Version control means tracking and managing changes to software code. With version control, software development teams are able to work faster and more efficiently. A version control tool allows for improved collaboration. The software development team can work simultaneously on different parts of the codebase without collisions.
This guide teaches you what version control is on a high level. You will learn why software developers should use one and how it even works. Besides, you are going to learn what are the most popular choices for version control systems. At the end of this guide, you are going to see a quick example of how to version and manage a simple example code project.
What Is Version Control?
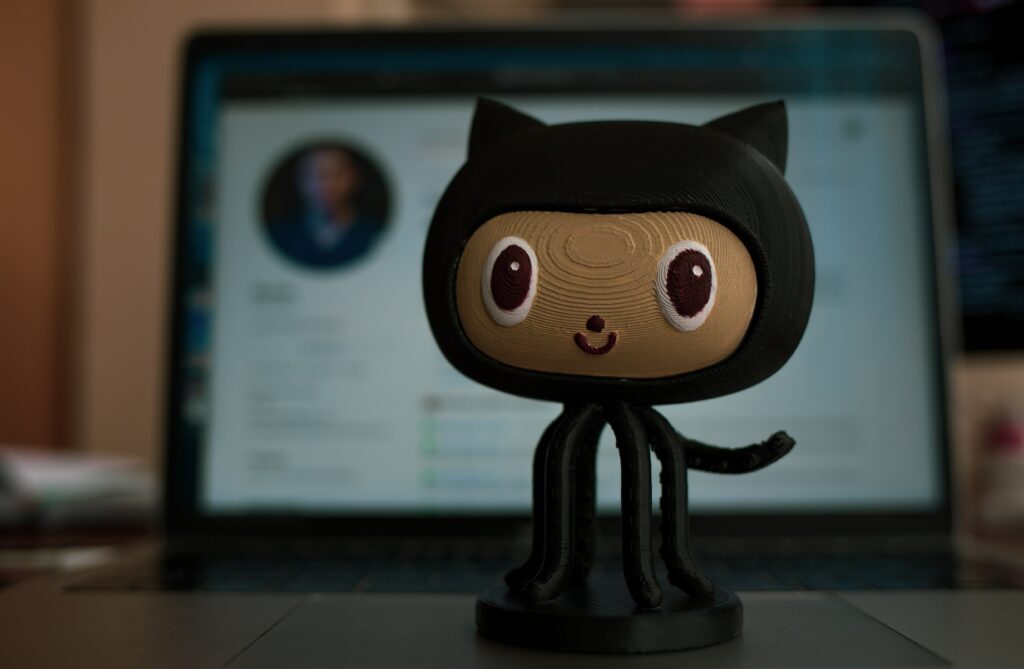
As the name suggests, version control is a software code management system. To understand the word version control better, think about software versioning. Whenever you make a change to the codebase, you are essentially creating a new version of the software. A system that manages these code changes is then naturally a version control system.
Version control keeps track of every single code change. The system stores the changes in a specific type of version control database. If a developer makes a mistake, it’s easy to jump back into the version history to restore the version before the change.
In a sense, version control is like “CTRL + Z on steroids”. You can not only undo the changes made in the current session but also jump back in time to the early days of your project. If you removed a feature a year ago that you now want back, you can do it with version control with no problem.
Developing software at scale wouldn’t be possible without version control. Of course, if you are getting started with coding and working with basic examples, you don’t need to spend time versioning them. That being said, learning how version control works are important, and you should get started with it as soon as possible. No matter what software position you land on, you will need to learn version control.
The version control is not only great for undoing mistakes, but it’s also a great overall system that promotes collaboration. With a version control system, each team member can work on their own branch that doesn’t interfere with the main program.
The developers take a copy of the current version of the software to make edits to it. When the edits are ready, the copy version of the software needs to be merged with the actual version of the software. With version control, this is easy. You can merge changes to the main software. If there are contradictions, the version control system highlights them in an easy-to-understand fashion.
