This is a comprehensive list of the popular terms and definitions programmers and software developers use.
You can benefit from reading this article if you:
- Are new to programming
- Are interested in programming
- Work with programmers
- Have software developer friends
- Want to test your knowledge as a programmer
This list is in alphabetical order.
Abstraction
Abstraction means removing the characteristics of something. The goal of abstraction is to reduce complexity.
In programming, abstraction is a commonly used technique. It means hiding unnecessary details of an object. The process of abstraction simplifies the complexity and boosts efficiency.
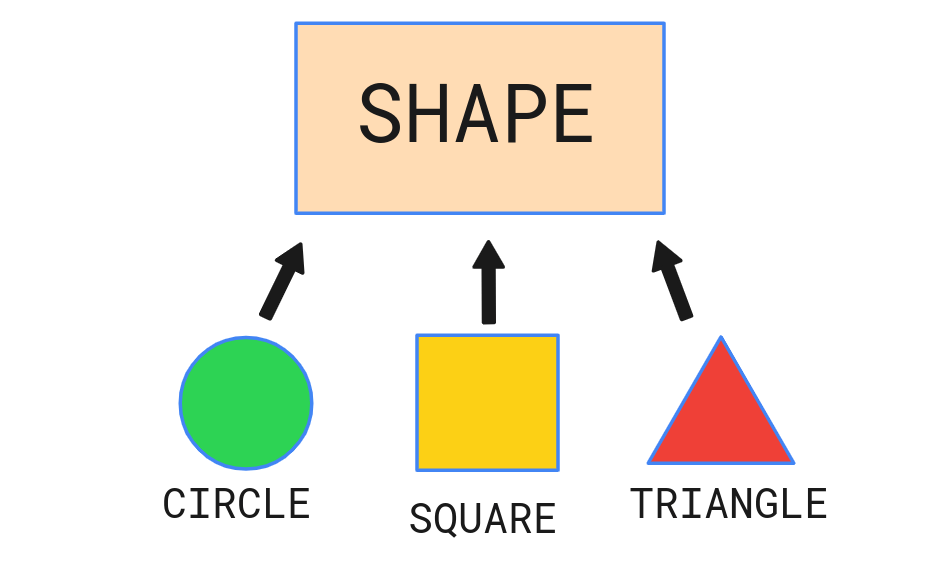
In coding, the result of abstraction consists of carefully chosen attributes and behavior specific to the original object.
Abstraction is one of the key concepts of Object-Oriented Programming (defined later in this article).
Agile Software Development
Agile software development is an iteration-based ideology where software development teams solve customers’ problems quickly.
It is a really common approach to software development and is one of the 21st-century buzzwords that startups and tech companies use.
Instead of launching big, agile teams work in smaller increments. An agile team delivers features that are “just enough” for the customers. This way the teams can cut costs, solve problems quicker, and get feedback sooner.
Agile software development teams have the tools to react to landscape changes rapidly. This is because in agile development, the customer needs, plans, and end results are evaluated constantly.
AI
AI stands for Artificial Intelligence. It is the science of simulating the human intelligence process by computers.
AI programs use linear algebra to analyze data and make predictions on future data based on the analysis. An AI program has the ability to learn from labeled data. When the program receives input, it makes a prediction based on the data it consumed.
Meanwhile, AI is not a new concept, it has become a 21st-century buzzword. There are more cool AI innovations and applications than ever before.
Algorithm
An algorithm is a set of instructions to solve a problem or task. An algorithm is a key concept in programming. Every computer program consists of algorithms.
A typical algorithm:
- Takes input.
- Performs an operation on the input.
- Returns an output as a result.
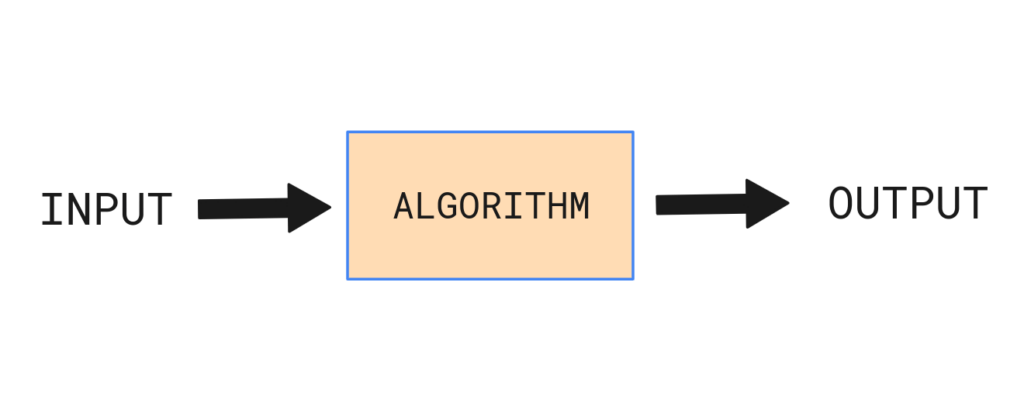
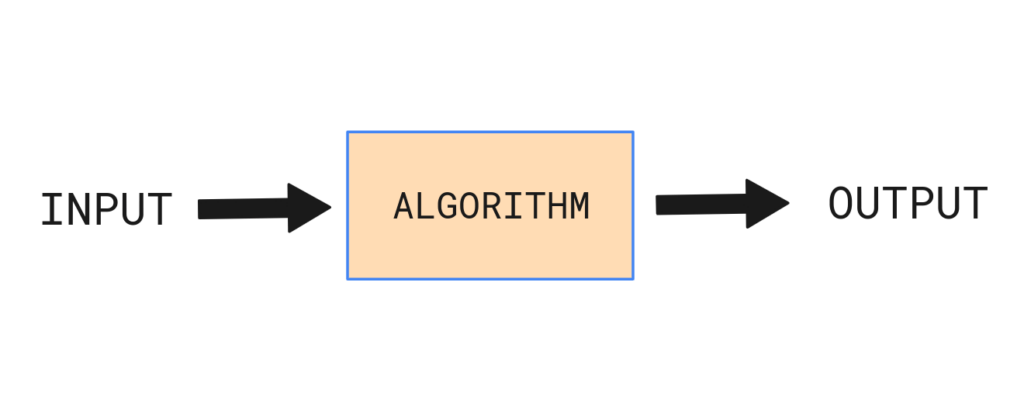
A basic example of an algorithm is calculating the Pythagorean theorem. This algorithm takes the sides of the triangle as input. Then it applies the Pythagorean theorem to the side lengths. As a result, the algorithm returns the length of the hypotenuse.
API
An API or Application Programming Interface allows two applications to communicate with one another.
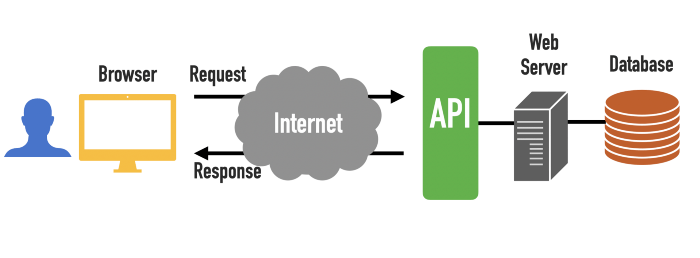
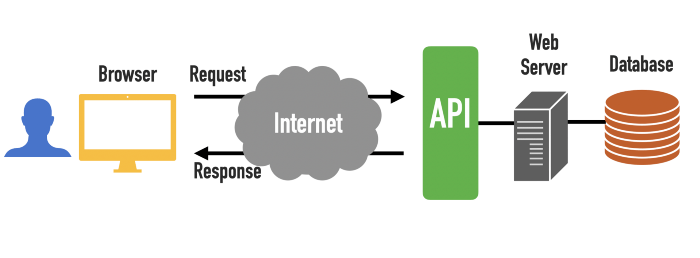
As a programmer, you need to handle data on a regular basis. Typically, the data is not on your computer locally. Instead, the data is stored securely on a remote server.
To access the data on a remote server, your program needs to communicate with an API. The API is a middleman that “speaks” to the server where the data lives. The API retrieves the requested data from the server. It then creates a response object and sends it your way.
Also, you can use API to store data in the database. In this case, you send the data to the API. The API then inserts the data into the database and sends you a response.
A well-designed API makes it easy to retrieve and store data on a remote server.
Make sure to read What Is an API to learn more about APIs.
AR
AR or Augmented Reality is one of the biggest technology trends at the moment.
In AR, the real world is enhanced by adding virtual objects to it. To step into the AR world, you need to have AR glasses or a smartphone with a camera.
As an example, here is an image of AR achieved by an iPad program.
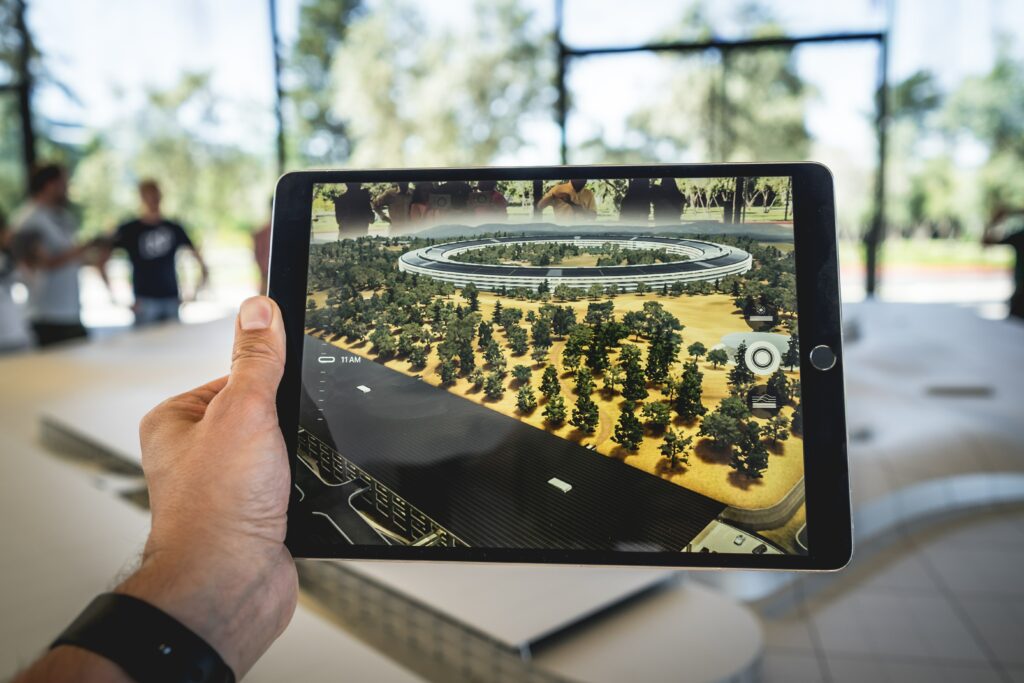
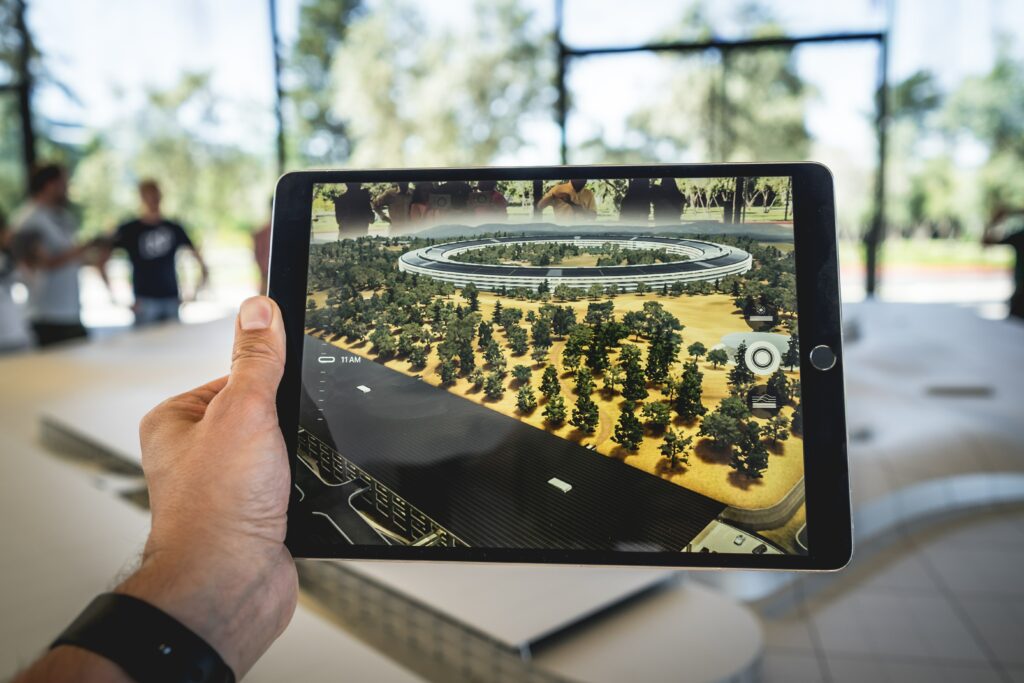
Here the iPad camera points to the table in front of it. The program then lays 3D objects in a realistic way. In an AR scene, it appears as if the virtual objects belonged to reality.
With AR, developers can get creative. For example, you can build an AR scene where UFO lands in the parking lot, and aliens walk out of the ship.
Argument
In programming, an argument is information you pass into a function.
The function then uses the argument to perform an operation and return a result.
The data you pass into a function has many names:
- Argument
- Parameter
- Input
These names are used interchangeably among programmers.
Arithmetic Operators
Arithmetic operators are the “regular math operators” you use every day. The fundamental four arithmetic operators are:
- Addition (+)
- Subtraction (-)
- Multiplication (x)
- Division (÷)
More formally, arithmetic operators are mathematical functions that take two arguments and do a calculation using them.
