To write to CSV in Python, use Python’s csv
module.
For example, let’s write a list of strings into a new CSV file:
import csv data = ["This", "is", "a", "Test"] with open('example.csv', 'w') as file: writer = csv.writer(file) writer.writerow(data)
As a result, you see a file called example.csv
in the current folder.
This was the quick answer. But there is a lot more to cover when it comes to writing CSV files in Python. Let’s jump into the details and see some useful examples.
By the way, if you are interested in becoming a data scientist, check out these awesome Python Data Science courses!
Writing to CSV in Python in Detail
CSV or comma-separated values is a text file. It consists of comma-separated data.
CSV is a useful data format for applications to transfer data. The CSV is a simple lightweight text file. This makes it a perfect fit for sending and receiving data in an efficient way.
For example, you can retrieve data from a database as a CSV. Or you can add a new entry to a database using CSV format.
Here is an example of what CSV data looks like:
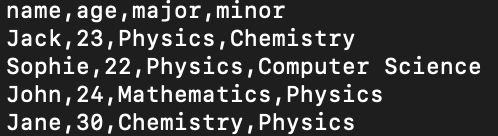
However, in this guide, I’m using Mac’s built-in CSV viewer. Thus the CSV data looks more like this:
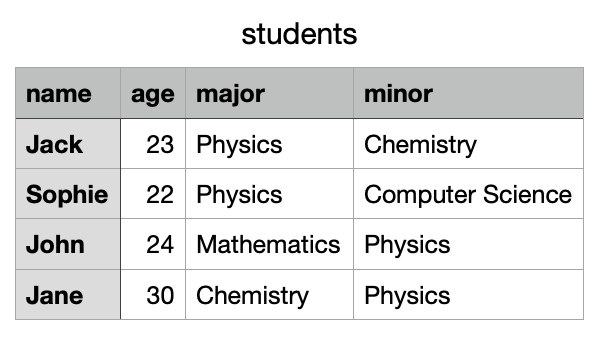
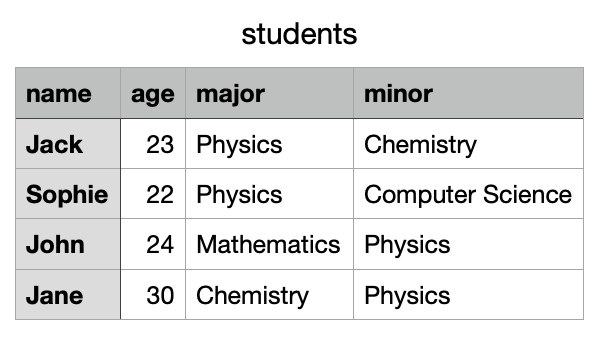
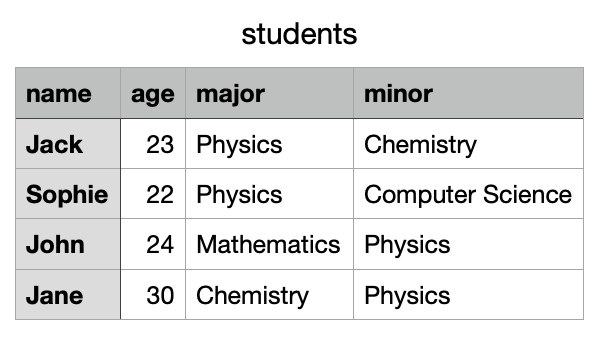
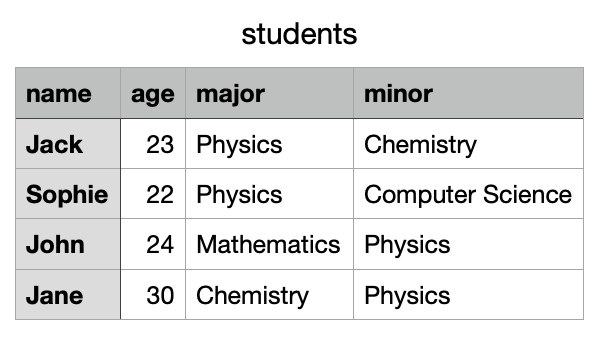
In the introduction, you saw a simple example of how to write a piece of data into a CSV file. Let’s now take a deeper look at how to write CSV files with Python.
