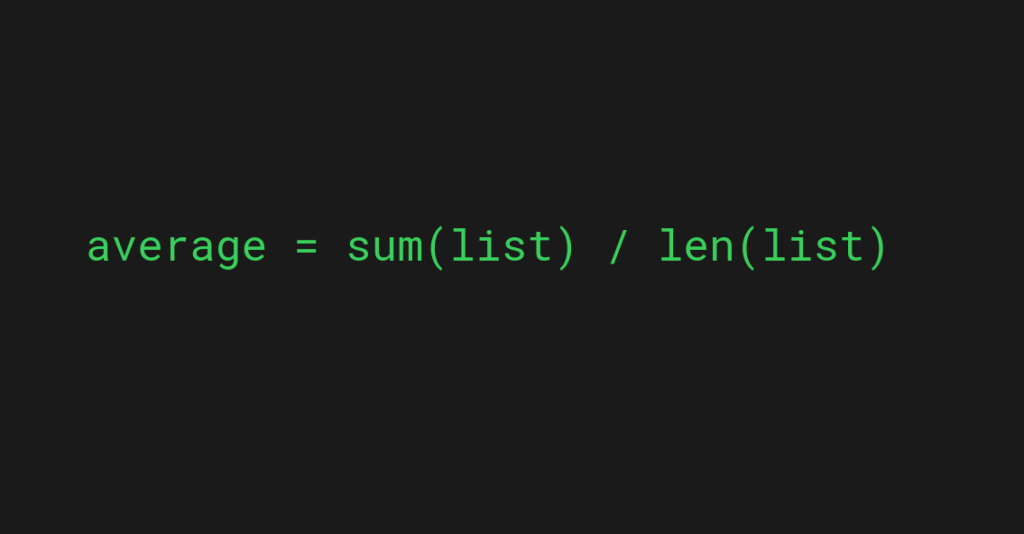
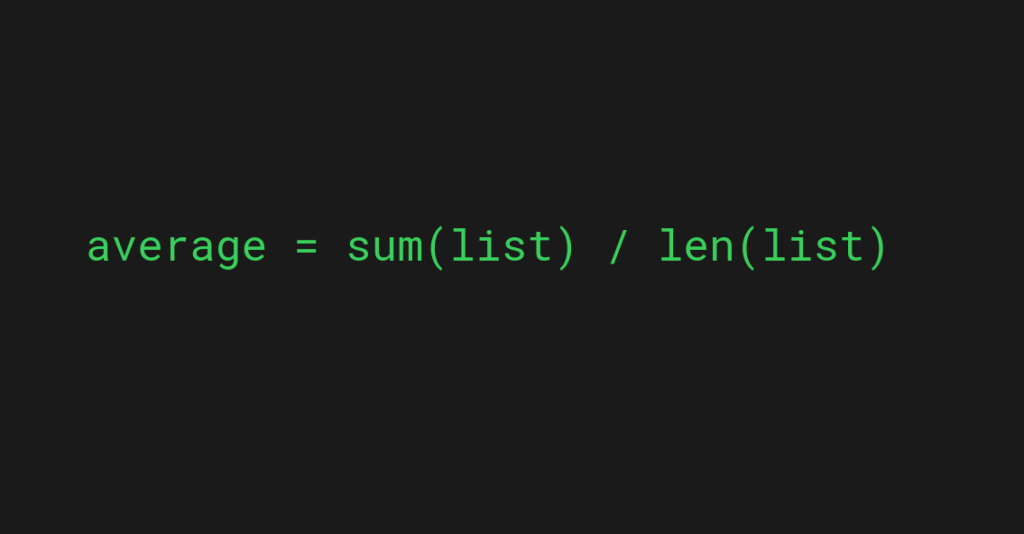
To find the average of a list in Python:
- Sum up the elements of a list.
- Divide the sum by the length of the list.
For instance, let’s calculate an average of a list of numbers:
grades = [4, 3, 3, 2, 5] average = sum(grades) / len(grades) print(f"The average is {average}")
Output:
The average is 3.4
This is a comprehensive guide to calculating the average of a list in Python. In this guide, you learn four separate ways for calculating averages.
- The for-loop approach
- The statistics mean() function
- The numpy mean() function
- The reduce() function
4 Ways to Calculate the Average of a List in Python
Let’s take a closer look at four different ways to calculate the average of a list in Python.