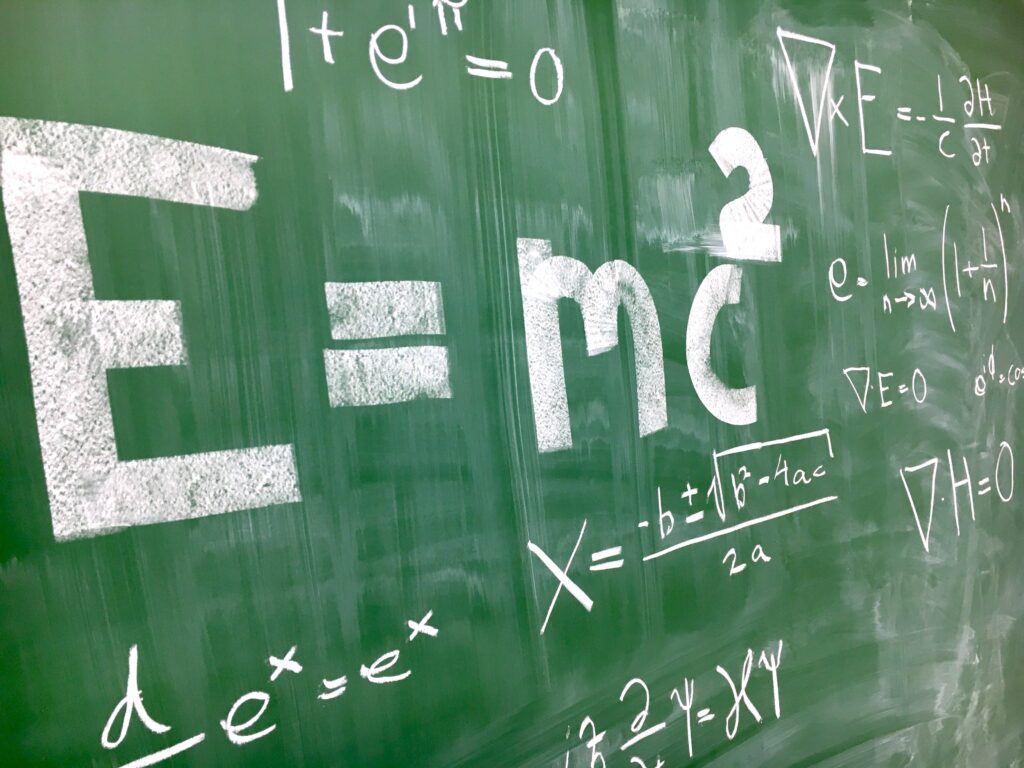
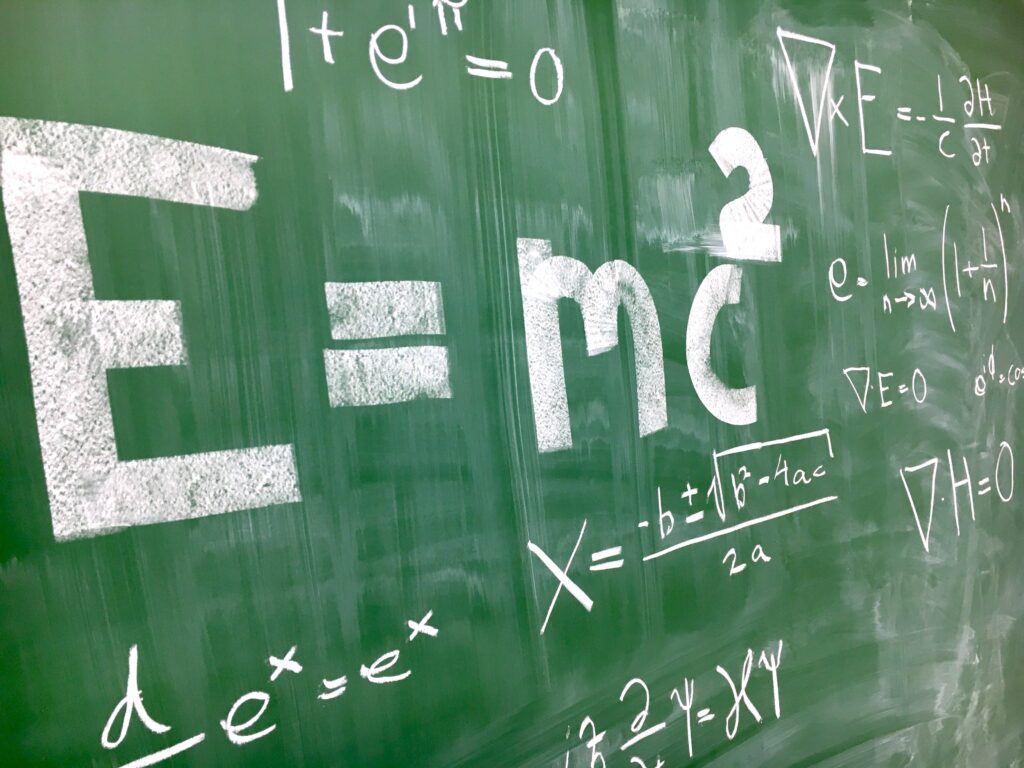
Array
In programming, an array is a collection of objects, such as numbers or names.
["Alice", "Bob", "Charlie", "David"]
It is one of the most commonly used data types in all fields of software development.
Typically, arrays are used to store elements of the same kind. For example, if you have a program that represents students, you might keep all the students in an array for easy access and operations.
In general, arrays are useful because computer programs need to deal with loads of data. This data must be easy to read, access, and modify. This is when it becomes useful to store data in an array.
In programming, it is easy to add, modify, and remove data from an array. An array makes processing large quantities of data easier.
ASCII
ASCII is a shorthand for American Standard Code for Informational Interchange.
Basically, ASCII maps each English alphabet to a number. Here is the ASCII table of the English alphabet:
Unicode | Character | Unicode | Character | Unicode | Character | Unicode | Character |
64 | @ | 80 | P | 96 | ` | 112 | p |
65 | A | 81 | Q | 97 | a | 113 | q |
66 | B | 82 | R | 98 | b | 114 | r |
67 | C | 83 | S | 99 | c | 115 | s |
68 | D | 84 | T | 100 | d | 116 | t |
69 | E | 85 | U | 101 | e | 117 | u |
70 | F | 86 | V | 102 | f | 118 | v |
71 | G | 87 | W | 103 | g | 119 | w |
72 | H | 88 | X | 104 | h | 120 | x |
73 | I | 89 | Y | 105 | i | 121 | y |
74 | J | 90 | Z | 106 | j | 122 | z |
75 | K | 91 | [ | 107 | k | 123 | { |
76 | L | 92 | \ | 108 | l | 124 | | |
77 | M | 93 | ] | 109 | m | 125 | } |
78 | N | 94 | ^ | 110 | n | 126 | ~ |
79 | O | 95 | _ | 111 | o |
These ASCII values exist because a computer doesn’t know what are letters. The computers only know about 0s and 1s. The ASCII values are numbers that have binary representations (0s and 1s) that the computer knows.
Asynchronous
Asynchronous (or async) means events that don’t occur at the same time, thus being “out of sync”.
When you perform a network request, you want to use an asynchronous function. This is because you don’t want to halt the entire program to wait for the response. This is a typical use case for asynchronous code.
A real-life example of something being asynchronous is messaging. When you send an email, you don’t expect an immediate response. Instead, the response can arrive at any moment in time without affecting you.
Asynchronous tasks don’t occur at fixed intervals. Thus, they cannot depend on one another. Instead, async tasks take place independently to serve a separate purpose.
Backend
To put it short, the backend is part of a computer program inaccessible to the user.
The data of a program or application is stored in the backend in a database. For example, usernames and profiles of a social media app are stored in a backend.
The idea of apps and programs is that the end user uses the front end to interact with the data and logic in the backend.
For example, when you change your social media profile picture, you use the front end of the app to store the picture on the backend.
Binary System
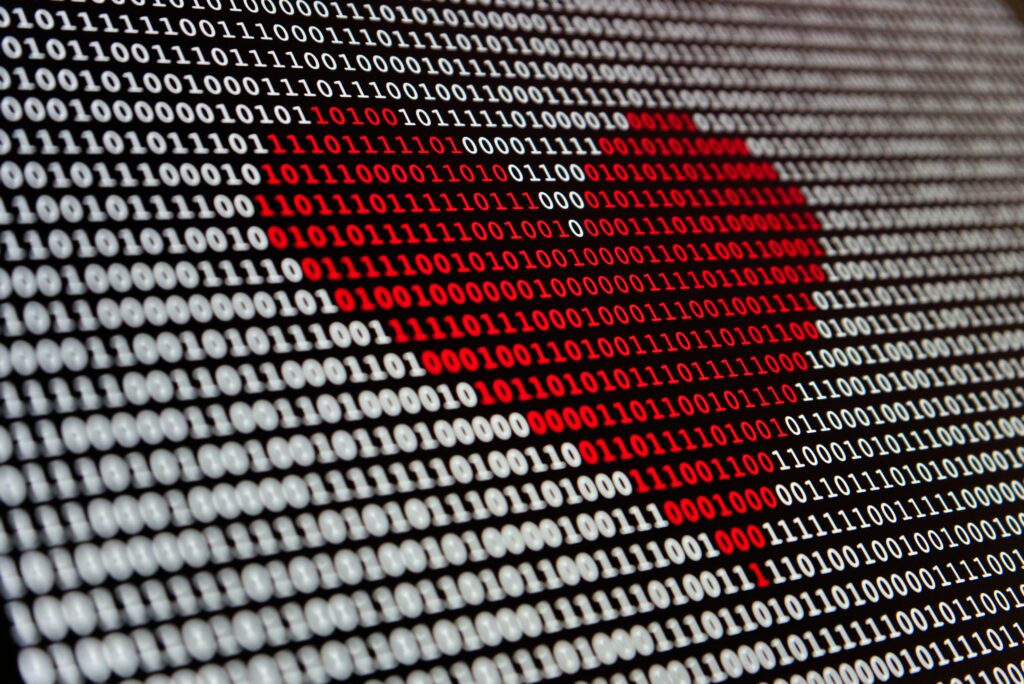
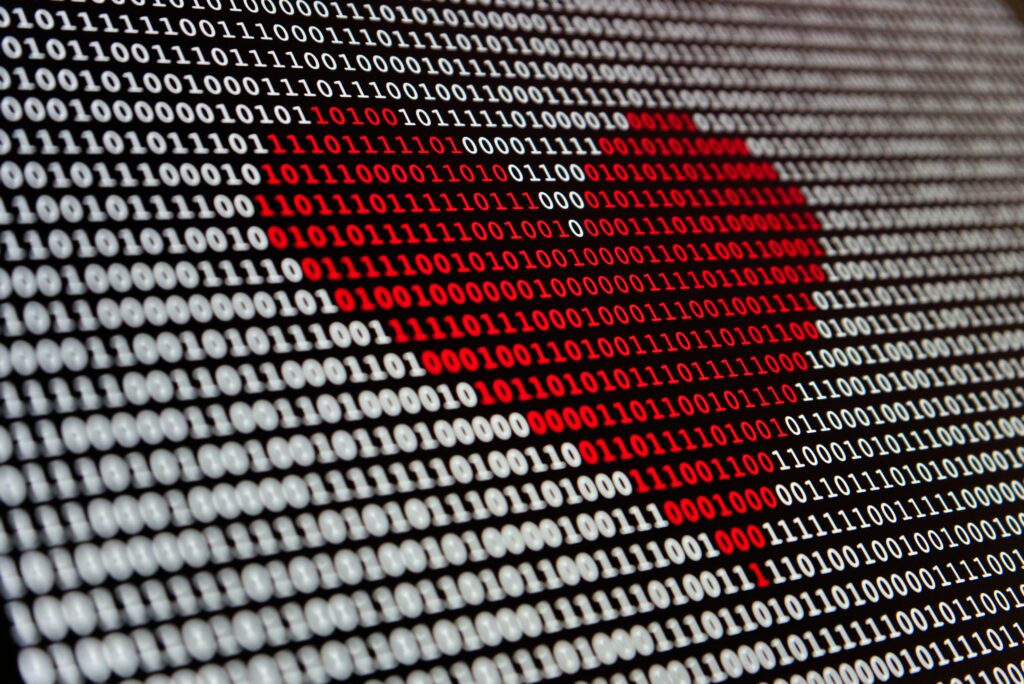
A binary system uses two numbers, 0 and 1, to perform calculations.
Because a computer only understands 0s and 1s, it has to use a binary number system to do calculations.
The “traditional” number system you use every day is the decimal system. In the decimal system, you can use numbers 0-9 to perform calculations. When you exceed the number 9, you need to reuse the numbers 0-9. For example, the number 15 is a combination of 1 and 5.
The same idea applies to the binary system. Except there are only two numbers to use instead of the ten in our decimal system.
This means you can only calculate to 1 in a binary system with unique numbers. After 1, you must start reusing the 0s and 1s.
For example, let’s count to eight in binary:
0 1 10 11 100 101 110 111
Learn more about binary numbers by reading Binary Number System
Bit
In programming, a bit is a small unit of information. A bit is a shorthand for a binary digit.
The bit can hold one of two values: 0 or 1. In electrical terms, a bit can be on or off.
The bit is a fundamental information unit that forms the basis for all modern-day computers.
Because bits are so small units of information, you typically don’t work with one of them at a time. Instead, the usual arrangement found in computers combines eight bits to form a byte.
With eight bits you can create 2⁸=256 unique arrangements of 0s and 1s. In other words, you can calculate from 0 to 255 using eight bits.
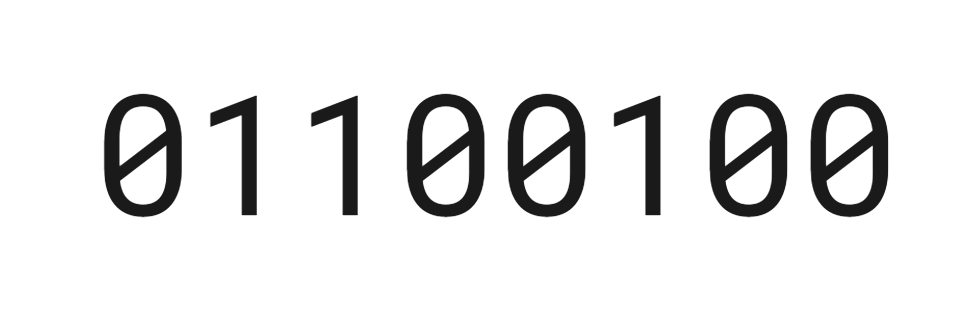
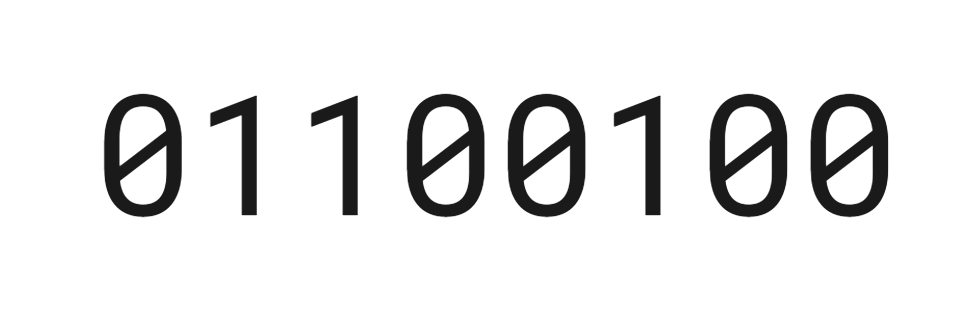
A byte is thus big enough that it can represent all the letters in the English alphabet.
In the ASCII table (earlier in this article), the letter “d” has an ASCII value of 100. If you convert this to binary, you get 01100100. Thus, to store “d” in a byte, the bits have to be turned on or off as follows: 01100100.
Boolean
Boolean values represent something being either true or false.
More formally, a boolean is a datatype that represents the truth. It has two and only two possible values, true or false.
Boolean values are used in every computer program. With boolean values, you can introduce logic to your code.
If something is true, you perform one action, if it’s false, you perform another. Without boolean values, computer programs would be very basic and couldn’t get much done.
Bug
In programming, a bug is an unexpected problem that causes the program to crash or behave unexpectedly.
There are many types of bugs that can occur. These include:
- Token errors
- Syntax errors
- Syntax constraint errors
- Execution errors
- Intent errors
Bugs are typically what cause headaches for the developer. Programmers deal with countless bugs on daily basis.
Build
A build is a computer program that is converted into a standalone format.
During the build phase, your source code is converted into machine-level code executable by your computer. This process is called compilation.
A build is typically created when the code is ready for testing or a release.
The complexity of a build can vary. In the simplest case, a software developer can build the program using their desktop and IDE.
On the other hand, a build can be a complex process where professional assistance and tools are needed.
Byte
A byte is an arrangement of eight bits.
Notice that there is no standard definition of the size of a byte. So there could be systems where the number of bits in a byte is not eight.
A typical computer uses bytes to represent characters, such as letters, numbers, or other typographic symbols.
Make sure to read about Bits earlier in this list to see how bytes are put together by bits.
C
C is one of the most venerable and popular programming languages to date. It is a coding language applicable to many areas of software development.
Some people treat C as the base for any programming language. If you understand C, you can learn any other language.
C is as low-level programming language as it gets. It is characterized by technical memory management features. No other general-purpose programming language gets you as close to the hardware level as C.
C is typically used in programming embedded systems, operating systems, databases, IoT, and compilers.
C++
C++ is a very similar language to C. So much so that you can compile about 99% of C programs without changing a single line of code in C++.
The reason why C++ has become so popular is that it’s a more general-purpose language than C.
