Python list is a common data type used to store multiple values for easy access. Sometimes, you may want to clear Python lists either by removing all the elements or removing elements one by one.
This is a comprehensive guide to clearing a list in Python in 4 different ways.
The short answer: To clear the whole list in Python, use the list.clear() method.
For example, let’s wipe out a list of numbers:
numbers = [1, 2, 3, 4, 5] numbers.clear() print(numbers)
Output:
[]
To remove an item at a specific index, use the list.pop(index) method.
For example, let’s remove the second element of a list:
nums = [1,2,3] nums.pop(1) print(nums)
Output:
[1, 3]
All in all, there are four ways to remove elements from a list in Python:
- The clear() method.
- The pop() method.
- The remove() method.
- The del statement.
Let’s go through each of these.
1. Clear() in Python
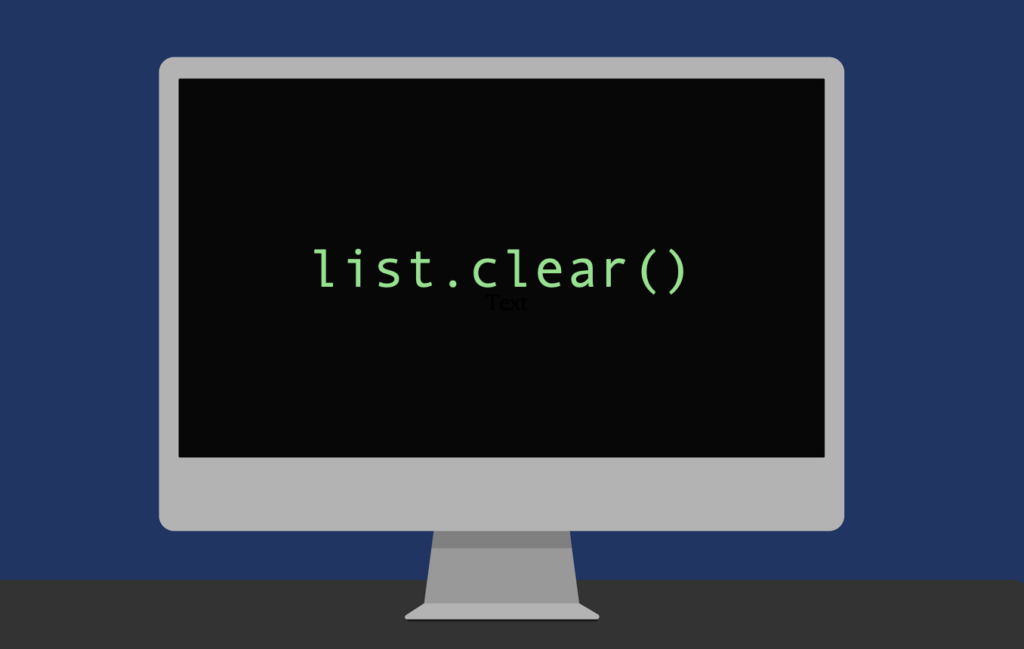
To completely clear a list in Python, use the built-in clear() method. This removes all the elements of the original list.
For instance:
numbers = [1, 2, 3, 4, 5] numbers.clear() print(numbers)
Output:
[]
Now there are no longer values in this list.
Notice that this is not always what you want. Sometimes you only want to get rid of particular elements of the list. The rest of this guide focuses on removing particular elements rather than wiping out the entire list.