1. The For Loop Approach
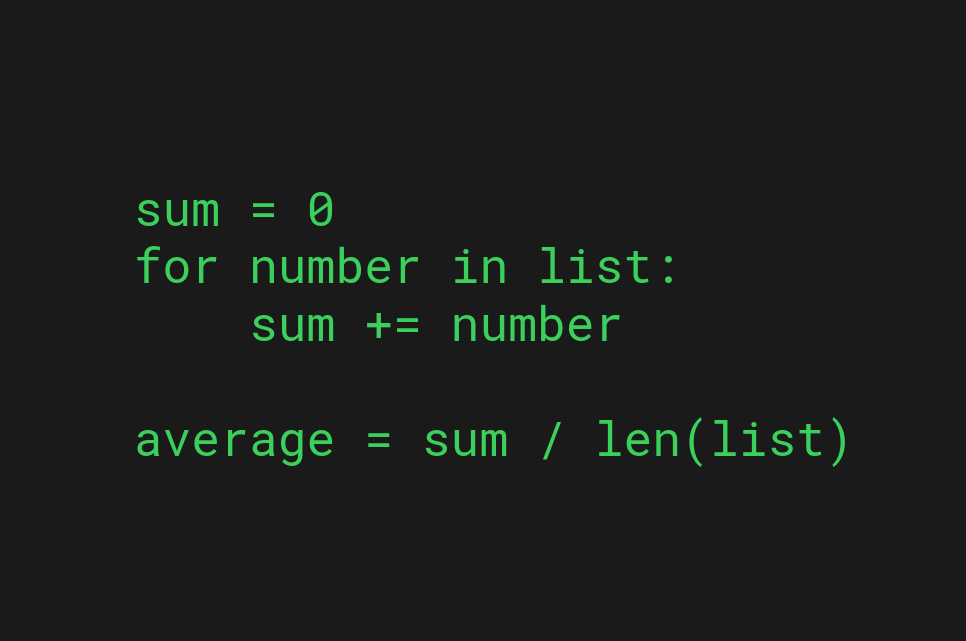
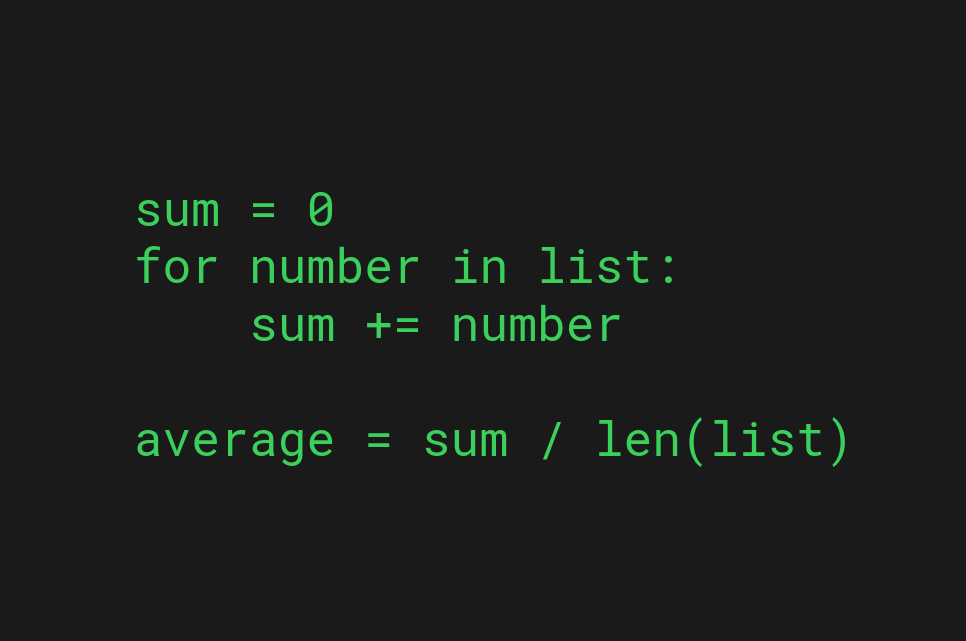
The basic way to calculate the average of a list is by using a loop.
