In Python, you can place an else statement at the end of a loop.
The else block only runs if a break statement was not used in the loop.
For example, let’s loop through a list of numbers and break the loop if a target number is encountered:
numbers = [1, 5, 43, 2, 7, 9, 19, 10] target = 100 for number in numbers: if number == target: print("Target found, escaping the loop") break else: print("Target not found. The loop ran through all the numbers.")
Output:
Target not found. The loop ran through all the numbers.
The target number was not found. Thus, the break statement was not used. This caused the else statement to run.
This guide teaches how to use the else statement in a for loop/while loop. The theory is backed up with useful real-life examples.
The ‘else’ Statement in Python
Most of the time you use the else statement in an if-else statement to perform actions if the if block is not executed on a False condition.
For example:
age = 10 if age >= 18: print("You can drive") else: print("You cannot drive")
Output:
You cannot drive
But you can also place an else statement to the end of a for loop or a while loop. However, the meaning of an else statement in a loop is quite different from what you expected.
The ‘else’ Statement in Loops
When used in a loop, the else statement checks if a break statement was used.
- If a break statement is used, the loop is terminated and the else block is not going to be executed.
- If a break statement is not used, the loop runs all the way through and triggers the else block.
In other words, a loop that does not have a break statement will always run the else block.
By the way, if you don’t know how the break statement works, the next section is a quick primer. If you are familiar with this stuff, feel free to skip to the next section.
The ‘break’ Statement in Python
In Python, you can control the flow of a loop.
Normally, the loop body is executed line by line from top to bottom.
But you can change this by using one of Python’s built-in control flow statements:
- The continue statement. Skips the rest of the current iteration and starts the next one.
- The break statement. Terminates the loop altogether.
These statements can be used in both for and while loops.
Let’s see an example of the continue statement by printing all the even numbers in a list of numbers:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] for number in numbers: if number % 2 != 0: continue print(number)
Output:
2 4 6 8 10
Here the if statement checks if the number is odd. If it is, the continue statement is used to skip printing the number.
Sometimes you also might want to terminate the whole loop before it finishes.
This is useful in situations where you want to avoid excess looping, such as when you find a target value.
To terminate a loop in Python, use the break statement.
When the loop encounters a break statement, it terminates the loop and continues executing the program from the next line.
For example, let’s search for a target number and exit the loop if the number is found:
numbers = [1, 5, 43, 2, 7, 9, 19, 10] target = 43 for number in numbers: print(number) if number == target: print("Target found, escaping the loop") break
Output:
1 5 43 Target found, escaping the loop
As you can see from the output, the loop was terminated once the target number was found.
Here it makes sense to stop the loop because why continue searching for something you already found?
This was a quick intro to the control flow statements and especially the break statement in Python.
Let’s continue with the topic of the day, that is, how and why to use the else block in a loop in Python.
First, let’s look at how the else statement works in a for loop.
The ‘else’ Statement in a For Loop
In Python, you can place an else statement into a for loop.
To do this, insert the else keyword into the same indentation level as the for keyword.
for var in iterable: # loop actions else: # actions after loop
The else statement works such that if a break statement is not used, the else block will run.
Let me show you an example.
Let’s use a for loop to iterate over a list of numbers in search of a target number. If the target number is found, let’s break the loop. If not, let’s print a message.
For example:
numbers = [1, 5, 43, 2, 7, 9, 19, 10] target = 100 for number in numbers: if number == target: print("Target found, escaping the loop") break else: print("Target not found. The loop ran through all the numbers.")
Output:
Target not found. The loop ran through all the numbers.
Here the else block is executed because the target number was not found and the break statement was not used.
Let’s see another example.
If you specify an else block to a for loop that does not have a break statement, the else block is always executed:
for i in range(5): print(i) else: print("Loop completed")
Output:
0 1 2 3 4 Loop completed
Using the else block this way makes no sense because it will always run. Here you could display the message right after the loop without using an else block.
for i in range(5): print(i) print("Loop completed")
It only makes sense to specify an else block into a loop to perform actions if the loop was not stopped by a break statement.
