In Swift, a for loop is used to repeat code. Commonly, you use for loops to iterate over collections in Swift.
For example, let’s loop through a list of names using a for loop:
let names = ["Alice", "Bob", "Charlie"] for name in names { print(name) }
Output:
Alice Bob Charlie
Here is a flowchart that depicts a for loop in Swift.
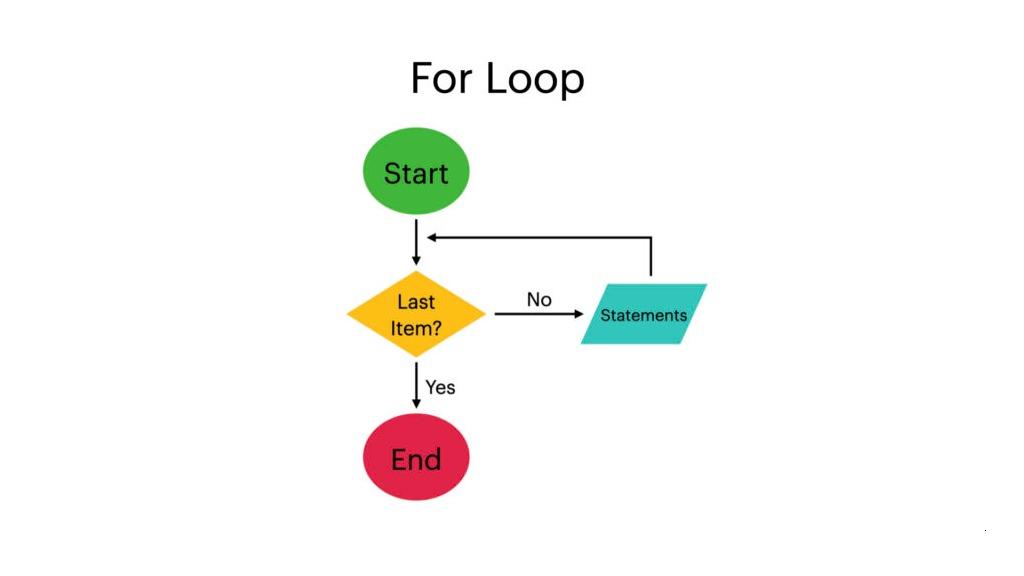
In this guide, you learn everything about for loops in Swift. More specifically, you learn how to use for loops to iterate over collections and ranges of values. You also learn how to control the flow of your for loops and much more.
For Loops in Swift
Looping means repeating an action over and over again.
For example, a loop could be used to show social media posts one by one in an app.
In Swift, there are two main types of loops:
- For loops
- While loops
In this guide, we are going to take a look at for loops in Swift.
In Swift, a for loop follows the for…in syntax.
for element in collection { // statements }
Here for element in collection
means “One by one, pick an item from a collection and call it element“. The curly braces that follow form a block of code in which you can perform actions for the elements.
For example, you can loop through a list of numbers and print each number out using a for loop:
let nums = [1, 2, 3] for n in nums { print(n) }
Output:
1 2 3
The block of code inside the for loop can be used to run any valid Swift code. However, it is important to notice that the block inside a loop has its own scope. This means you cannot access any variables declared inside the block.
For example:
for n in [1, 2, 3] { var something = 100 print(n) } print(something)
This results in an error:
main.swift:8:7: error: use of unresolved identifier 'something' print(something)
This error says it does not know what the variable something refers to. This happens because the variable something is declared inside a for loop. Thus, you cannot access it outside of the scope of the for loop, that is, inside of the parent scope.
However, you can access objects declared outside of the scope of the for loop inside the loop.
For instance:
var x = 100 for n in [1, 2, 3] { print(n) x += 10 } print(x)
This successfully prints the numbers in the list and the incremented x.
1 2 3 130
Now that you understand how a for loop works in Swift, let’s start using it with collections, such as arrays, dictionaries, and strings.
