Dealing with number sequences in data science is common. Having the tools to easily produce sequences of numbers of different lengths, stepsizes, and start/end values is crucial. This way you can avoid having to write loops to produce number sequences over and over again. In R, you can use the built-in seq() function to create number sequences easily.
x <- seq(10) # 1,2,3,4,5,6,7,8,9,10
This is a comprehensive guide to the seq() function in R.
You will learn the syntax among the different optional parameters. All the theory is backed up by great and illustrative examples that cover each of the seq() function parameters.
What Is the seq() Function in R?
In R, you can generate a sequence of numbers with the built-in seq() function. The seq() function allows you to control the start and end values of the sequence as well as the desired length and step size.
Before taking a look at examples, let’s see what the complete syntax of the seq() function looks like.
Syntax
Typically, you see the seq() function being used with a single argument like this:
x <- seq(10)
This produces a sequence of numbers from 1 to 10.
But the full syntax of the seq() function in R is:
seq(from=1, to=1, by=1, length.out=NULL, along.with=NULL)
Where:
- from is the starting value of the sequence.
- to is the end value of the sequence.
- by is the “jump length” by which the values are incremented every step.
- length.out is the desired sequence length.
- along.with is the desired sequence length that matches the length of another data object.
In the examples section, you will learn how to use each of these parameters in the seq() function call.
Examples of the seq() Function in R
Let’s take a look at 5 different examples of the seq() function in R that covers all the different parameters.
Example 1: The ‘from’ Parameter
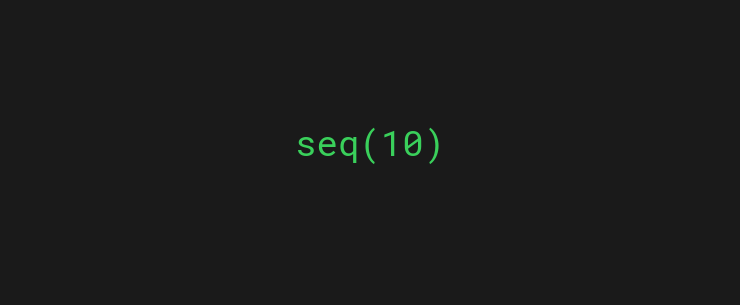
The most basic way to call the seq() function in R is by giving it a single parameter, that is, the end value of the sequence. When you do this, the default start value of the sequence is 1 as well as the default step size.
For example, let’s create a sequence of numbers from 1 to 10:
x <- seq(10) x
Output:
[1] 1 2 3 4 5 6 7 8 9 10
Example 2: The ‘from’ and ‘to’ Parameters
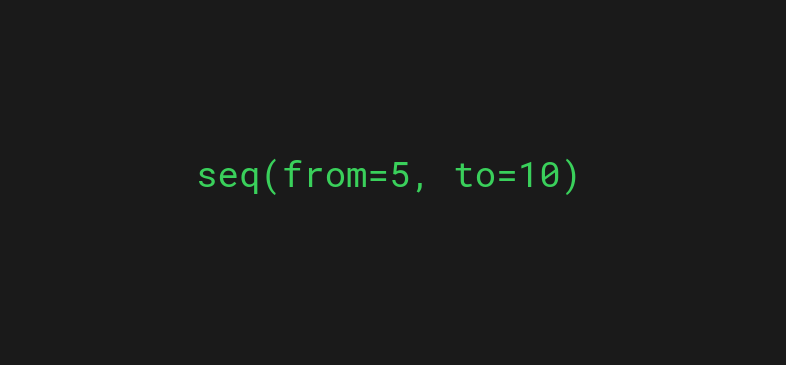
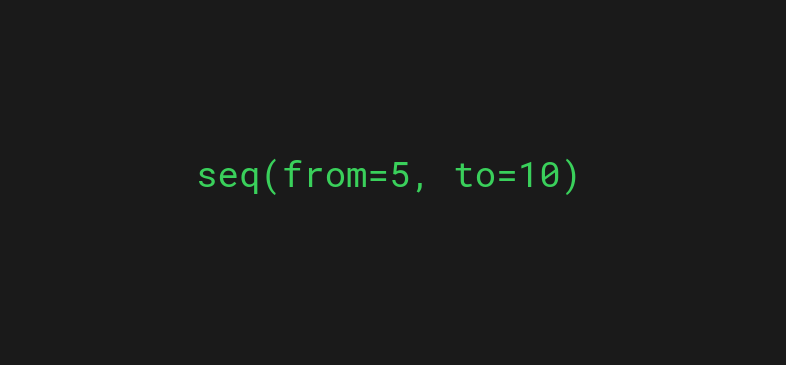
Not always do you want the sequence to start from 1. In this case, you can specify both the start and the end values for the sequence by specifying the from and to parameters in the seq() function call.
