A loop refers to a set of instructions that is repeated as long as a criterion holds. The two types of loops are for loops and while loops. Both can be described using a flowchart.
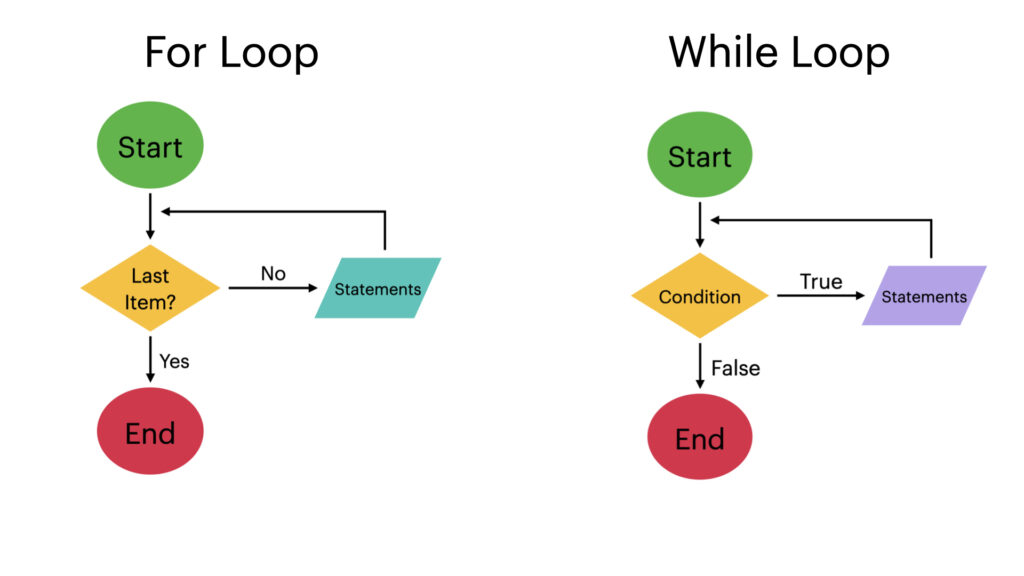
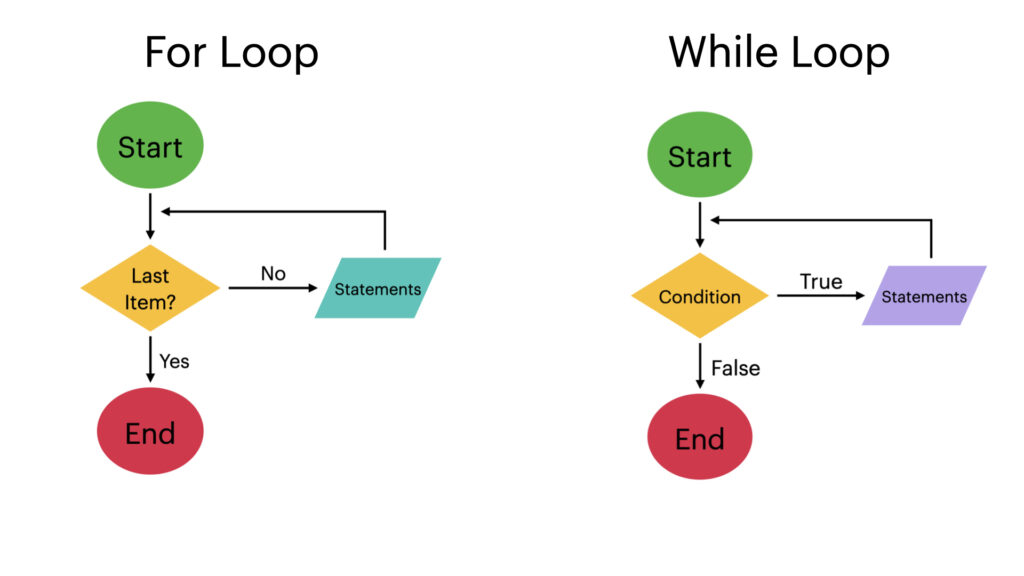
For Loop Flowchart with an Example
A for loop repeats statements as long as the last item in the range has not been reached yet.
Let’s create a simple for loop using Python. This loop prints out the numbers of a list.
numbers = [1, 2, 3, 4, 5] for number in numbers: print(number)
Output:
1 2 3 4 5
Here the print(number) is executed as long as there are numbers left in the list.