In this approach, you sum up the elements of the list using a for loop. Then you divide the sum by the length of the list.
For example, let’s calculate the average of grades using a for loop:
grades = [4, 3, 3, 2, 5] sum = 0 for number in grades: sum += number average = sum / len(grades) print(f"The average is {average}")
Output:
The average is 3.4
2. Statistics mean() Function
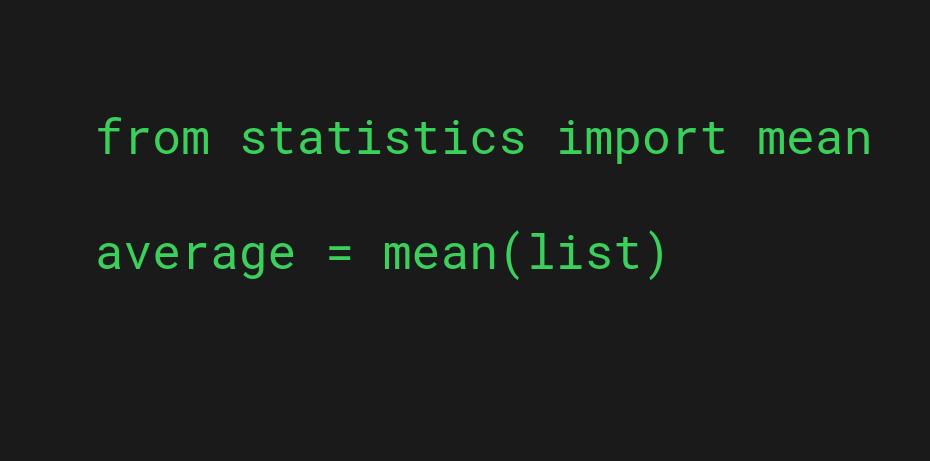
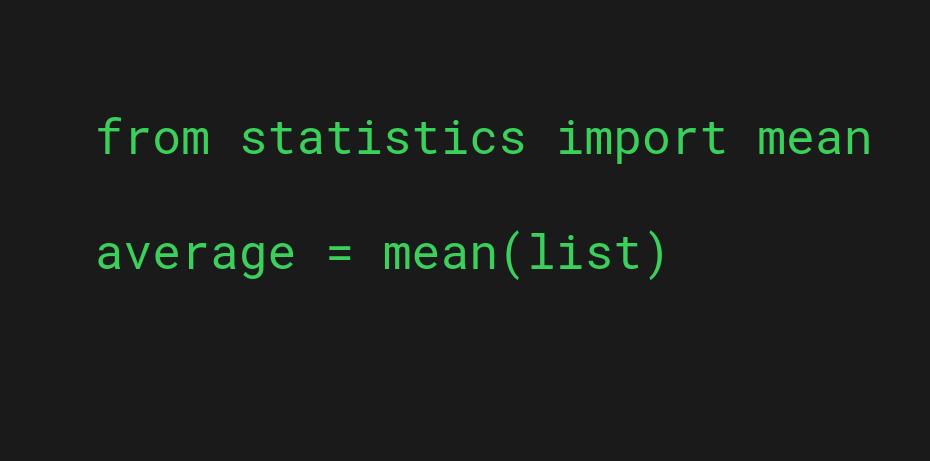
Python has a built-in library called statistics. This library provides basic functionality for mathematics in statistical analysis.
One of the useful functions provided by the statistics library is the mean() function. You can use it to calculate the average for a list.
