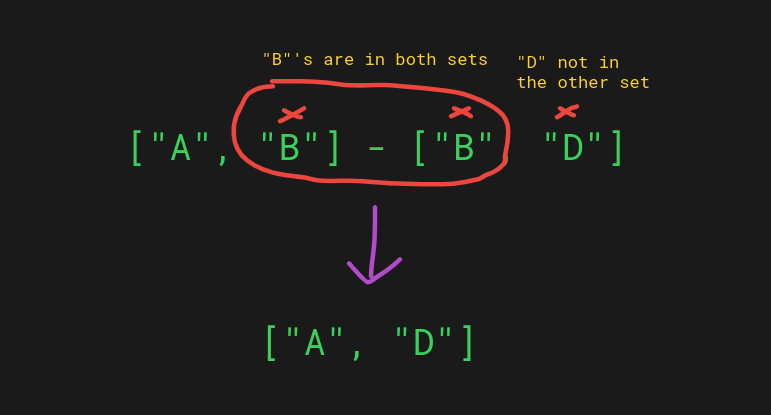
The example you saw above is about the asymmetric difference between two lists in Python.
For instance:
names1 = ["Alice", "Bob"] names2 = ["Bob", "Charlie"] difference = list(set(names1) - set(names2)) print(difference)
Gives you:
['Alice']
Even though you might expect ["Alice", "Charlie"]
as neither name occurs in both sets. But because the asymmetric difference is about finding only the elements that are in set A but not in B, it only returns “Alice”.
To fix this problem, you need to calculate the symmetric difference between the two lists.
Symmetric Difference between Lists in Python
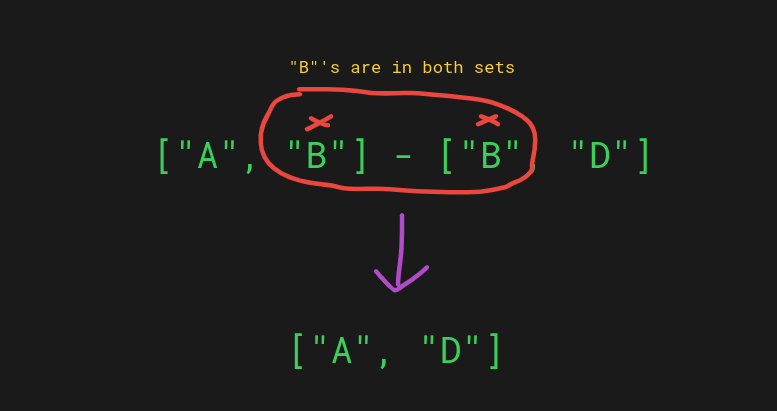
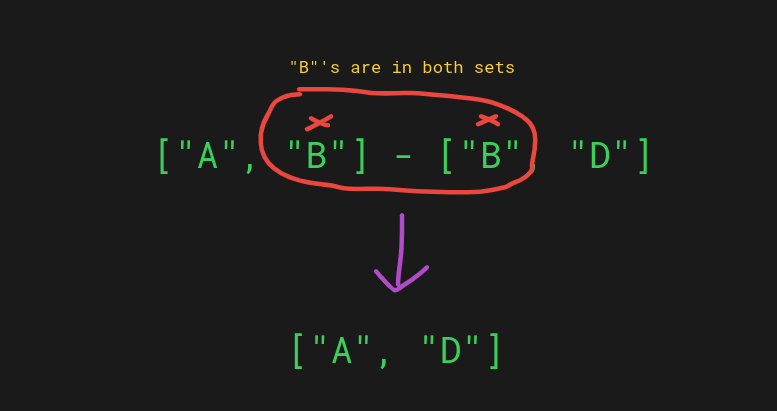
The symmetric difference in set theory means given sets A and B, return the elements that are in set A but not in set B and vice versa.
To get the symmetric difference between two lists in Python:
- Convert the lists to sets.
-
Use the built-in
symmetric_difference()
function of sets to get the symmetric difference. - Convert the result back to a list.
For example:
names1 = ["Alice", "Bob"] names2 = ["Bob", "Charlie"] difference = list(set(names1).symmetric_difference(set(names2))) print(difference)
Output:
['Charlie', 'Alice']
Now both names that do not occur in both lists are in the result.
Conclusion
The Asymmetric difference between two lists in Python returns the items that list A has but list B does not. This means if list B has an item that is not found in A, it does not count.
To calculate the asymmetric difference in Python lists:
- Convert the lists to sets.
- Compute the difference by subtracting one set from the other.
- Convert the result to a list.
The symmetric difference between two lists in Python returns the items in list A that are not in list B and vice versa. To calculate the symmetric difference in Python lists:
- Convert the lists to sets.
-
Compute the difference with
symmetric_difference()
method. - Convert the result to a list.
Thanks for reading. Happy coding!