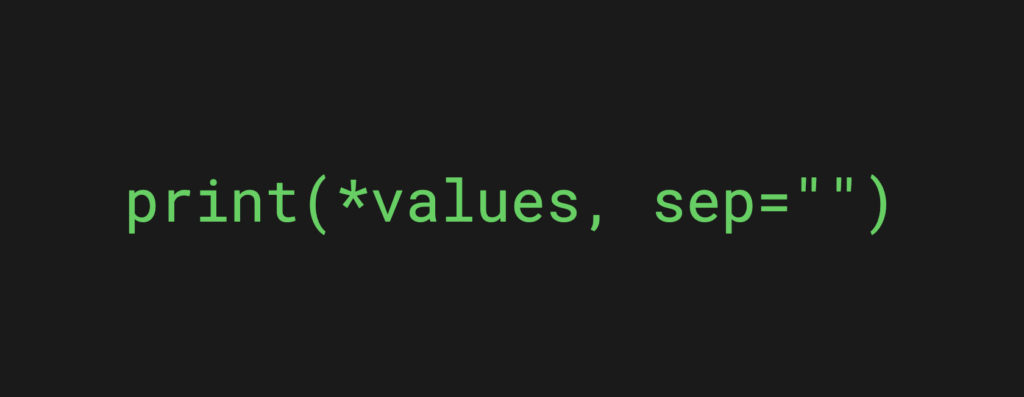
To print without a space in Python, you can set the sep
parameter to specify a separator between the values you are printing.
For example, to print a list of numbers without any spaces between them, you could use the following code:
numbers = [1, 2, 3, 4, 5] # Use sep to specify a separator of "" (an empty string) print(*numbers, sep="")
This will print the numbers 12345
without any spaces between them.
You can use the sep
parameter with any value you want, not just an empty string.
For example, you could use the sep
parameter to print the numbers separated by commas instead of spaces, like this:
# Use sep to specify a separator of "," print(*numbers, sep=",")
This will print the numbers 1,2,3,4,5
with commas between them instead of spaces.
If you’re looking for a quick solution, I’m sure the above example will do. But to learn more situations and ways to separate strings without spaces, feel free to read along!
3 Ways to Print without a String
You can use F-strings, string concatenation (+ operator), and str.format()
methods to print values without spaces in Python. This section shows you how these three approaches work and how you can use them to print values without spaces.
1. Use F-Strings to Print without Spaces
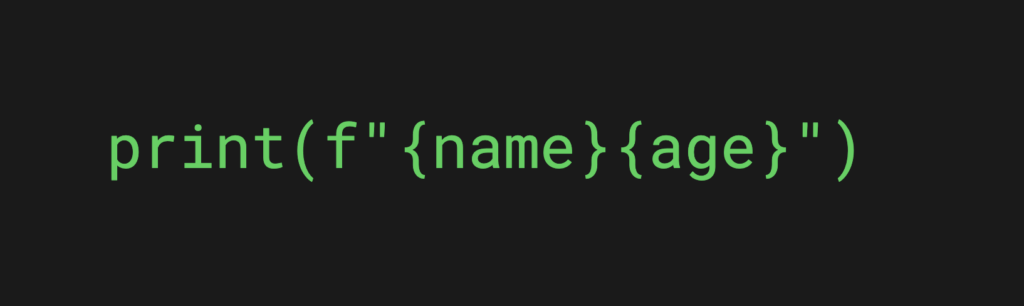
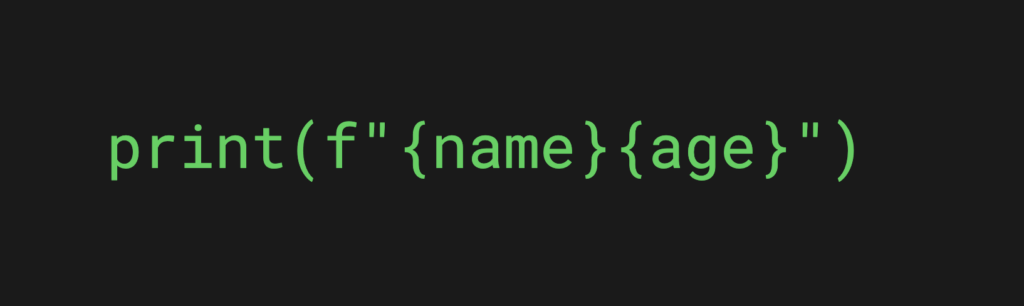
In Python, f-strings (formatted string literals) are a way to include the value of a variable inside a string. They are introduced in Python 3.6 and are denoted by prefixing the string with the letter f
or F
.
