To check if an object has an attribute in Python, you can use the hasattr()
function.
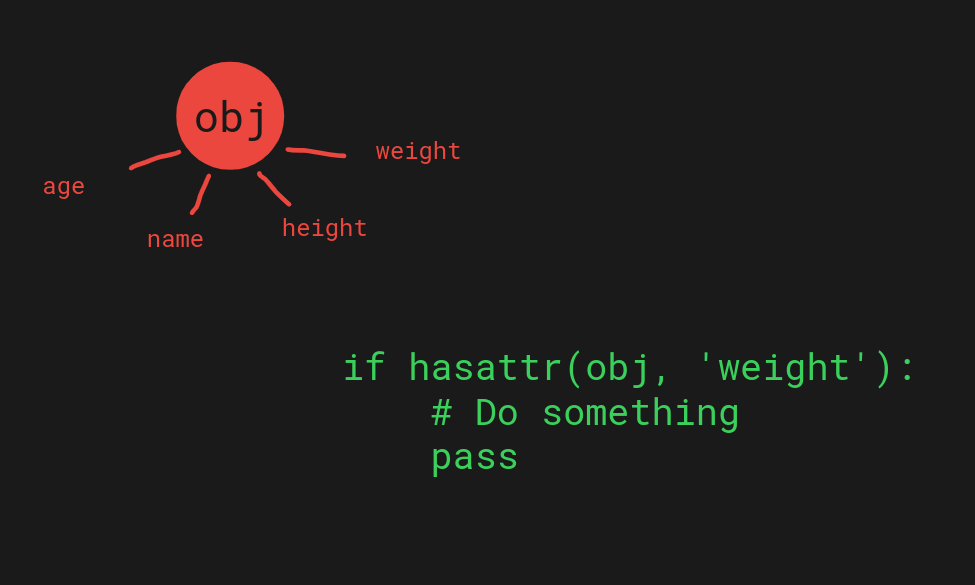
This function takes two arguments: the object that you want to check, and the name of the attribute that you are looking for.
For example:
class MyClass: def __init__(self): self.attr = 5 obj = MyClass() # Check if obj has the attribute 'attr' if hasattr(obj, 'attr'): # Do something pass
In this example, hasattr(obj, 'attr')
will return True
, because obj
has the attribute attr
. If obj
did not have the attribute attr
, hasattr(obj, 'attr')
would return False
.
You can also use the getattr()
function to check if an object has an attribute in Python.
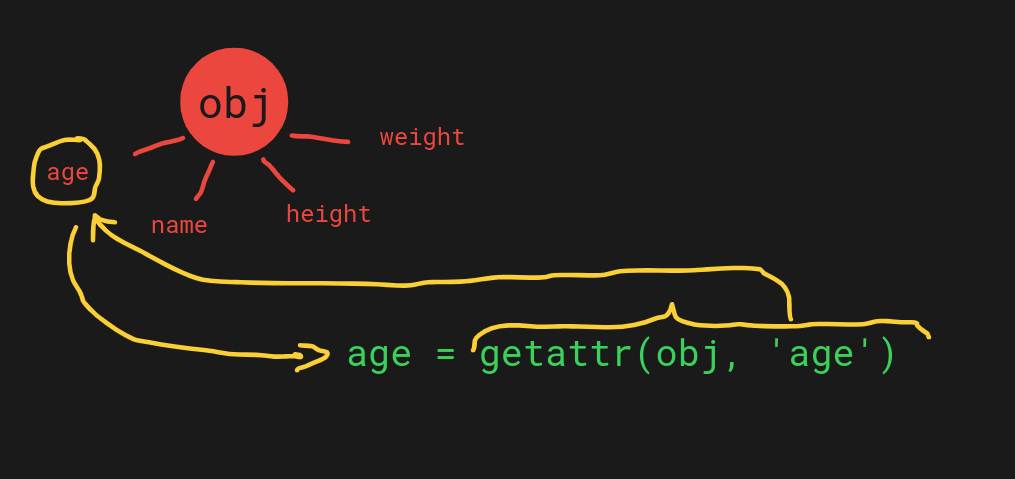
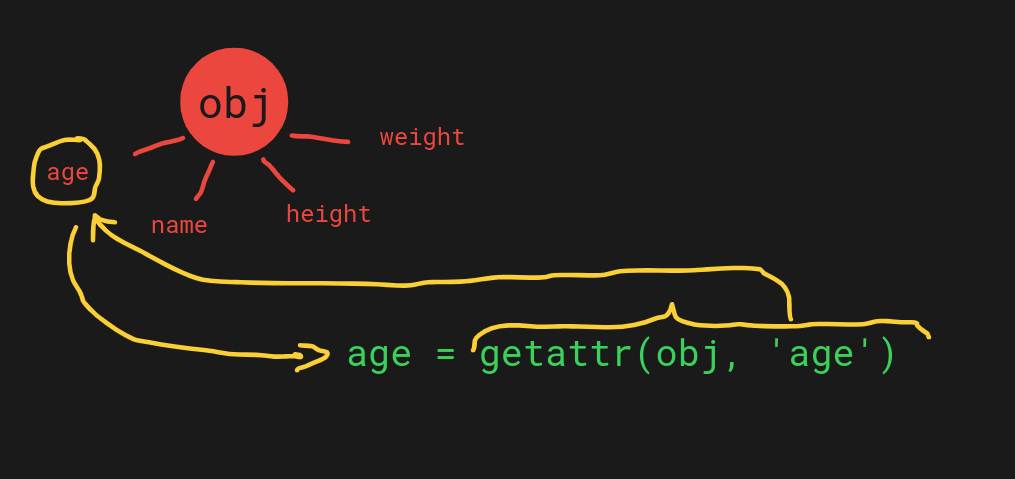
The getattr()
function takes three arguments: the object that you want to check, the name of the attribute that you are looking for, and a default value to return if the attribute does not exist.
For example:
class MyClass: def __init__(self): self.attr = 5 obj = MyClass() # Check if obj has the attribute 'attr' value = getattr(obj, 'attr', None) if value is not None: # Do something pass
By the way, make sure to check the AI code generator. This kind of tool can solve coding problems much more easily than reading articles.
How to List All Attributes of an Object
To list all the attributes of a Python object, you can use the dir()
function. It takes an object as its argument and returns a list of all the attributes and methods of the object.