2. Pop() Method in Python
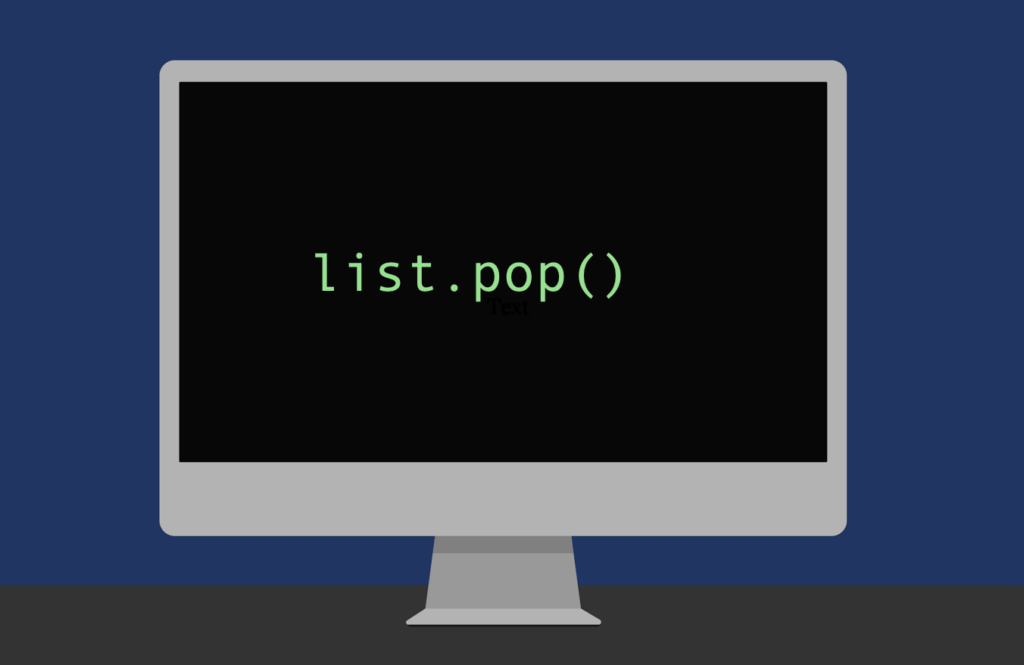
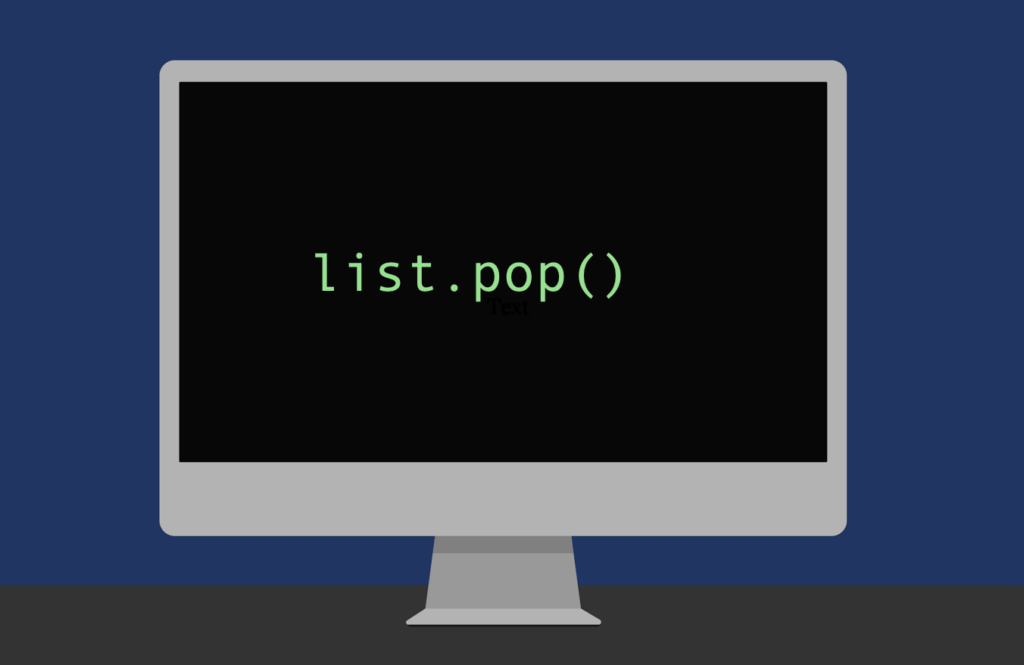
You can remove a single element with a specific index using the list’s pop() method.
To do this, pass the index of the item you want to remove as an argument. (And keep in mind that Python indexing starts at 0):
For example, let’s remove the second number from a list of numbers:
numbers = [1,2,3] numbers.pop(1) print(numbers)
Output:
[1, 3]
Notice the pop() method also returns the removed value. If you need to store it somewhere, you don’t need to do it separately.