Here’s an example of how to use f-strings to print values without a space:
name = "John" age = 34 # Print the values without a space using an f-string print(f"{name}{age}") # Output: John34
In this example, the f
prefix indicates that the string is an f-string, and the curly braces {}
are used to include the value of the name
and age
variables in the string.
Since there is no space between the curly braces, the values are printed without a space.
John34
Make sure to read my complete guide to F-Strings in Python.
2. String Concatenation
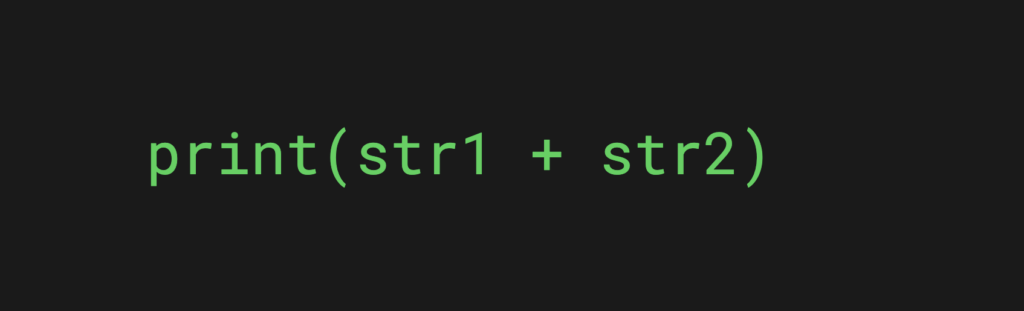
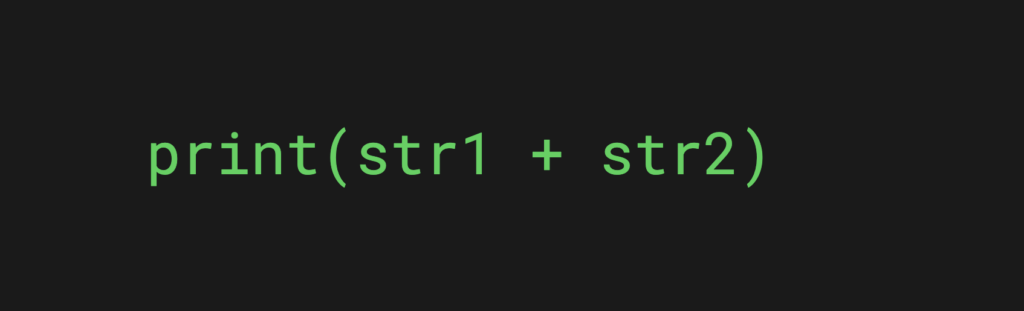
To print values without a space using string concatenation, we can simply concatenate the values together without adding a space in between them.
For example:
first_name = "John" last_name = "Smith" print(first_name + last_name)
Output:
JohnSmith
String concatenation is the process of joining two or more strings together to form a new string. In Python, this is done using the “+” operator.
For example, the code above uses concatenation to combine the “first_name” and “last_name” variables into a single string without a space.
3. str.format() Method
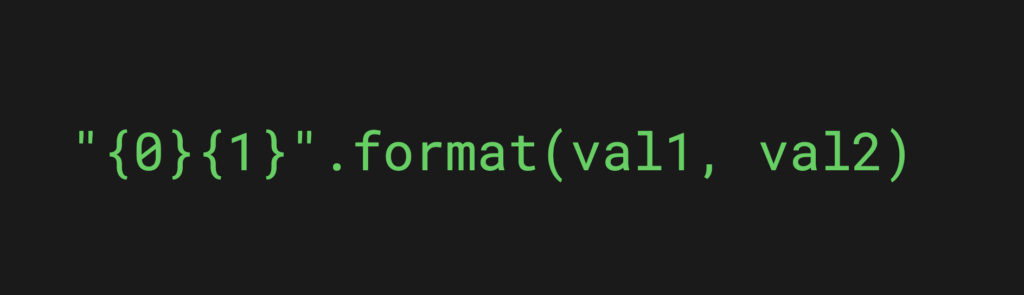
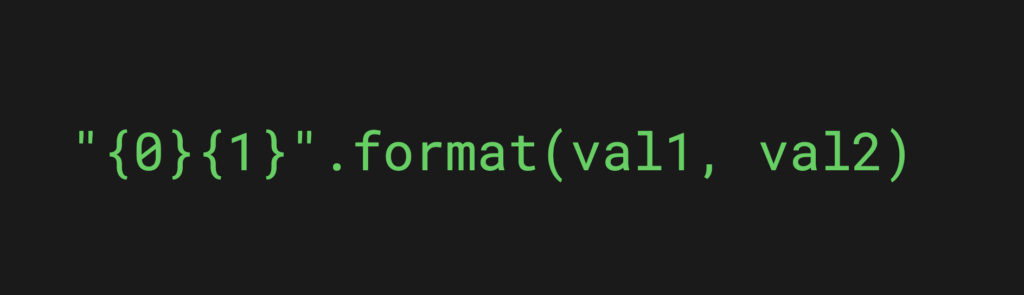
Here is an example of how to use the str.format()
method to print values without spaces in Python:
# Define a string with placeholders for values string = "The quick {0}{1} over the lazy {2}." # Use the str.format() method to replace the placeholders with values # without adding any spaces print(string.format("brown", "fox", "dog"))
Output:
The quick brownfox over the lazy dog.
