In Python, the built-in str()
and repr()
functions both produce a textual representation of an object.
import datetime today = datetime.datetime.now() print(str(today)) print(repr(today))
Output:
2021-10-14 10:15:31.405463 datetime.datetime(2021, 10, 14, 10, 15, 31, 405463)
The difference between str()
and repr()
is:
- The
str()
function returns a user-friendly description of an object. - The
repr()
method returns a developer-friendly string representation of an object.
In this guide, you learn:
- What are the functions
str()
andrepr()
. - How to override the behavior of these functuons in custom objects.
Example of str() and repr() in Python
Let’s create a datetime
object for today, and inspect its string representations:
import datetime today = datetime.datetime.now() print(str(today)) print(repr(today))
Output:
2021-08-14 08:18:25.138663 datetime.datetime(2021, 8, 14, 8, 18, 25, 138663)
-
str()
displays today’s date so that an end-user could understand it. -
repr()
prints the developer-friendly representation of the date object. This string is valid Python you can use to reconstruct the date object.
How Do str() and repr() Work Under the Hood
- When you call
str()
on an object, it calls the special method__str__
of the object. - And when you call
repr()
on an object, it calls the special method__repr__
of the object. - Also, when you call
print()
on an object, it calls__str__
method of the object. If__str__
is not implemented, the__repr__
is called as a fallback.
So, the special methods __str__
and __repr__
define how the object is presented “textually”. These special methods are implemented in the class that produces the objects.
By the way, make sure to read my complete guide to the __repr__() method in Python.
Custom Classes with String Representations
If you’re writing your own class, it may be useful to create a clear textual representation of the class for both developers and end-users.
This means you need to implement the special methods __str__
and __repr__
into the class.
For example, let’s say you have a class that represents Fruits:
class Fruit: def __init__(self, name): self.name = name
If you create a Fruit
object and print it:
banana = Fruit("Banana") print(banana)
You get a somewhat unambiguous output:
<__main__.Fruit object at 0x7f0ece0e8d00>
This is the default string representation of an object. But this string representation is not useful for a user.
You can change the default string representation by providing an implementation to the string representation methods, __str__
and __repr__
. Conventionally the __str__
should produce a user-friendly result and __repr__
a developer-friendly one.
For example:
class Fruit: def __init__(self, name): self.name = name def __str__(self): return f'I am a {self.name}' def __repr__(self): return f'Fruit("{self.name}")'
Now, if you create a Fruit object and print it:
banana = Fruit("Banana") print(banana)
You get an output:
I am a Banana
This is because the print()
function calls the __str__
method of the instance under the hood. So essentially the above is equivalent to:
print(banana.__str__())
Similarly, if you print the string representation of the fruit object using the str()
function:
print(str(banana))
You get an output:
I am a Banana
Because calling str(banana)
is the same as calling banana.__str__()
.
And using repr()
produces a more developer-friendly string representation:
print(repr(banana))
Output:
Fruit("Banana")
Because calling repr(banana)
is the same as banana.__repr__()
.
Now you know how to provide a string representation for your custom objects and what is the difference between __str__
and __repr__
.
Real-life Usage of __str__ and __repr__
Let’s go back to the datetime
example in the very beginning:
import datetime today = datetime.datetime.now() print(str(today)) print(repr(today))
Output:
2021-08-14 08:18:25.138663 datetime.datetime(2021, 8, 14, 8, 18, 25, 138663)
So str()
and repr()
call __str__
and __repr__
methods behind the scenes.
Let’s take a source dive into Python’s source code. As we want to know how the datetime
works, let’s inspect the datetime.py
file.
It seems that the base class for datetime
is date
. Let’s see if we can find methods __repr__
and __str__
from the date
class.
If you search for class date
and scroll down, you find the __repr__
method. This is what is responsible for producing the output when calling repr()
on a datetime
object.
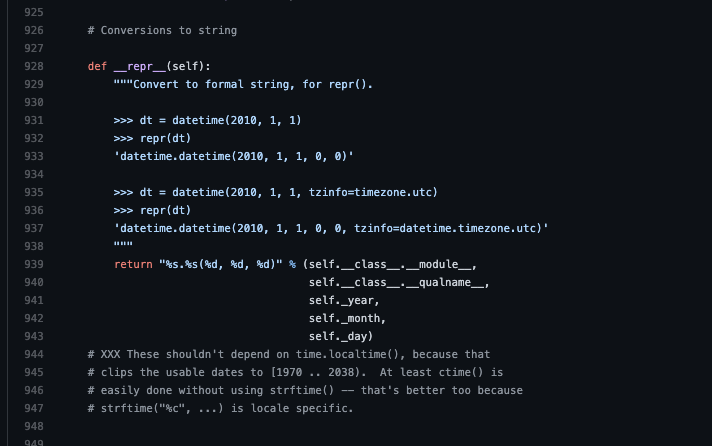
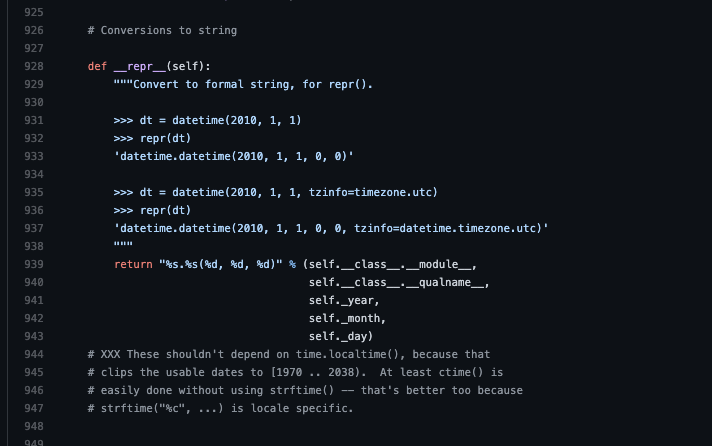
If you scroll down a bit further, you also encounter the __str__ method that shows the user-friendly representation of the date:
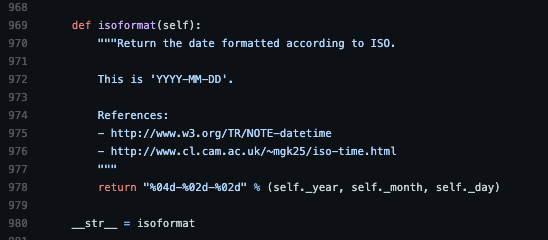
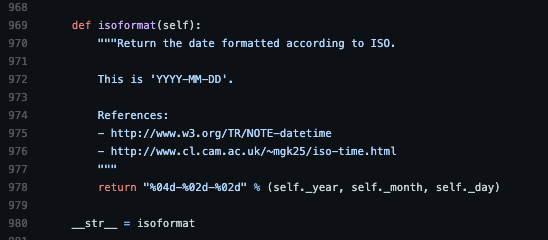
The __str__
method, in this case, is the isoformat
function. This means the default string representation of a datetime
object is the date in the ISO format.
Conclusion
Both str()
and repr()
return a “textual representation” of a Python object.
The difference is:
-
str()
gives a user-friendly representation -
repr()
gives a developer-friendly representation.
In Python, you can customize the string representations of your objects by implementing methods __str__
and __repr__
.
Thanks for reading. I hope you find it useful.
Happy coding!