In R, there’s a built-in function names() you can use to get or set the values of an R object. For example, you can set the names of list elements or the names of the columns in a data frame.
This comprehensive guide teaches you how to use the names() function with a bunch of simple examples.
What Is the names() Function in R?
The names() function in R is a built-in function you can use to set or get the name of an R object.
The names() function takes a vector, matrix, or data frame as an argument and returns the names of these objects. To change the names of these objects, you can use the assignment operator and provide a new value for the objects.
Syntax
Here’s the syntax of the names() function:
names(x) names(x) <- value
Where the two use cases are as follows:
- names(x) returns the names of x, which can be a vector, matrix, or data frame.
- names(x) <- value assigns a new value as the name of x. The value is a character vector whose length is up to the length of x (or NULL).
The best way to learn how to use the names() function in R is by seeing some examples.
Examples of names() Function in R
In this section, you find practical examples of using the names() function on different data types in R.
Example 1: names() Function with Vectors
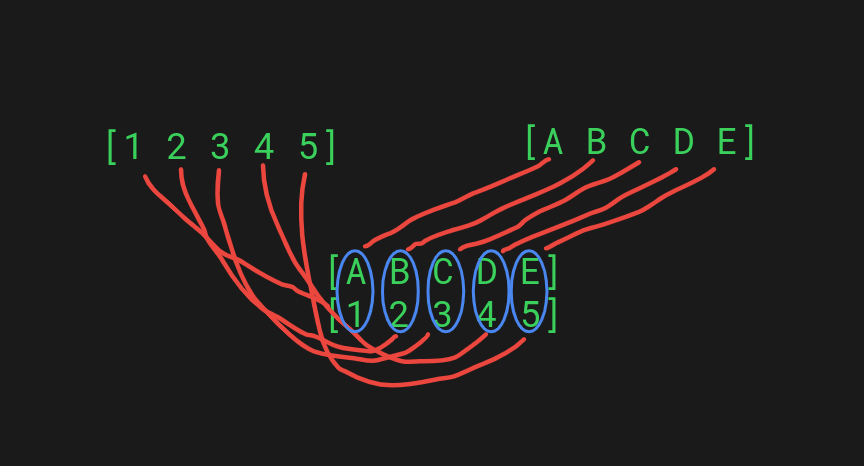
You can use the names() function on vectors in R to associate each vector element with a label.
For example, let’s associate each number in a vector of numbers 1-5 with a letter A-E:
example_list = c(1:5) names(example_list) <- LETTERS[1:5] example_list
Output:
A B C D E 1 2 3 4 5
Now you can access the vector elements using the names associated with each element:
example_list["C"]
Output:
C 3
Example 2: names() Function with Lists
Very similar to how you can call the names() function on vectors in R, you can call it on a list.
You can either check the current names of the list elements or assign new names to them.
For example, given a list that consists of numbers, letters, and strings, let’s associate a string label to each of these groups:
my_list = list( c(13, 24, 99), LETTERS[1:5], c("Alice", "Bob", "Charlie") ) names(my_list) <- c("Numbers", "Letters", "Strings") my_list
Output:
$Numbers [1] 13 24 99 $Letters [1] "A" "B" "C" "D" "E" $Strings [1] "Alice" "Bob" "Charlie"
Here’s an illustration of what the above code does:
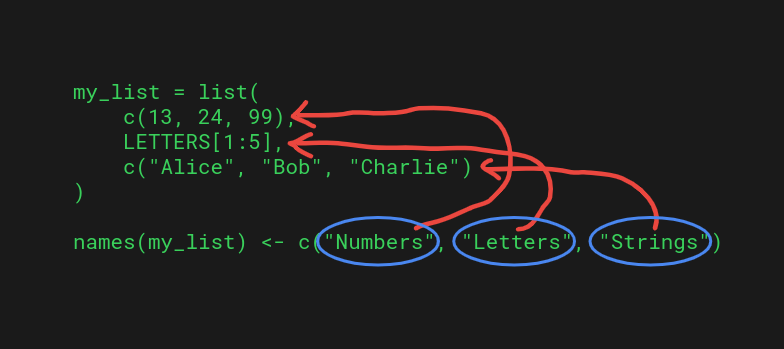
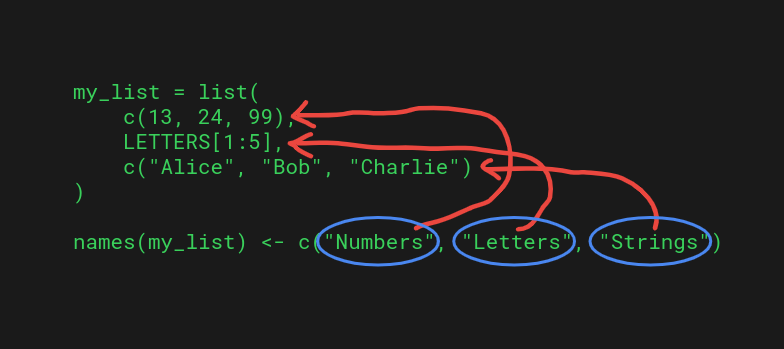
Example 3: names() Function with Data Frames
In the previous examples, you learned how to use the names() function to associate each element with a name in lists or vectors.
Last but not least, let’s take a look at an example where you set the names of the columns in a data frame.
For example, let’s create example soccer data on the Champions League
df_example <- data.frame(A=c('Arsenal', 'Bayer Munchen', 'Chelsea', 'Derby', 'Everton'), B=c(503, 350, 389, 398, 191), C=c(108, 79, 49, 65, 25), D=c(50, 39, 45, 35, 30)) df_example
Output:
A B C D 1 Arsenal 503 108 50 2 Bayer Munchen 350 79 39 3 Chelsea 389 49 45 4 Derby 398 65 35 5 Everton 191 25 30
This table has a bunch of data but the columns aren’t labeled so you have no idea what these numbers represent. To change this, let’s set the names for the columns of the data frame and print the result:
names(df_example) <- c('team', 'total_shots', 'shots_on_goal', 'goals') df_example
Output:
team total_shots shots_on_goal goals 1 Arsenal 503 108 50 2 Bayer Munchen 350 79 39 3 Chelsea 389 49 45 4 Derby 398 65 35 5 Everton 191 25 30
Summary
Today you learned how to use the names() function in R.
To recap, the names() function returns the names of the input argument. You can also use the names() function when setting the new name for an object. To do this, call the names() function on your object and use the assignment operator to assign a new value for the object.
Thanks for reading. Happy coding!