In Python, the dir()
function returns a list of the attributes and methods that belong to an object. This can be useful for exploring and discovering the capabilities of an object, as well as for debugging and testing.
For example, let’s list the attributes associated with a Python string:
# List the attributes and methods of a string dir('Hello')
Output:
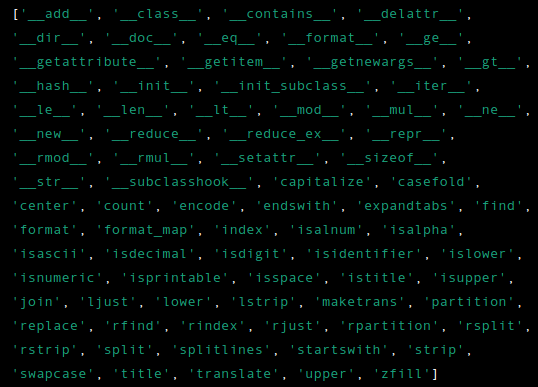
The result is a complete list of all the methods and attributes associated with the str data type in Python. If you’ve dealt with strings before, you might see methods that look familiar to you, such as split
, join
, or upper
.
This is a comprehensive guide to understanding what is the dir()
function in Python. You will learn how to call the function on any object and more importantly how to analyze the output. Besides, you’ll learn when you’ll most likely need the dir()
function.
What Is the dir() Function in Python?
The dir()
function in Python lists the attributes and methods of an object. It takes an object as an argument and returns a list of strings, which are the names of its attributes and methods. Using the dir()
function can be useful for inspecting objects to get a better understanding of what they do.
For example, you can use dir()
to list the attributes of a built-in data type like a list or a dictionary, or you can use it on a custom class to see what’s in it. Besides, you can explore a poorly documented module or library with the dir()
function.
Syntax and Parameters
The syntax of the dir()
function in Python is as follows:
dir(object)
The dir()
function takes a single parameter:
-
object
: This is the object or type whose attributes you’re interested in. This can be any type of object in Python, such as a built-in data type like a list or a dictionary, or a user-defined class.
The function returns a list of strings that represent the methods and attributes of that object.
Examples
Let’s call the dir()
function on a string ‘Hello’:
# List the attributes and methods of a string print(dir('Hello'))
Output:
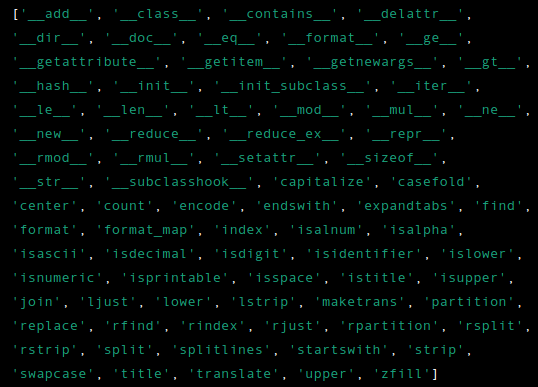
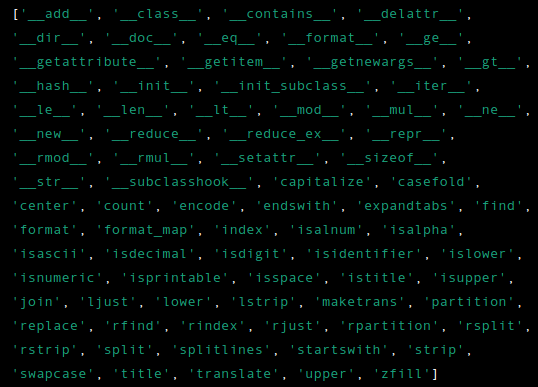
The above result is a list that has all the attributes and methods of a string object.
For example, the upper()
method can be used to convert a string to uppercase, and the find()
method can be used to search for a substring within a string. Both these methods belong to the string class and are thus present in the output of the dir()
function call.
Speaking of the variety of string methods in Python, make sure to read my complete guide to Strings in Python.
