In Python, everything is an object. But each object represents some particular data type, such as an integer, string, or float. To check the type of an object in Python, use the built-in type() function.
Here are some examples of checking data types of different Python objects:
type(10) # --> <class 'int'> type("Test") # --> <class 'str'> type(['a', 'b', 'c']) # --> <class 'list'> type((1,2,3)) # --> <class 'tuple'> type({"temp": 30, "clouds": False}) # --> <class 'dict'> type(lambda x: x ** 2) # --> <class 'function'>
This quick yet comprehensive guide teaches you the perks of using the type() function in identifying data types in Python. As an interesting side application, you will also learn how to create a new data type with the type() function.
The type() Function in Python—Check the Type of an Object
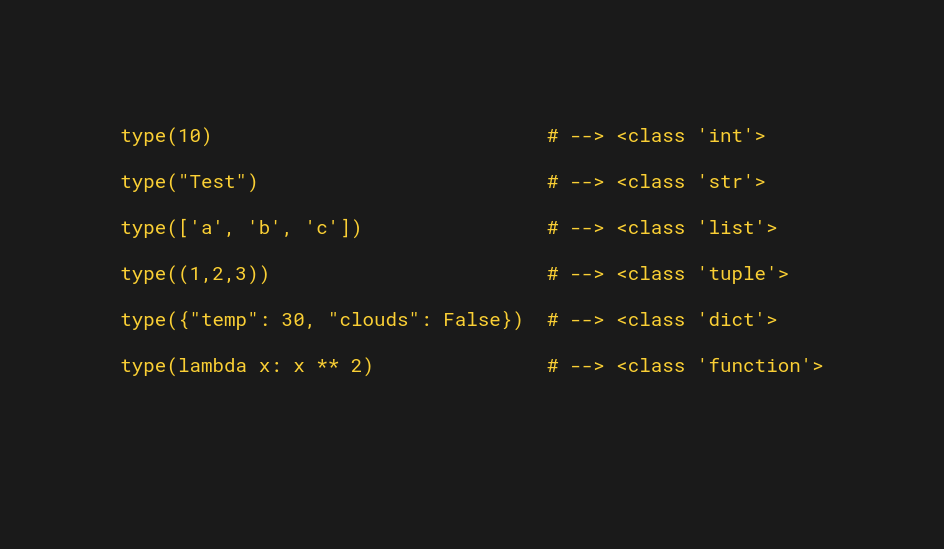
There are two ways you can use the built-in type function in Python:
- You can check the type of an object
- You can define a completely new custom type (or class). Notice, however, that this use case is very rare.
The first use case is the more popular one, so let’s see how it works first.
1. Use type() Function to Check the Type of an Object
To use the type() function for checking the type of a Python object, pass the object as an argument to it.
For example:
type(10) # --> <class 'int'>
Notice how you can use this result to make comparisons with other Python types in an easy way.
For example, let’s check if the type of an object is a string:
if type("test") == str: print("It is a string")
Output:
It is a string
2. Use type() Function to Define a Class
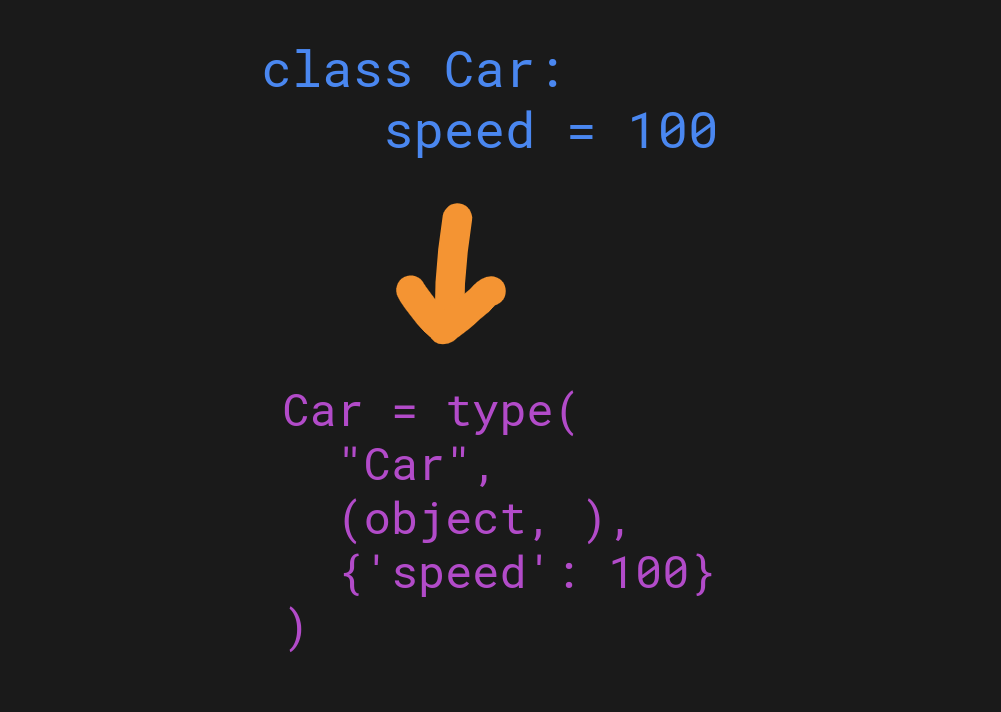
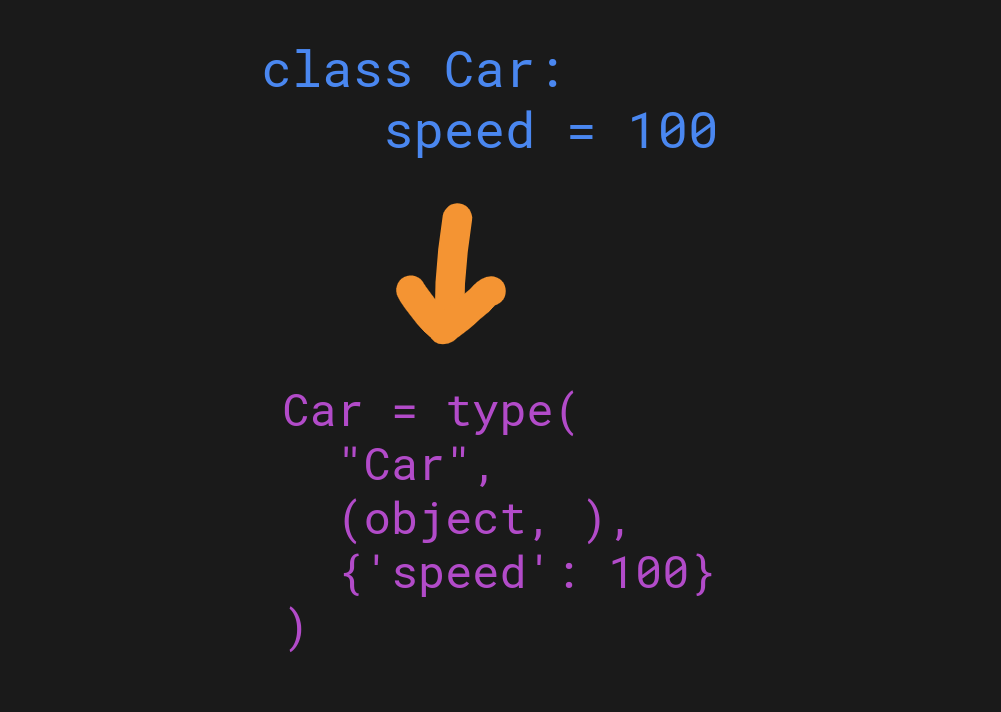
This use case for the type() function is something you will unlikely use. But in case you are interested, read along.
