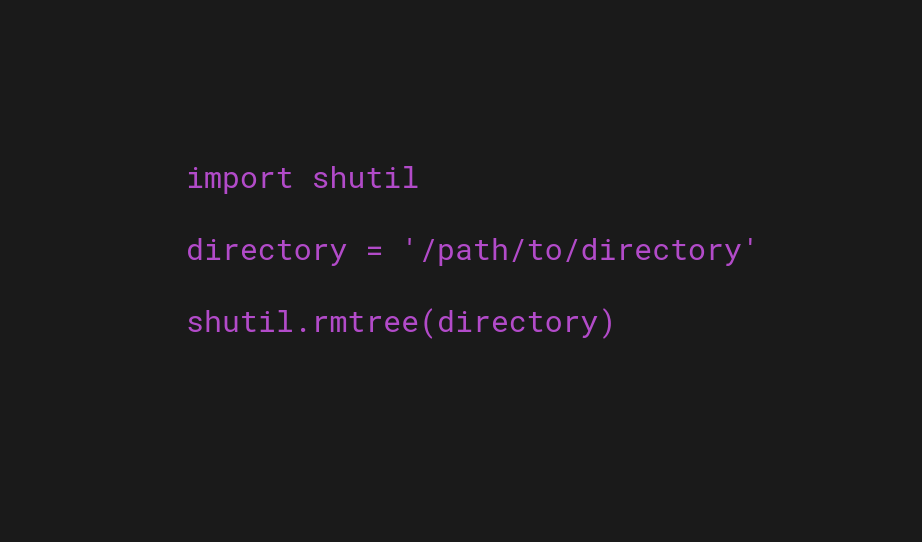
To remove a non-empty folder in Python, you can use the shutil
module, which provides functions for copying and removing files and directories.
ATTENTION! This guide shows you how to permanently delete the files and directories. Make sure you’ve crafted the file paths so that you’re 100% sure to delete the directories you want to delete.
To remove a directory and all its contents, including any subdirectories and files, you can use the rmtree
function.
For example:
import shutil # Replace with the path to the directory you want to remove directory = '/path/to/directory' shutil.rmtree(directory)
This will remove the directory and all its contents, including any subdirectories and files. Notice that this approach does not delete read-only files! Instead, you’re going to see an error.
Let’s have a look at some alternative approaches to deleting non-empty folders with Python.
Alternative Approaches
1. The ‘os.walk’ Function
To remove a non-empty folder in Python, you can delete the files individually using the os.walk
function.
Here’s what it looks like in the code:
import os # Replace with the path to the directory you want to remove directory = '/path/to/directory' # Use os.walk to traverse the directory tree for root, dirs, files in os.walk(directory): # For each file in the directory for file in files: # Construct the full path to the file file_path = os.path.join(root, file) # Delete the file os.remove(file_path) # For each subdirectory in the directory for dir in dirs: # Construct the full path to the subdirectory dir_path = os.path.join(root, dir) # Delete the subdirectory os.rmdir(dir_path) # Delete the top-level directory os.rmdir(directory)
This code uses the os.walk
function to traverse the directory tree and delete each file and subdirectory within the specified directory. Once all the files and subdirectories have been deleted, the top-level directory is also deleted.
2. pathlib Module
To remove a non-empty folder in Python using the pathlib
module, you can use the following code:
import pathlib # A recursive function to remove the folder def del_folder(path): for sub in path.iterdir(): if sub.is_dir(): # Delete directory if it's a subdirectory del_folder(sub) else : # Delete file if it is a file: sub.unlink() # This removes the top-level folder: path.rmdir() # Example use del_folder(pathlib.Path('path/to/folder'))
This code uses the iterdir
method to traverse the directory tree and delete each file and subdirectory within the specified directory. Once all the files and subdirectories have been deleted, the top-level directory is also deleted.
Thanks for reading. Happy coding!