A nested loop in Python is a loop that has loops inside it.
Here’s a generic pattern:
for element in sequence1: for element in sequence2: # inner loop body here # outer loop body here
Even though there’s nothing special about nested loops, as a beginner, they might seem a bit scary or verbose.
This is a comprehensive guide to nested loops in Python.
Theory
The term “nested” is commonly used in programming. If you have attended any Python Certification training, you might have noticed that these concepts are typically covered because they are fundamental to writing efficient and effective Python code, especially in areas like data science, web development, and automation, where handling complex data and control flow is common.
By definition, the word “nested” means having an object placed inside another object of the same kind.
In Python, you can place any valid code inside a loop. It could even be another loop.
A loop that lives inside a loop is called a nested loop. Notice that a nested loop is not a Python-only concept. Nested loops are present in all other programming languages, so the term is good to learn!
How Does a Nested Loop Work in Python?
There’s no limit as to how many loops you can place inside a loop.
To demonstrate how a nested loop works, let’s describe a nested loop of two loops: An outer loop and an inner loop.
Here’s what the generic syntax of a nested for loop looks like:
for element in sequence1: for element in sequence2: # inner loop body here # outer loop body here
Every iteration of the outer loop causes the inner loop to run all its iterations. The outer loop doesn’t run before the inner loop has terminated.
To clarify: Nested loops are not about for loops only. You can place while loops inside while loops, while loops inside for loops, and more. A nested loop is a loop that has at least one loop inside of it.
A typical scenario for using a nested loop is when working with multi-dimensional data, such as lists of lists or such.
Let’s see some simple examples of nested loops.
Example 1. Nested For Loops
Let’s use a nested for loop to print the multiplication table of the first 10 numbers.
for i in range(1, 11): for j in range(1, 11): print(i * j, end=" ") print()
Output:
1 2 3 4 5 6 7 8 9 10 2 4 6 8 10 12 14 16 18 20 3 6 9 12 15 18 21 24 27 30 4 8 12 16 20 24 28 32 36 40 5 10 15 20 25 30 35 40 45 50 6 12 18 24 30 36 42 48 54 60 7 14 21 28 35 42 49 56 63 70 8 16 24 32 40 48 56 64 72 80 9 18 27 36 45 54 63 72 81 90 10 20 30 40 50 60 70 80 90 100
Let’s take a closer look at how this program works:
- The outer loop iterates numbers from 1 to 10 and stores the current number in a temporary variable i. So in the first iteration, i = 1, on the second, i = 2, and so on.
- The inner loop also iterates numbers from 1 to 10. The inner loop stores the current iteration number in a variable j.
- For every single outer loop iteration, the inner loop will run entirely, that is, 10 times.
- So for example, during the first iteration of the outer loop, i = 1 while j goes from 1 to 10. During the second iteration, i = 2, and j goes from 1 to 10 again, and so on.
- The result is a multiplication table where each number 1…10 gets multiplied by 1…10.
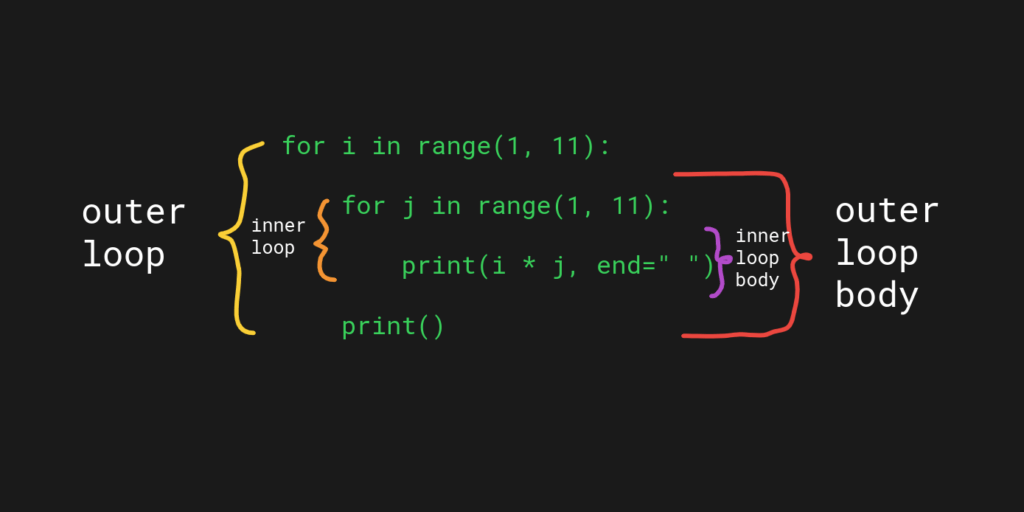
By the way, if you want to format the multiplication nicely so that the numbers align in each column, you can convert the multiplication result to a string and use the str.ljust() method to force the width to be the same.