As the name C++ suggests, it’s an “upgraded C”. The ++ is a code notation and means to add one. So in code, C++ is the same as C = C + 1.
C++ language is a powerful and flexible language applicable to many areas of software development. It’s most commonly used in:
- Game development
- Browser development
- Operating systems
- Maths and data science
Call
In programming, a call means requesting a service from another part of the program. Typically, you call functions in your program code.
For example, to get the distance between two game characters, you can call a distance function that uses the Pythagorean theorem to measure the distance.
The called part of the code is responsible to return the control of the program back to the caller. This means the program logically continues from where it made the call.
Camel Case
Camel case is a common typographical convention for programmers.
In the camel case, word combinations that have no spaces are separated by capital first letters.
For example myFancyHouse.
The reason why the camel case is popular in programming is that typical coding syntax doesn’t allow blank spaces. Thus, the combinations of multiple words cannot have spaces and must be represented in another readable manner. The Camel case is one example of a commonly used convention.
If the first letter of a camel-cased word is uppercased, it’s called Pascal Case. For example, file names and class names are typically Pascal-cased in coding.
Read more about the camel case and case styles in coding.
Class
A class is one of the basic building blocks of a computer program. Programmers define classes that act as blueprints for creating objects in the code.
The idea of a class is to group together related values and behavior.
For example, in a social media app, there could be a class for a User. The User class holds reusable code and properties, such as name, picture, hobbies, and more.
Client-Side
Client-side is a popular term in web development.
The Client-side refers to everything that an end user sees. It is quite literally the side that the clients get to see.
On a web page, client-side refers to:
- Text
- Images
- Any other UI component visible/accessible to you
- Actions that your browser performs
For example, the markup languages HTML and CSS are interpreted on the client side. This simply means your browser renders the web page.
Opposition to the client side is server-side. The server-side things happen remotely on a server and are inaccessible to the client. For example, when you log in to your favorite website, the authentication takes place server-side.
Cloud
Cloud refers to the vast amount of servers in data centers across the globe. These servers are accessible via the Internet.
These days, more and more services are cloud-based. This helps companies save money and introduce convenience for users.
Instead of having programs on your desktop, they live in the cloud and are accessible as long as you have an internet connection.
When you are using cloud apps, there is practically no risk of losing data. If your computer crashes or gets stolen, all your data is still accessible via the cloud. This is the benefit of the cloud for regular internet users.
For companies, the cloud can be a great time and money saver. Instead of updating and maintaining their own servers, companies can let the cloud vendor take care of it.
This option is typically cheaper and thus more affordable for smaller businesses. Also, cloud-based apps help internationalize the company. The services can be accessed all around the world.
Code
Code or program code is a set of instructions written for a computer to perform desired actions.
The code is written in some programming language, such as Python, JavaScript, or Java.
To write code, you typically need to create a code file and write the code with a code editor. These days, code can also be written to cloud-based online editors without having to worry about file types or code editing tools.
Learn more about programming as a concept by reading What Is Programming.
Code Review
Code review is a process used by teams to make sure code is of high enough quality and follows the best practices.
When developing software in teams, the developers must follow best practices and write high-quality, readable, and maintainable code. Otherwise, the codebase becomes a mess and infeasible to maintain. More importantly, the code must make sense and pose no risk to the project.
Because there are as many coding approaches as there are developers, there must be team-specific best practices and guidelines. To enforce these, the developer of a new code piece asks for a code review from a teammate. This might involve some back and forth to make the code good.
Once the code review is accepted, the code is ready to go live.
Coding Language
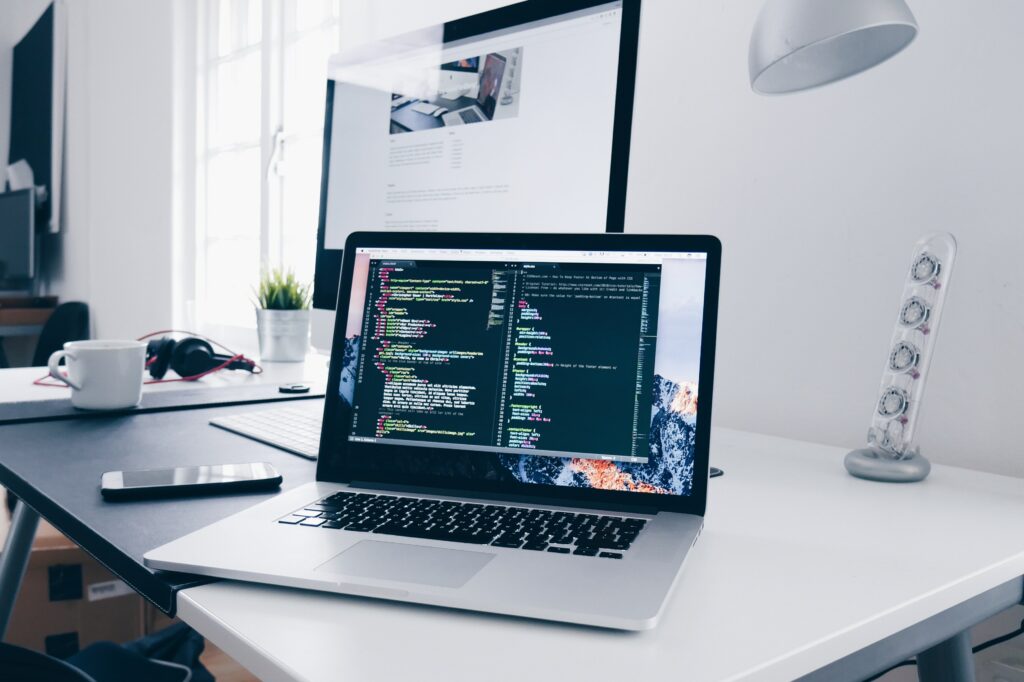
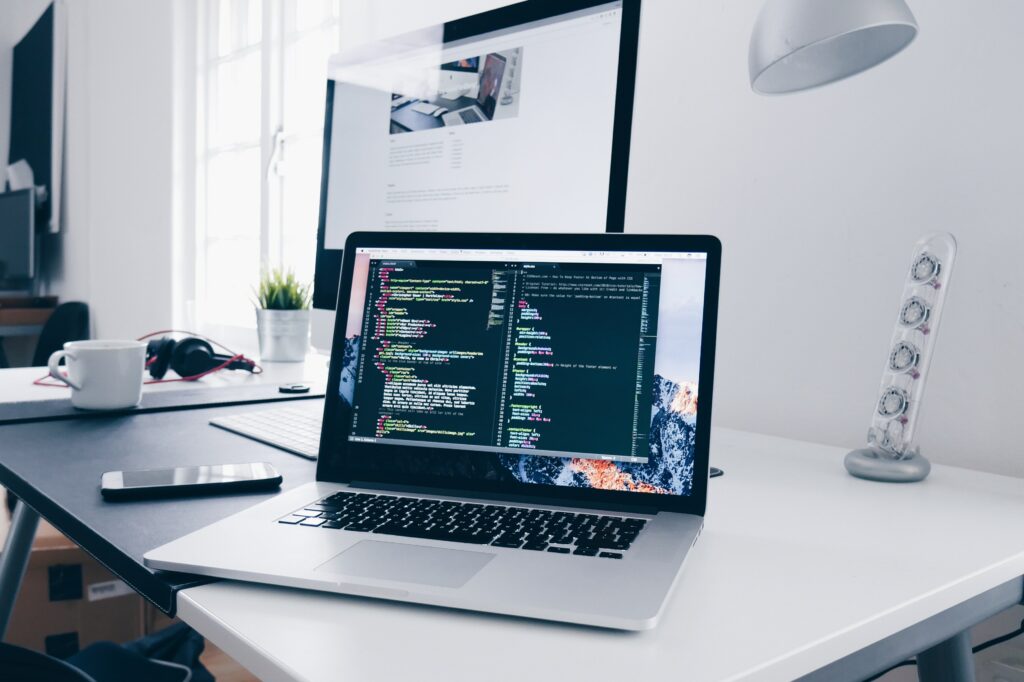
Coding language is a language that both developers and computers understand. It is the missing link between the human and the hardware.
When writing a program, some task-specific programming language is used.
For example, if you want to create an iOS mobile app, you typically use Swift programming language.
When you run the program, the code gets translated into a machine-level language that only the computer can understand.
Command
A command is typically a one-line instruction given to an application to perform an action.
For example, you can run a shutdown command on a command line interface. This shuts down your computer after a specified number of seconds.


Command-Line Interface (CLI)
Command-Line Interface (CLI) is a program that accepts text-based commands to perform functions on your operating system.
Back in the 1960s, CLIs were the only way to interact with computers.
These days, GUIs (Graphical User Interfaces) are used instead. These make using the software much easier for the end user.
For example, instead of typing a command to download a file, you can click a “download” button.
Compiler
A compiler translates program code to machine-level code.
A programming language is something the developers understand. But a machine doesn’t understand programming language as-is. Instead, a machine only understands low-level machine code that eventually is just 0s and 1s.
To run a program, the code must be compiled for the machine to understand. This is where a compiler is used.
The compiler can also translate code to something known as bytecode or even into another programming language.
Another way to translate code to machine-level language is by using interpretation. See “Interpreter” in this article to learn more.
Computer Science
Computer science is a software-centric study of computers and other computational systems.
Computer scientists spend time with software theory, design, and development. A computer scientist doesn’t deal with hardware. Instead, the study focuses on the software side of computers.
Some of the key studies of computer science include:
- Data Science
- Machine Learning
- Artificial Intelligence
- Software Engineering
- Programming Languages
- Database Systems
- Computer Graphics
- Numerical Analysis
And much more.
Studying computer science is possible in universities as well as online. Computer science is one of those skills where you can become a self-taught expert and have no education to succeed.
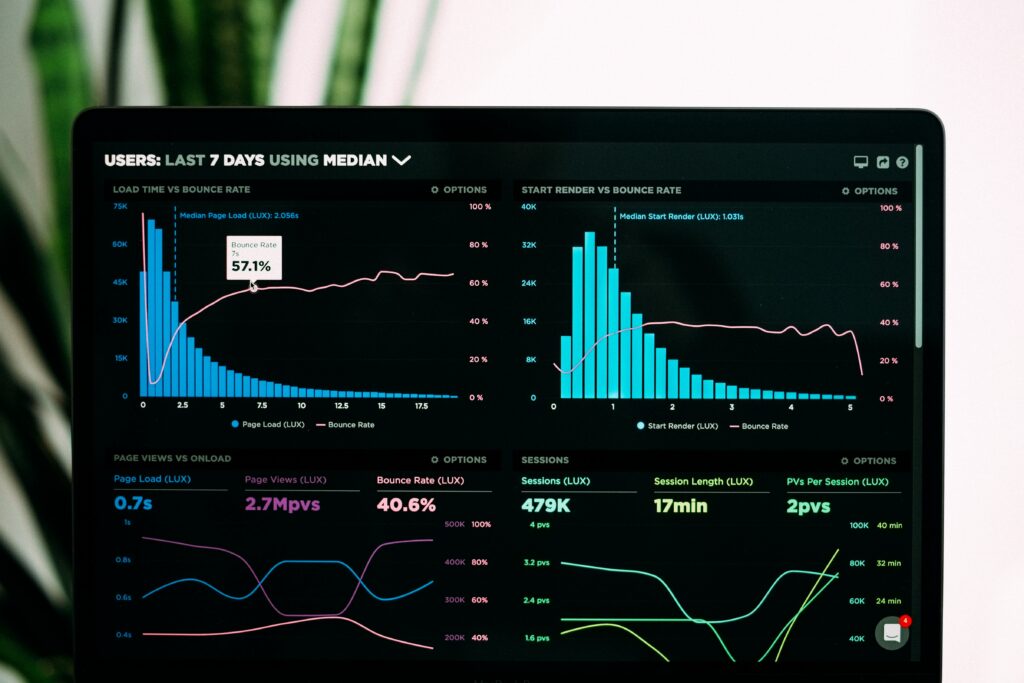
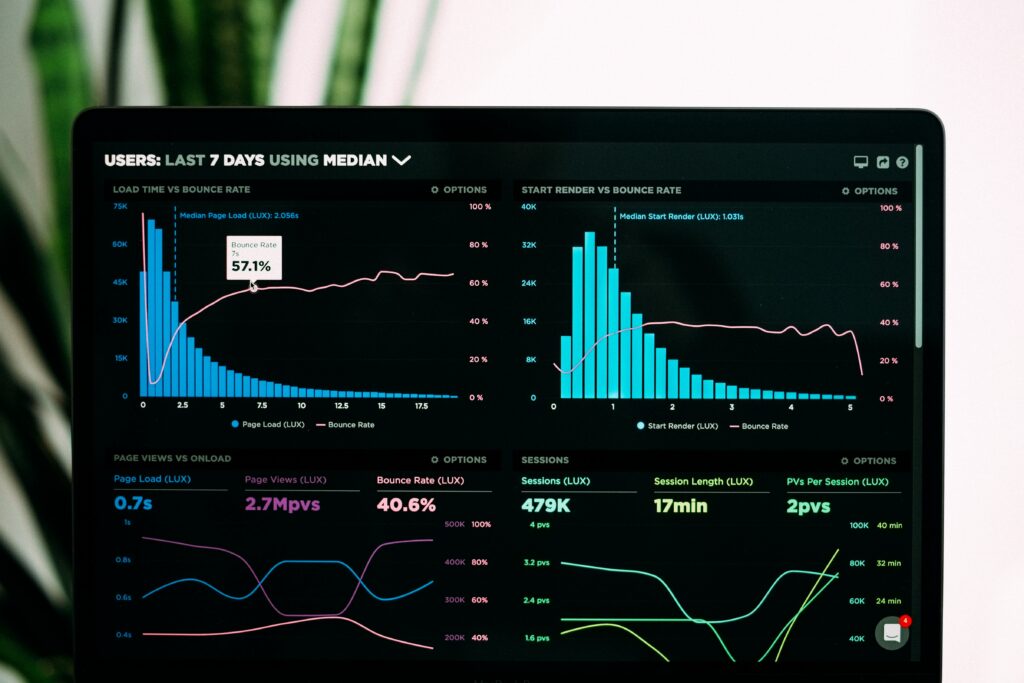
Conditional Statements
In programming, you can use conditional statements to check if a condition is true or false.
With conditional statements, the computer can make decisions. The conditional statements bring logic to the code.
A typical conditional statement is an if-else statement.
if age > 18: print("You can drive") else: print("You cannot drive")
Constants
Constants refer to values that aren’t supposed to change. These values remain constant during the execution of the program.
CSS
CSS or Cascading Style Sheets make it possible to stylize web pages. It is a simple design language that brings an HTML page to life.
CSS is not a programming language. Instead, it’s a style-sheet language that works in conjunction with the markup language HTML or XML.
With CSS, web developers can separate the presentation of the web page into a separate style sheet. This makes the code more accessible and easier to maintain.
A typical web page consists of three separate files:
- HTML. All the web page components, like text, colors, backgrounds, and forms are created using HTML. These are the building blocks of a web page.
- JavaScript. JavaScript brings interaction to web pages. In a sense, it wires some of the HTML components to perform a specific function, such as an action when clicking a button.
- CSS. With CSS, a web developer controls what the HTML components look like. For example, a CSS command can make all HTML buttons have round corners.