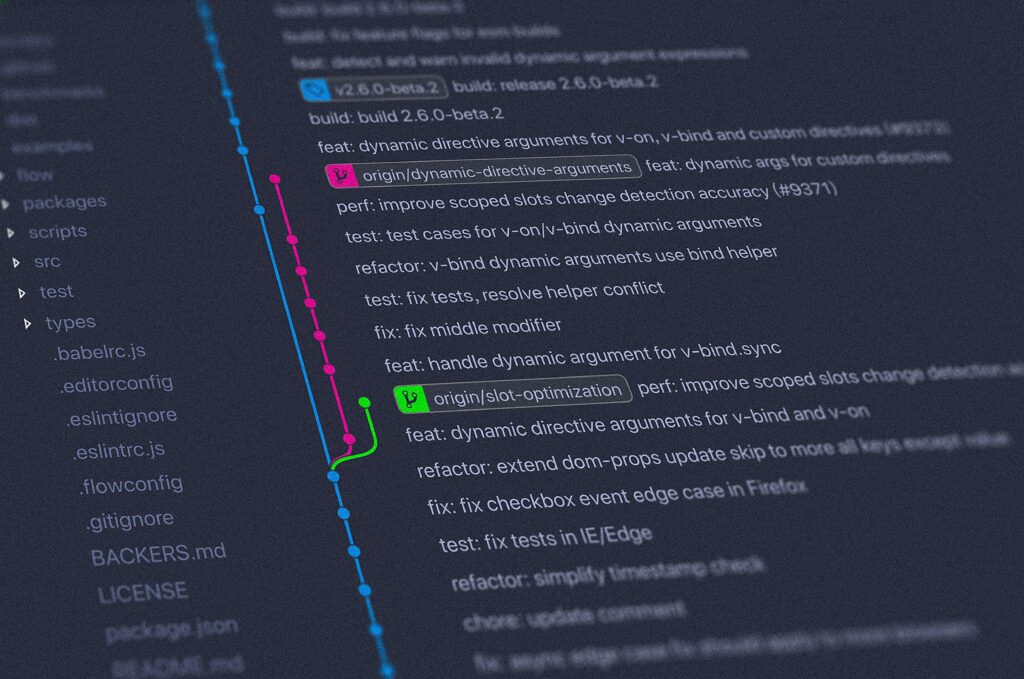
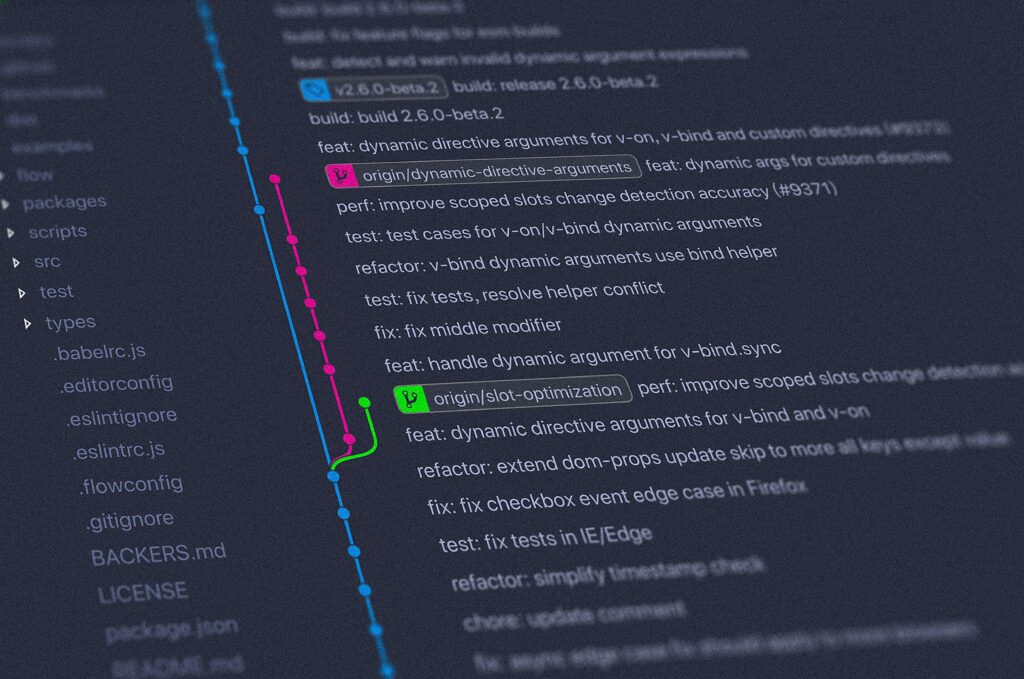
Software developers don’t send code changes over WhatsApp or email. They use version control.
