API or Application Programming Interface allows communication between applications. It is not a server. Instead, it’s an intermediary software that is used to access it.
When you look up the weather from your smartphone or desktop, the browser talks with an API under the hood. Here is an illustration:


How Do APIs Work
An API defines a set of rules on how applications can communicate with one another. It is the middleman between an application and a web server. It’s responsible for forwarding the requests from an application to a server and vice versa.
Here is how it works:
- A browser makes an API call to retrieve data from a web server. This can be weather data for example.
- The API in front of the server catches the request and calls to the server and asks for the data.
- The server sends a response to the API that contains the requested data.
- The API forwards the response data back to the browser that requested it.
A Real-Life Analogy
A waiter of a restaurant is a perfect real-life analogy for an API. They are the intermediaries between the tables and the kitchen. They communicate orders from a table to the kitchen and bring the food from the kitchen to the table.
When you open up a weather app, your device tells an API that it wants to know the weather. It then forwards this request to a server that prepares the data. When the data packet is ready, the API grabs it from the server and sends it your way.
What Problem Do APIs Solve
APIs allow applications to easily communicate with each other.
If you’re a developer, APIs save you a whole lot of time.
For instance, say you want to build a weather application but you don’t own weather stations or weather-predicting software. So you have to rely on third-party data.
To get the data, you could email a third-party weather service for a spreadsheet of weather predictions. But the forecasts get outdated quickly. So you’d need to exchange dozens of emails to stay up to date.
Wouldn’t it be better if there was a way for your app to automatically request the weather data every 15 minutes for example?
This is exactly what APIs let you do. In short, they enable applications or programs to communicate with one another.
Common Types of APIs
There are different types of APIs. Here is a list of the four most common APIs:
- Open APIs are publicly available to anyone. Usually, they require registration and the use of an API key.
- Partner APIs are exposed to business partners only.
- Internal (private) APIs are only exposed to the organization’s internal systems. They are used across different internal development teams for productivity and reuse of existing services.
- Composite APIs bundle multiple requests from different APIs together. Rather than performing separate queries, a client can make one extensive API request.
How Do Businesses Benefit from APIs
There are many good reasons why a business might want to make use of an API.
Data Monetization
If a company stores valuable data, such as weather data, it can decide to make it publicly available. This means the company sets up an API that any developer can access. Usually, this API is free to some extent but requires a paid premium plan for wider use.
This is a win-win situation for the community and the business. The developers are granted access to valuable data otherwise infeasible to obtain. And the business is able to monetize their data and gain exposure.
Automate Enterprise Workflows
These days, an average enterprise uses around 1200 cloud apps. Most of these apps are not talking to one another.
An API enables integration between the disconnected apps. This way companies can automate time-consuming workflows and improve their collaboration.
Innovation
Application programming interfaces also offer a way to connect with new business partners. They make it possible to access new markets and create new opportunities.
A good example use case of an API is Stripe. Stripe offers a payment processing system to your website. This system supports a list of universally accepted payment types.
The way it works is when a customer makes a purchase on your site, an API call is sent to Stripe who then takes care of the rest.
Additional Layer of Security
API also provides a secure way to communicate between servers and devices.
By using an API, your device’s data is not exposed to the server and vice versa. The communication happens in small data packets, sharing only what’s necessary.
For example, a payment processing API is secure as it does not have to access your bank account.
Common APIs You Have Seen
You have certainly used different kinds of APIs hundreds of times without realizing it.
Here are some common use cases.
Sign Up with Google
How many times have you seen a signup page similar to this?