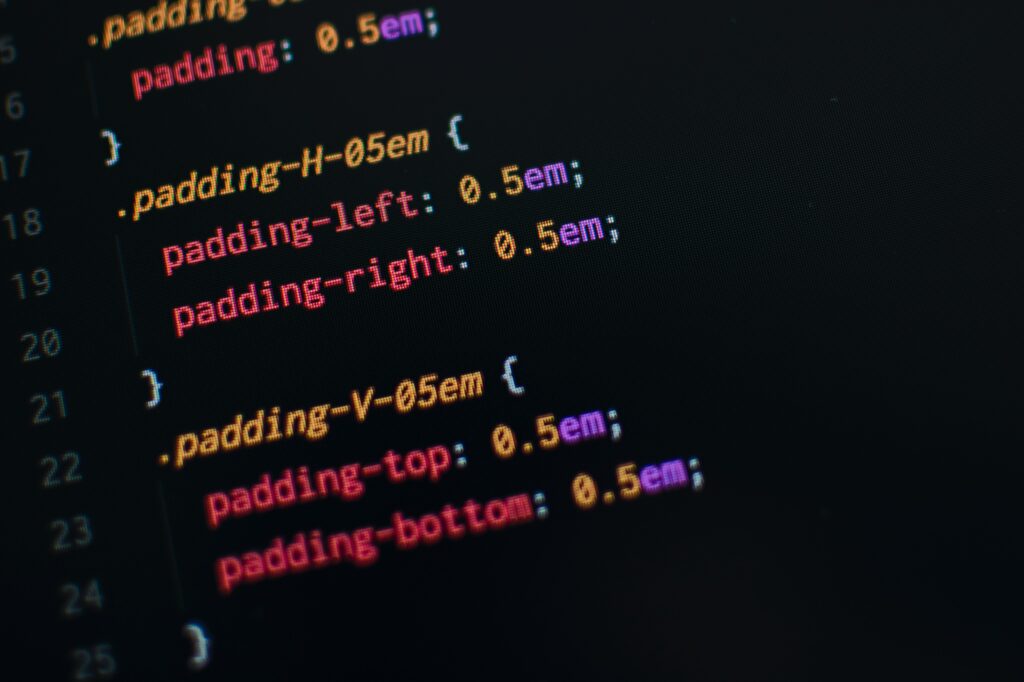
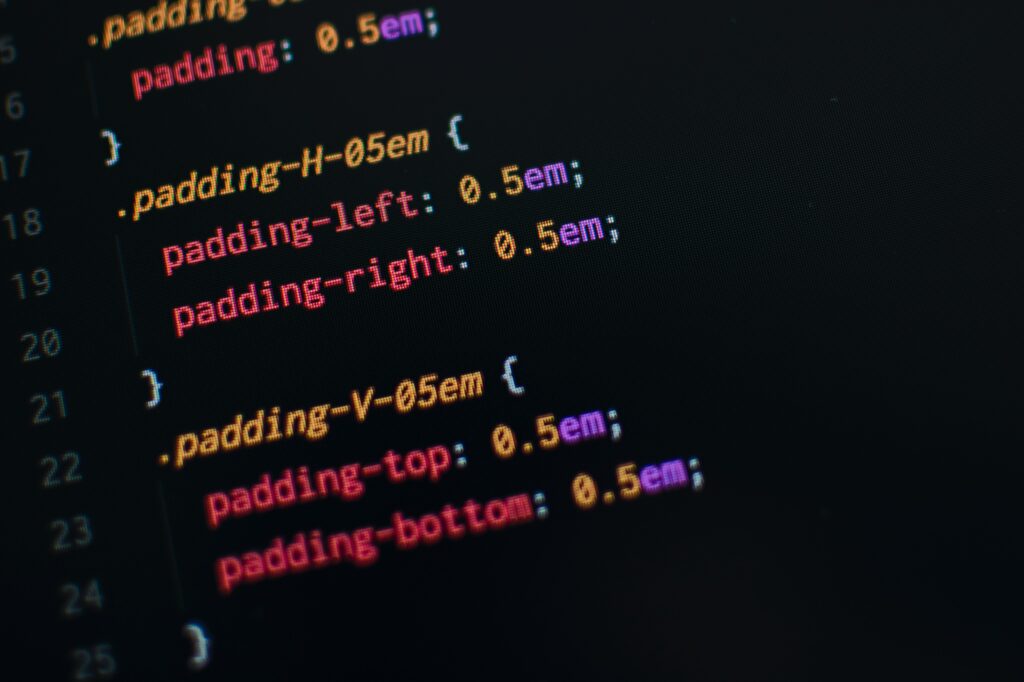
Database
A database is a place where a computer program can store data.
Depending on the application and use case, a database can be found on your machine locally. But usually, a database refers to a remote storage system.
For example, on a social media app, a database stores the information of its users, such as the username or profile picture.
Databases don’t just throw some random data into them. Instead, databases are organized with structured information. The database is typically controlled by a database management system (DBMS).
Commonly, a database models data as rows and columns on a table. This makes data processing and accessing lightweight.
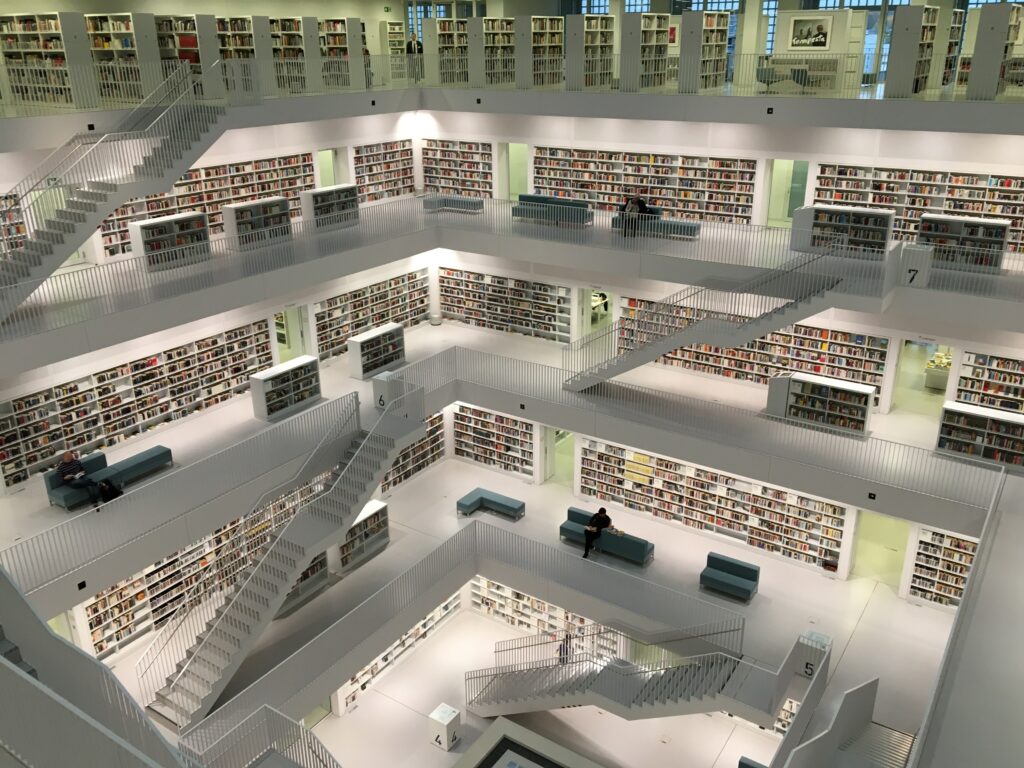
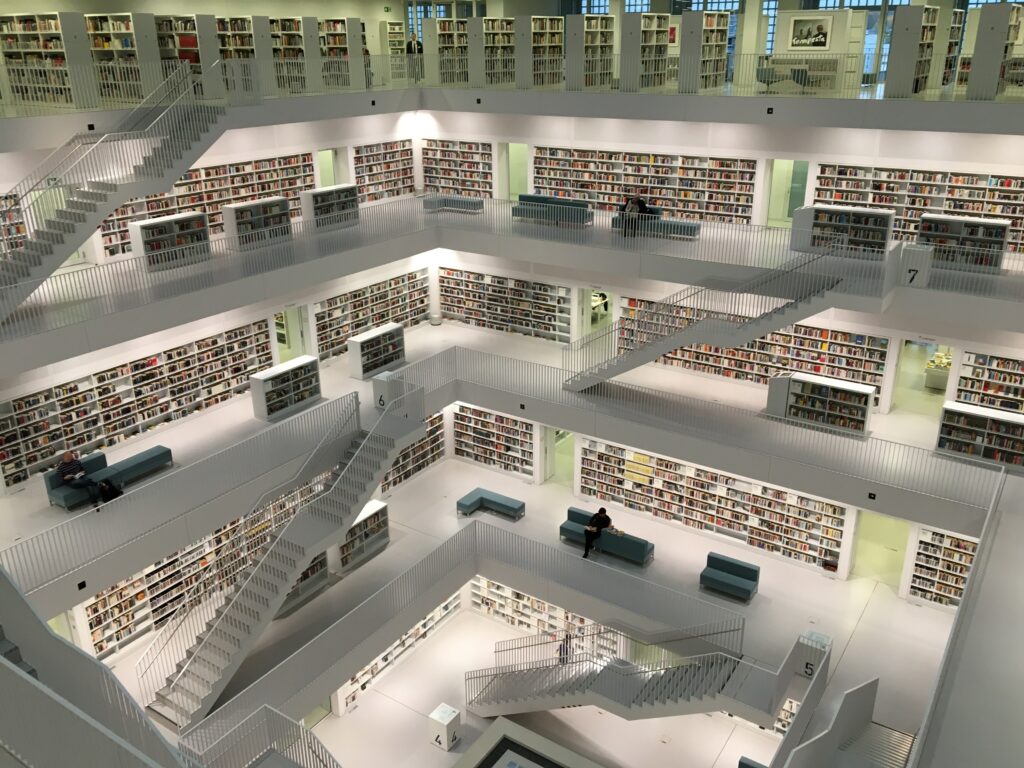
Data Type
In programming, you have to deal with lots of data. This data can be numbers, text, fractional numbers, or truth values.
But a computer doesn’t know what is “text” or “number”. Thus, programming languages define the different possible data types. Data types make it possible to handle different types of data. You can perform mathematical, relational, or logical operations on the data based on its type.
Common data types in programming are:
- String for alphanumeric characters.
- Integer for whole numbers.
- Float for numbers with decimal points.
- Character for numeric encoding of the text.
- Boolean for logical values (true or false).
Debugging
Debugging is the process of hunting bugs in a program.
The main goal is to track down the line of code that causes problems. Developers spend a lot of time debugging their code. Debugging may take hours and can be really frustrating. Worse yet, sometimes the issue was caused by something silly, such as a typo.
There are special debugging tools that are typically present in code editors. These tools let you manually run the code and pause the execution on a particular line to inspect the values and behavior.
Declaration
In programming, declaration means creating the name and data type of a new code element, such as a variable or constant.
The declaration is common action and takes place in every single program.
For example, to create a number, you can declare it as a variable or constant of a type integer.
Deployment
Deployment is the process of shipping code from developers to the users.
You can deploy:
- Applications
- Updates
- Modules
- Patches
DNS
DNS stands for Domain Name System.
Each website or web resource has an IP address, which is something like 241.152.131.208.
But because this is hard to memorize, a human-readable URL is used instead, such as apple.com.
When a browser visits a website, it still uses the IP address to navigate through the internet.
This is what the DNS does.
The DNS converts the human-readable URL to an IP address of the resource. The browser then uses this IP address to request data to “visit” the page.
Downtime
Downtime is the time during which a program is down or unavailable for use.
There are many reasons why applications have periods of downtime. These include:
- Hardware failure
- Power failure
- System crashes
- System reboots
- Operating system updates
- Software updates
- Network connectivity issues
Some downtimes are planned because they belong to the maintenance of the software. But more often than not, downtime is a result of an unplanned event.
DRY
DRY stands for Don’t Repeat Yourself.
It’s a principle that promotes cleaner and more maintainable code.
The idea of DRY is to not introduce unnecessary repetition and patterns in a codebase. Instead, each piece of code should have a single and clear representation in the codebase.
For example, if you need to calculate the distance in a game app, you shouldn’t repeat the Pythagorean theorem everywhere. Instead, you should create a single function that does the calculation and call that function everywhere.
Endless Loop
An endless loop (or infinite loop) is an accidental condition in programming where a piece of code is repeated forever.
An endless loop is caused by a developer who forgot to update the looping condition to exit the loop. When this happens, the program crashes as all the computing power is focused on maintaining the loop.
To terminate an endless loop, the program must be stopped entirely.
Although an endless loop sounds bad, it doesn’t break your computer. It only stalls the program and requires some code fixes before a re-run.
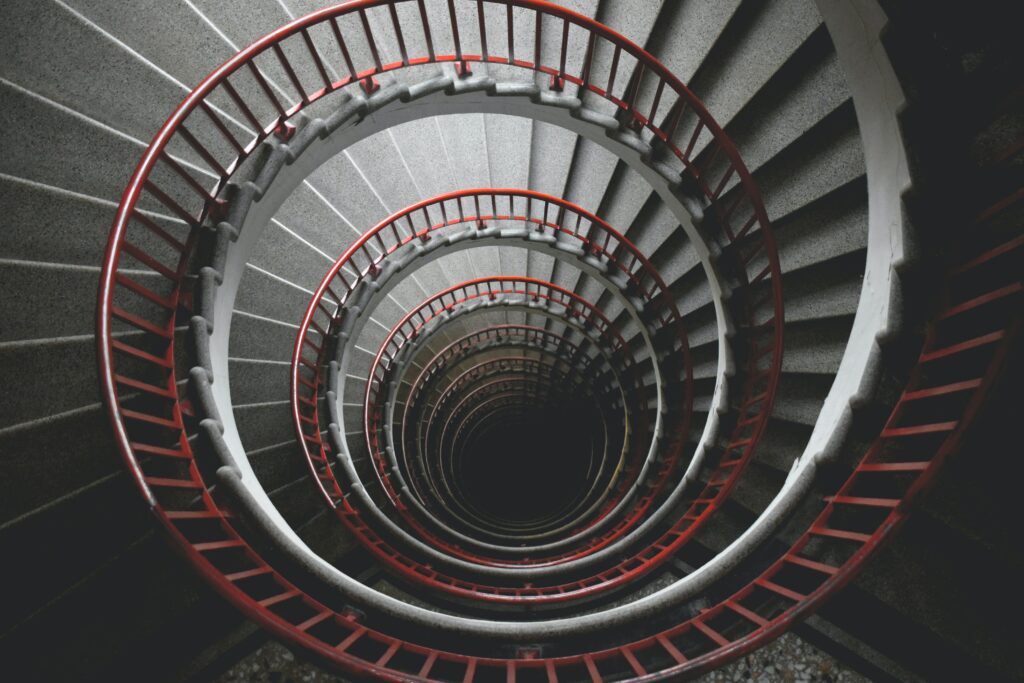
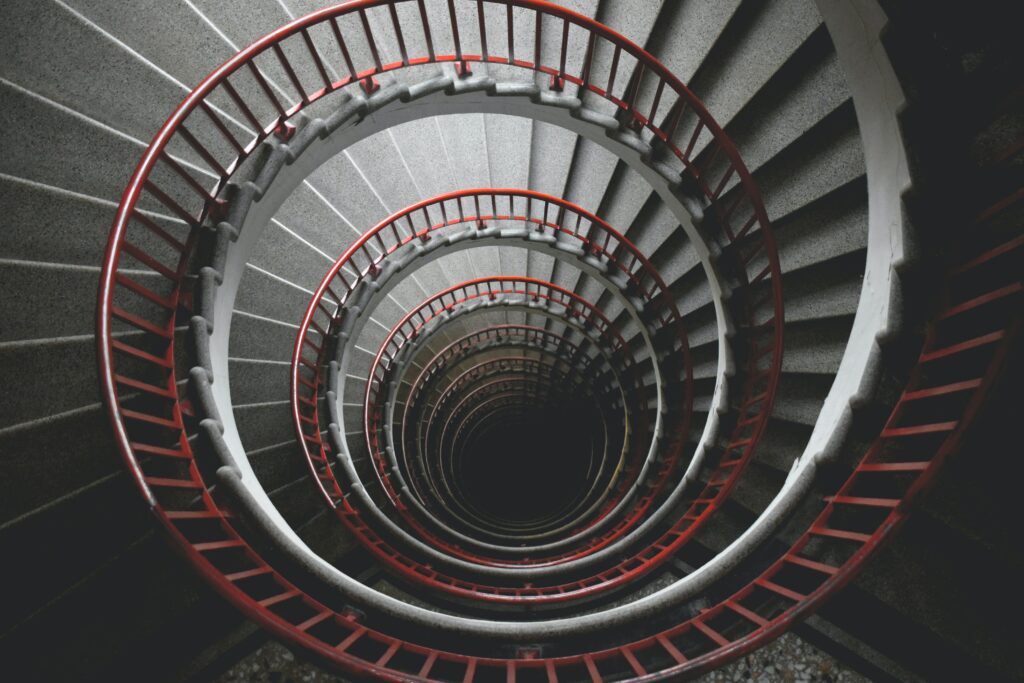
Environment Variable
An environment variable is a variable that lives outside a program. Typically, an environment variable lives in the operating system or microservice.