The 4 Steps to Writing a CSV in Python
To write to a CSV file in Python:
-
Open a CSV file in the write mode. This happens using the
open()
function. Give it the path of the file as the first argument. Specify the mode as the second argument ('r'
for read and'w'
for write). -
Create a CSV writer object. To do this, create a
csv
module’swriter()
object, and pass the opened file as its argument. -
Write data to the CSV file. Use the
writer
object’swriterow()
function to write data into the CSV file. -
Close the CSV file using the
close()
method of a file.
Here is an example that illustrates this process:
import csv # 1. file = open('test.csv', 'w') # 2. writer = csv.writer(file) # 3. data = ["This", "is", "a", "Test"] writer.writerow(data) # 4. file.close()
This piece of code creates a file called test.csv
into the current folder.
The open()
function opens a new file if the file specified does not exist. If it does, then the existing file is opened.
The Shorthand Approach
To make writing to CSV a bit shorter, use the with
statement to open the file. This way you don’t need to worry about closing the file yourself. The with
takes care of that part automatically.
For instance:
import csv # 1. step with open('example.csv', 'w') as file: # 2. step writer = csv.writer(file) # 3. step data = ["This", "is", "a", "Test"] writer.writerow(data)
This creates a new CSV file called example.csv
in the current folder and writes the list of strings to it.
How to Write Non-ASCII Characters to a CSV in Python
By default, you cannot write non-ASCII characters to a CSV file.
To support writing non-ASCII values to a CSV file, specify the character encoding in the open()
call as the third argument.
with open('PATH_TO_FILE.csv', 'w', encoding="UTF8")
The rest of the process follows the steps you learned earlier.
How to Create a Header for the CSV File
So far you have created CSV files that lack the structure.
In Python, it is possible to write a header for any CSV file using the same writerow()
function you use to write any data to the CSV.
Example. Let’s create an example CSV file that consists of student data.
To structure the data nicely, create a header for the students and insert it at the beginning of the CSV file. After this, you can follow the same steps from earlier to write the data into a CSV file.
Here is the code:
import csv # Define the structure of the data student_header = ['name', 'age', 'major', 'minor'] # Define the actual data student_data = ['Jack', 23, 'Physics', 'Chemistry'] # 1. Open a new CSV file with open('students.csv', 'w') as file: # 2. Create a CSV writer writer = csv.writer(file) # 3. Write data to the file writer.writerow(student_header) writer.writerow(student_data)
This creates students.csv
file into the folder you are currently working in. The new file looks like this:
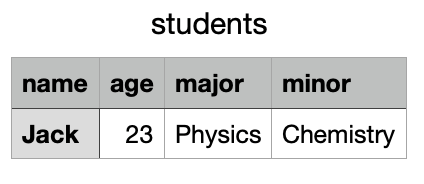
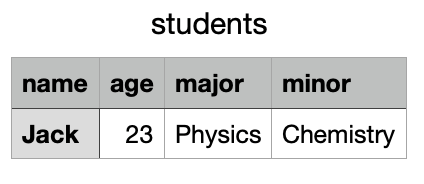
How to Write Multiple Rows into a CSV File in Python
In Python, you can use the CSV writer’s writerows()
function to write multiple rows into a CSV file on the same go.
Example. Let’s say you want to write more than one line of data into your CSV file. For instance, you may have a list of students instead of only having one of them.
To write multiple lines of data into a CSV, use the writerows()
method.
