In Python, you can use sys.maxsize
from the sys
module to get the maximum and minimum integer values.
The sys.maxsize
gives you the maximum integer value and its negative version -sys.maxsize - 1
gives you the minimum integer value.
import sys # Get the maximum integer value max_int = sys.maxsize # Get the minimum integer value min_int = -sys.maxsize - 1 # Print the values print(f"The maximum integer value is {max_int}") print(f"The minimum integer value is {min_int}")
Notice that Python 3.0+ doesn’t limit the int
data type and there’s no maximum/minimum value. But to get the practical maximum integer value for a single integer word in your operating system, use sys.maxsize
as shown above.
This guide teaches you how to get the maximum and minimum integer values in Python.
You will understand why maximum and minimum integer values exist in the first place. You’ll also learn how to get the maximum/minimum integer values in Python versions earlier than 3.0 as well as Python 3.0+. Besides, you’ll learn how to get the maximum/minimum integers in NumPy.
Let’s jump into it!
Integer Value Extrema
Computer memory is limited. A typical operating system uses a 32-bit or 64-bit system for representing numbers. This means there are 2³² or 2⁶⁴ numbers the system can represent.
Notice that the maximum/minimum integer size restriction is not a Python-only thing as it depends on the capabilities of the device, not the programming language.
Let’s take a closer look at what limits the size of an integer in general.
Why Is There a Maximum/Minimum for Integers?
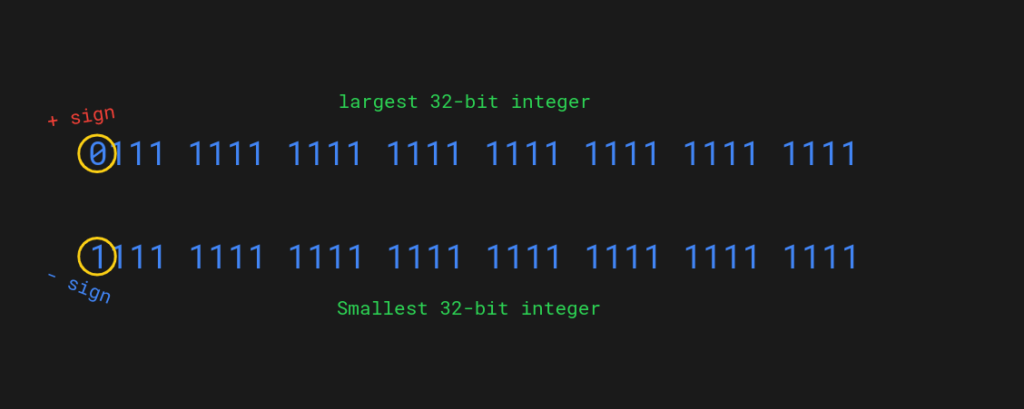
A maximum integer is the maximum number that a binary store can hold. Many operating systems use 32 bits for storage. This means you have to fit any number that you want to use in those 32 bits.
A bit is a binary digit whose value is either 0 or 1. If you form a line of these bits, you can get a number of different combinations of 0s and 1s.
For example, if you have 2 bits, you have 2² = 4 possible combinations:
- 00
- 01
- 10
- 11
And for N bits, you have 2^N possible combinations.
A computer that uses 32 bits to represent numbers has a total number of 2³² = 4,294,967,296 possible combinations. This means the computer can represent 2³² numbers in total such that each combination of bits corresponds to a number in the decimal number system.
But as you know, you don’t only have positive numbers but also negatives and zero. But bits don’t understand negative signs. Instead, a computer can use 1 as a negative sign and 0 as a positive sign.
In a 32-bit sequence, this means that the sign is denoted by the left-most bit (1 for negative, 0 for positive values). Because one bit is used as a sign, it leaves 31 bits for representing the actual numbers.
This means the biggest integer in a 32-bit system is 0 followed by 31 1’s. In other words, 2³⁰ + 2²⁹ + … + 2² + 2¹ + 2⁰. This is 2³¹ – 1 or 2,147,483,647. So you can count from 0 to 2,147,483,647 using this type of 32-bit signed integer system.
When it comes to negative values, the idea is exactly the same. The smallest negative value is 1 followed by 31 1’s, that is, 2³⁰ + 2²⁹ + … + 2² + 2¹ + 2⁰. As a negative value, this is -2,147,483,647. But remember, because the 0 value is already included in the range of countable positive numbers, we start counting the negative values from -1 instead of 0. This means the smallest possible negative value in the 32-bit signed integer system is actually one less than -2,147,483,647, that is, -2,147,483,648.
And if the computer uses 64 bits for storing numbers, the idea is the same.
The maximum value of a 64-bit signed integer is 2⁶³ – 1 = 9,223,372,036,854,775,807 and the minimum value is -(2⁶³ – 1) – 1 = -9,223,372,036,854,775,808.
To take home, the maximum and minimum values for integers in programming languages are determined by the amount of memory that is allocated for storing them and the type of integer that is being used. These values are important because they determine the range of values that an integer variable can store, and they can affect the precision and accuracy of calculations that involve integers.
Python Maximum Integer Value
To obtain the maximum value of the integer data type, use the sys.maxsize
.
import sys # Get the max integer value max_int = sys.maxsize print(f"The maximum integer value is {max_int}")
Python Minimum Integer Value
To obtain the minimum value of the integer data type, use the negative sys.maxsize
value and subtract 1 from it to account for 0 being in the positive value range.
import sys # Get the min integer value min_int = -sys.maxsize - 1 print(f"The minimum integer value is {min_int}")
Python 3+ ‘int’ Type Has No Limits!
As of Python 3, the int type is unbound. This means there’s no limit to how big a number you can represent using the int type.
Back in Python versions earlier than 3.0, the int type was bound to be between [-2⁶³, 2⁶³ – 1]. If you wanted to use a number larger or smaller than what is in the range, you’d have to use the long
data type. As a matter of fact, a conversion from int
to long
takes place automatically.
As an example, let’s try printing some large numbers in Python 2 where the int type was still bounded:
print(9223372036854775807) print(9223372036854775808)
Output:
9223372036854775807 9223372036854775808L
Notice the ‘L’ at the end of the second number. Because 9223372036854775808
is bigger than the maximum int
value, it automatically became a long
which Python denotes by adding ‘L’ to the end.
But in Python 3, the int type is unbounded. Essentially, what was long in Python 2 is now int in Python 3+. Besides, the ‘L’ letter is no longer added to the end of big integers!
Let’s repeat the previous example in Python 3:
print(9223372036854775807) print(9223372036854775808)
Output:
9223372036854775807 9223372036854775808
Even though there’s no limit to how big an integer can be in Python, the sys.maxsize
gives you the upper bound for practical lists or strings. The maximum value works as a sentinel value in algorithms, so even though it’s not a hard limit in Python integers, it indicates the maximum word size used to represent integers behind the scenes.
If this makes no sense, let’s see what happens if you try to use an integer larger than sys.maxsize
to access a list element:
l = [1,2,3,4,5,6] value = l[10000000000000000000000000000000000]
Output:
Traceback (most recent call last): File "<stdin>", line 1, in <module> IndexError: cannot fit 'int' into an index-sized integer
The error says that the integer index you’re using to access the list elements is too big to be an index. This happens because of the limitations of your operating system. A Python index must still be an integer bounded by the 32-bit or 64-bit limits.
So even though the int
is unbounded in Python, the underlying operating system still uses either 32 or 64 bits to represent numbers.
Thanks for reading. Happy coding!