Environment variables are useful when the software uses information specific to the developer’s computer.
To simplify environment variables, consider this example.
Alice, Bob, and Charlie develop a game app together. For personal preferences, each developer wants to use different background colors when testing the app.
- Alice uses red.
- Bob uses green.
- Charlie uses blue.
The background color of the app is specified in the code.
Here is the problem. When Alice starts developing the app, she must update the background color to red first. This means the color becomes red for Bob and Charlie too. So when Bob or Charlie wants to develop the app, they must change the color to green or blue.
This back-and-forth takes time and eats up resources.
Here is where an environment variable helps. Each developer specifies a color as an environment variable on their device. When it’s time to develop, the program then pulls the color from the environment and inserts it into the code. This way the color is always correct for the three developers.
Exception
An exception is an unplanned event. It is a shorthand for “Exceptional event”.
When there is an exception in a program, the normal flow of the program is disrupted.
Examples of exceptions include falsely formatted user input or network connection issues.
If an exception is not handled properly in the code, the program crashes.
Expression
Expression is a written statement in code. A typical expression takes one line of code and is thus short.
An expression is a piece of code written by a developer. The simplest form of expression is a mathematical equation, such as 5 + 10. These are typically called arithmetic expressions.
Framework
One of the essential tools in programming is called a framework.
A framework has built-in code solutions that you can reuse as a developer. Frameworks speed up the development process as you don’t need to spend time reinventing the wheel.
In case you are new to programming, it’s untypical to code everything from scratch. Instead, a developer uses ready-made building blocks to build the software. Bigger software projects might use multiple frameworks and external dependencies to streamline the development process.
Front-End
The front end is the “user side” of a program. Sometimes front-end is also called the client side.
A front-end developer develops the client side of the web app. This typically involves using HTML, CSS, JavaScript, or Frameworks like Angular.js or React.
If you visit a web app like Instagram or TikTok, you are interacting with the front end of the software in technical terms.
Function
A function is a reusable chunk of code.
The purpose of a function is to bundle a specific behavior or action together for easy reuse.
Whenever you need the function, you don’t have to re-implement the behavior. Instead, you can call the function.
Typical software is made up of countless functions. The main purpose of the functions is to break down the problem into smaller chunks of code. This makes the code easier to read and maintain.
For example, you could write a function that calculates the volume of a sphere. Then, wherever you need to calculate the volume, you can call the function instead of re-writing the equation.
Git
Git is a version control system for software development projects.
With Git, you can handle small hobby projects as well as large enterprise-level software projects.
Git is software that runs locally on your machine. Its main function is to track changes to your code, thus the name version control.
When you make a change to the codebase, you can commit the changes to Git. If you, later on, notice that the change broke your program, you can use Git to travel back in time to revert the change.
Git keeps track of all the changes you ever make to your code. This makes it possible to travel back in time to the very first lines of code you committed to the project.
GitHub
GitHub is a cloud-based hosting service for Git.
As you learned earlier, Git runs locally on your computer. But if you connect the project to GitHub, you can store the project in the cloud.
GitHub is useful for development teams. Without a version control like GitHub, developers would need to send code changes using channels like Slack or Dropbox. This would make software development infeasible.
Thanks to GitHub, this is not the case.
On GitHub, there is one main version of your program. Then, each developer can create independent branches of the main version. Each branch is an independent copy of the original software.
Inside the branch, a developer can make code changes and test them safely without affecting the main program. When it’s time to go live with the changes, the developer requests a review of the changes before merging them into the main branch.
GitHub also supports deployments and continuous integrations. This means the live version of the software is updated whenever you make changes to the main branch of your program.
Hardcode
Hardcoding means placing data directly into the source code, instead of getting the data from a central location.
Usually, hard coding is considered bad practice. This is because hardcoded values must be manually changed everywhere. If there was a single source of truth, one would only need to change it once if necessary.
High-Level Language
A high-level language is a more user-friendly programming language.
In technical terms, a high-level language comes with a higher level of abstraction. This means the programming language doesn’t focus on the underlying hardware components, such as:
- Memory management
- Register utilization
Practically speaking, all modern-day programming languages are high-level languages. These include Python, C, Java, JavaScript, and much more. Although some people consider C being between a high-level and a low-level language.
HTML
HTML stands for Hypertext Markup Language.
As the name suggests, HTML is not a programming language. Instead, it’s a markup language.
HTML is the de-facto language for creating web pages. With HTML, web developers structure web page components, such as sections, paragraphs, and forms.
But don’t forget about CSS and JavaScript. With HTML, websites would look boring and have no colors or interaction.
When the HTML components are placed, a developer stylized them with CSS and adds interaction with JavaScript.
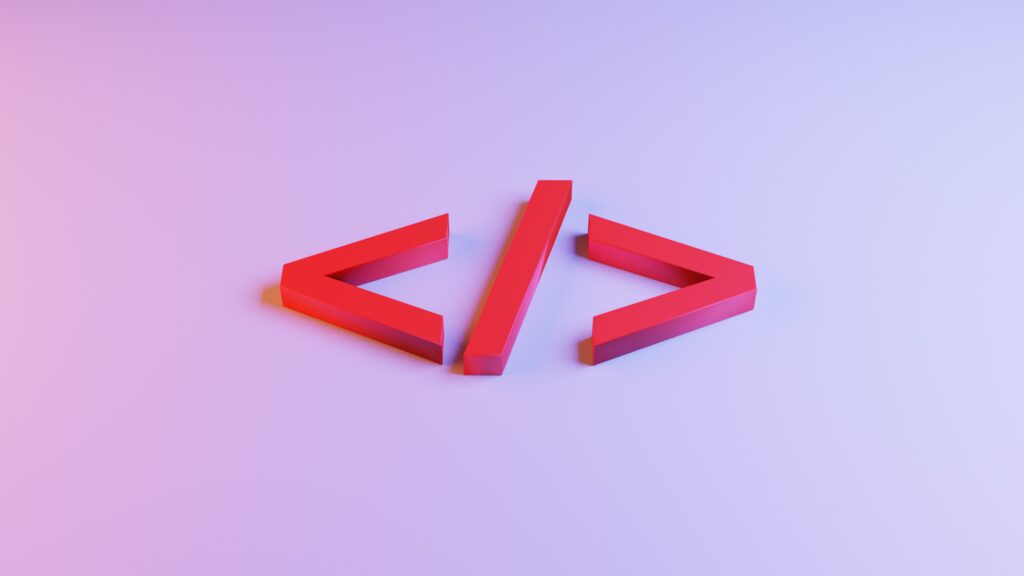
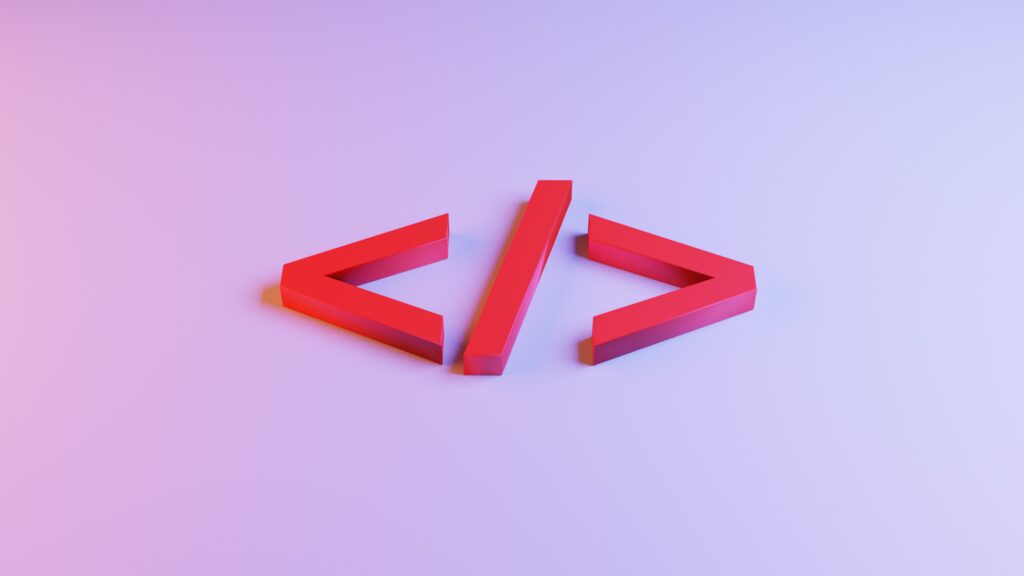
HTTP Request
An HTTP request is a network call performed by a client to access a resource on a remote server.
Typically, you perform an HTTP request to access a website behind a URL.
When you “visit” a web page, the browser doesn’t actually visit anywhere. Instead, the browser performs an HTTP request to a web server to retrieve a bunch of files. These files are HTML files that make up the web page.
Due to security concerns, these days a more secure request type, HTTPS, is being used. The difference between HTTP and HTTPS is that the latter uses TLS to encrypt the connection. So if a hacker were to intercept the request, they wouldn’t be able to do much with it.
IDE
IDE or Integrated Development Environment is a tool where programmers can build applications.
It is a combination of all the necessities in coding. With an IDE, you can:
- Edit source code
- Debug the software
- Build and run the program
Some of the most popular and well-rounded IDEs include VSCode, Eclipse, and NetBeans.
Inheritance
Inheritance is a commonly used concept in object-oriented programming.
The idea is that you can inherit properties from a parent class to a child class. This way you don’t have to rewrite the same properties or behavior. Instead, you can inherit and reuse.
For example, if a developer has a class called Pet, they can inherit properties, such as owner, to subclasses Dog, Cat, and Rabbit.
Input
Input refers to data entered into a program.
Typically, input is given either by a developer or the end user.
A program that takes input uses the input to perform some function. Typically, it also returns an output.
For example, a program that takes a name as input might return a greeting as an output.
input: "Alice" --> output: "Hello, Alice!"
Interpreter
An interpreter translates source code to machine-level code.
Humans don’t understand machine code. Machines don’t understand humans. This is why programming languages exist.
A programming language is an interlink between a machine and a human.
But machines don’t understand programming languages either! Instead, they only do 0s and 1s.
This is why every single piece of code has to be translated to the machine. This is what an interpreter does. The interpreter goes through your code and translates it to the machine line by line.
If you read about compilers earlier, you may wonder what is the difference between an interpreter and a compiler. To put it short:
- An interpreter translates one line of code at a time. This makes it fast to analyze the code. But the overall execution time takes longer.
- A compiler scans the entire program. It translates the program into machine code in one go. The analyzing phase takes much longer than with an interpreter. But the execution process is then much faster.
IoT
IoT stands for Internet of Things.
The idea of IoT is that everything can be part of the internet.
The word “Thing” in the Internet of Things can be pretty much anything, such as:
- Bread toaster
- A person with a brain chip
- Doorbell
- A car with built-in sensors
In the IoT, there is a lack of human interaction. Instead of human-to-human or human-to-computer actions, the IoT objects are independent nodes on the internet network.
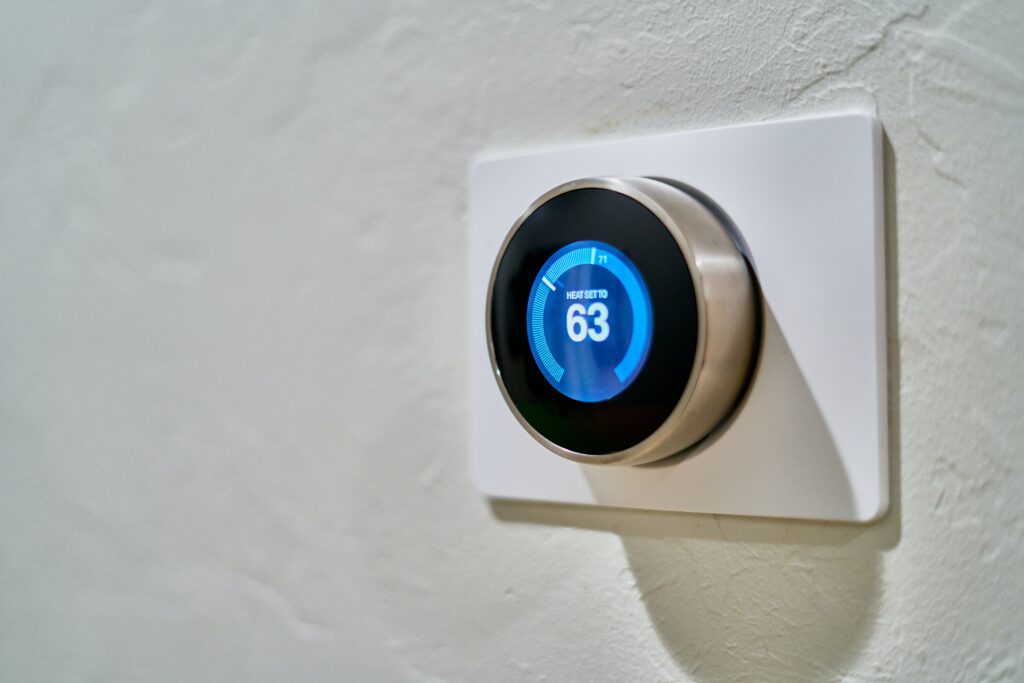
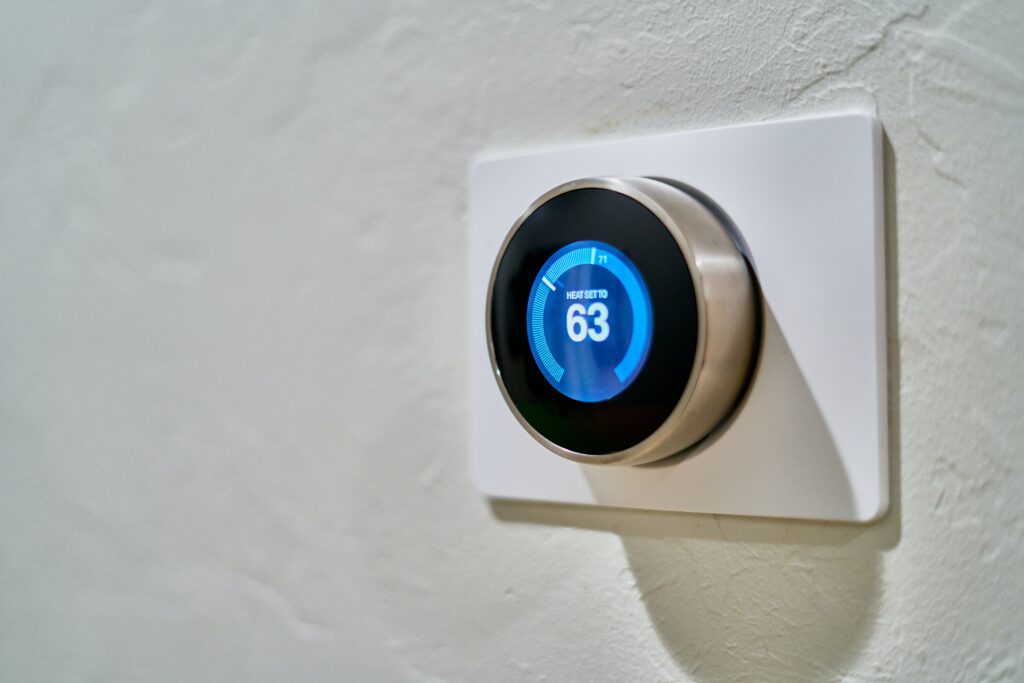
IP Address
IP Address is a series of unique numbers that identify a node on a network.