This authentication feature allows a website to use the API of a popular service to authenticate a new user. This saves the time and hassle of setting up a new profile.
Under the hood, the website delegates the signup process to a third party through an API call.
Travel Booking Comparisons
Travel booking sites compare thousands of flights, displaying the cheapest options for a visitor.
This is made possible through APIs.
Under the hood, the travel booking program:
- Sends API calls to different travel sites to obtain pricing info for the flights.
- It then compares the results and shows the users the cheapest options.
Third-Party Payment Processing
For example, the “Pay with PayPal” option is a common feature on an e-commerce site. This also works through an API.
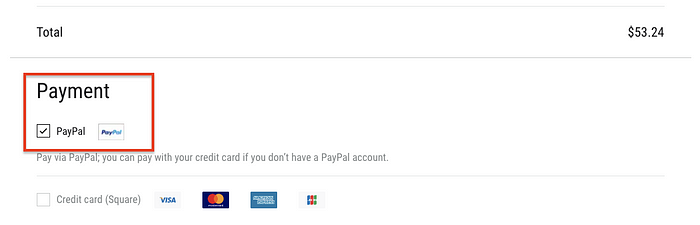
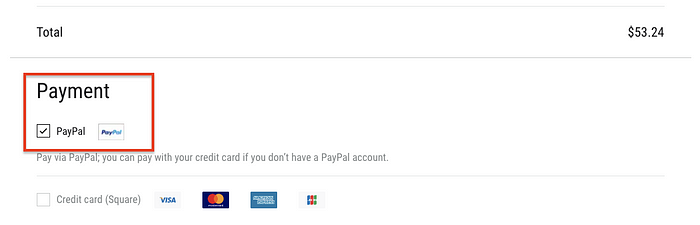
When a user purchases something via this option, an API call to PayPal is made. After this, PayPal takes care of the rest.
APIs on a Technical Standpoint
Now you understand the basics of an API on a high level.
Next, you are going to take a look at APIs from a technical standpoint. In this section, you learn how to use an API with a concrete example.
This part involves writing a little bit of HTML and JavaScript code. If you’re not into technical details, feel free to skip this part.
What Is an API Call
An API call is an HTTP request to an API endpoint (it is just a URL address).
In other words, you can perform an API request with your browser by typing in the API URL and hitting enter.
What Is an API Endpoint
An API endpoint is a base URL used to communicate with an API.
For example, a weather API can have endpoints for lightning data, air pressure, and temperature.
The API URL structure could look like this:
https://example.com/weather/lightning
An endpoint is a touchpoint between an API and a server. It’s the location where your program sends a request to retrieve specific data.
An API typically has multiple endpoints.
API Response—What Happens After an API Call
When you’ve made an API call, the API interprets it and forwards it to a web server. The web server then prepares a data packet according to your needs and sends it back to the API. The API then forwards it to you.
This response is not a pretty-looking web page. Instead, it’s an object, which is commonly formatted as JSON, XML, or CSV. Your app has to handle this data and convert it to a human-readable representation.
JSON in a Nutshell
JSON or JavaScript Object Notation is a text-based data format.
JSON is the most common data format used with APIs. It’s powerful because it is supported by most programming languages.
A JSON object looks like this:
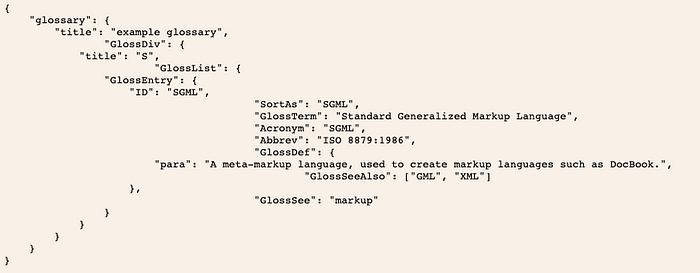
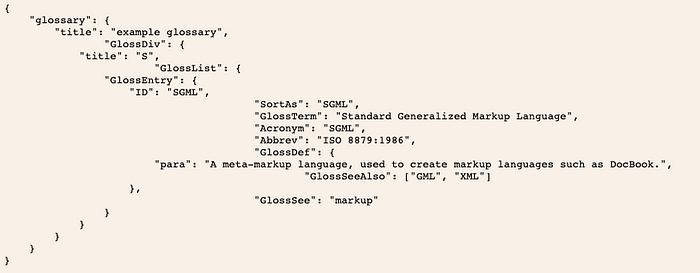
It contains the data as comma-separated key-value pairs. For example "title": "example glossary"
.
When you perform an API request, you commonly get a JSON response back. To make use of the data, you need to extract values from the JSON object in your program. This is called JSON parsing.
Read also my complete guide to JSON.
How to Use an API in a Nutshell
Now you’ve learned all the basics of APIs. It’s time to learn how to use one.
Each API is different. Each project is different. There’s not a single guide that would explain step-by-step how to use any API in any project. But the process can be summarized into these five steps:
- Find an API by Googling.
- Get an API key. Usually, you need to sign up to grab one. Keep this key a secret. Most APIs offer a free plan for basic use and premium plans for advanced users, such as for more API calls.
- Review the documentation provided by the API. These usually contain examples of how to get started with API calls and integrations.
- Make your first API request. Don’t forget to examine the response.
- Integrate the API with your project. This is a project-specific step. If you don’t know how there are always tutorials on the Internet.
Next, let’s apply these five steps to a concrete example of using an API.
How to Use an API—An Example Project
This example is meant to demonstrate the steps of using an API by showcasing a simple real project.
For example, imagine your customer asked you to create a web page that shows the current weather in London like this:



To pull this off you clearly need to rely on third-party weather data. In other words, you are going to use an API.
Let’s get started:
1. Find an API
Write “Free weather API” to Google and hit search.
The first search result is the OpenWeatherMap’s website. It says it has a free API that one can sign up and start using right away. Let’s choose this to our small project.
2. Get an API Key
To make an API call you need an API key that is a unique client identifier.
To find the API key, let’s pick the service. You are interested in the current weather, so choose the Current Weather Data option.


