In short, the main difference between tuples vs lists in Python is mutability.
- A list is mutable.
- A tuple is immutable.
In other words, a Python list can be changed, but a tuple cannot.
This means a tuple performs slightly better than a list. But at the same, a tuple has fewer use cases because it cannot be modified.
In short, here are all the key differences between tuples and lists.
List | Tuple |
|
|
|
|
|
|
|
|
|
|
Notice how all these differences are a consequence of a tuple being immutable.
Let’s go through the differences in a bit more detail. But before we do that, let’s briefly describe what are tuples and lists in the first place.
What Is a Tuple in Python?
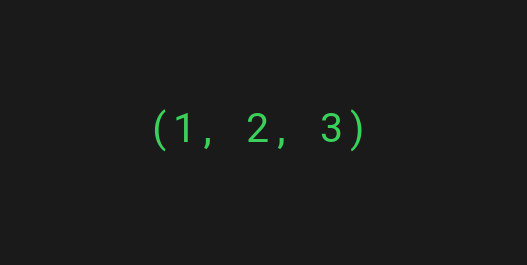
A tuple is a commonly used data type in Python. You can create tuples by comma-separating items (between parenthesis).
For example:
names = ("James", "Adam", "Paul")
Or just:
names = "James", "Adam", "Paul"
After creating a tuple, you cannot make changes to it. This is because the main purpose of a tuple is to hold values that are not supposed to change over time.
What Is a List in Python?
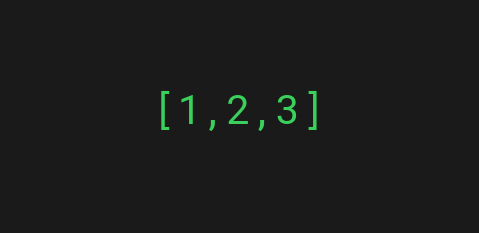
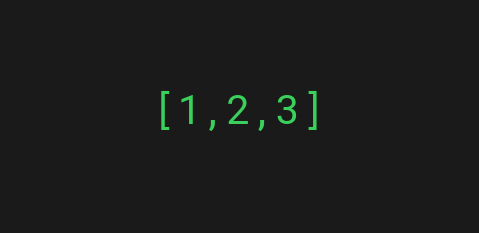
A list is a popular data type in Python. Lists store multiple items that can be accessed, modified, and removed easily.
To create a list, add comma-separated items between a set of square brackets.
For example:
names = ["James", "Adam", "Paul"]
Tuples vs Lists in Python—Detailed Comparison
Let’s take a detailed look at the differences between tuples and lists in Python.
1. Syntax Differences Between Tuples and Lists
The most obvious difference between a tuple and a list is the syntax for creating them.
While a list is created using square brackets, a tuple is created using regular parenthesis or without parenthesis.
For example:
num_list = [1, 2, 3, 4, 5] num_tuple = 1, 2, 3, 4, 5
2. Mutability
Mutability means being able to change the object after it’s been created. The major difference between a list and tuple lies in the mutability of the objects.
A List Is Mutable
A list is mutable. In other words, you can change its elements after creating it. You can add, remove, or update elements of the list.
numbers = [1, 2, 3] # Add a new element to the end of a list. numbers.append(4) # Remove the first element of a list. numbers.pop(0) # Update the 3rd element of a list. numbers[2] = 1000 print(numbers)
Tuple Is Immutable
A tuple is immutable. This means you cannot change its contents after it has been created.
For example, let’s try to update the first element of a tuple:
numbers = 1, 2, 3 # TRYING to update a value of a tuple FAILS numbers[0] = 1000
This results in an error:
TypeError: 'tuple' object does not support item assignment
If you want to add, remove, or update a value in a tuple, you need to create a new tuple. But you cannot directly modify a tuple in any way.
3. Memory and Performance of Tuples vs Lists
Tuples consume a bit less memory than lists.
For example, let’s compare two identical groups of items where the other one is a tuple and the other is a list.
names_tuple = 'Nick', 'Jan', 'Sophie' names_list = ['Nick', 'Jan', 'Sophie'] print(names_tuple.__sizeof__()) print(names_list.__sizeof__())
Output:
48 104
The result of calling the __sizeof__()
method is the internal size of the object in bytes. Here you can see that the tuple takes only 48 bytes, whereas the list requires 104.
As a tuple cannot be modified, its size remains the same. Thus, a tuple does not have to over-allocate space. In contrast, a list mildly over-allocates space to make the append()
method works efficiently.
4. Built-In Methods of Tuples and Lists
A tuple has fewer built-in methods than a list. This is mainly because a tuple is immutable. Thus operations such as insertion, deletion, or update would be meaningless.
Here are a bunch of useful methods for updating a list (that you already saw):
numbers = [1, 2, 3] numbers.append(4) numbers.pop(0) numbers[2] = 1000
None of these built-in list methods work for tuples.
To get a list of all the available methods of tuples and lists, use the dir()
function:
dir(tuple) dir(list)
The dir()
function returns a big list that consists of all the built-in methods for the type. At the time of writing, the Python list has 47 built-in methods, whereas a tuple only has 34.
Should I Use a Tuple or a List?
Now that you know the physical differences between tuples and lists in Python, it’s good to finish off by learning which one to pick.
Most of the time, you benefit from lists more than tuples.
But a tuple is useful when you want to create a collection of elements that should not change over time. Using a tuple, in this case, is good, as it makes it harder for you to make mistakes. This is because changing a tuple value is not possible, as tuples are immutable.
Conclusion
Today you learned what is the difference between a tuple and a list in Python.
To recap, the key difference is mutability. A Python list can be changed after creation. But a tuple cannot.
When it comes to syntactical differences, a tuple is created with regular parenthesis or without parenthesis. A list is created with square brackets.
A tuple has fewer “moving parts” to it so it performs slightly better than a list.
A tuple is meant to hold values that are not supposed to change. If you want to change the values of a tuple, use a list instead.
Thanks for reading. I hope you find it useful.
Happy coding!