The idea is the same as phone numbers. For person A to talk with person B over the phone, they must know each other’s phone numbers.
The same applies to computers and smart devices. For a computer to communicate with another, there has to be a unique identifier. With computers, it’s the IP address that looks something like this: 192.168.1.163.
Iteration
In programming, iteration means the repetition of the same action. Iteration takes place as long as a condition is true.
For example, if a program wants to go through a list of names, it has to iterate the list until there are no names left.
Iteration is usually called looping. These terms are used interchangeably.
Java
Java is one of the most popular programming languages to date.
It is a general-purpose programming language for application development. Java is an efficient and secure programming language.
The most typical use cases for Java are developing Java applications for:
- Desktop devices
- Game consoles
- Android
- Data centers
And more.
JavaScript
JavaScript is the most popular and in-demand language.
Unlike many people believe, JavaScript has absolutely nothing to do with Java.
JavaScript is a web development language that brings interactivity to web pages.
For example, you can use HTML to build a form for your web page. But it’s JavaScript that controls the form submissions and button clicks.
JavaScript is a full-on programming language that has a lot of demand in the job market.
JSON
JSON stands for JavaScript Object Notation. It’s a commonly used data type to transfer data across the internet.
Basically, JSON is structured text data. Thus, it’s lightweight and easy to send, retrieve, and operate.
Here is an example of JSON data that represents a person:
{ "name": "Nick", "age": 10, "married": false, "hobbies": ["Jogging", "Golf", "Gym"] }
JSON is the de-facto data format in data transmission. In the past, other formats, such as XML were used actively.
Read my complete guide to JSON.
Junior Developer
A Junior developer is a programmer (software developer) who has at most a couple of years of experience in a software development company.
When you get a job as a software developer for the first time, you will be a junior developer. Even if you have 10+ years of coding experience, if none of that comes from a company you’ve worked for, you will start as a junior.
The distinction between a junior and senior developer is not set in stone. Some companies call developers with less than five years of working experience junior developers. In other companies, a developer with three years of experience is already considered a senior.
Library
A library is a collection of useful pre-written code solutions. By using a library, a developer can save a ton of time. Instead of writing a feature or functionality from scratch, the developer can reuse the code from the library.
Developing software at scale requires you to use libraries. Most code projects include dozens of external libraries to work.
Building software is all about combining existing solutions to form a new one. This is what libraries are made for.
Make sure to read more about libraries in programming.
Linter
A linter is a code-quality enforcement tool.
Each developer has a unique style of writing code. Unfortunately, this is usually bad because the code projects end up looking incoherent.
This is where best practices and team-specific rules are used.
But who has time to enforce that?
This is where linters help. Instead of manually going through every single line of written code, the linter does the job for you.
A linter can warn coders of making style mistakes or breaking the best practices.
With linters, teams are able to develop software whose code looks uniform and is easier to maintain.
Loop
In programming, a loop is a commonly used structure to repeat actions in code.
Typically, programmers have lots of data to deal with. This can mean millions of entries of users for example.
Going through this big of a list of users manually would be infeasible. Thus, programming languages support a code construct called a loop. A loop lets you write one expression that is applied to each item in the group.
Low-Level Language
A low-level language is a programming language that is a computer’s “native language”.
A low-level programming language is next to impossible for us humans to understand. Instead of writing the instructions in code, one must use machine-level instructions.
A low-level language directly uses the computer’s hardware components.
Examples of low-level language include:
- Assembly language
- Machine language
Practically all the programming languages you use are high-level languages. Out of the popular in-demand languages, C is the closest one to a low-level language. This is because C has some memory management features that let you work closely with the hardware side.
Machine Language
Machine language is the 0s and 1s that a computer understands.
More formally, machine language is the arrangement of bits that the computer reads.
Machine language is sometimes called machine code or object code.
A computer doesn’t understand programming languages. To run a program, the source code must be translated into machine code. This is what compilers and interpreters do. Whenever you run a program, your source code becomes machine language behind the scenes.
Markup Language
A markup language is used to both write and stylize text documents. The markup language consists of symbols you can insert into the text document. These symbols then control the text documents:
- Structure
- Formatting
- Relationship between the parts
Here is an example of markup language, HTML:
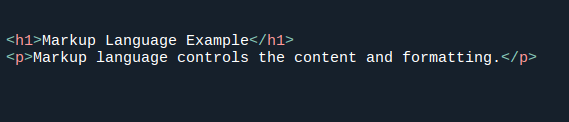
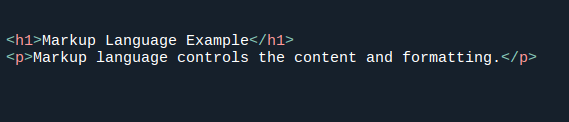
In this markup language sheet, I specify a heading by using a <h1> </h1> tags. Then I add a paragraph below it using the <p></p> tags.
When a browser renders the above HTML, the file looks like this:
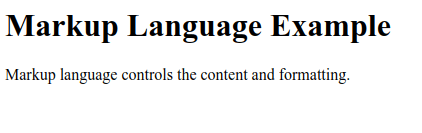
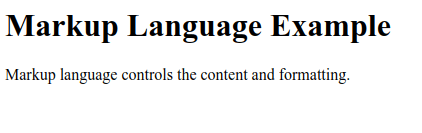
This is the idea of a markup language. It’s a text file that contains symbols that control what the document ends up looking like.
ML
ML stands for Machine Learning.
Machine Learning is a field in computer science where programs use data to make predictions. A machine learning program is trained with training data. Based on this training data, the program “learns” the characteristics of the data. When it gets an input, it can make a prediction of the output based on the training data.
For example, a machine learning model could predict the highest temperature of the day given the temperature in the morning. To do this, you have to code a linear regression model, which is nothing but linear algebra taught in high schools and universities.
So even though the word Machine Learning sounds fancy, it’s nothing but linear algebra and matrix calculations.
Null
Null is the intentional absence of a value.
If you have no technical background, here is a real-life analogy to understand null.
Imagine you have a variable X that represents the current time.
If X = 10:00 PM, the time is 10:00 PM.
But if X = Null, it means you don’t know the current time.
Object-Oriented Programming (OOP)
Object Oriented Programming is a commonly used programming style.
The idea behind OOP is to organize your software around objects instead of functions. The key programming concepts in OOP are classes and objects. In an OOP program, the problem is split into reusable parts of code (classes). These classes are used to create individual objects.
For example, in an OOP program, there could be a class called Employee. This class represents employees and specifies the following properties:
- Name
- Age
- Role
Then, to introduce new employee objects to the program, you can use this class as a blueprint for creating them.
OOP is the standard approach to teaching programming. Thus, most if not all developers use OOP when writing code at least at some point in their careers.
Objects
In programming, an object is an entity that has a state and behaviors.
For example, you could create employee objects in your code. Each employee stores the following information and behavior:
- Name
- Age
- Role
- An ability to introduce themselves
As another example, in your favorite game, the enemies are objects in code.
An object is one of the key concepts in object-oriented programming (OOP). In OOP, you can create a class to act as a factory for creating objects.
Operand
An operand is a value being manipulated by an operator.
For example, take a look at this simple math equation:
1 + 2
In this equation, the numbers 1 and 2 are operads. The + sign is an operator that works on the two operands.
Operator
In programming, an operator is a symbol that represents an action. An operator can be called on an operand or operands to manipulate their value.
One of the most common operators is the addition operator. This operator is capable of adding two numeric operands together.
1 + 2
In the above equation, the + symbol is the addition operator (and the numbers 1 and 2 are operands).
OS
OS is a shorthand for Operating systems.
An operating system is a software that connects the user with hardware.
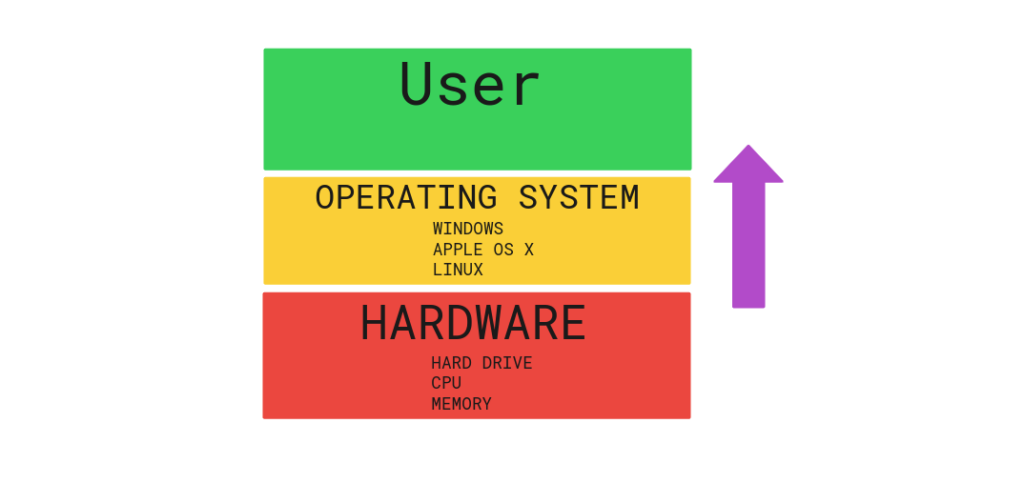
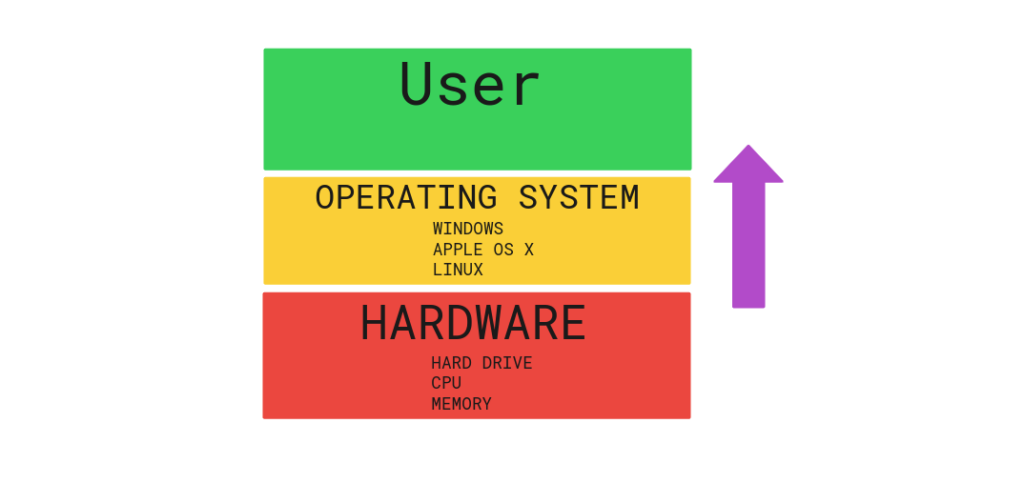
Without an operating system, such as Windows, a user would need to control the hardware manually.
Output
Output is the “result” of an action in a computer program.
Typically, a computer program works based on input and output. A function takes a user input, processes it, and spits out an output.
The output could be something like a result of a calculation.
Also, an output of one function might be an input for another function.
Package
In programming, a package organizes classes and interfaces together to form logical packages of code.
The idea of a package is the same as having a shelf in your room. On the shelf, there is one box for T-shirts, one for socks, and one for underwear.
In software development, packages are also known as modules. You can easily import or include packages to your program to use some additional features or functionality.
Parameter
A parameter is a value passed into a function. Parameters are also called arguments.
In a typical program, there are functions that take input and return output. For example, a function might take the date of birth as an input and return the age as an output.
The function input can be a single value or multiple values. Regardless, the input elements are called arguments or parameters.
Pattern Matching
Pattern matching is a technique to find specific sequences of data in a larger group of data.
The simplest type of pattern matching is something you probably use every day. It’s the CTRL + F search on a web page. When you are looking for a word in a text document, you can use CTRL + F to find a match. This is an example of pattern matching.
In programming, pattern matching is usually more complex. Instead of finding a specific word, a developer might want to find all the email addresses in a text document. Each email address has three parts:
- A prefix (usually the person’s name)
- The @ symbol
- The domain name
This is an email address pattern. To match all the email addresses, a programmer must specify the address pattern in the code. The most popular way to do this is by using Regex, which is a pattern-matching feature most programming languages support.
PHP
PHP is a general-purpose scripting language typically used in web development.
The initial abbreviation for PHP was Personal Homepage. But these days it’s a bit funkier recursive acronym, PHP: Hypertext Preprocessor.
PHP is used as a server-side language. It can be used to develop both static and dynamic websites and web apps.
Pixel
Pixel is shorthand for picture elements.
In a computer, the image on your view is not continuous. If you take a close enough look at the screen, you see tiny dots that are lit up. These are called pixels. On a typical modern-day device, there are millions of pixels on the screen.
When you look at the group of pixels far enough, you cannot tell there are such things in the view. The pixels give you an illusion of a high-definition and continuous image.