For Loops with Collections in Swift
Next up, you are going to learn how to use a for loop to iterate over the following Swift data types:
Iterate Elements of an Array
In Swift, an array is one of the most popular data types. You can use an array to store elements for later use.
For example, you can store a group of user objects into an array in your app. Then you can perform some useful operations for the array of users, such as sort them or delete some of them.
To loop through each element in an array, you can use a for loop, instead of manually accessing each element one by one.
For instance, let’s loop through a list of strings that represent names and print each name out:
let names = ["Alice", "Bob", "Charlie"] for name in names { print(name) }
Output:
Alice Bob Charlie
Iterate Key-Value Pairs in a Dictionary
Another popular data type in Swift is a dictionary.
You can store key-value pairs in a dictionary. The whole point of a dictionary is to associate each key with a value. You can later use that key to access the corresponding value. A dictionary in Swift works similar to a dictionary in real life.
If you want to iterate through a dictionary you can use a for loop.
However, unlike looping through an array looping through a dictionary requires you to declare two looping variables:
- One for the key.
- One for the value.
To do this, declare key and value as variables in a loop by comma-separating them.
for (key, value) in dictionary { // statements }
For instance, let’s loop through a dictionary that consists of data related to a person:
let data = [ "name": "Alice", "age": "30", "address": "Imaginary Road 22" ] for (key, value) in data { print("\(key): \(value)") }
Output:
age: 30 name: Alice address: Imaginary Road 22
Here each key is assigned to the key variable and the corresponding value to the value variable one by one.
Iterate Letters in a String
In Swift, a String is a collection of characters. Because a string is a collection, it can also be looped through using a for loop.
For example, let’s loop through a string and print out each letter:
let greeting = "Hello" for letter in greeting { print(letter) }
Output:
H e l l o
As you can see, looping through a string follows the exact same structure as looping through an array of values.
Iterate Enum Cases
In Swift, an enumeration is used to group together related values for type safety. It allows using an “official” type instead of hard-coded strings for example.
By the way, if you are unfamiliar with enumerations, please read this article or skip to the next section.
An enumeration (enum) is characterized by the cases defined in it.
But what you might not know is you can loop through the enumeration cases using a for loop. To make this possible, you need to make the enumeration CaseIterable.
For example, let’s create an enumeration for directions and loop through the cases of the enumeration:
enum Direction: CaseIterable { case North case East case South case West } for case_ in Direction.allCases { print(case_) }
Output:
North East South West
Awesome!
So far you have focused on looping through collections in Swift. Next, let’s take a look at how to loop through a range in Swift.
For Loops with Ranges in Swift
A range is an interval from the lower bound to the upper bound.
Swift has two types of ranges:
Let’s take a look at each type of range in Swift and how to loop through them.
Closed Range
In Swift, you can create a closed range of values using the … operator.
start...end
Where
- start is the starting value of the range.
- end is the end value of the range.
This produces a range of values with 1 step interval.
One way to use a range in Swift is to create a range of numbers that can be looped through.
For example, let’s create a range of numbers from 1 to 5 and print them into the console by using a for loop:
for i in 1...5 { print(i) }
Output:
1 2 3 4 5
Using a range to produce numeric values can be useful especially if there are many numbers you want to include. This is because using a range you do not have to write down the numbers manually.
Half-Open Range
Another type of range in Swift is the half-open range. To create one, use the ..< operator.
start..<end
Where
- start is the starting value of the range
- end is the end value of the range. In a half-open range this value is not included in the range.
The half-open range also produces a range of numbers separated by 1.
Just like looping through a regular range in Swift, you can use a for loop to loop through a half-open range.
For example, let’s loop through numbers from 1 to 4:
for i in 1..<5 { print(i) }
Output:
1 2 3 4
For Loops with Stride in Swift
Another range-like object in Swift is called a stride.
A stride is a sequence of numbers where you can customize how many numbers to step over.
stride(from:to:by)
Where:
- from is the starting number.
- to is the (excluded) end number.
- by is the stepsize.
A stride is useful for creating ranges of numbers that have a stepsize other than 1. Striding also makes it possible to create reversed ranges.