Here’s how it looks in the code:
for i in range(1, 11): for j in range(1, 11): print(f"{i * j}".ljust(3), end=" ") print()
Output:
1 2 3 4 5 6 7 8 9 10 2 4 6 8 10 12 14 16 18 20 3 6 9 12 15 18 21 24 27 30 4 8 12 16 20 24 28 32 36 40 5 10 15 20 25 30 35 40 45 50 6 12 18 24 30 36 42 48 54 60 7 14 21 28 35 42 49 56 63 70 8 16 24 32 40 48 56 64 72 80 9 18 27 36 45 54 63 72 81 90 10 20 30 40 50 60 70 80 90 100
Example 2. Nested While Loops
For the sake of completeness, let’s repeat the above example by using a nested while loop. The point of this example is to demonstrate you can also place a while loop inside another.
Here’s the code:
i = 1 j = 1 while i <= 10: while j <= 10: print(i * j, end = " ") j += 1 j = 1 print() i += 1
Output:
1 2 3 4 5 6 7 8 9 10 2 4 6 8 10 12 14 16 18 20 3 6 9 12 15 18 21 24 27 30 4 8 12 16 20 24 28 32 36 40 5 10 15 20 25 30 35 40 45 50 6 12 18 24 30 36 42 48 54 60 7 14 21 28 35 42 49 56 63 70 8 16 24 32 40 48 56 64 72 80 9 18 27 36 45 54 63 72 81 90 10 20 30 40 50 60 70 80 90 100
Here’s an illustration of the parts of the nested while loop above:
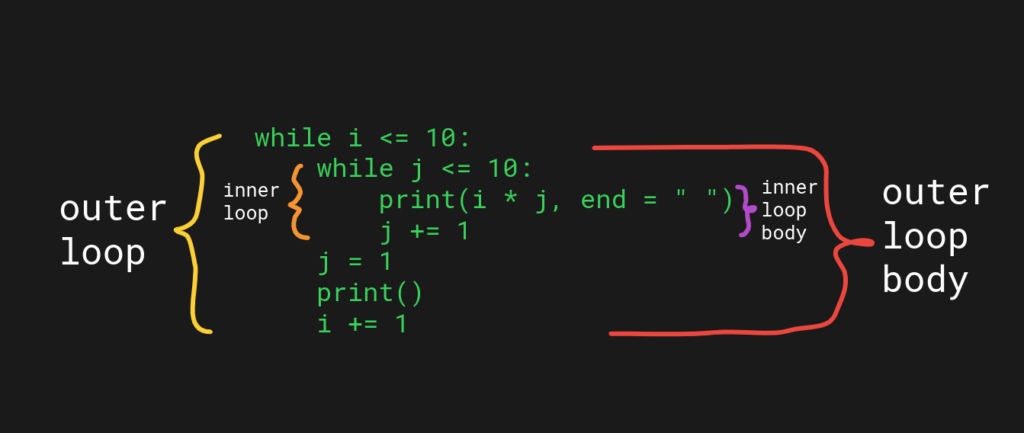
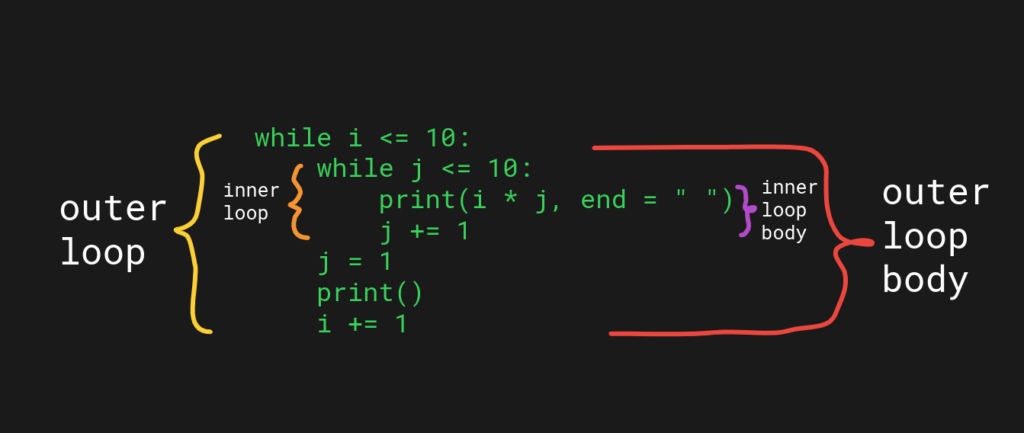
How to Control the Flow of a Nested Loop?
