In programming and computer science, the word “atomic” means “one at a time”.
Atomic operations are important in programs where a shared resource, such as a database, is accessed by multiple threads.
An atomic operation is an operation that appears to be instantaneous in the eyes of the other threads. In other words, you cannot catch an atomic operation in the middle. Instead, the operation is either completed or not.
The Origin of the Word “Atomic”
The word “atom” refers to something that is so elementary that it cannot be broken down any further.
During the earlier days in physics, an atom was taught to be the smallest possible object that cannot be split any further.
This is what the word “atomic” refers to in programming.
An atomic operation is an operation that cannot be split into smaller subprocesses.
Why Are Atomic Operations Important in Programming
In computer science, an atomic operation refers to an operation that either:
- Happens completely all at once.
- Does not happen at all.
In other words, there is no in-between state to the atomic operation. The atomic operation cannot be a “work in progress”.
Atomic operations are extremely useful in parallel programming, where multiple threads can access a shared resource simultaneously.
Before seeing an example, let’s support the understanding with a playful real-life example.
Real-Life Analogy
Let’s take a look at a real-life analogy to atomic operations.
If building a house was an atomic operation, it would mean that the house would appear from thin air in an instant.
In other words, there would be no building phase. The house would be either built or not started at all.
Now that you have a basic understanding of atomicity, let’s take a look at atomic operations in action.
Atomic Operations in Action
Atomic operations are crucial when it comes to accessing shared resources, such as databases.
Without atomic operations, some multi-threaded programs would not work.
To understand why let’s look at a simple example of transferring electric funds.
Imagine a couple, Alice and Bob doing some online shopping simultaneously.
Their mutual bank account has a total of $100 left in funds.
- Alice buys a $100 makeup kit.
- The banking software confirms there is enough balance to complete the purchase.
- At the exact same moment, Bob buys a $50 soccer ball.
- The banking software checks if there is at least $50 is in the account and confirms the purchase.
- Alice and Bob were able to spend $100 + $50 = $150, even though their account only had $100.
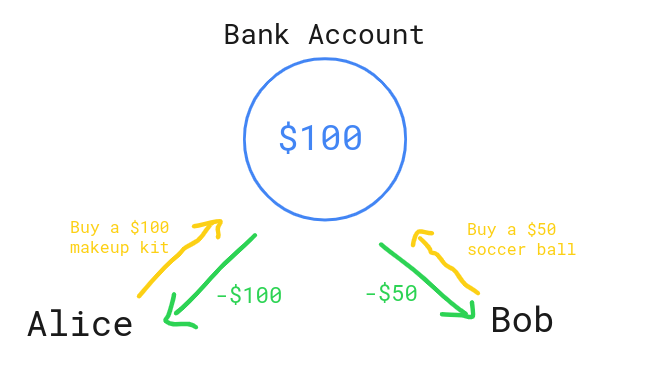
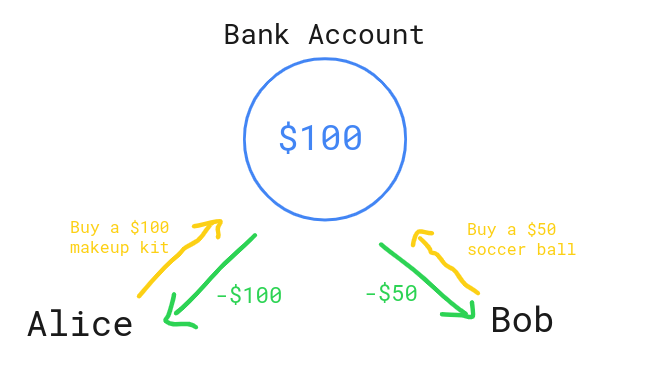
Alice and Bob were able to overdraw funds because the transaction operations are not atomic.
To fix this issue, the bank should introduce a mutual exclusion (mutex) system.
This system makes the process of checking the funds and withdrawing them invisible.
One way to do this is by locking the shared resource when it is being accessed. The resource should be unlocked only when the process is finished.
In other words, accessing the shared resource (the bank account) is turned into an atomic operation.
After updating the banking system to use atomic transactions, the previous experiment would play out like this:
- Alice buys a $100 makeup kit.
- The banking software locks the account for the transaction period.
- At the exact same moment, Bob buys a $50 soccer ball.
- The banking software waits for the account to be unlocked.
- Alice’s transaction completes. The balance drops to $0 and the account is unlocked.
- Bob’s transaction takes over and locks the account. The banking software notices there is no funds left, thus, invalidating Bob’s transaction.
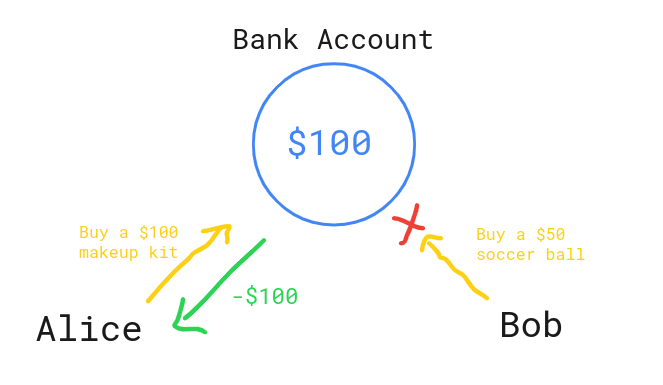
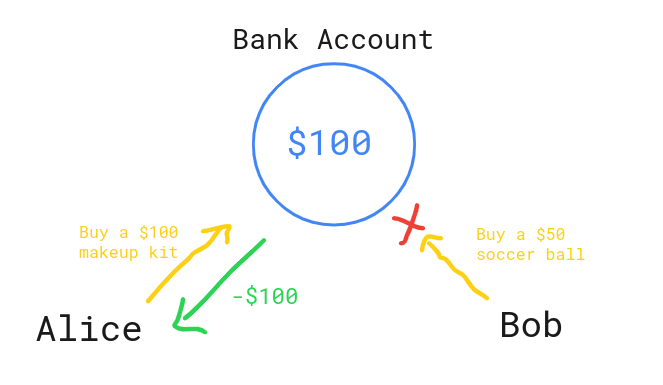
Now there is no way to make simultaneous transactions.
Instead, each transaction happens one at a time.
- If a transaction is completed, a new transaction can take place.
- If a transaction has not started yet, no other transactions can take place.
This is the basic description of atomicity and atomic operations in Programming.
Conclusion
Today you learned what “atomic” means in the context of programming and computer science.
To recap, atomic operations are important when it comes to accessing and modifying shared resources on a multi-threaded program.
Atomic operation means only one operation is possible at a time. An atomic operation has either not started or is fully completed. There aer no partially completed states.
If a bank transaction was not an atomic operation, users could overdraw bank accounts by making simultaneous transactions.
Thanks for reading.
Happy coding!