A stride object can also be looped through using a for loop.
For example, let’s loop through every second number from 10 to 0.
for n in stride(from: 10, to: 0, by: -2) { print(n) }
Output:
10 8 6 4 2
Now that you understand how to use for loops with ranges in Swift, let’s move on to filtering for loops with the where clause.
Where Clause in For Loop
In Swift, you can use a where clause to filter elements based on a condition. It can be used instead of using an if statement.
When it comes to for loops, you can place a where clause right after the loop to check a condition.
For example, let’s loop through numbers from 1 to 10 and print even numbers only (numbers for which n % 2 == 0 holds):
for n in 1...10 where n % 2 == 0 { print(n) }
Output:
2 4 6 8 10
Next, let’s take a look at how to keep track of an index in a for loop.
For Loop with Index in Swift
By default, a for loop in Swift has no idea about the index of the elements.
However, sometimes the index can be handy. To keep track of the index, you can specify a separate variable that you update on each iteration of the loop.
However, as this is such a common thing to do, Swift has a built-in mechanism for it. To group each element in an array with an index, use the Array.enumerated() function.
Array.enumerated()
In Swift, Array.enumerated() function groups each element in an array with an index. This is a handy way to keep track of the index of a current element in a for loop.
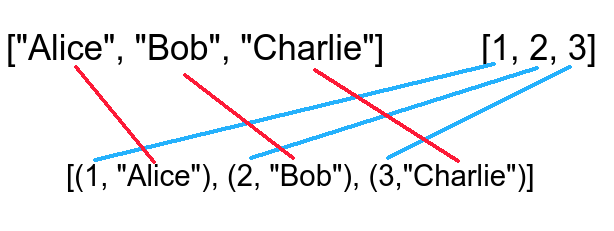
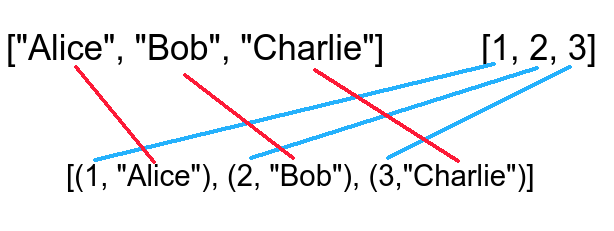
For instance, let’s print the position of each name in a queue:
let names = ["Alice", "Bob", "Charlie"] print("Queue order:") for (position, name) in names.enumerated() { print(" \(position + 1). \(name)") }
Output:
Queue order: 1. Alice 2. Bob 3. Charlie
This is super useful as there is no need to keep track of a separate index variable that would need to be updated in each iteration.
If you are only interested in the indices of the elements, you can also resort to the Array.indices property.
Array.indices
If you are solely interested in the indices of the elements of an array, use the Array.indices property. It returns a collection of numbers that represents the indices of the elements.
For example, let’s print the indices of the elements of an array:
let names = ["Alice", "Bob", "Charlie"] for i in names.indices { print(i) }
Output:
0 1 2
Next, let’s take a look at how to deal with optionals in for loops.
For Loops with Optionals
Sometimes a collection can contain optional values. In other words, the values can be either defined or nil.
To filter out the nil values in a collection of optionals, you can use the for case let element?
structure in the for loop. This executes the for loop only for the non-nil elements. It stores the non-nil value to a variable called element.
for case let element? in collection { // statements }
For example:
let names: [String?] = ["Alice", nil, nil, "Bob", "Charlie", nil] for case let name? in names { print(name) }
Output:
Alice Bob Charlie
As you can see, the nil values were skipped altogether.
It is important to understand that the above piece of code is just a shorthand for checking if a name is nil this way:
let names: [String?] = ["Alice", nil, nil, "Bob", "Charlie", nil] for name in names { if let name = name { print(name) } }
Output:
Alice Bob Charlie
Last but not least, let’s have a look at how you can alter the flow of a for loop in Swift.
Control Flow Statements in For Loops
Sometimes you need to control the flow of a for loop in Swift.