For example, let’s produce a sequence of numbers from 5 to 10:
x <- seq(from=5, to=10) x
Output:
[1] 5 6 7 8 9 10
Example 3: The ‘by’ Parameter
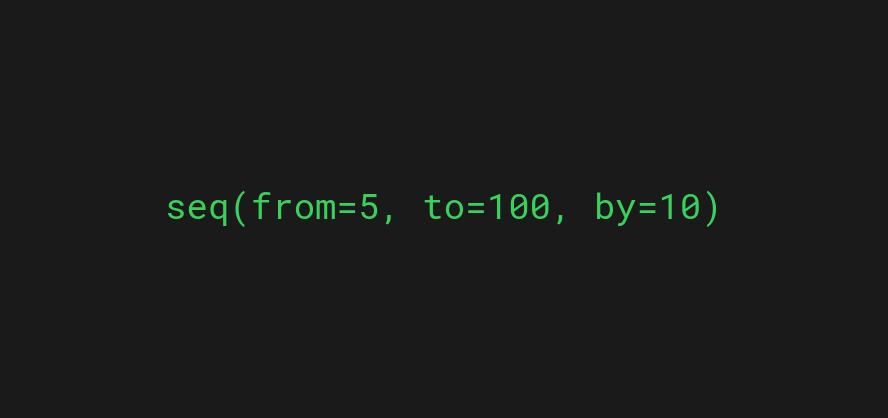
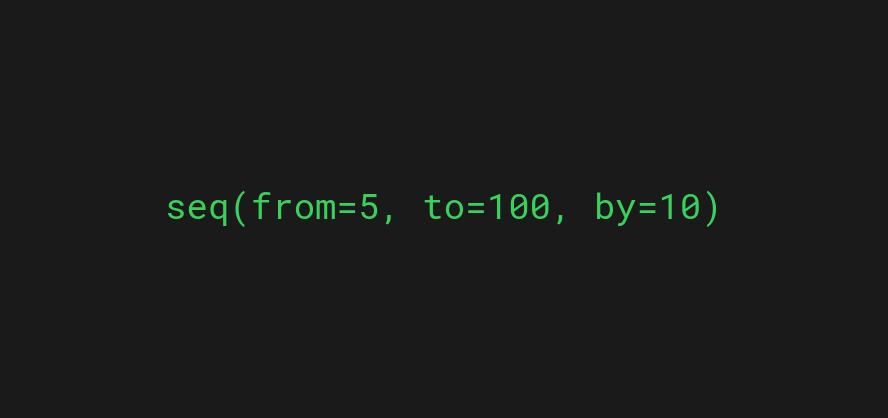
Thus far you’ve seen examples where the stepsize is 1, that is, consecutive numbers are incremented by 1 in the sequence. But if you want to produce sequences with stepsizes other than 1, you need to specify the optional by argument in the seq() call.
For example, let’s create a sequence of numbers from 5 to 100 with a stepsize of 10:
x <- seq(from=5, to=100, by=10) x
Output:
[1] 5 15 25 35 45 55 65 75 85 95
Example 4: The ‘length.out’ Parameter
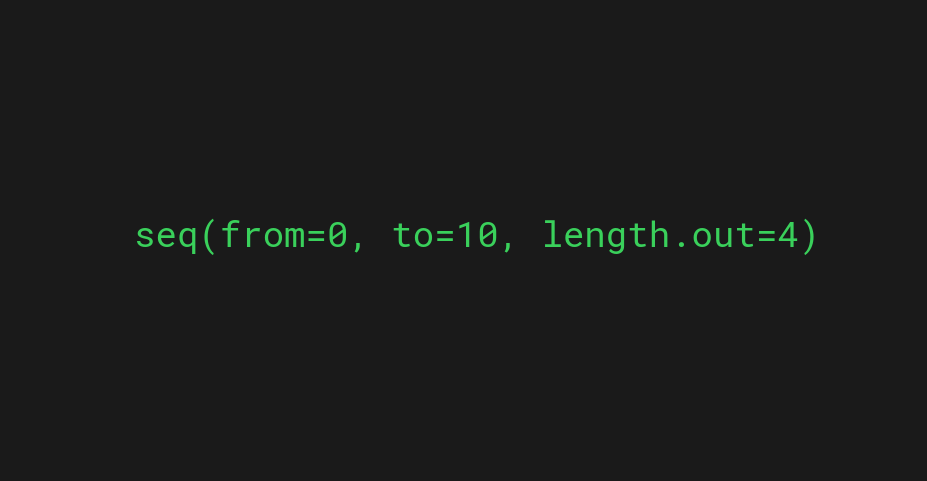
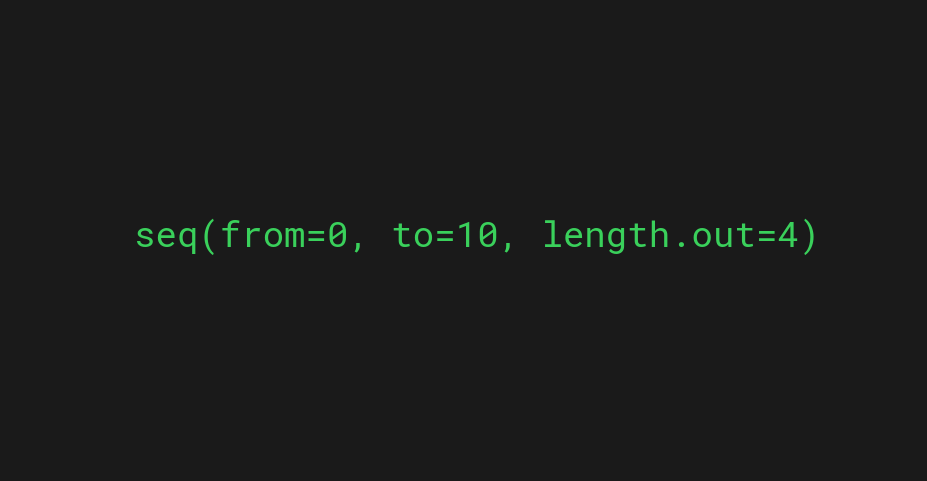
Sometimes it’s useful to specify a sequence of numbers in a range with a fixed length. This way you can uniformly distribute values of a certain length to a sequence of predetermined length.
For example, let’s generate a sequence of values between 0 and 10 and set the desired length of the sequence at 4:
x <- seq(from=0, to=10, length.out=4) x
Output:
[1] 0.000000 3.333333 6.666667 10.000000
The seq() function calculates the numbers such that they are evenly distributed across the range.
Example 5: The ‘along.with’ Parameter
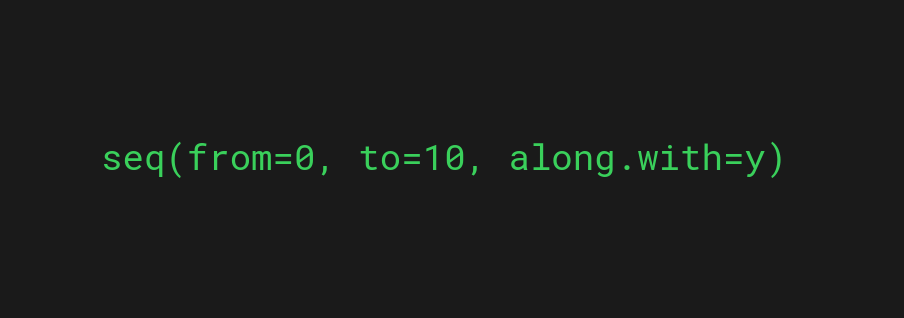
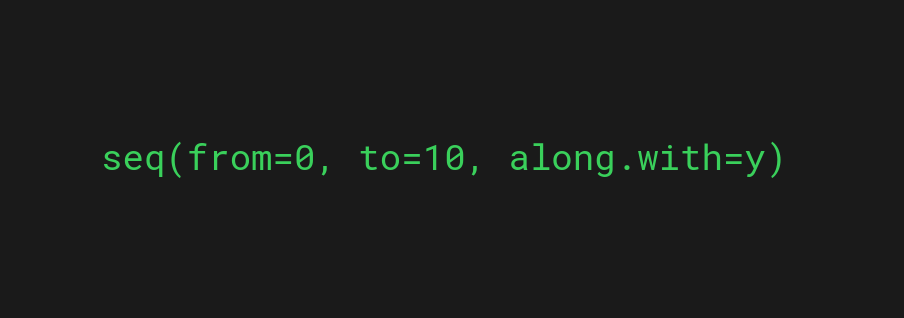
When dealing with multiple sequences of numbers, you sometimes might want to generate a new sequence such that it matches the length of another one.
Of course, you could set the optional out.length variable to the length of the desired sequence. But if the length needs to match with another sequence, it’s safer to specify the optional along.with parameter. This way the code is more consistent and the intent is clearer.
For example:
y <- c(0.1, 1.5, 2.9, 4.6) x <- seq(from=0, to=10, along.with=y) x
Output:
[1] 0.000000 3.333333 6.666667 10.000000
Summary
Today you learned how to use the built-in seq() function in R.
To recap, the seq() function produces a sequence of numbers from a start value to the end. You can use the seq() function to produce sequences with the desired length, stepsize, and start/end values.
Thanks for reading. Happy coding!