Here is an example:
import csv student_header = ['name', 'age', 'major', 'minor'] student_data = [ ['Jack', 23, 'Physics', 'Chemistry'], ['Sophie', 22, 'Physics', 'Computer Science'], ['John', 24, 'Mathematics', 'Physics'], ['Jane', 30, 'Chemistry', 'Physics'] ] with open('students.csv', 'w') as file: writer = csv.writer(file) writer.writerow(student_header) # Use writerows() not writerow() writer.writerows(student_data)
This results in a new CSV file that looks like this:
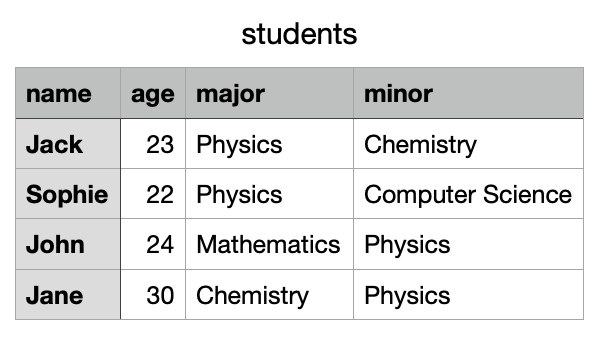
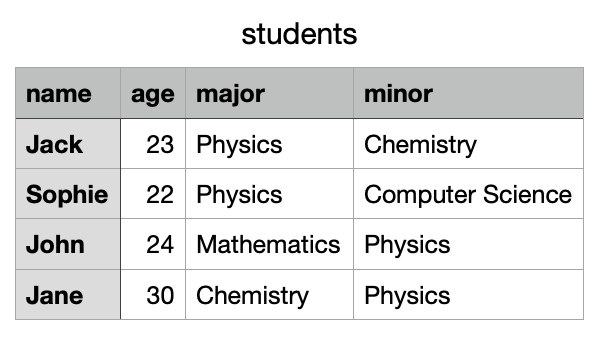
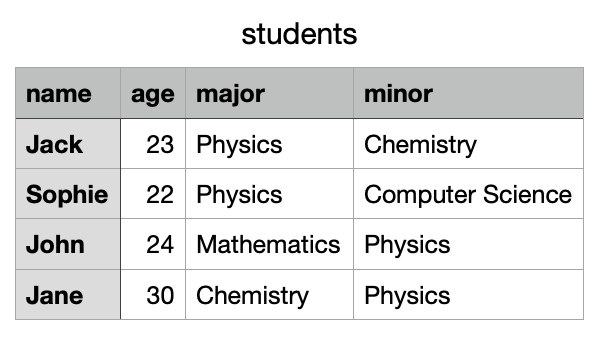
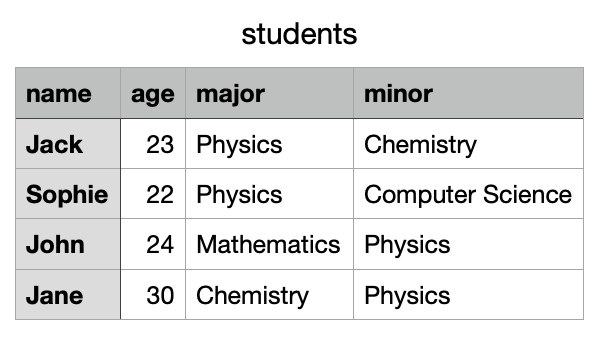
How to Write a Dictionary to a CSV File in Python
To write a dictionary into a CSV file in Python, use the DictWriter
object by following these three steps:
- Use a
csv
module’sDictWriter
object and specify the field names in it. - Use the
writeheader()
method to create the header into the CSV file. - use the
writerows()
method to write the dictionary data into the file.
