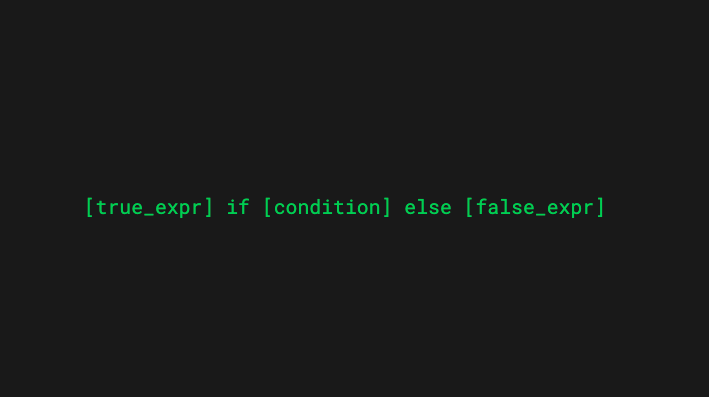
A Python ternary operator makes it possible to write if-else statements as simple one-liner expressions.
Here’s what the syntax of a ternary operator looks like in Python.
[true_expr] if [condition] else [false_expr]
This is a complete guide to using the ternary operator in Python.
You will learn why and when you should or should not use it. The theory is backed up with great illustrative examples.
First, let’s talk about ternary operators in general because they are not just a Python thing, but a concept found in other programming languages.
Ternary Operators in Coding
One of the fundamental concepts of programming is if-else statements. With an if-else statement, developers can add logic to their code. This allows you to write programs that have decision-making capabilities.
A typical if-else statement in general follows this pattern (in pseudo-code):
if condition { true_expressions } else { false_expressions }
But in some very basic if-else statements, it may not be necessary to expand a conditional statement like this to multiple lines.
This is why many programming languages support short-hand expressions for if-else statements called ternary operators. Sometimes the ternary operator is also called a conditional expression or simply a one-liner if-else statement.
In your typical programming language, a ternary operator might look something like this:
condition ? true_expr : false_expr
Where the true_expr is executed if the condition is true and the false_expr is executed if the condition evaluates false.
Python also supports ternary conditional operators. But the syntax is a bit different than what other programming languages’ ternary operators look like.
Python Ternary Operator
In Python, you can compress an if-else statement as a one-liner expression by using the ternary conditional operator.
In Python, the ternary operator follows this rather unique syntax:
true_expr if condition else false_expr
Where true_expr is executed if the condition is True and false_expr if the condition evaluates False.
Notice that you can only apply the ternary conditional operator with single expressions. If you have more than one expression to run, use a traditional if-else statement.
Also, you need to have both the true_expr and false_expr in place. In other words, you cannot have a ternary operator without the else part.
Let’s see examples.
Example 1.
For example, let’s use a ternary operator to convert an if-else statement into a one-liner expression.
First, have a look at this piece of code.
age = 25 if age < 18: candrive = False else: candrive = True print(candrive)
Output:
True
This small Python script checks the age variable and determines whether a person with that age can drive or not. This particular example uses a very basic if-else statement to make the age check.
With a ternary conditional operator, you can compress this if-else statement into a one-liner expression.
Here’s what the exact same piece of code looks like when using the ternary operator:
age = 25 candrive = False if age < 18 else True print(candrive)
Output:
True
This way you can save some lines of code when working with basic if-else statements.
But don’t go change all your multi-line if-else statements! There are many reasons why you should not use the ternary operator. Next, you will learn the pros and cons of ternary operators in Python.
The Downside of Python’s Ternary Operators
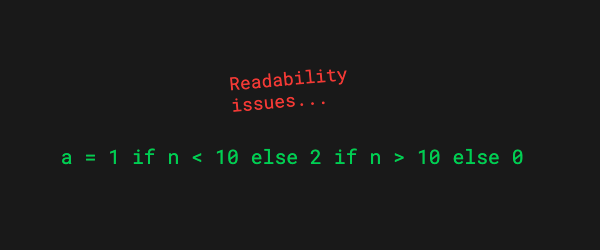
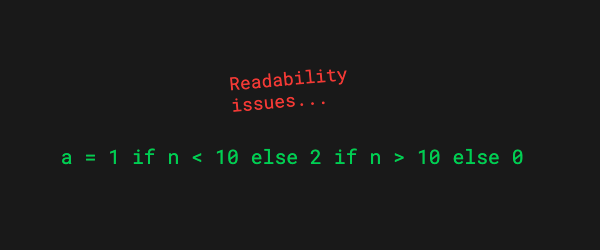
Even though ternary conditional operators let you write fewer lines of code by compressing if-else statements as one-liners, you shouldn’t always do it. As a matter of fact, most of the if-else statements you write are more readable when written in multiple lines.
Example 2.
For example, consider this ternary conditional operator example:
n = 10 a = 1 if n < 10 else 2 if n > 10 else 0
This one-liner is an example of nested ternary operators. For sure, all the code takes place in one line but it’s really hard to read.
Now, let’s change this one-liner back to a traditional if-elif-else statement:
n = 10 if n < 10: a = 1 elif n > 10: a = 2 else: a = 0
Much better, isn’t it?
This example shows you an important lesson about code readability. Even if you could express something as a one-liner, it doesn’t mean you should.
To take home, only use the ternary operator if it makes your code more readable. If reducing three lines of code comes at the expense of readability and maintainability, there’s no reason to do it.
Summary
In Python, you can use ternary operators to shorten your if-else statements.
true_expr if condition else false_expr
A ternary conditional operator can be useful if the if-else statements contain simple logic and compressing the code into one line doesn’t hurt the code quality.
But even with slightly more complex expressions, you should keep if-else statements on multiple lines.
Thanks for reading. Happy coding!