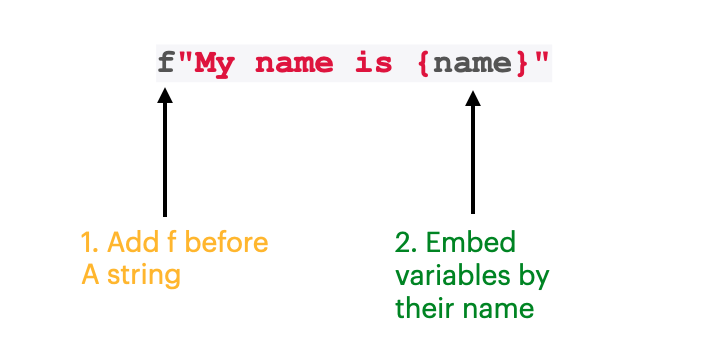
Formatted string or F-string in Python makes it possible to insert variables into strings in a handy and readable way.
For example:
name = "Nick" print(f"My name is {name}")
Output:
My name is Nick
Let’s compare this to the old-school approach using the format() method:
name = "Nick" print("My name is {}".format(name))
The f-string is more convenient especially when the number of embedded variables is big.
This is a comprehensive guide to using F-strings in Python. This guide teaches you the basics for modern string formatting in Python using F-strings. You will also see what problem the F-strings solve and what were the earlier ways to string formatting.
How to Format Strings in Python—The Old Way
Since the release of Python 2.6, you have used the format() method to embed variables into strings.
As an example:
name = "Nick" print("My name is {}".format(name))
Output:
My name is Nick
This approach is pretty straightforward and thus easy to understand. However, if you increase the number of variables to embed the code’s complexity increases quite a bit.
For example, let’s embed four strings into a string using the format() method:
first_name = "Nick" last_name = "Jones" profession = "Software Engineer" platform = "Codingem" print("Hi! I am {first_name} {last_name}, a {profession}. I'm writing a new article on{platform}.".format(first_name= first_name, last_name =last_name , profession = profession, platform = platform))
Output:
Hi! I am Nick Jones, a Software Engineer. I'm writing a new article on Codingem.com.
As you can see, the print() function call is ridiculously long.
This is the main problem when using the format() method in Python. Since the Python 3.6 release, using format() is no longer needed. Now there is a way more elegant solution called the F-strings.
Let’s take a look at how F-strings help you make string formatting easier and cleaner.
F-Strings in Python
Due to the messy string formatting with the format() method, Python 3.6 comes with a clean way to format strings called F-strings.
Using an F-string in Python is easy:
- Add character f in front of a string.
- Inside the string add any number of variables wrapped around curly braces {}.
For example:
name = "Nick" print(f"My name is {name}")
Output:
My name is Nick
This makes the code look way nicer and it is easier for us developers to read.
Now adding multiple variables does not mess things up that bad anymore:
first_name = "Nick" last_name = "Jones" profession = "Software Engineer" platform = "Codingem.com" print(f"Hi! I am {first_name} {last_name}, a {profession}. I'm writing a new article on {platform}.")
Output:
Hi! I am Nick Jones, a Software Engineer. I'm writing a new article on Codingem.com.
Looks really good!
Now you know how to use F-strings in Python to solve the problem of formatting strings.
Next, let’s take a look at some commonly used formatting options related to F-strings.
Arbitrary Expressions and Formatted Strings
You can add any valid Python expression inside an F-string.
For example, let’s embed a result of a calculation into a string using an F-string:
print(f"The result of 3*6 is {3*6}")
Output:
The result of 3*6 is 18
Multi-Line Formatted Strings in Python
Python line length should not exceed 79 characters according to the PEP8 style guide.
Sometimes when you write a lengthy string, it can exceed this limit. If this is the case, you can break the string down into multiple lines and keep the output in a single line.
To do this:
- Break string into multiple lines.
- Convert each line to a separate F-string.
For example:
first_name = "Nick" last_name = "Jones" profession = "Software Engineer" platform = "Codingem" message = ( f"Hi! I am {first_name} {last_name}." f"I'm a {profession}." f"I'm writing a new article on{platform}." ) print(message)
Output:
Hi! I am Nick Jones, a Software Engineer. I'm writing a new article on Codingem.com.
F-String Table in Python
You can specify the number of spaces taken by each variable inside your F-string.
To do this, add a :{} after the variable name inside the F-string, and specify the number of spaces taken inside the curly braces.
This can be useful for example if you want to format your data to look like a table.
For instance:
data = [("x", "y", "sum"), (1, 2, 3), (3, 5, 8)] for x, y, sum in data: print(f"{x:{1}} {y:{1}} {sum:{2}}")
Result:
x y sum 1 2 3 3 5 8
F-String Decimal Places in Python
To specify the desired precision of a floating-point number, use a colon (:) followed by the number of digits you want to see in the output.
For instance, let’s print a float with 2 decimal accuracy using an F-string:
f_num = 12.3241233 print(f"The number is: {f_num:.2f}")
Output:
The number is: 12.32
Conclusion
Today you learned how to use F-strings in Python as a new way to format strings.
To recap, formatted string or F-string in Python is a cleaner way to insert variables into strings.
As an example:
name = "Nick" print(f"My name is {name}")
The benefit of F-strings over the format() becomes clear when dealing with multiple variables: Using format() makes the code look ugly. With F-strings you can write the variable exactly where you want it to appear.
Thanks for reading. I hope you find it useful.
Happy coding!