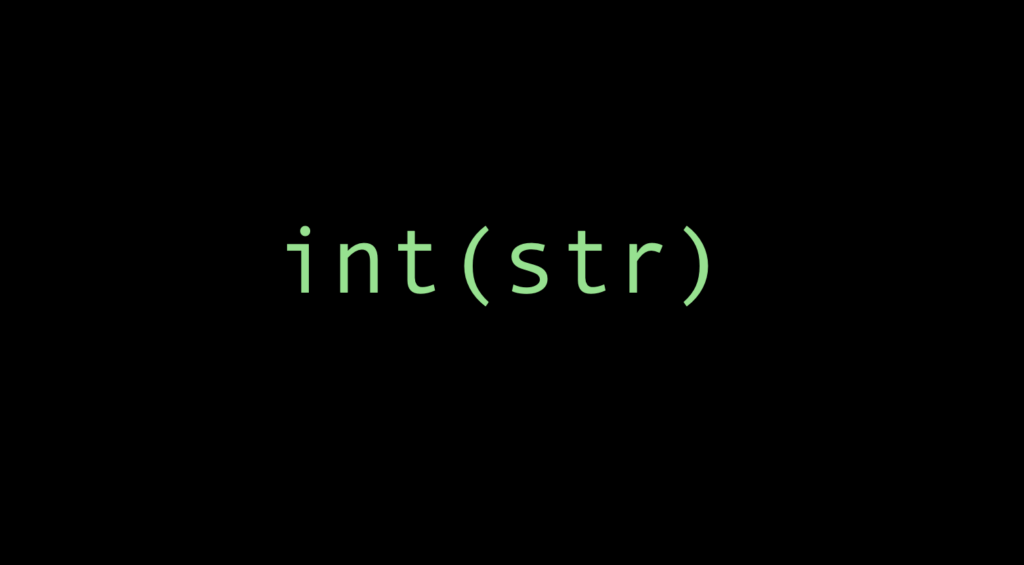
Converting string to int in Python is easy using the built-in int()
function.
For example, let’s convert the string “100” to an integer:
num_str = "100" num_int = int(num_str)
How to Convert Comma-Separated Number String to Int?
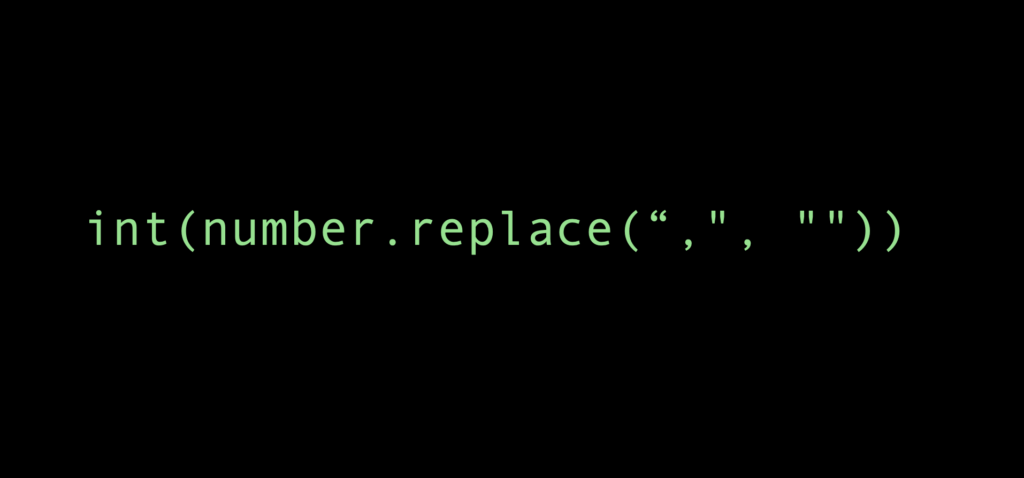
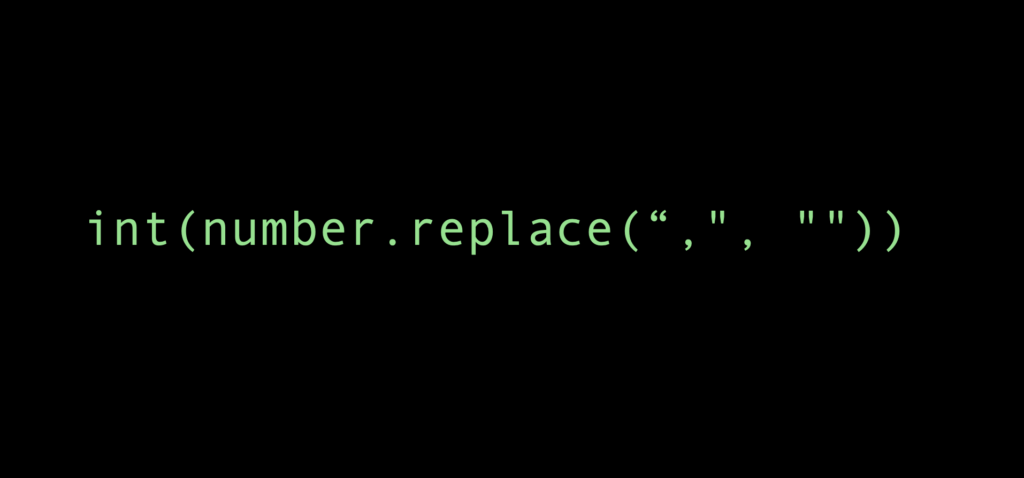
To convert a comma-separated number string (e.g. 1,000,000
) to an int in Python, first, get rid of the commas with the replace()
method. Then convert the result to an int using the int()
function:
big_num_str = "80,129,553" big_num_int = int(big_num_str.replace(",", "")) print(big_num_int)
Output:
80129553
How to Convert Number Strings in Different Bases
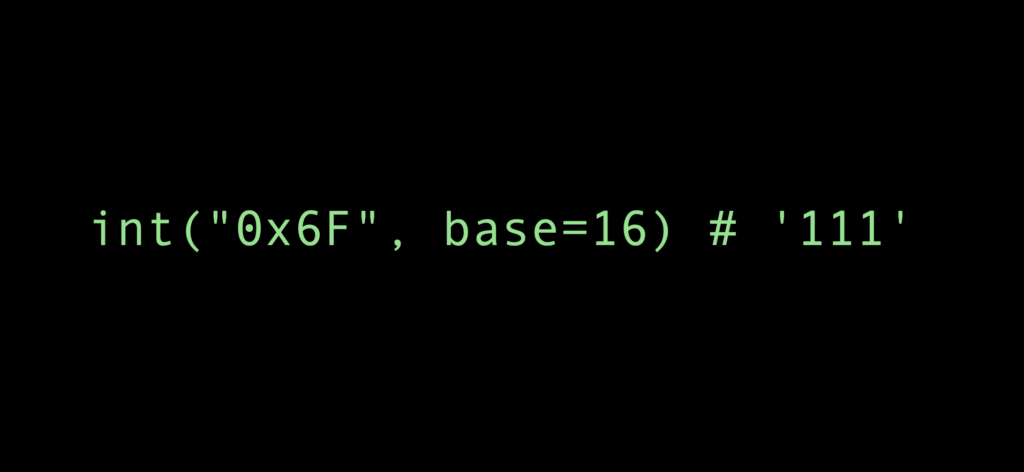
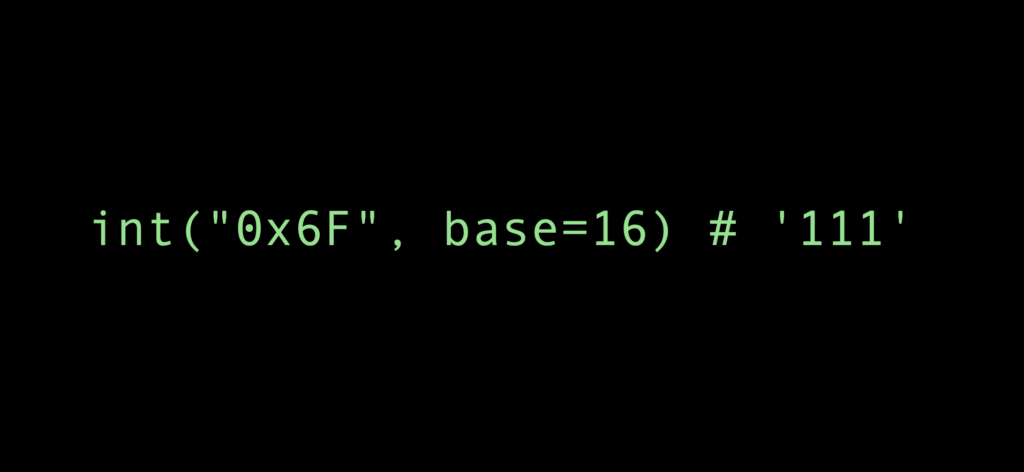
Using int()
to convert a string to an integer assumes that a string represents a decimal integer (a 10-base number).
String to Int in Different Base Systems
If you pass for example a hexadecimal string into the int()
function, you see an error:
int("0x6F")
Outcome:
ValueError: invalid literal for int() with base 10: '0x6F'
This ValueError
says that the string you tried to convert is not a valid decimal integer.
There is an easy way to fix this problem.
When you use the int()
function to convert a string to an int, you can specify the number system as the second argument. This happens with the base
attribute.
To convert a hexadecimal string to an int, let the int()
function know you’re dealing with a 16-base system:
int("0x6F", base=16) # '111'
You now know how to convert a string into an integer in Python. To make it complete, let’s turn things around and convert integers back to strings.
How to Convert Ints to Strings in Python
You can convert an integer to a string using the built-in str()
function.
num_int = 100 str(100) # '100'
This is the basic use case. But if you are dealing with other numeric systems, read along.
The str()
function is clever enough to tell that a binary literal is passed into it. For example, let’s convert a binary integer 0011
to a string that represents a regular integer:
bin = 0b0011 str(bin) # '3'
But if you want to represent an integer as a string in another number system, use the f-string formatting.
The number systems into which you can convert an integer are binary, hexadecimal, and octal.
Examples:
num = 100 num_binary_str = f"{num:b}" # '1100100' num_hexadecimal_str = f"{num:x}" # '64' num_octal_str = f"{num:o}" # '144'
Conclusion
Converting string to int in Python is easy with the built-in int()
function:
int("300") # 300
To convert an integer to a string, use the built-in str()
function.
str(23) # '23'
Thanks for reading. I hope you find it useful. Happy coding!