To grab a free API key, choose the Free plan by clicking Get API Key:


To access the API key, sign up to the page.


Once you’ve signed up and verified your account, you receive an email that contains your API key.
3. Review the API documentation and API Guides
Now you’re all set for starting to make API calls. But at this point, there’s no way for you to know how to create one.
This is where the API documentation helps. In this case, you want to learn how to perform an API request to get the weather data of a specific location.
OpenWeatherMap provides you with this piece of information. It shows you how to request the weather of a location by crafting a URL to the API endpoint:


With this information, you’re can format your API URLs and make your first API call.
4. Make Your First API Call
Let’s write an API call to query the weather in London, UK. To make the request, follow the instructions given by OpenWeatherMap:
- Copy-paste the following URL to the URL bar of your browser:
api.openweathermap.org/data/2.5/weather?q=London,uk&APPID=API_KEY
- Replace the
API_KEY
with your own API key. - Hit enter.
Now you’ve performed your first successful API request. As a result, the browser opens up a page that looks like this:


This is the API response. It’s in JSON format and contains the weather data of London. If you take a closer look, you see it has weather-specific information, such as temp
and pressure
.
The next step is to convert this cryptic data into a human-readable web page.
5. Integrate the API with your project
You can finally create an HTML page that shows the weather. The end result of this step is going to be what the customer wanted—A web page with London’s weather:



In the previous step, you manually made an API request with a browser. As a response, you got back a weather data as JSON.
On your web page, however, you want the API request to happen automatically when the page opens. Furthermore, you want the response to look human-readable by showing an icon of the weather instead of displaying the JSON garbage.
Let’s take a closer look at the JSON response from earlier.


You can see an icon
field that likely represents the current weather in London as an icon. This is can be useful.
But wait. It’s not an image. It says "icon":"04d"
.
What can you do with it then? Let’s go to the API documentation to figure it out.
The documentation says you can get weather icons from this URL http://openweathermap.org/img/wn/
by adding icon
+ .png
at the end of the URL.
Let’s pick the icon "04d"
from the JSON, and add it to the end of the URL to test if it leads to a weather icon:
http://openweathermap.org/img/wn/04d.png
If you search for this URL, you find out a page that shows this tiny icon:


It works. This is the weather icon you want to show for the customer on the web page.
But now you did this manually. The next thing you need to do is to automate this process. In other words, you need a web page, that:
- Performs the API call.
- Picks the icon type from the response object.
- Constructs a URL to the weather icon.
- Embed the icon behind the URL to your web page.
To do this:
- Create a file called
weather.html
. - Copy-paste the following basic HTML page template into it:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>Weather in London</title> </head> <body> <div class="weather-container"> <p>Weather in London</p> <img class="icon"> </div> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js"></script> </body> </html>
If you now open up the weather.html
file with your browser, you see a page that says Weather in London
. But there’s no weather information because the HTML code does not make API calls yet.
To perform the API request in the HTML document let’s use JavaScript’s fetch
library. Add the following piece of code below code line 15 and replace YOUR_API_KEY_HERE
with your personal API key:
<script> fetch('http://api.openweathermap.org/data/2.5/weather?q=London,uk&APPID=YOUR_API_KEY_HERE') .then(response => response.json()) .then(data => { var iconURL = "http://openweathermap.org/img/wn/" + data.weather[0].icon + ".png"; $(".icon").attr("src", iconURL); }); </script>
In short, this code makes the API call and picks the icon
from the response. It then constructs the icon URL where it gets the icon to display on the web page.
If you now open up weather.html
with your browser, you can finally see the weather in London:


This completes the example project.
Conclusion
An API or Application Programming Interface is an intermediary software that defines rules on how two applications communicate.


APIs can combine disconnected enterprise apps to optimize workflow. They act as a layer of security when data has to be transferred between two applications. APIs also make data monetization possible and can open up new business opportunities and increase exposure.
Thanks for reading. I hope you enjoy it!