Notice that the values inserted into the string using the str.format()
method are not separated by any spaces. This is because we did not include any spaces in the format string.
If we want to add spaces between the values, we can simply include spaces in the format string, like this:
# Define a string with placeholders for values and include spaces in the format string string = "The quick {0} {1} over the lazy {2}." # Use the str.format() method to replace the placeholders with values # and include the spaces defined in the format string print(string.format("brown", "fox", "dog"))
Output:
The quick brown fox over the lazy dog.
Finally, let’s take a closer look at the sep
parameter you learned earlier in this guide.
The ‘sep’ Parameter in print() Function
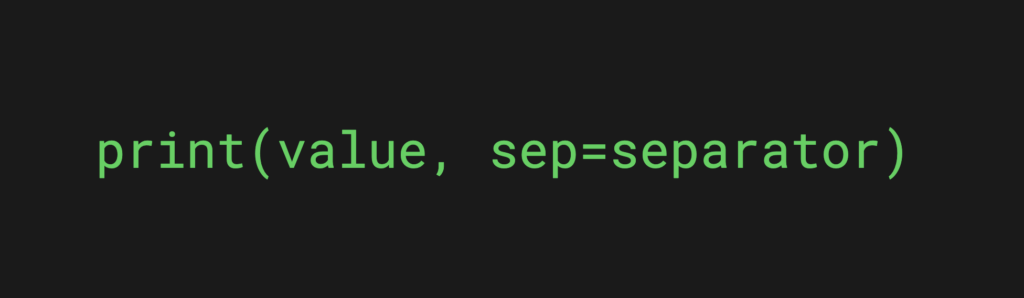
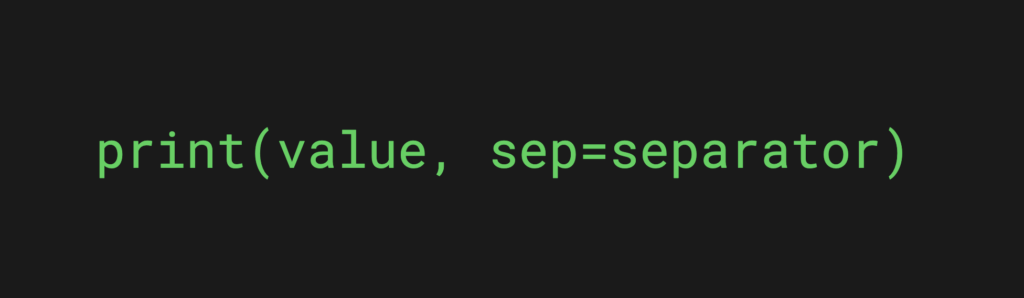
In Python, the sep
parameter is used to specify the string that should be placed between the values being printed.
By default, the print()
function separates the values it prints with a single space.
However, you can use the sep
parameter to specify a different string to be used as the separator.
For example, if you want to print a list of numbers with a comma between each one, you could use the following code:
numbers = [1, 2, 3, 4, 5] print(*numbers, sep=', ')
This would print the following output:
1, 2, 3, 4, 5
The sep
parameter can be used with any type of value that you want to print, not just lists. You can use it to separate strings, numbers, or any other type of value.
If you want to omit the white space between values being printed by the print()
function, you can set the sep
parameter to an empty string. This will prevent the print()
function from adding any space between the values it prints.
For example, the following code will print the numbers 1, 2, 3, 4, and 5 on the same line without any white space between them:
numbers = [1, 2, 3, 4, 5] print(*numbers, sep='')
Output:
12345
Summary
Today you learned how to print values without spaces in Python.
The sep
parameter in the Python print()
function is used to specify the string that should be placed between the values being printed.
By default, this parameter is set to a single space, which means that the print()
function will add a single space between the values it prints.
However, you can use the sep
parameter to specify a different string to be used as the separator.
For example, you could use the sep
parameter to specify a comma and a space as the separator, or you could set it to an empty string to omit the white space between the values being printed.
Overall, the sep
parameter provides a way to customize the formatting of the output generated by the print()
function.
Thanks for reading. Happy coding!