Here is a flowchart that describes the process:
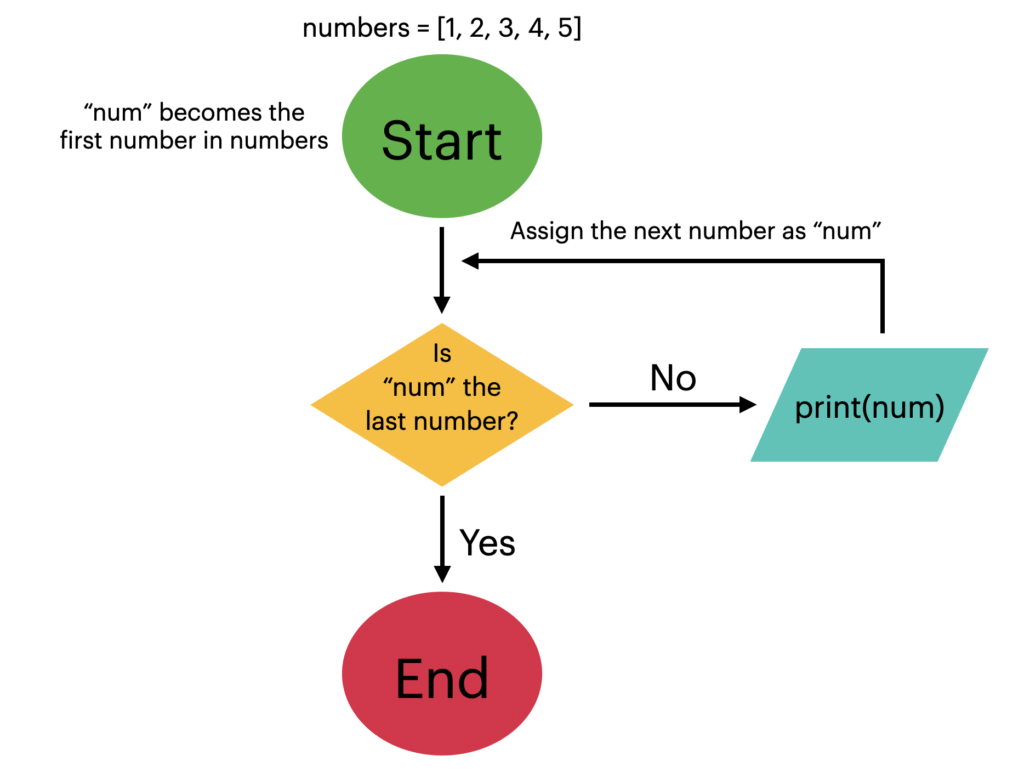
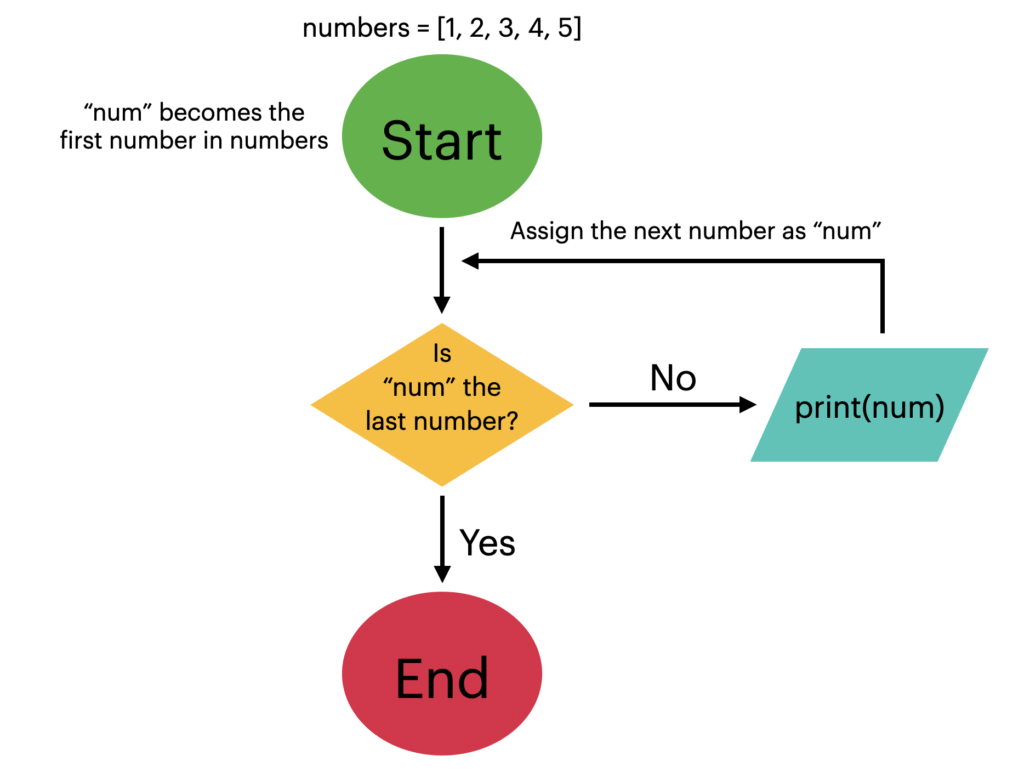
Flowchart for While Loop with an Example
The idea behind a while loop is to execute statements as long as a condition holds. When the condition becomes false, the statements are no longer executed. To avoid endless loops, you need to update the factors that affect the condition.