Let’s start by creating a basic Car class in Python:
class Car: speed = 100
There’s nothing interesting in this Car class. But did you know you can create the same class using the type() function?
To create a new class with the type() function, you need to pass it three parameters:
- A class name. This is just the name of the new class.
- Superclasses. This is a list or tuple with the superclasses of the new class. (It becomes the __bases__ attribute of the class under the hood.)
- Attributes dictionary. In this dictionary, you define the class attributes.
Using type() this way returns a new class you can use to create objects.
Let’s see an example by creating the same Car
class you saw above. But this time let’s use the type() function:
Car = type("Car", (object, ), {'speed': 100}) new_car = Car() print(new_car) print(new_car.speed)
Output:
100
This is actually what happens under the hood when you create a custom class yourself.
If you’re interested in learning more about metaprogramming classes, check out this article.
Conclusion
To check the type of a Python object, use the built-in type() function:
type(10) # --> <class 'int'> type("Test") # --> <class 'str'> type(['a', 'b', 'c']) # --> <class 'list'> type((1,2,3)) # --> <class 'tuple'> type({"temp": 30, "clouds": False}) # --> <class 'dict'> type(lambda x: x ** 2) # --> <class 'function'>
The type() function has another interesting but rare use case. You can define new classes with it.
For example, instead of traditionally creating a Car
class, you can use the type() function this way to do the same:
Car = type("Car", (object, ), {'speed': 100})
This creates a Car class with a single attribute speed, which is set to 100.
Thanks for reading. I hope you find it useful. Happy coding!