3. Remove() Method in Python
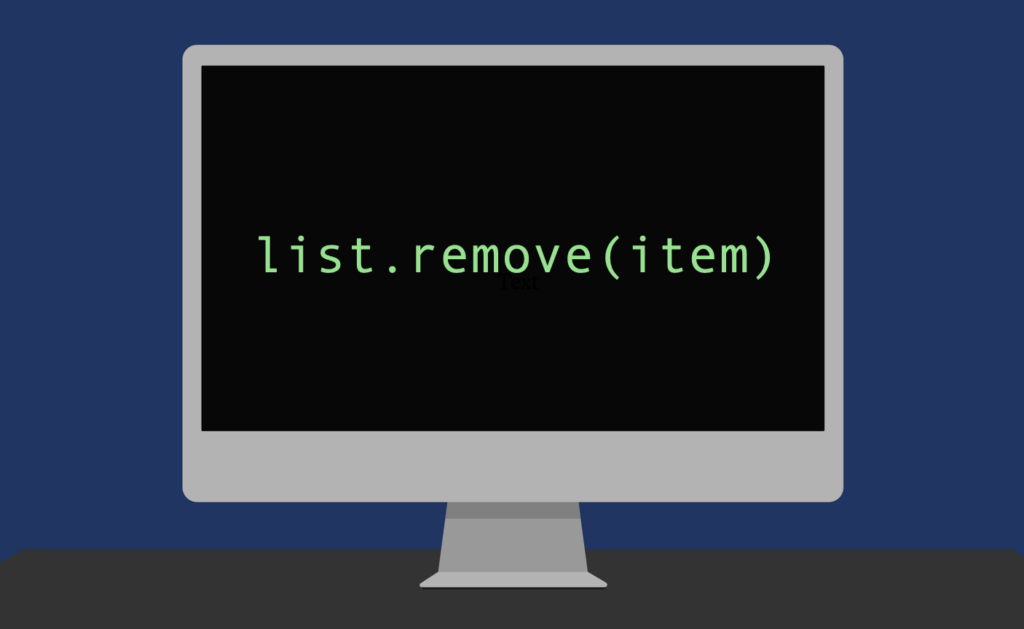
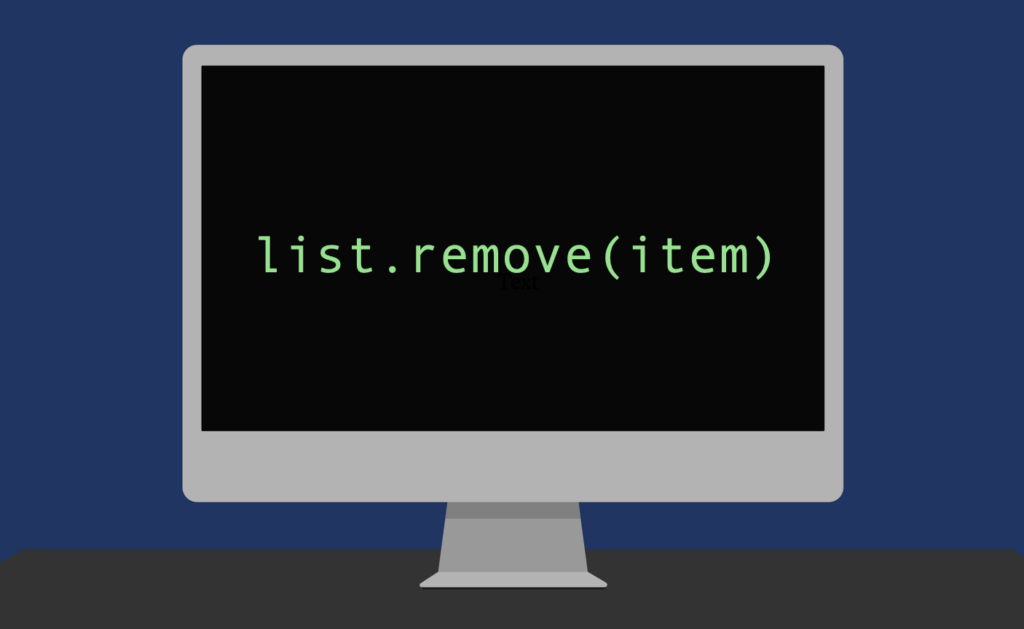
Another way to remove elements from a list is by using the list.remove() method to remove the first occurrence of a particular element from the list. To do this, pass the element you want to remove into the method.