Notice that you can call the dir()
function on any object in Python, not just built-in types like string. In other words, you can list the attributes your custom class has. You’ll find more examples of this later on.
What Are the Double Underscore Methods (E.g. ‘__add__’)?
In the previous example, you saw a bunch of methods that start with __, such as __add__
, or __class__
.
The double underscore methods that appear in the outputs of the dir()
function are called “magic methods” or “dunder methods” in Python.
These methods are special methods that are defined by the Python language itself, and they are used to implement some of the built-in behavior of objects in Python.
For example, the __len__()
method is called when you use the len()
function on an object, and it returns the length of the object. The __add__()
method is called when you use the +
operator on two objects, and it returns the result of the operation.
Magic methods are sometimes considered to be an advanced concept of Python. You can use them to customize the behavior of custom objects. For example, you can tell what to do with your custom class object when calling + on them by specifying the __add__
method in the class.
The dunder methods are not meant to be called directly, but they are invoked automatically by the Python interpreter when certain operations are performed on an object.
Anyway, let’s go back to the topic.
Using dir() to Inspect Classes and Instances
The dir()
function is useful if you want to inspect the attributes of classes and their instances.
When you use dir()
with a class, lists the attributes defined in the class, including any inherited attributes and methods from the class’s superclasses.
In the earlier examples, you called the dir()
function on a Python string instance. But you can call it on any other class instance, including custom classes created by you. More importantly, you can call the dir()
function directly on a class instead of an instance of it.
Here’s an example of using dir()
to inspect the attributes and methods of a custom class you’ve just created:
class MyClass: def __init__(self, x, y): self.x = x self.y = y def my_method(self): return self.x + self.y print(dir(MyClass))
Output:
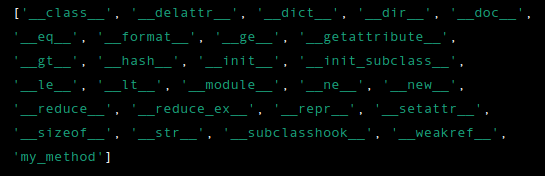
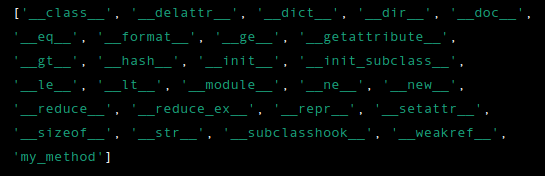
In this example, there’s a custom class called MyClass
that has an __init__()
method and a my_method()
method. Calling the dir()
function returns a big list of strings. These are all the attributes of the custom class MyClass
.
The very last name on the above list is ‘my_method
‘. This is the method we defined in MyClass
. But notice that the variables x
and y
aren’t there. This is because you called the dir()
function on the class, not on an instance of it. Because of this, the variables x
and y
aren’t technically there. Those instance variables are created upon instantiating the objects.
As another example, let’s call dir()
on a class instance instead of a class:
class MyClass: def __init__(self, x, y): self.x = x self.y = y def my_method(self): return self.x + self.y my_instance = MyClass(1, 2) print(dir(my_instance))
Output:
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'my_method', 'x', 'y']
Again, toward the end of the list, you can see the attribute my_method
. But this time, because you called the dir()
on an instance, you will also see the variables x
and y
.
When Use the dir() Function?
The dir()
function is useful in Python for finding out what attributes an object has. This is helpful when you are working with an object that you are not familiar with, or when you just want to see all of the available methods of an object.
Another common use for the dir()
function is to find out what attributes and methods are available in a particular module or package.
For example, to see what attributes and methods are available in the math
module, call dir()
on it:
import math print(dir(math))
This prints out all the attributes and methods that are available in the math
module. It’s helpful for quickly finding out what functions and other objects are available in the module, and might save you time when you are working with a module that you are not familiar with. This is especially true if the module is poorly documented (unlike the math
module, though)
Summary
In conclusion, the dir()
function is a valuable tool in Python for finding out what attributes and methods are available for a given object. It can be used to quickly explore new objects and modules, and can save time when you are working with an object or module that you are not familiar with.
Thanks for reading. Happy coding!