But keep in mind, that you won’t learn version control overnight. It takes a ton of practice because there are lots of new terms and concepts you need to embrace. Some universities even have version control courses. But once you get the hang of it, you’ll never avoid using version control ever again.
Version Control Benefits
Using version control is a necessity in software development teams. It allows teams to move faster and deliver quicker. When a development team grows, version control makes it possible to keep up with the pace. During the past decades, the version control landscape has improved by a ton. One of the most popular version control systems is Git.
Here is a quick breakdown of the benefits of using version control systems, such as Git.
1. Best Version Control Systems Are Free
The best version control systems are free. For instance, the Git version control system comes at no cost. This lowers the barrier for individuals as well as larger enterprises to adapt to the version control systems. Besides being free, version control is easy to set up and the workflow is relatively easy to learn.
2. Access to the Entire Project History
With version control, you can track the entire history of a project or a file. Any changes made throughout the years remain in the version control system. This applies to creating and deleting entire files too.
The history information comes with details of the author, date, purpose of the changes, and more.
Having such a complete picture of the history of the software allows for tracking issues. Besides, this makes it possible to update older versions of the software.
3. Branching and Merging for Collaboration
Think about a software development team. Each individual developer needs to be able to work on the same codebase at the same time.
This is where version control helps. A version control system allows you to create your own branch. This is an independent copy of the original codebase where you can work without interfering with the main codebase. Once you have made changes, you can merge the branch back to the main version of the software.
In a software development team, each developer can create their own branch and work there at the same time. This is the only manageable way to simultaneously work on the software.
Without branching and merging, developers would quite literally need to send code changes in WhatsApp or email. Imagine what chaos that would be.
4. Integrations with Project Management Tools
With version control, you can connect every code change to project management and bug-tracking software, such as Jira.
In a project management system, you can create bug tickets and tasks to represent new features and changes. Furthermore, you can assign these tickets to developers in a transparent fashion. Also, you can have discussions in the tickets’ comment sections.
You can couple the project management tools with a version control system. This makes it possible to automatically update the bug/feature ticket statuses based on the commits made to the codebase. All in all, this improves the clarity of developing software in teams and makes the code history even more accessible.
Most Popular Version Control Systems
In the version control space, Git dominates. It’s the most popular version control system in use. Git comes with the most powerful feature set for developing software at scale. Most third-party platforms support Github. Also, the workflow is the most reliable and relatively easy to adapt to.
Here are the most popular version control systems.
- Git
- Apache Subversion (SVN)
- Mercurial
- Perforce Helix Core
- Concurrent Versions System (CVS)
If you are not sure where to start, start with Git. It’s the most reliable and popular version control system. Needless to mention, it’s free to use!
Version Control Example with Git
Let’s take a quick look at how version control works in a nutshell. The main point here is not to necessarily teach you how to start using version control. Instead, I’m going to show you quickly how easy it is to set up. Furthermore, you are going to see a couple of the most common version control commands and workflows.
Let’s start with a Python project folder exampleProject that has an empty file called file.py.
