The key difference between a function and a method in Python is:
- A function is implemented outside of a class. It does not belong to an object.
- A method is implemented inside of a class. It belongs to an object.
Calling a function on an object looks like this:
function(object)
And calling a method of an object looks like this:
object.method()
Here is a simple illustration of this key difference:
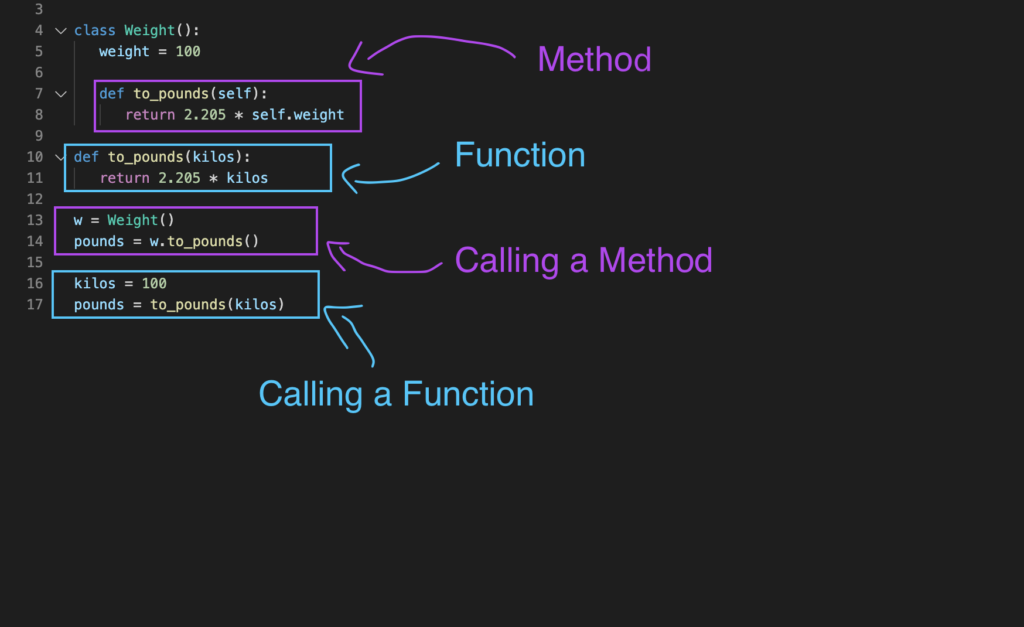
Let’s take a look at a table that summarizes the main differences between methods and functions in Python. Notice that most of these differences apply to other programming languages too.
Functions vs Methods in Python—A Comparison Table
Here is a comprehensive comparison table that compares the key differences between methods and functions in Python.
Methods | Functions |
---|---|
A method lives in a class. | A function lives outside classes. |
A method is associated with a class object. | A function is not associated with any objects. |
You can only call a method on an object. Calling a method by its name only is not possible. | You can call a function by only using its name. |
A method operates on the data of the object it belongs to. | A function operates on the data you give it as an argument. |
A method is dependent on the object it belongs to. | A function is an independent block of code in the program. |
A method always requires ‘self‘ as the first argument. | A function doesn’t take ‘self‘ as an argument. |
Function vs Method Example in Python
Probably the most famous function in Python is the print()
function. You can call it on any object to output the object as text in the console.
To print a string, for example, call print()
function on a string.
For example:
print("Test")
This is a great example of calling a function on an object in Python.
To demonstrate methods next, let’s continue with the string data type.
The string type str
has a ton of built-in methods. One of which is the upper()
method that converts the string object into uppercase.
To convert a string into uppercase, call the upper()
method of a string.
string = "Test" upper_string = string.upper()
This is a great example of calling a method on an object in Python.
Methods vs Functions: A Code Example
Here is a simple code example of a class with a method. Outside the class, there is a function with the same name.
Please read the code comments to understand what’s going on.
class Weight(): weight = 100 # Defining a method def to_pounds(self): return 2.205 * self.weight # Defining a function def to_pounds(kilos): return 2.205 * kilos # Calling a method on an object. w = Weight() pounds = w.to_pounds() # Calling a function on an object kilos = 100 pounds = to_pounds(kilos)
Conclusion
Today you learned what is the difference between a function and a method in Python.
- A function does not belong to a class. It is implemented outside of a class.
- A method belongs to a class and it can only be called on objects of that class. A method is implemented inside of a class.
An example of a function in Python is the print()
function.
An example of a commonly used method in Python is the upper()
method of a string.
Thanks for reading. I hope you find it useful.
Happy coding!