In Python, you can start indexing from the end of an iterable. This is known as negative indexing.
last = list_items[-1]
For example, let’s get the last value of a list:
numbers = [1, 2, 3, 4, 5] last = numbers[-1] print(last)
Output:
5
Here is an illustration of how the list indexing works in Python:
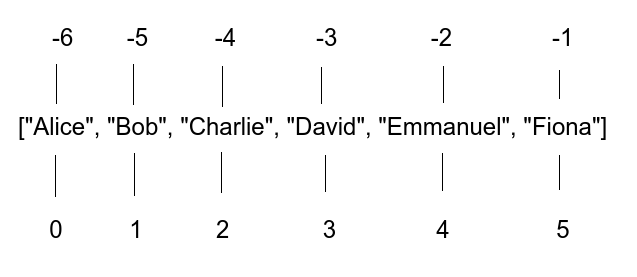
This means you can use both positive and negative indexes to access iterables.
This is a comprehensive guide about negative indexing and its applications in Python.
Indexing in Python
Let’s start by having a look at how traditional indexing works in Python.
To access elements of a Python iterable, such as a list, you need to know the index of the element.
Python supports indexing in two ways:
- Positive zero-based indexing.
- Negative indexing that “starts from the end”.
Let’s take a closer look at both of these.
Zero-Based Indexing in Python
The basic way to access iterable elements in Python is by using positive zero-based indexing.
This means each element in the iterable can be referred to with an index starting from 0.
In zero-based indexing, the 1st element has a 0 index, the 2nd element has 1, and so on. Here is an illustration:
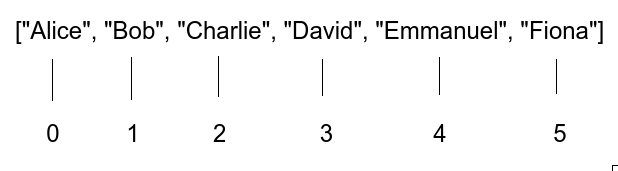
For example, let’s get the first name in a list of names:
names = ["Alice", "Bob", "Charlie", "David", "Emmanuel", "Fiona"] first = names[0] print(first)
Output:
Python
Negative “From the End” Indexing in Python
Python supports “indexing from the end”, that is, negative indexing.
This means the last value of a sequence has an index of -1, the second last -2, and so on.
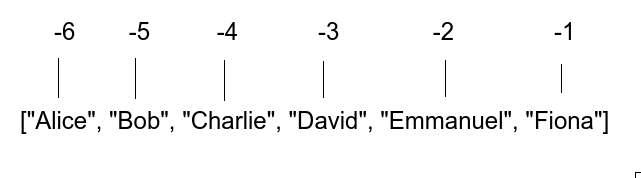
You can use negative indexing as your advantage when you want to pick values from the end (right side) of an iterable.
For instance, let’s get the last three names from a list of names:
names = ["Alice", "Bob", "Charlie", "David", "Emmanuel", "Fiona"] last = names[-1] second_last = names[-2] third_last = names[-3] print(last, second_last, third_last)
Output:
Fiona Emmanuel David
Slicing and Indexing in Python
Negative indexing is really useful with slicing iterables in Python.
If you are unfamiliar with the concept of slicing, please read this article.
Slicing in a Nutshell
In short, slicing means retrieving the desired subsequence of a sequence in Python. Slicing iterable returns a slice object. This is a part of the iterable, such as the first three numbers of a list of ten numbers.
Slicing follows this syntax:
iterable[start:stop:step]
Where:
- start is the starting index of the slice.
- stop determines the end of the slice. The stop index is excluded from the slice!
- step determines the step size of how many elements to jump over when slicing.
For example, let’s get the first three names from a list of names:
names = ["Alice", "Bob", "Charlie", "David", "Emmanuel", "Fiona"] first_three = names[0:3:1] print(first_three)
Output:
['Alice', 'Bob', 'Charlie']
It is useful to know:
- You can leave out the start parameter if you want to start from the beginning of the iterable.
- If you want to slice until the end of the iterable, you can omit the stop parameter.
- And if you want to take steps of size 1, you can leave the step parameter out too.
So the above example becomes:
first_three = names[:3]
Slicing “From the End” with Negative Indexes
In Python, slicing supports negative indexing too. This makes it easier to slice iterables from the end.
For instance, let’s get the last three names from the list:
names = ["Alice", "Bob", "Charlie", "David", "Emmanuel", "Fiona"] last_three = names[-3:] print(last_three)
Output:
['David', 'Emmanuel', 'Fiona']
Negative slicing also supports negative step size. This makes the slicing go backward.
For example, let’s reverse the list of names. To do this, you do not need the start and stop parameters, as you start from the beginning and stop at the end:
names = ["Alice", "Bob", "Charlie", "David", "Emmanuel", "Fiona"] last_three = names[::-1] print(last_three)
Output:
['Fiona', 'Emmanuel', 'David', 'Charlie', 'Bob', 'Alice']
Conclusion
Today you learned how to start indexing from the end of an iterable in Python.
To recap, Python supports positive zero-based indexing and negative indexing that starts from -1.
Negative indexing in Python means the indexing starts from the end of the iterable. The last element is at index -1, the second last at -2, and so on.
Thanks for reading. Happy coding!