To put this project under version control, you need to open up Terminal (or command line) and change directory to the project folder. Inside the project folder, run the command git init:
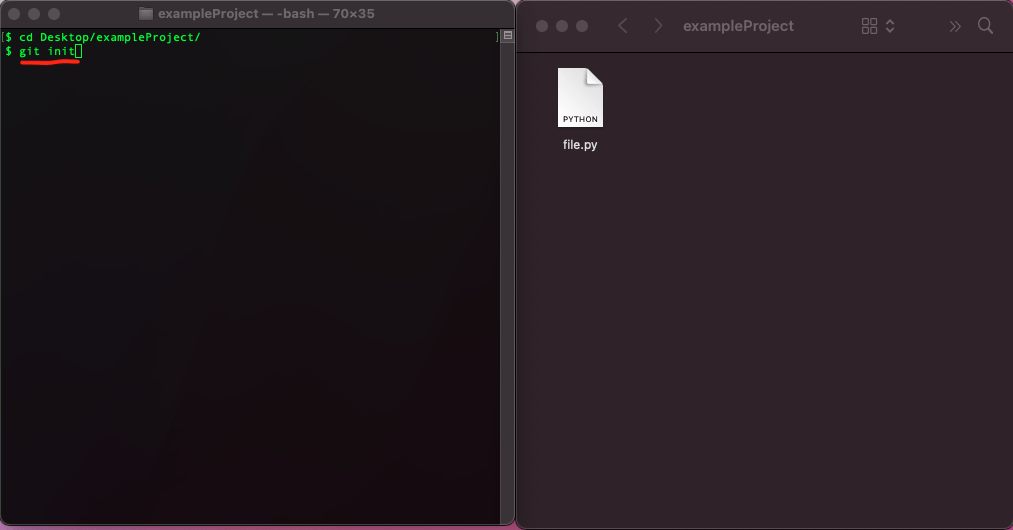
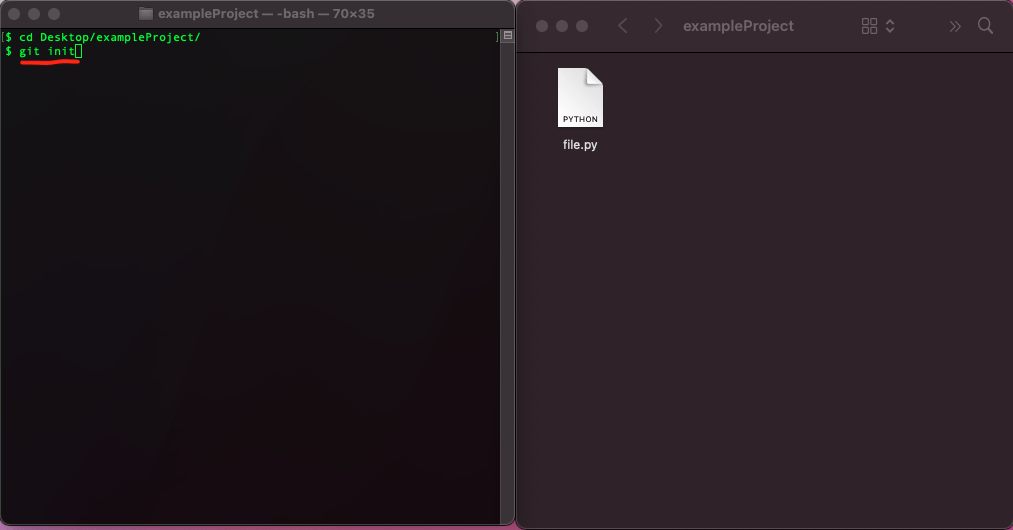
Now the example project is under version control!
Next, let’s commit the empty file.py to the project. This means the version control system starts tracking changes to the file.py. To commit the file, you need to:
- Prepare the file for commit. This happens with git add filename command.
- Commit the file with a message. This happens with git commit -m “message here”.