Let’s create a simple while loop for printing out a list of numbers using Python.
numbers = [1, 2, 3, 4, 5] i = 0 while i < len(numbers): print(numbers[i]) i += 1
Output:
1 2 3 4 5
In the above while loop, the condition is whether the index i
exceeds the length of the list. If it does, there are no more values in the list to be printed.
The example while loop above can be described with this flowchart:
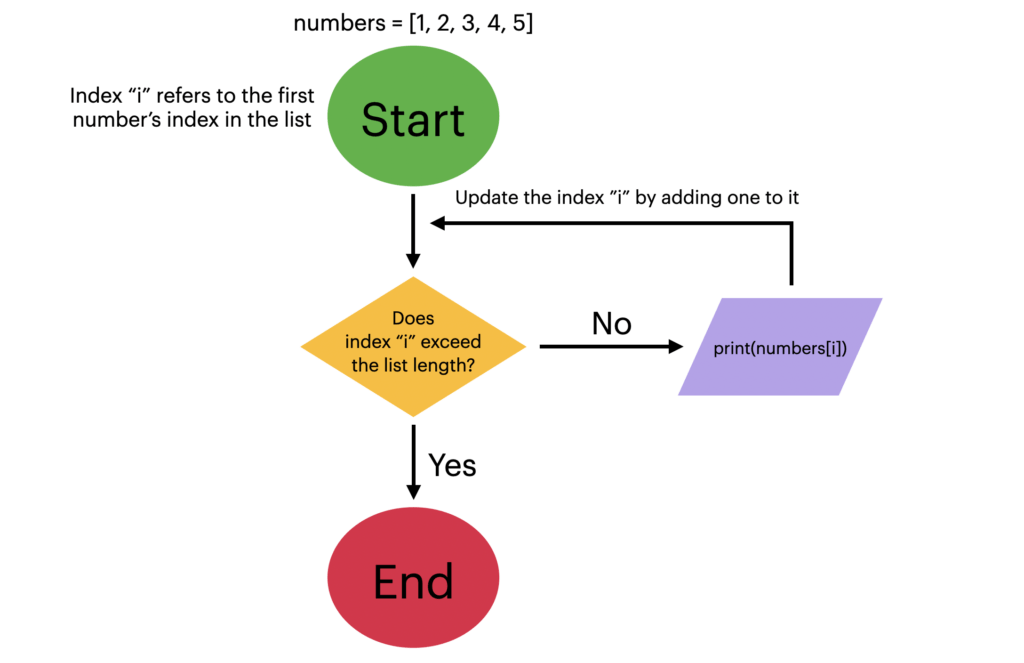
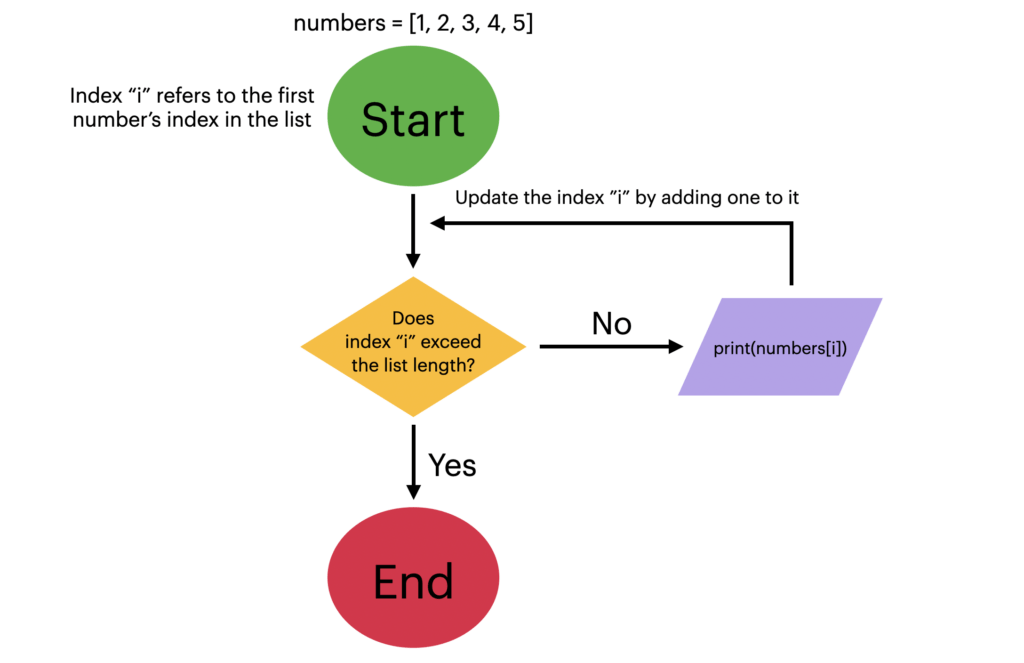
Conclusion
Today you saw some flowcharts of loops. I hope these help you understand how looping works in general, and in Python programming.
Thanks for reading. I hope you enjoy it
Happy coding!