In Python, you can control the flow or a loop. The two main control flow statements in Python are:
- break. The break statement exits the loop.
- continue. The continue statement terminates the current iteration of the loop and jumps into the next one.
Let’s take a closer look at how these statements work with the nested loops in Python.
How to Break a Nested Loop?
In Python, you can use the break statement to exit a loop. This is useful if there’s no reason to continue looping. For example, if you’re using a loop to find a target value, once the target is found, the loop should stop.
Let’s see a simple example of the break statement in action:
target = 3 for number in range(10): print(number) if number == target: break
Output:
0 1 2 3
Here the loop terminates when the iteration number is 3, which is the target number.
In a nested loop, the break statement exits the inner loop but continues running the outer loop. So if you call the break statement in the inner loop of a nested loop construct, it doesn’t stop the entire loop but just the inner one.
For instance, let’s print a half-pyramid of asterisks such that the number of asterisks is the row number in the shape read from top to bottom:
for i in range(1, 11): for j in range(1, 11): if j - i > 0: break print("*", end=" ") print()
Output:
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
In a nested loop like this, you can think of i as the row number and j as the column number.
Here the break statement exits the inner loop if j – i is less than 0. In other words, you stop running the inner loop if the number of stars (*) is greater than the row number.
Read more about controlling the flow of a loop using the break statement.
How to Continue a Nested Loop?
In Python, the continue statement lets you skip “the rest” of the iteration of a loop and start the next iteration.
This statement doesn’t terminate the entire loop but only the current iteration. The continue statement is useful if there’s no reason to continue the current iteration of the loop. For example, if you only want to do actions based on a condition, you can use the continue statement to skip the actions.
You can use the continue statement in a nested loop just like you would use it on a single loop.
- If you call the continue statement in the inner loop, the current iteration stops, and the next iteration of the inner loop begins.
- If you call the continue statement before the inner loop in the outer loop body, then the iteration of the outer loop jumps to the next iteration by skipping the inner loop.
For instance, let’s print an inverted half-pyramid of asterisks such that the number of asterisks is the row number in the shape read from bottom to top:
for i in range(1, 11): for j in range(1, 11): if i + j > 11: continue print("*", end=" ") print()
Output:
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
Here the continue statement jumps the inner loop to the next iteration if the i + j is more than 11. In other words, the inner loop skips printing asterisks (*) if the row number plus the column number is more than 11.
Anyway, the point is to demonstrate how the continue statement works in a nested loop rather than focusing on the details of the shape. Feel free to play with the example in your code editor, though!
One-Liner Nested Loops with List Comprehensions
In Python, you can make the for loops more compact by using list comprehensions. This allows for a neat one-liner expression rather than having the loop expand on multiple code lines.
Similar to how you can create a nested for loop, you can create a nested list comprehension.
It’s up to debate whether it makes sense to compress for loops using comprehensions. Some argue it makes the code more compact and read easier. Others say it just makes the code more complex. If you create a nested list comprehension, be careful. The code quality might suffer due to a verbose and unreadable expression. Make sure to only do list comprehensions if it improves the code quality!
mul_tab = [[i * j for in range(1, 11)] for j in range(1, 11)] for row in mul_tab: print(row)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10] [2, 4, 6, 8, 10, 12, 14, 16, 18, 20] [3, 6, 9, 12, 15, 18, 21, 24, 27, 30] [4, 8, 12, 16, 20, 24, 28, 32, 36, 40] [5, 10, 15, 20, 25, 30, 35, 40, 45, 50] [6, 12, 18, 24, 30, 36, 42, 48, 54, 60] [7, 14, 21, 28, 35, 42, 49, 56, 63, 70] [8, 16, 24, 32, 40, 48, 56, 64, 72, 80] [9, 18, 27, 36, 45, 54, 63, 72, 81, 90] [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
How to Turn a Nested For Loop into a List Comprehension?