For example:
from statistics import mean grades = [4, 3, 3, 2, 5] average = mean(grades) print(f"The average is {average}")
Output:
The average is 3.4
3. NumPy mean() Function
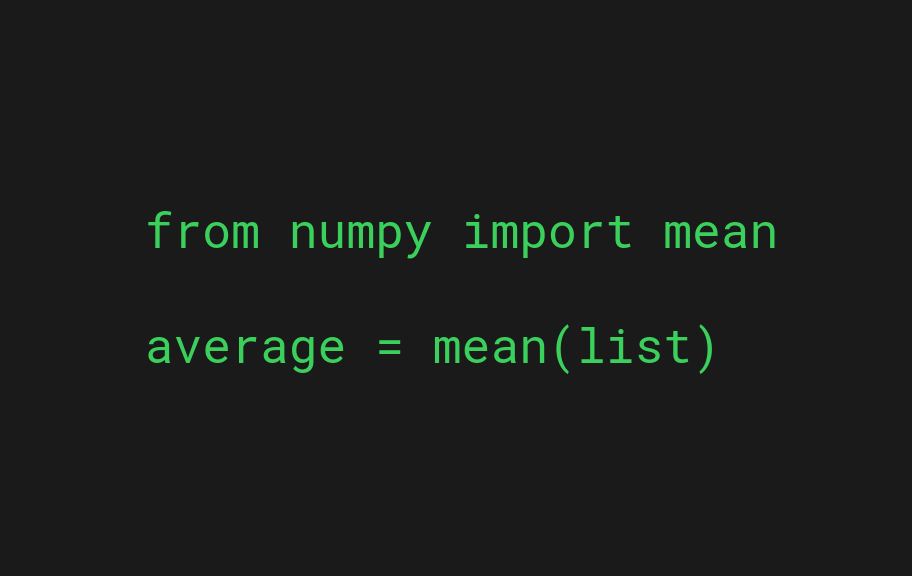
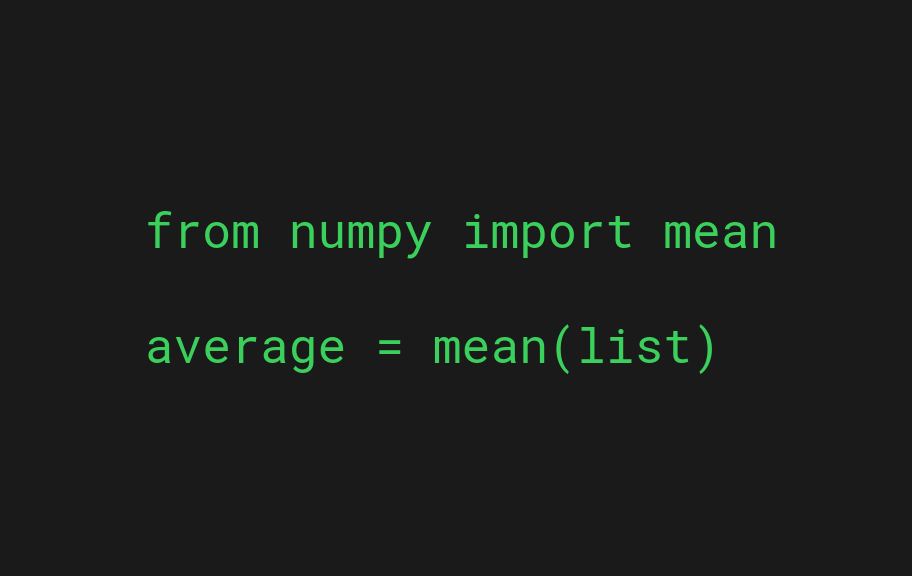
NumPy is a commonly used Python library in data science. It provides you with powerful numerical computing tools and multi-dimensional arrays.
This library has also its own implementation of the mean() function.
To use it, first install NumPy by running the following command in your command line window:
pip install numpy
With Numpy installed, you can use the mean() function to calculate the average of a list:
from numpy import mean grades = [4, 3, 3, 2, 5] average = mean(grades) print(f"The average is {average}")
Output:
The average is 3.4
Notice that installing and using the mean() function from NumPy is overkill if you’re never going to use the NumPy library again. But if you’re already using it in your project, then calculating the average with it might make sense.