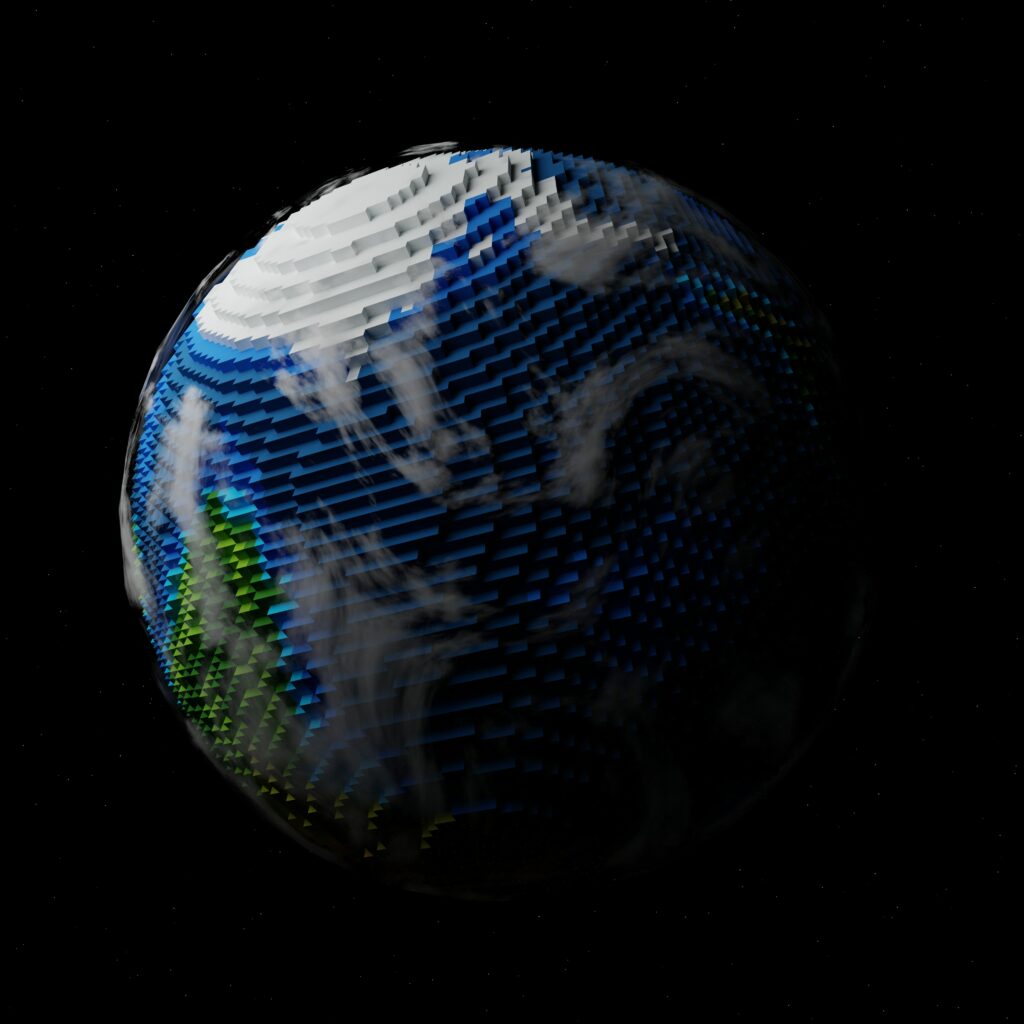
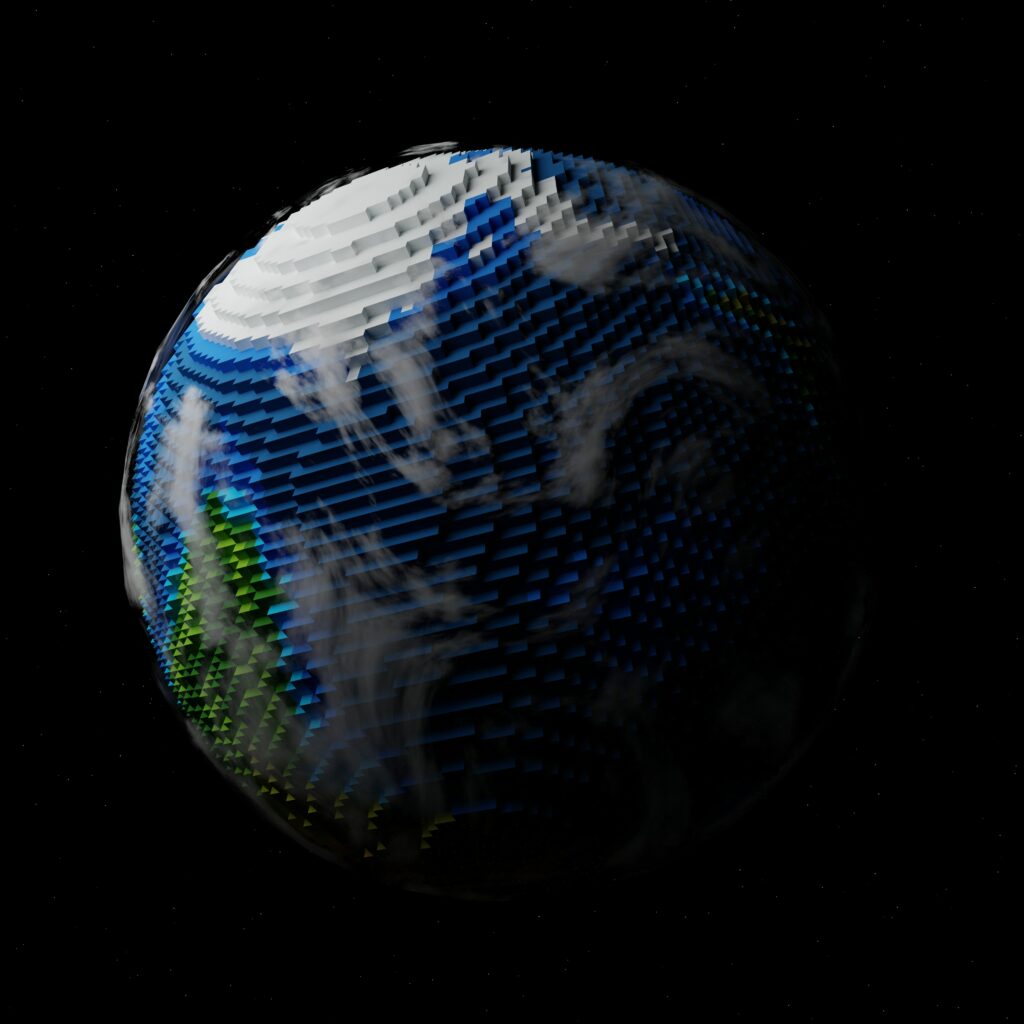
Program
In programming, a program refers to a piece of code that uses code to perform an action.
This can be something very simple, such as a program that calculates the BMI.
On the other hand, a program can be something massive, such as an online game that requires hundreds of developers to build it.
Pull Request
A pull request (PR) is a code-reviewing practice in development teams.
Software development teams use version control, such as GitHub to manage the code project. One of the key features of GitHub is a pull request.
Here is how it works.
When a developer makes a change to the codebase, they aren’t directly modifying the live version of the program. Instead, they work in a safe environment called a branch. The branch is a copy of the main program that doesn’t affect its performance.
Once the new feature or fix is tested and ready, it’s time to merge the branch with the main program. But this is something a developer shouldn’t do on their own. Instead, they should create a pull request to request making the change to the main codebase.
One of the team members should review the PR. In other words, they take a look at the code you wrote. If they decide to accept the changes, you are free to merge your branch with the main version of the program.
Python
Python is one of the most popular programming languages.
Python is commonly recommended as the first programming language for beginners.
The massive hype and popularity of Python don’t seem to go away. This is because Python is a flexible, beginner-friendly, and multi-purpose programming language.
You can use Python in almost any area of software development, including:
- Web development
- Data Science
- Machine learning
- Scripting
- Game development
- IoT
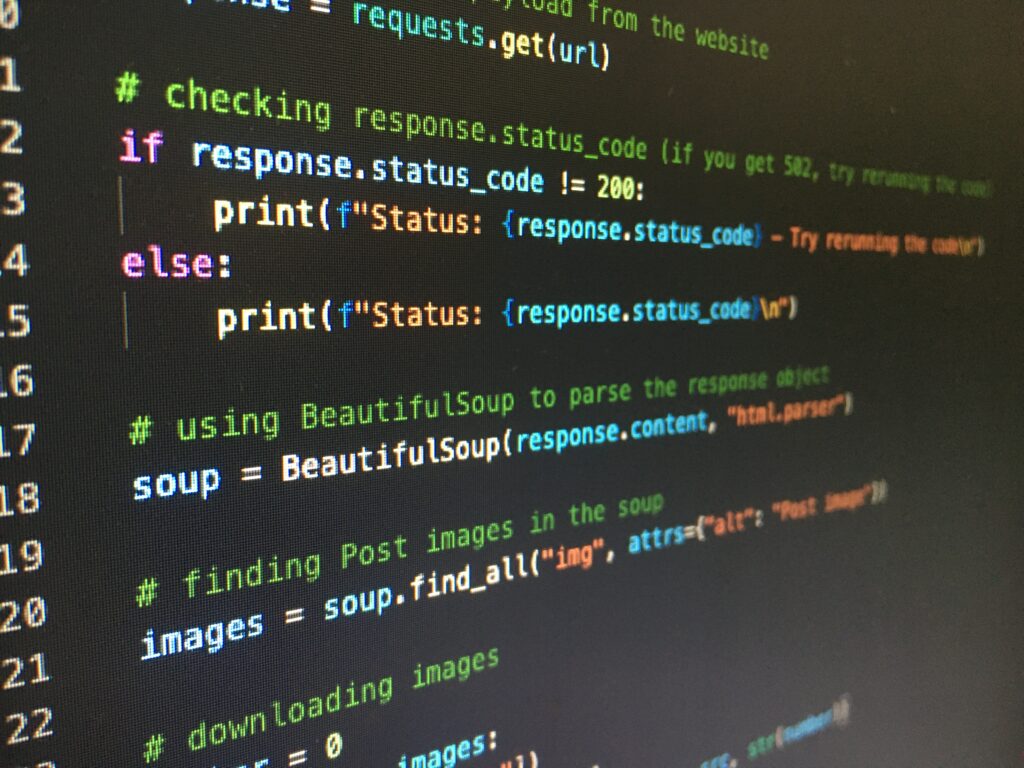
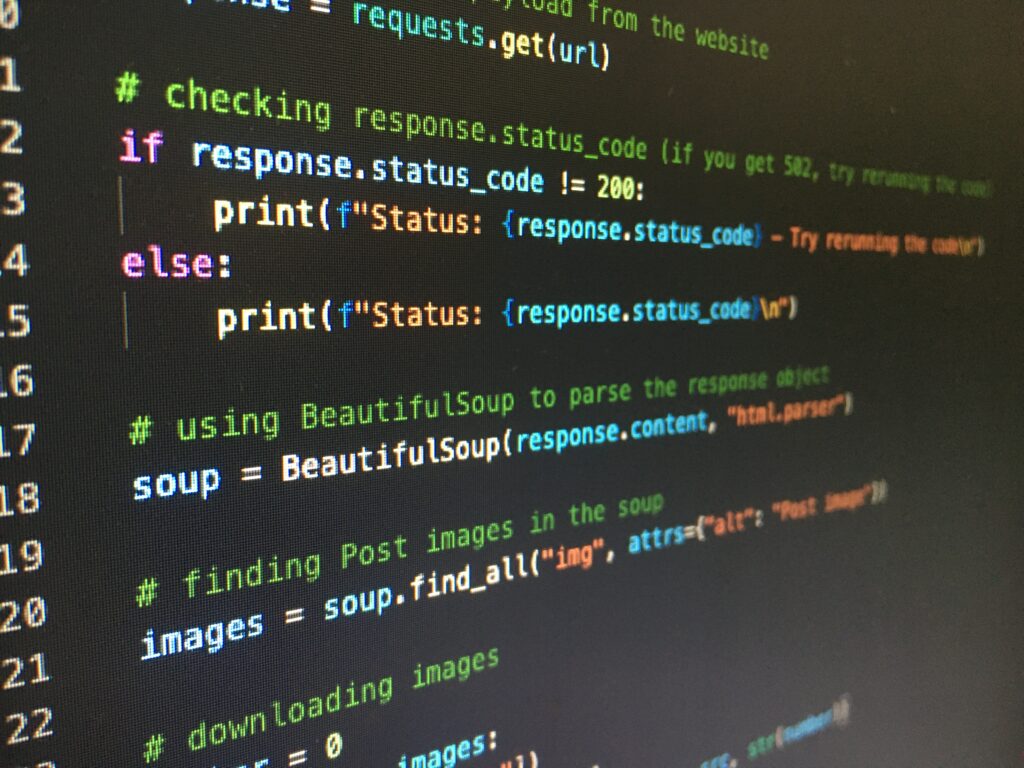
Read also reasons why Python is still so popular.
Regex
Regex is a common pattern-matching technique in programming.
Regex is like CTRL + F on steroids. Instead of matching with specific words, it can find complex patterns in text documents.
For example, given a text document, one can use Regex to find all phone numbers or email addresses on it.
Learn more about Regex by reading my complete guide to Regex.
REST
REST stands for Representational State Transfer. REST is a standardization for the communication between the systems on the internet. In other words, it’s an architectural choice for building APIs.
The idea of REST is to separate the client and the server. A client shouldn’t have to worry about what the server looks like and vice versa. The client-side and server-side can be implemented without knowing anything about one another.
Run
Running a program means launching or starting a program. It’s also called executing the program.
Running a program also means that the program is “on” right now. For example, your device is running a browser to access this website.
“Let’s fix that line and then run the program.”
Runtime
Runtime has nothing to do with the time it takes to run a program. It’s actually a shorthand for a runtime system or runtime library.
The runtime refers to the libraries, frameworks, or platforms that your program code runs on.
For example, C++ runtime is a collection of functions. The .NET runtime consists of an interpreter, a garbage collector, and more.
Scratch
Scratch is a free visual programming language. With Scratch, you can create interactive programs, such as games.
Scratch is geared toward children that have no programming knowledge.
Scratch is a great way to get an introduction to programming concepts. However, by learning scratch, you don’t learn how to code. Instead, you will get an introduction to the logic and mindset of a programmer.
Script
In programming, a script is a set of instructions interpreted by another program.
The difference between a script and a program is:
- The script is interpreted behind the scenes.
- The program is executed behind the scenes.
Another typical distinction between a script and a program is:
- A script is a short and concise single-use tool. Usually, a script is external to some system.
- A program is a large entity written in a compiled language, such as C++. The program code is what creates the entire system.
Programming languages that are mainly used for scripting are called scripting languages. These include Perl, Python, and JavaScript.
SDK
SDK stands for Software Development Kit.
As the name suggests, an SDK is a toolkit for making development possible. An SDK can include things like:
- Libraries
- Code samples
- Guides
- Processes
Developers can integrate the SDKs into their own projects. For example, to build an iOS app, you need to use an iOS App SDK.
A popular example of an SDK is Unity. Unity is a game engine that makes it possible to develop games at scale. In this SDK, there is a game engine that implements complex physics and real-life properties such that a game developer doesn’t have to care about those.
Search Engine
A search engine is a product that your browser uses to find web pages on the internet.
Examples of popular search engines are:
- Google Search
- Yahoo!
- Bing
- Baidu
- Yandex
- Ecosia
- DuckDuckGo
Senior Developer
A senior developer is an experienced software developer. Typically a senior developer has at least 5 years of full-time experience in a software development company.
But the definition can vary based on the company in question. Rather than focusing on the time spent coding, it’s the working experience that matters when making the distinction between junior and senior. A senior developer completes tasks independently and handles broader concepts while helping junior developers.
There is no standard definition for a senior developer. Sometimes a senior developer becomes a junior developer in the next company (intentionally).
Server
A server is a computer that stores, processes, and manages:
- Data
- Devices
- Systems
The idea of a server is to serve resources for the devices in the network. For example, a web server stores files that make up a website.
Servers typically offer:
- Great processing power
- Scalability
- Reliability
- Collaboration
- Savings in costs
Server-Side
Server-side refers to the side of a web app that the end user doesn’t see. It is all the actions and processes that take place behind the scenes on the server.
Sometimes, the server side is also called the backend. But these are not exactly the same.
Snake Case
The snake case is a popular typographical convention in programming.
Unlike the camel case, in the snake case, you separate multi-word combinations with underscores.
For example my_fancy_house.
This convention is used because programming languages don’t allow for spaces in code. The snake case mimics spaces by using underscores.
Sprite
Sprite is a game character or object in a 2D game.
In technical terms, a sprite is a 2D bitmap integrated into a broader scene, such as a video game.
Commonly, sprites come in sprite sheets. A sprite sheet is a large image of multiple sprites. Game developers can use sprite sheets to create a movement for the characters. This happens by showing the sprite sheet images one after another in a short time interval.
Statement
A statement is a single line of code that specifies an action to take.
For instance, assigning a value to a variable is considered a statement.
a = 10
Swift
Swift is the programming language of iOS apps. It is a relatively new language founded by Apple in 2014.
To build a mobile app for iOS devices, you need to learn Swift.
Thus far, Swift hasn’t been used for much more than iOS apps, which it was designed to.
But thanks to its versatility and beginner-friendliness, Swift is becoming a prominent data science and machine learning language.
Syntax
Similar to how spoken language has writing rules, a programming language has syntax.
Each programming language has a distinguishable syntax that specifies rules for writing “correct” code.
For example, Python syntax is characterized by the colons and indentations:
def sum(a, b): return a + b
Terminal
The terminal is the command-line interface for Apple devices.