A common use case for altering the flow of a for loop is to stop the current iteration and jump into the next one. To do this, use the continue statement.
Also, you may want to escape a for loop altogether based on some condition. To do this, use the break statement.
Next, let’s take a look at how the control flow statements continue and break work in Swift.
Continue – Jump to the Next Iteration of a Loop
In Swift, the continue statement stops the current iteration of a for loop and starts the next one.
With continue you can avoid doing excess processing in the for loop when a criterion is not met.
For example, let’s skip printing the name that equals “Bob” in an array of names:
let names = ["Alice", "Bob", "Charlie"] for name in names { if name == "Bob" { print("*Skipping*") continue } print(name) }
Output:
Alice *Skipping* Charlie
When the looping parameter name is Bob if statement returns true and the continue statement gets executed. This means the current iteration of the loop stops right there, and the name never gets printed out. Instead, the next iteration starts.
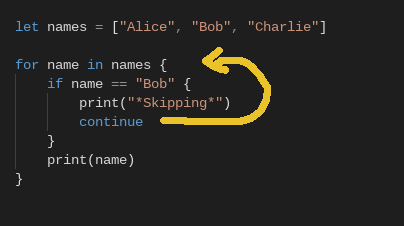
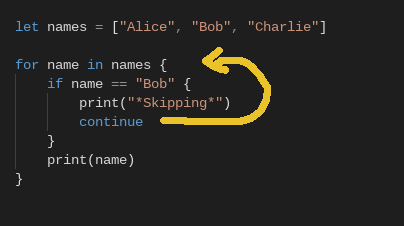
Break – Exit the For Loop
In Swift, the break statement escapes the a for loop altogether.
This can be useful if you are searching for a value in a for loop. When the desired value is encountered the loop is exited to not run unnecessary code.
For example, let’s search for “Bob” in an array of names. When “Bob” is encountered, we jump out of the loop.
let names = ["Alice", "Bob", "Charlie"] for name in names { if name == "Bob" { print("*Terminating to \(name)*") break } print(name) }
Output:
Alice *Terminating to Bob*
As you can see, the last name “Charlie” never got printed out. This is because the loop was terminated to “Bob”.
Here is an illustration of how the break statement works in the above example.
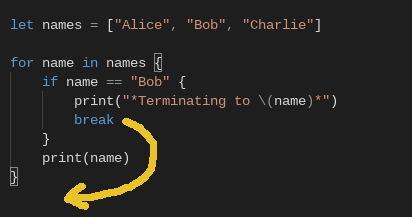
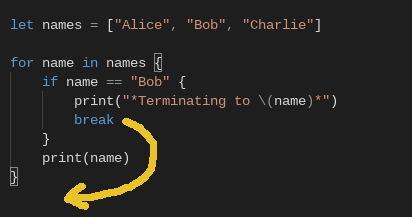
Now you should have a good understanding of what is a for loop and how you can use them in different situations in Swift.
Before we go, let’s take a peek at some for loop alternatives of Swift.
For Loop Alternatives in Swift
Last but not least, it is important to realize for loops are not the only loops in Swift.
In addition to for loops, you can iterate over collections using while loops, repeat-while loops.
In addition to these, Swift comes with a number of built-in higher-order functions for collections that can be used to loop through them. These include the forEach and the map functions.
Without digging further into the details, here are some examples of each of the aforementioned
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] // Print all the numbers with while loop var i = 0 while i < numbers.count { print(numbers[i]) i += 1 } // Repeat at least once with while-repeat loop var j = 1 repeat { print("Yay!") j += 1 } while (j <= 1) // Square numbers using map function let squared = numbers.map { $0 * $0 } // Print all numbers with forEach function numbers.forEach { print($0) }
Conclusion
Today you learned how to work with for loops in Swift.
To recap, a for loop is a commonly used loop type in Swift. You can use a for loop to iterate over collections and ranges of values one by one in an iterative fashion.
You can control the flow of a for loop using continue and break statements.
Thanks for reading.
Happy coding!