Example. Let’s write a dictionary of student data into a CSV.
import csv student_header = ['name', 'age', 'major', 'minor'] student_data = [ {'name': 'Jack', 'age': 23, 'major': 'Physics', 'minor': 'Chemistry'}, {'name': 'Sophie', 'age': 22, 'major': 'Physics', 'minor': 'Computer Science'}, {'name': 'John', 'age': 24, 'major': 'Mathematics', 'minor': 'Physics'}, {'name': 'Jane', 'age': 30, 'major': 'Chemistry', 'minor': 'Physics'} ] with open('students.csv', 'w') as file: # Create a CSV dictionary writer and add the student header as field names writer = csv.DictWriter(file, fieldnames=student_header) # Use writerows() not writerow() writer.writeheader() writer.writerows(student_data)
Now the result is the same students.csv
file as in the earlier example:
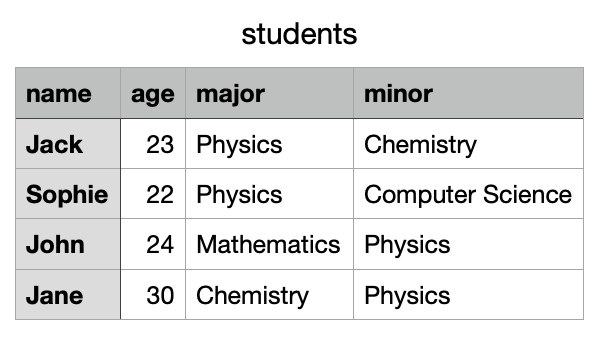
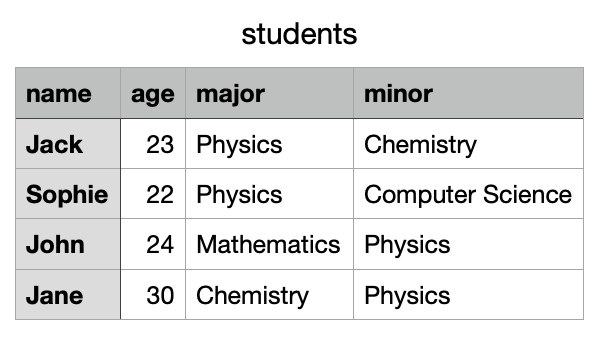
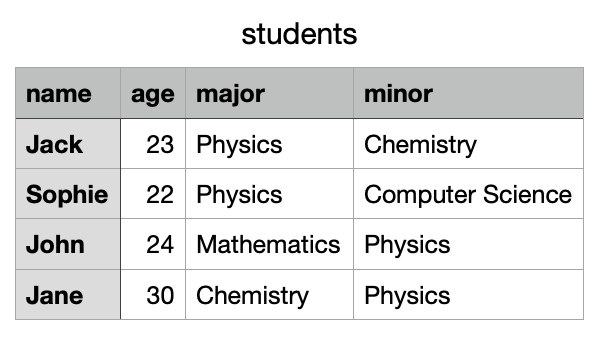
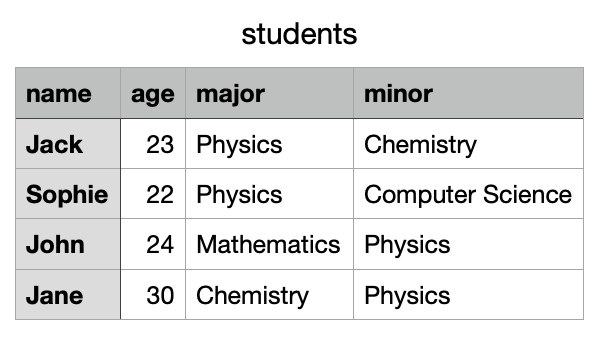
Conclusion
CSV or comma-separated values is a commonly used file format. It consists of values that are usually separated by commas.
To write into a CSV in Python, you need to use the csv
module with these steps:
- Open a CSV file in the write mode.
- Create a CSV writer object.
- Write data to the CSV file.
- Close the CSV file.
Here is a practical example.
import csv data = ["This", "is", "a", "Test"] with open('example.csv', 'w') as file: writer = csv.writer(file) writer.writerow(data)
Thanks for reading. I hope you find it useful.
Happy coding!