To create a range of floats in Python, use list comprehension.
For example, to create a range of floats from 0 to 1 with a 1/10th interval:
rng = [x / 10 for x in range(0, 10)] print(rng)
Output:
[0.0, 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9]
In this guide, you will see some alternative approaches to creating a range of floats in Python.
Problem: Python range() Function Doesn’t Work with Floats
In Python, the built-in range() function can be used to generate a range of values between m
and n
.
numbers = range(1, 6) # 1, 2, 3, 4, 5
However, the range is supposed to consist of integers only.
This means you cannot have a range() call like this:
numbers = range(0.1, 1.0)
A call like this would produce an error that warns you about misusing the range() function.
Solution 1: Divide Each Number in the Range
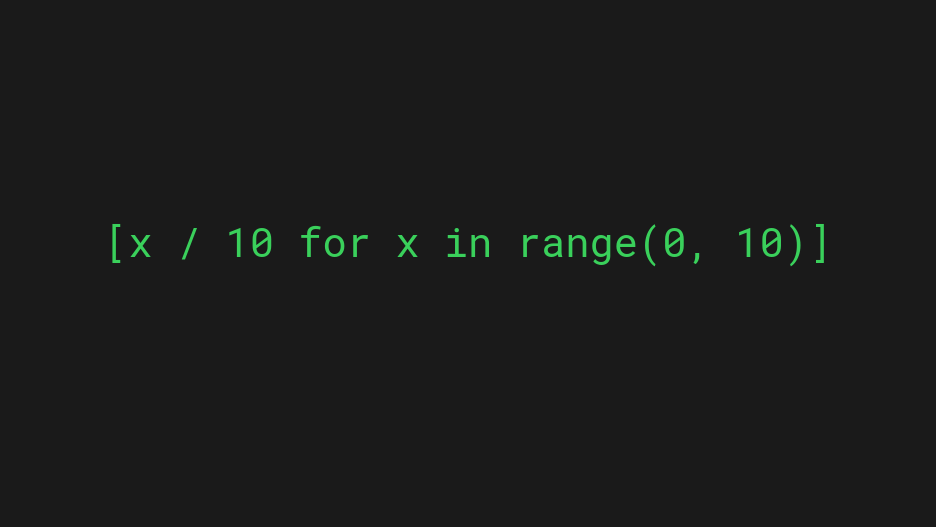
To overcome the issue of the range() function not working with floats, you can produce a range and divide each number in that range to get a range of floats.
For example, let’s generate a list that represents floats between the range 0.0 and 1.0:
numbers = range(0, 10) float_nums = [] for number in numbers: f = number / 10 float_nums.append(f) print(float_nums)
Output:
[0.0, 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9]
This for loop can be expressed in a smoother way using a list comprehension:
rng = [x / 10 for x in range(0, 10)] print(rng)
However, it gets a bit tricky when you want to produce other types of ranges.
For example, producing a list of numbers from 1.5 to 4.25, with 0.25 intervals using a for loop already requires some thinking. Needless to mention when the numbers are not evenly divisible.
This is where NumPy library can help you.
Solution 2: NumPy arrange()
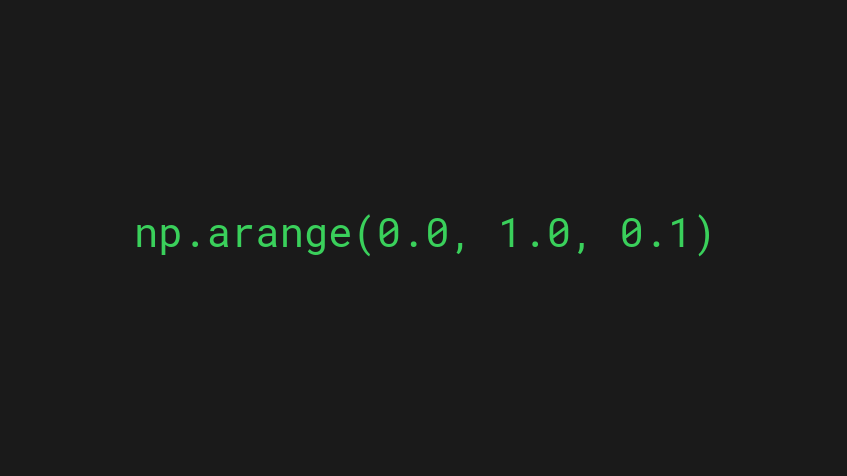
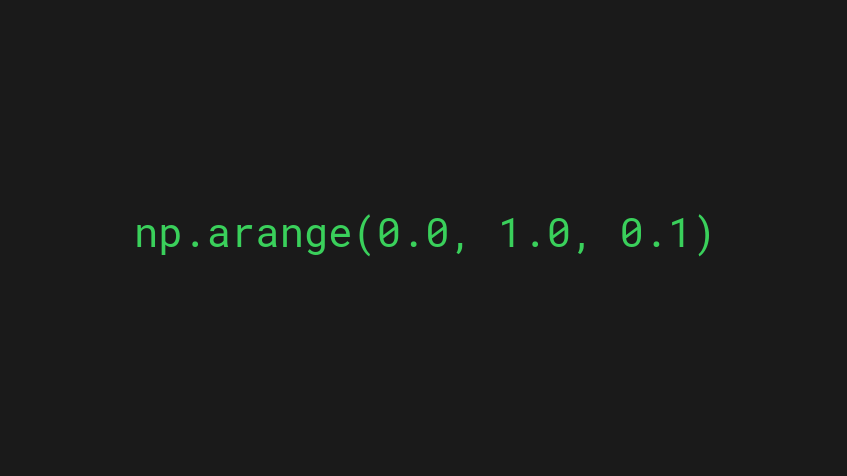
Another option to produce a range of floats is to use the NumPy module’s arange() function.