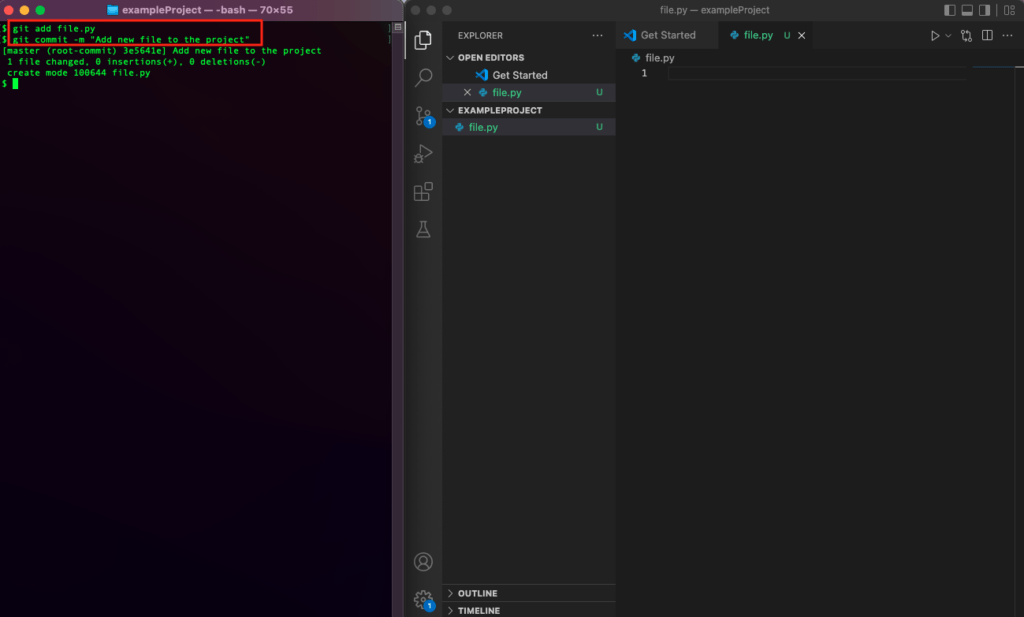
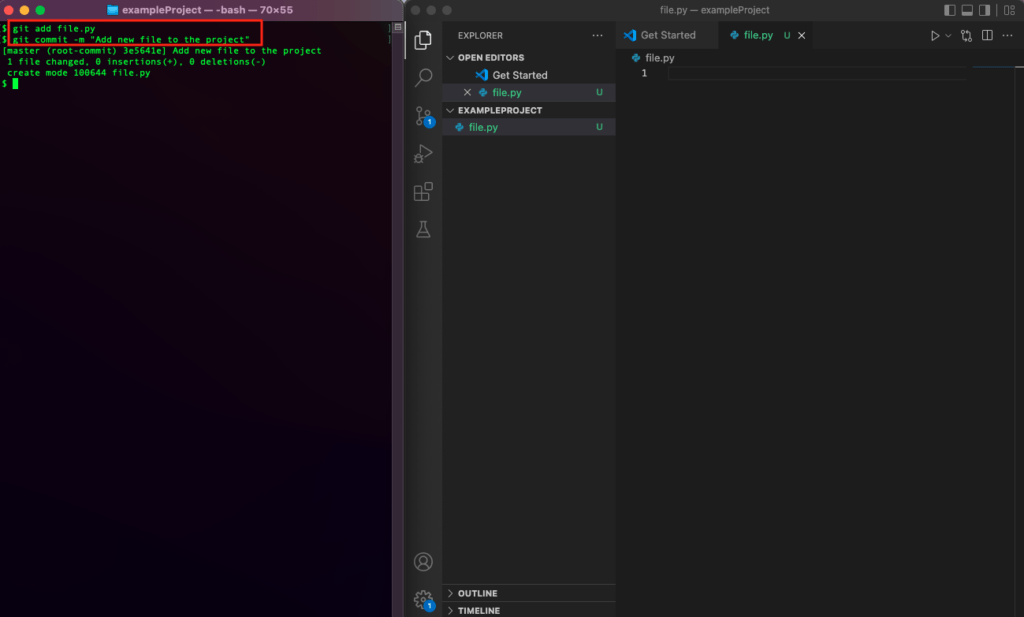
Now the file.py is being tracked for changes! Next, let’s add some code to the file. Once you have added some functionality to the file, you can check the changes the version control sees by calling git diff:
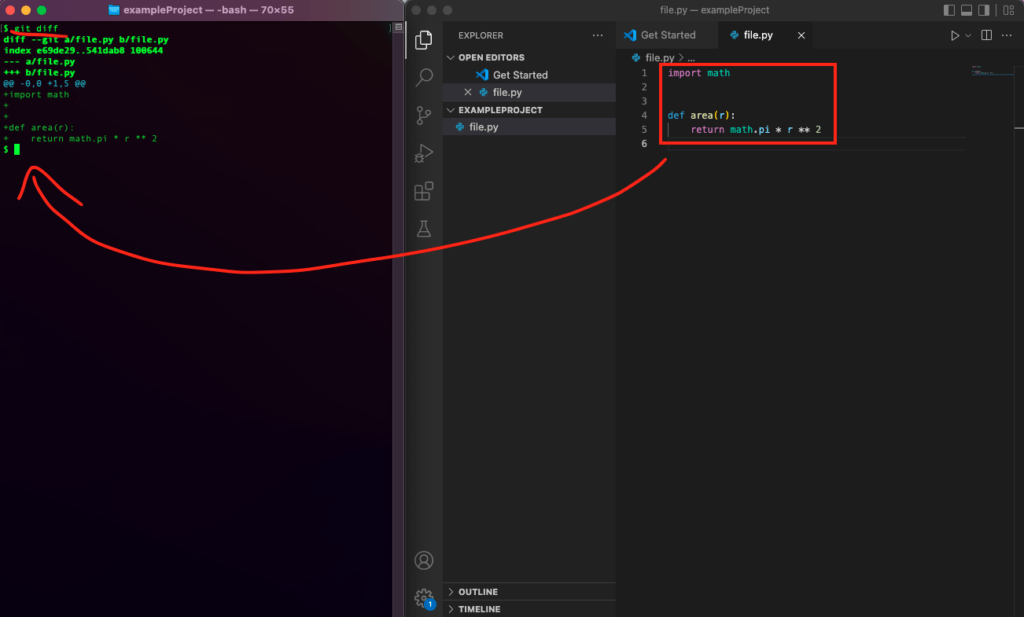