For example, let’s list all the attributes of our example class:
class MyClass: def __init__(self): self.attr = 5 def my_method(self): pass obj = MyClass() # List all the attributes and methods of obj attributes = dir(obj) print(attributes)
In this example, dir(obj)
will return a list of all the attributes and methods of obj
. The output of this code will be something like this:
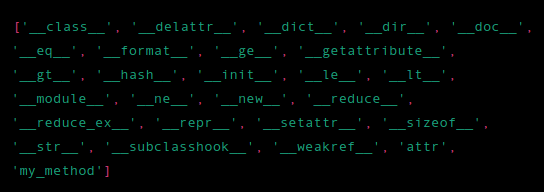
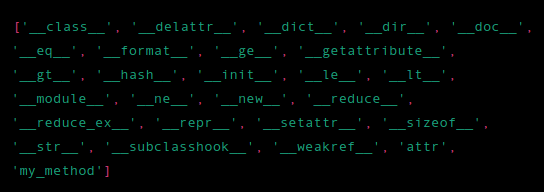
These are the default attributes that are associated with each class in Python. But if you pay close attention, the very last attribute of the list is called ‘my_method
‘. This is the method that you specified in the MyClass
example class.
How to List the Instantiated Object Attribute Values
To list all the attribute values of an instantiated object in Python, you can use the vars()
function. This function takes an object as its argument and returns a dictionary of all the attributes and their corresponding values for that object.
For example:
class MyClass: def __init__(self): self.attr1 = 5 self.attr2 = 'hello' obj = MyClass() # List all the attribute values of obj attributes = vars(obj) print(attributes)
In this example, vars(obj)
will return a dictionary of all the attributes and their corresponding values for the obj
object.
{'attr1': 5, 'attr2': 'hello'}
Notice how this function does not include the double underscore method that the dir()
function from the previous example did.
Thanks for reading. Happy coding!