In the previous section, you saw an example of creating a nested list comprehension from a nested for loop. But from the above example, it’s unclear how you actually do it.
Here’s the blueprint for creating nested list comprehensions from nested for loops:
[expression for outer_loop for inner_loop]
The order of the comprehension might confuse you because the outer loop happens in the “inner part” of the comprehension and the inner loop is on the “outer part”. Other than that, there’s not much going on in the conversion.
If you’re unfamiliar with list comprehensions, though, you should read a separate article about the topic!
For example, here’s an illustration that converts a nested for loop into a nested list comprehension.
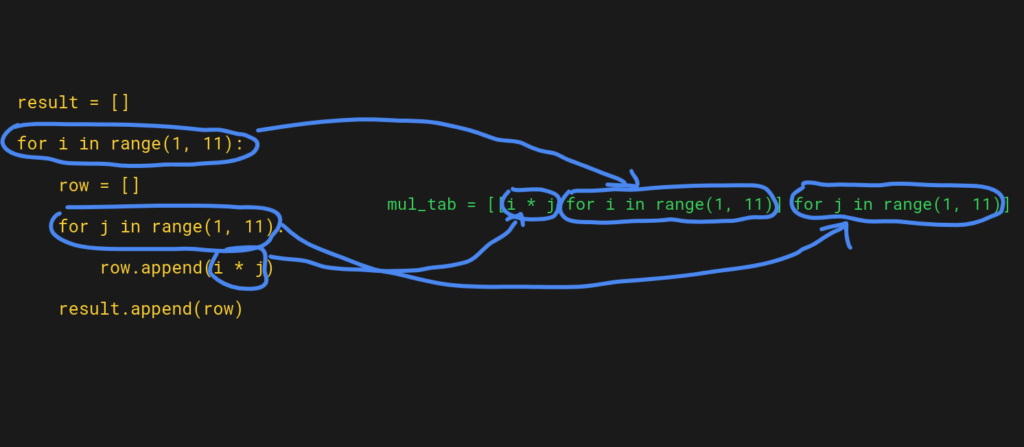
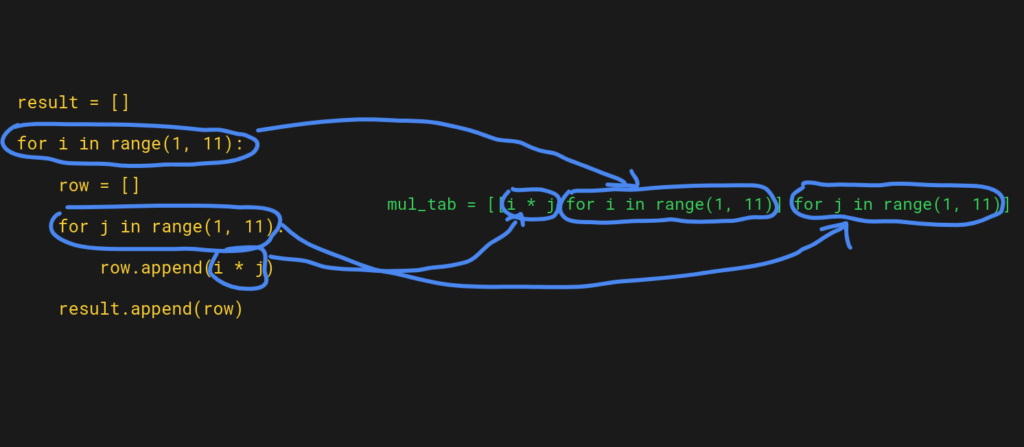
Summary
Today you learned how nested loops work in Python.
To take home, a nested loop refers to a loop inside a loop. There can be as many loops in a loop as you want.
Nested loops are usually practical when working with multi-dimensional data, such as lists of lists, or data tables.
As a shorthand, you can compress nested for loops to nested list comprehensions. Be careful when doing this not to make the code harder to read.
Thanks for reading. Happy coding!