4. reduce() Function in Python
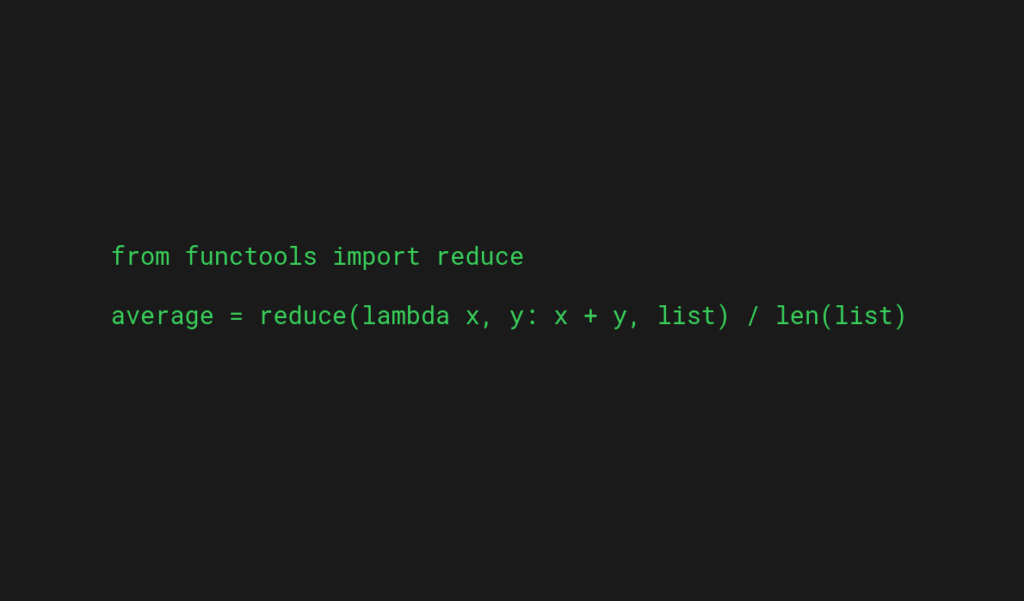
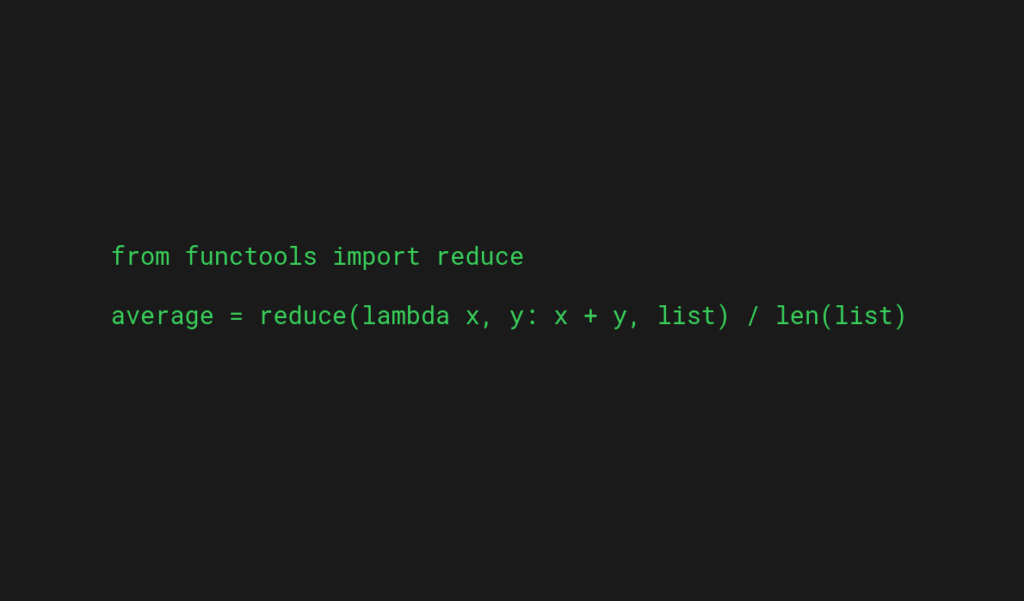
Python’s functools library has a function called reduce(). You use this function when computing the average of a list by calculating the sum of the list.
Notice that using reduce in Python isn’t recommended anymore as better solutions exist. Make sure to read my article about the reduce() function to understand how it works and why it’s not used anymore.
Anyway, for the sake of demonstration, let’s use the reduce() function to calculate the average of a list:
from functools import reduce grades = [4, 3, 3, 2, 5] average = reduce(lambda x, y: x + y, grades) / len(grades) print(f"The average is {average}")
Output
The average is 3.4
The reduce() function works by applying an operation on two elements of a list. It remembers the result and applies the operation to the next element and the result. It does this to accumulate a result for the whole list.
The operation in this example is the lambda expression lambda x, y: x + y. This is nothing but a function that takes two arguments x and y and returns the sum.
When we call reduce() on a list using this lambda, we tell it to sum up the numbers of a list.