This function follows the syntax:
numpy.arange(start, stop, step)
Where:
- start is the starting value of the range.
- stop specifies the end of the range. The stop is not included in the range!
- step determines how big steps to take when generating the range.
In case you do not have NumPy installed, you can install it with PIP by running the following command in the command line:
pip install numpy
Now that you have the library, you can use the arange() function to generate a range of floats:
import numpy as np rng = np.arange(0.0, 1.0, 0.1) print(rng)
Output:
[ 0. , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9]
Notice how this range is exclusive as it does not include the end value 1.0 in the range.
To make the range inclusive, add one step size to the stop parameter.
For example, to generate a range of floats from 0.0 to 1.0:
import numpy as np start = 0.0 stop = 1.0 step = 0.1 rng = np.arange(start, stop + step, step) print(rng)
Output:
[0. 0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9 1. ]
Problem with the arange()
The problem with the arange() approach is the floating-point rounding errors.
For example, this creates an array of four values (1, 1.1, 1.2, 1.3), even though it should produce only three values (1, 1.1, 1.2):
import numpy as np rng = np.arange(1, 1.3, 0.1) print(rng)
Output:
[1. , 1.1, 1.2, 1.3]
Solution 3: NumPy linspace()
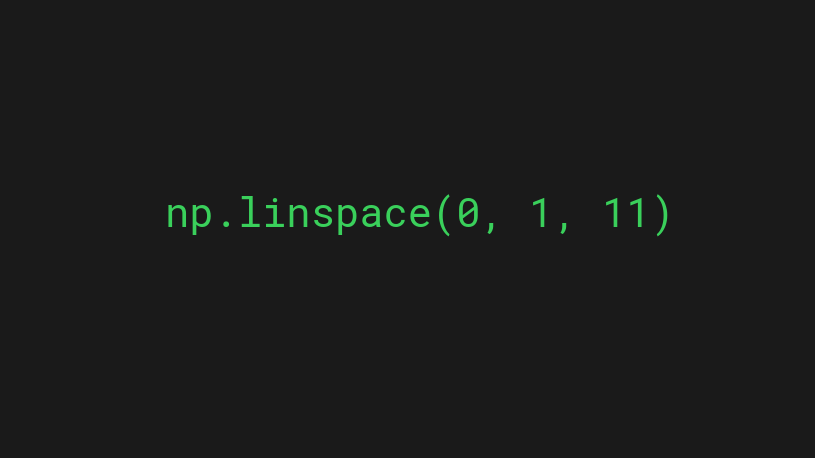
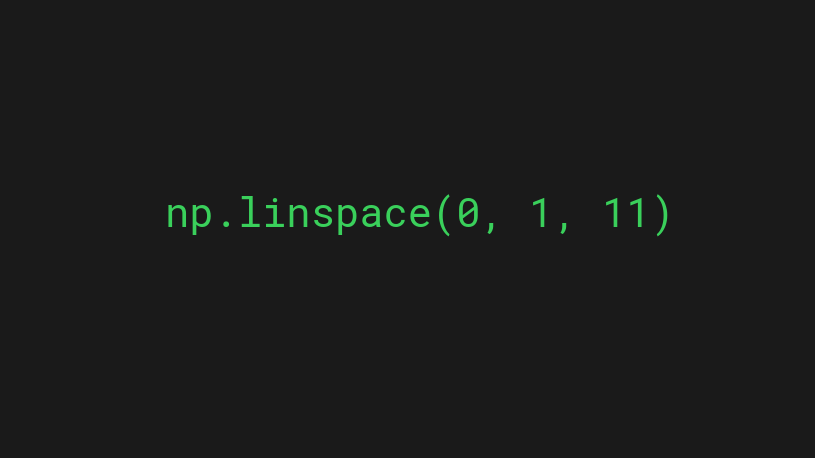
To overcome the floating-point rounding issues with the numpy’s arange() function, use numpy’s linspace() function instead.
Notice, however, that this function behaves differently. It asks how many numbers you want to linearly space between a start and an end value.
It follows this syntax:
numpy.linspace(start, stop, nvalues)
Where:
- start is the starting value of the range.
- stop is the ending value of the range.
- nvalues is the number of values to generate in-between start and stop.
For example, let’s generate values from 0.0 to 1.0 with 0.1 intervals. This means the start is 0 and the end is 1. Also, notice you want 11 values in total.
Here is how it looks in the code:
import numpy as np rng = np.linspace(0, 1, 11) print(rng)
Output:
[0. 0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9 1. ]
Conclusion
To create a range of floats in Python, you cannot use the range() function directly. This is because the range() function only supports integers. To overcome this problem, you have a couple of options:
- List comprehension
-
NumPy’s
arange()
function -
NumPy’s
linspace()
function
Thanks for reading. Happy coding!