You can execute commands in the Terminal to perform actions on your operating system. Here are some examples of what you can do on a Mac with the Terminal.
- Create, update, or delete files
- Download data from the internet
- Shut your computer down
- User version control to develop software
And much more.
Token
A token is the smallest programming language element. It refers to the characters that make up the source code. It can also refer to reserved keywords in a programming language.
There are five types of tokens in a programming language:
- Constants
- Identifiers
- Operators
- Separators
- Reserved words
UI
UI stands for User Interface.
Every single piece of software in the market has a UI.
The UI consists are made up of all the components in the view, such as:
- Text
- Backgrounds
- Buttons
- Animations
And much more.
UI design is a valuable skill in software development. To build software at scale and create a reputable brand, UI has to be taken seriously!
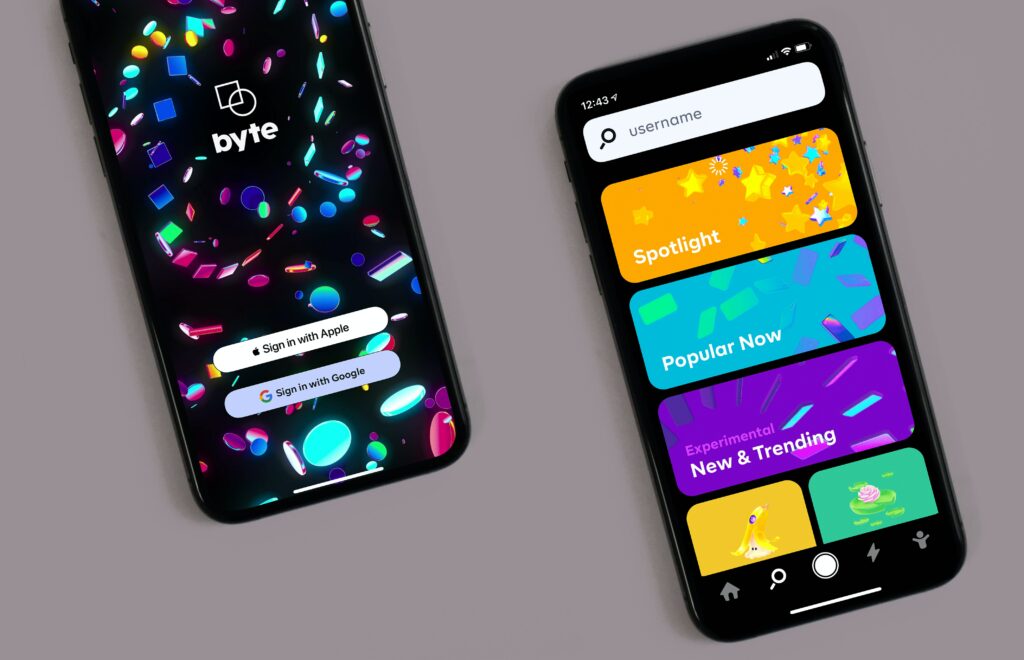
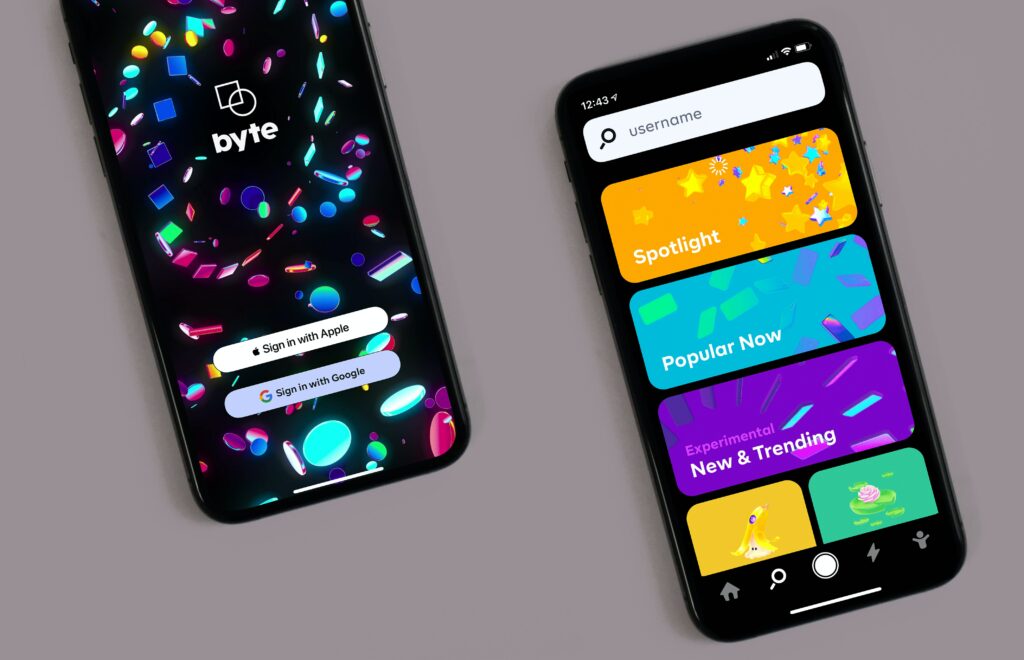
URL
URL or Uniform Resource Locator is an address to a resource on a remote server.
When you visit a website, your browser is requesting files from a remote server. To locate the files, the browser uses a URL.
Read also: What Is an URL?
UX
UX is a shorthand for User Experience. It describes how the user feels when using your app or program.
When developing programs or apps, it’s important to make the user experience great. Otherwise, you give an unprofessional image of your business. Worse yet, bad UX means unsatisfied customers that are less likely to use your tools or recommend them to anyone else.
Good UX is when an app is simple and intuitive, and each function contributes to solving the users’ problems. The program should be seamless and require little to no guidance. Users shouldn’t feel like they are lost.
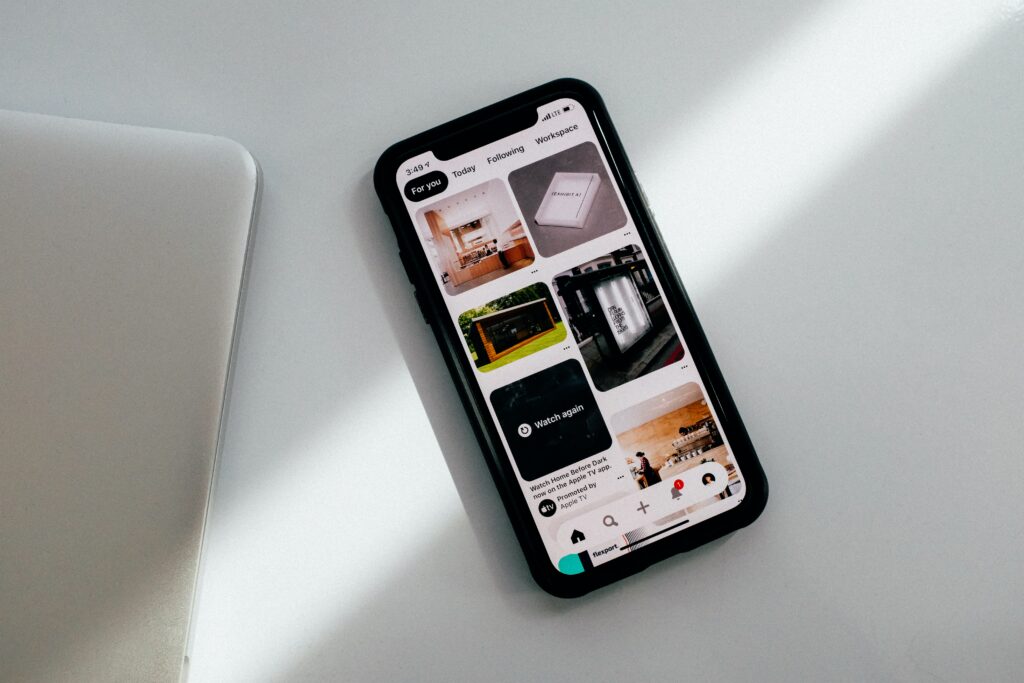
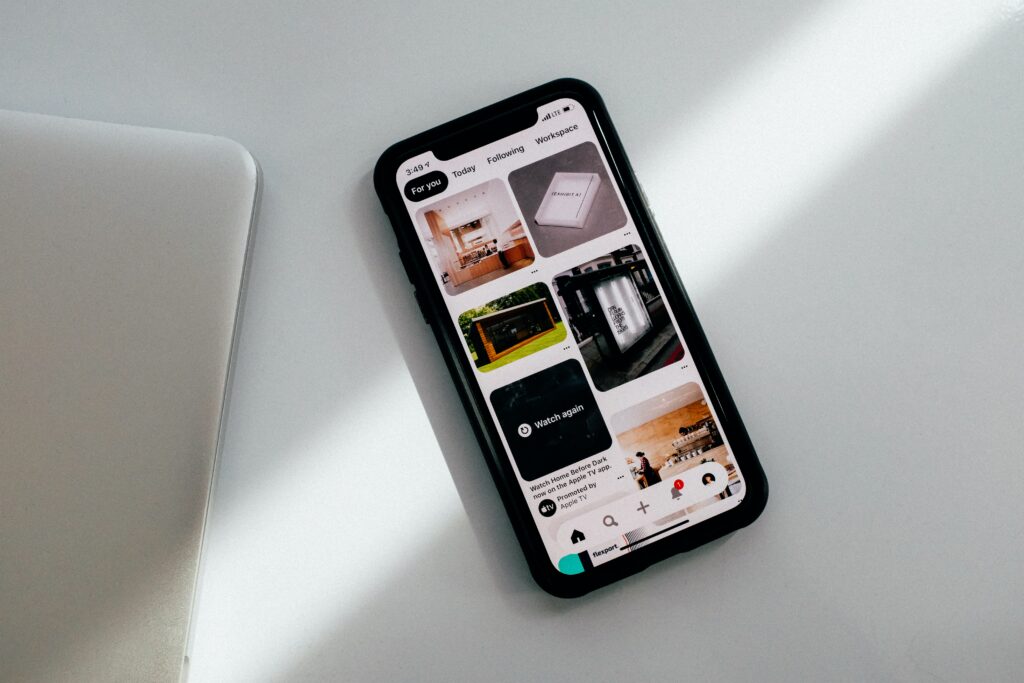
Variable
A variable is a value that can change. It is one of the cornerstones of programming. Every single program you’ll ever code uses variables.
A variable is easy to create, read, and modify. Besides, variables give a way to label data in a clear and concise manner.
Version Control
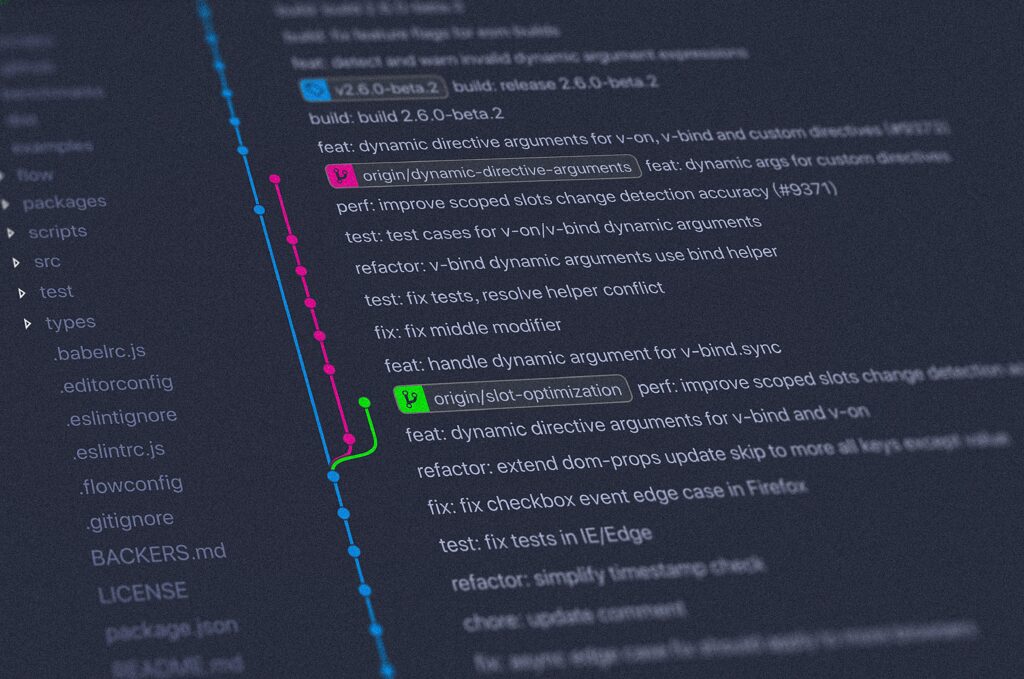
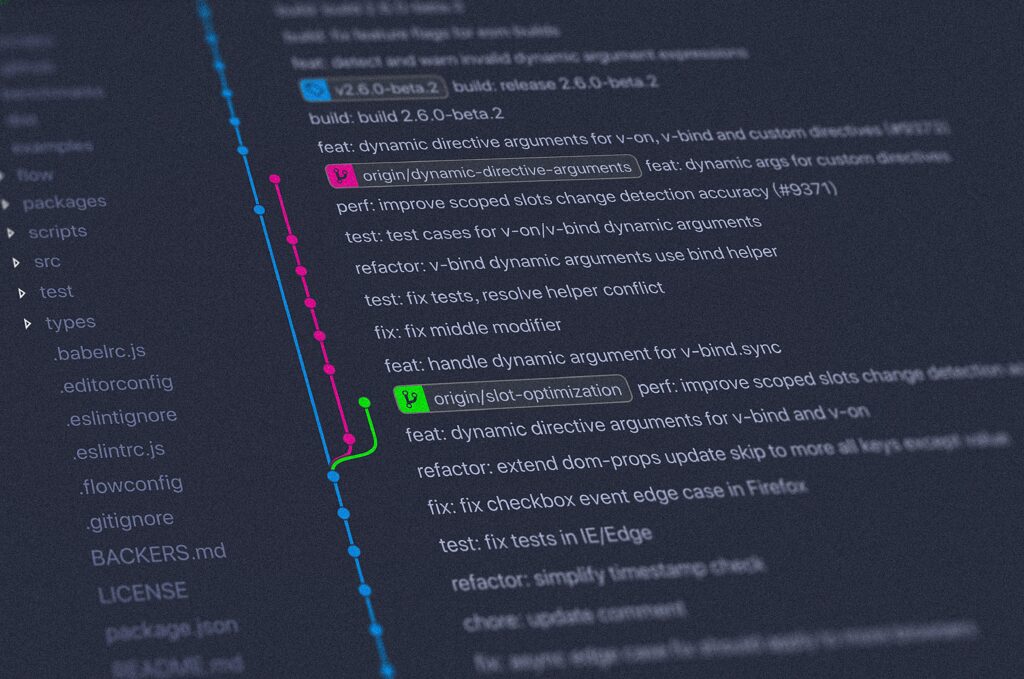
VPN
VPN or Virtual Private Network is software that promotes online security.
With a VPN, you can surf anonymously.
A VPN works by masking your IP address with another untraceable IP address. This way your internet service provider cannot track your online activity.
A common use case for a VPN is to access geo-restricted content, such as NetFlix or Hulu.
A VPN uses end-to-end encryption to protect your data. If a malicious middleman was able to intercept the connection, they wouldn’t be able to do much with the data thanks to the encryption mechanisms.
Wrap Up
That’s a whole lot of programming terminology!
I hope you find something new and intriguing on the list.
Remember, if you are new to programming, don’t try to memorize these terms. Instead, you will learn them as you learn to code. But if you want to keep up with the conversations with more experienced devs, then knowing the words in this list makes sense 🙂
Thanks for reading. Happy programming!