The next chapter teaches you how to use the else block in a while loop. TLDR; the idea is exactly the same as using it in the for loops.
The ‘else’ Statement in a While Loop
In Python, you can also insert an else statement into a while loop.
To do this, add the else keyword into the same indentation level as the while keyword.
while condition: # loop actions else: # actions after loop
Identical to the for loop example, if a break statement is not used in a while loop, the else block will run.
For example, let’s search for a target number using a while loop:
numbers = [1, 5, 43, 2, 7, 9, 19, 10] target = 100 i = 0 while i < len(numbers): if numbers[i] == target: print("Target found, escaping the loop") break i += 1 else: print("Target not found. The loop ran through all the numbers.")
Output:
Target not found. The loop ran through all the numbers.
Because the target number was not found, the break statement was never used. Thus, the else block was executed.
Last but not least, let’s briefly discuss the inconvenience of this.
Why ‘else’?
Now you understand how to use the else block in loops in Python.
But isn’t it confusing?
Most likely you did not expect the else block to work that way.
Syntactically, it is not clear that the else block only runs if the loop was not terminated by a break statement.
Perhaps Python authors did not want to create a new keyword for such a situation, even though they probably should.
For instance, it would be much cleaner if one could use something like nobreak instead of else in a loop.
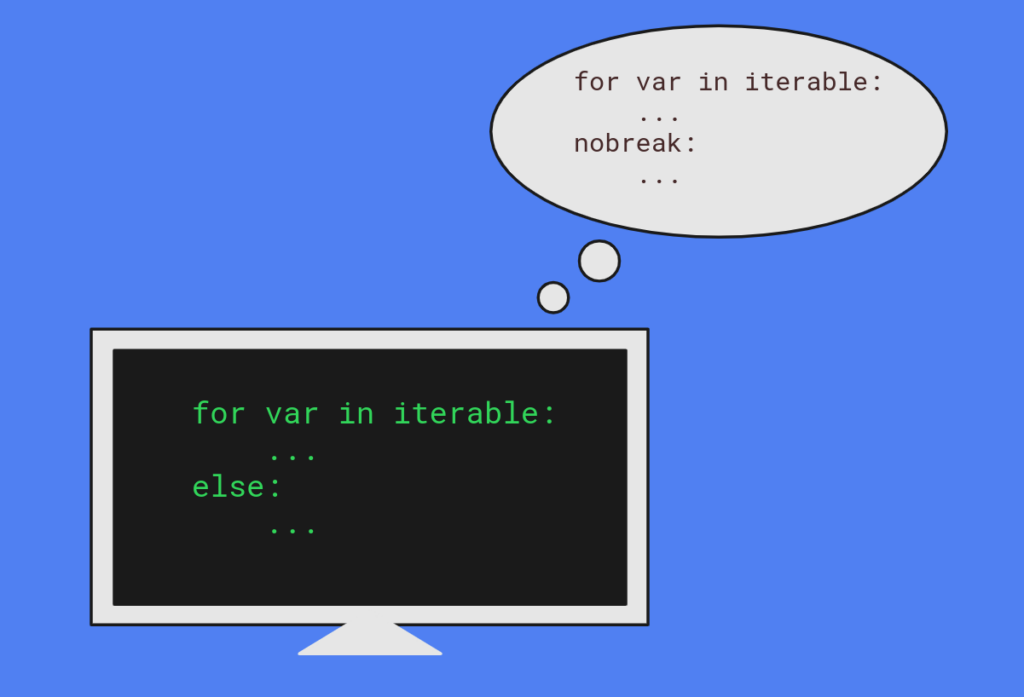
Conclusion
In Python, you can place an else statement after a loop.
This is executed if the break statement was not used in the loop. In other words, if the loop completes without interruption.
If a break statement is used, the else block will not run.
This is counterintuitive because else is not the best keyword to describe this. The authors of Python could have done a better job by introducing a new keyword such that nobreak.
Thanks for reading.
Happy coding!