For instance, let’s remove the first occurrence of the number 2 in the list of numbers:
nums = [1,1,2,2,3,3] nums.remove(2) print(nums)
Output:
[1,1,2,3,3]
4. The del Statement in Python
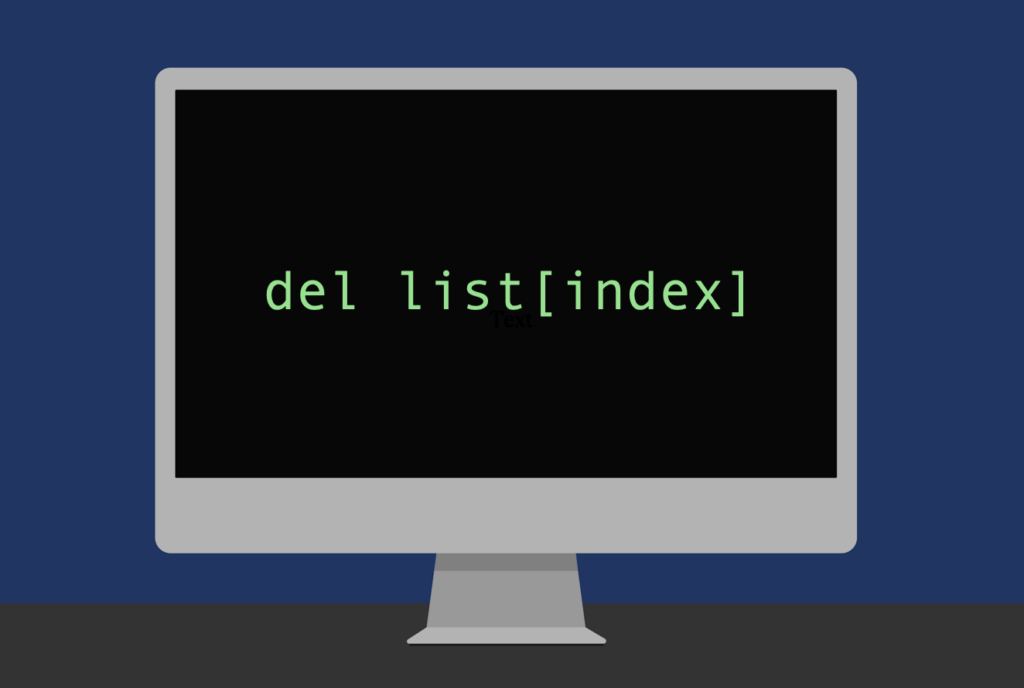
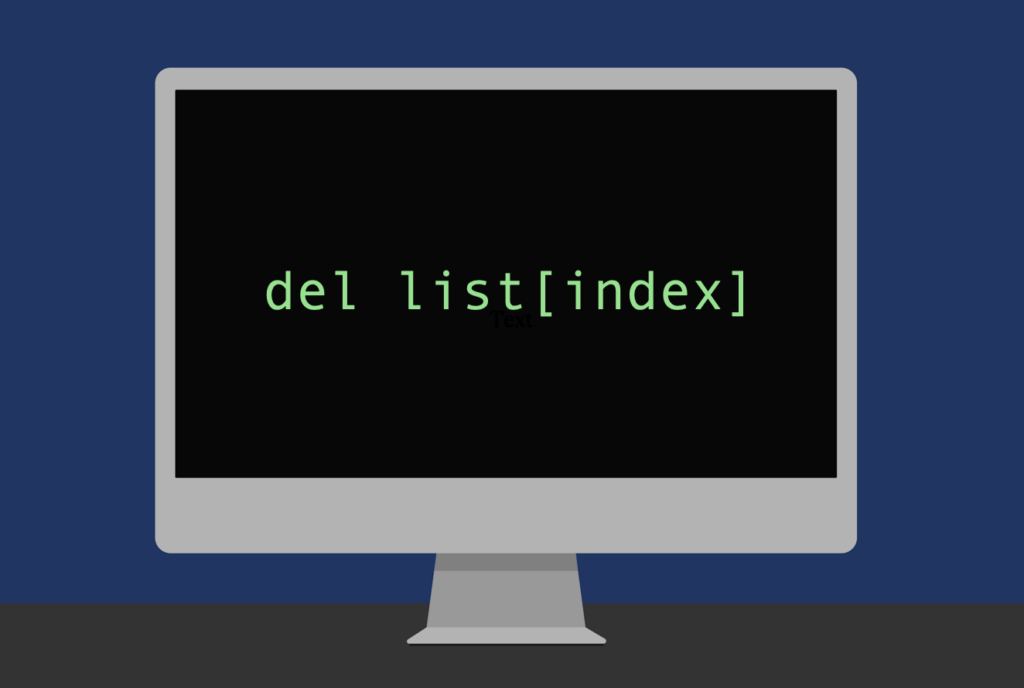
Last but not least, you can also remove elements from a list using the del statement.
To do this specify the index of the element you wish to remove.
For example, let’s remove the first element of a list of numbers:
nums = [1,2,3] del nums[0] print(nums)
Output:
[2, 3]
In contrast to the pop() method you saw earlier, the del statement only deletes the element and does not return it.
Conclusion
- To clear a whole Python list, use the clear() method of a list.
- To remove an element at a specific index, use the pop() method or the del statement.
- To remove the first occurrence of an element in a list, use the remove() method.
Thanks for reading.
Happy coding!