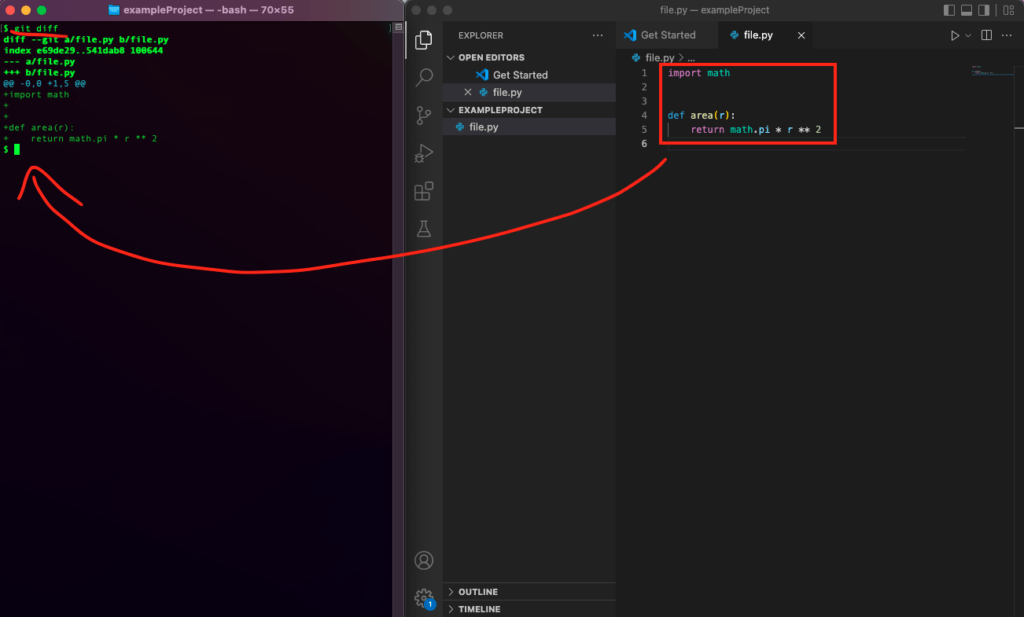
Git shows + sign in front of the newly added code lines on the terminal view. These are the changes you just made. But now these changes are not labeled in any way. To store these changes in the version control system, you need to commit them, similar to how you committed the empty file:
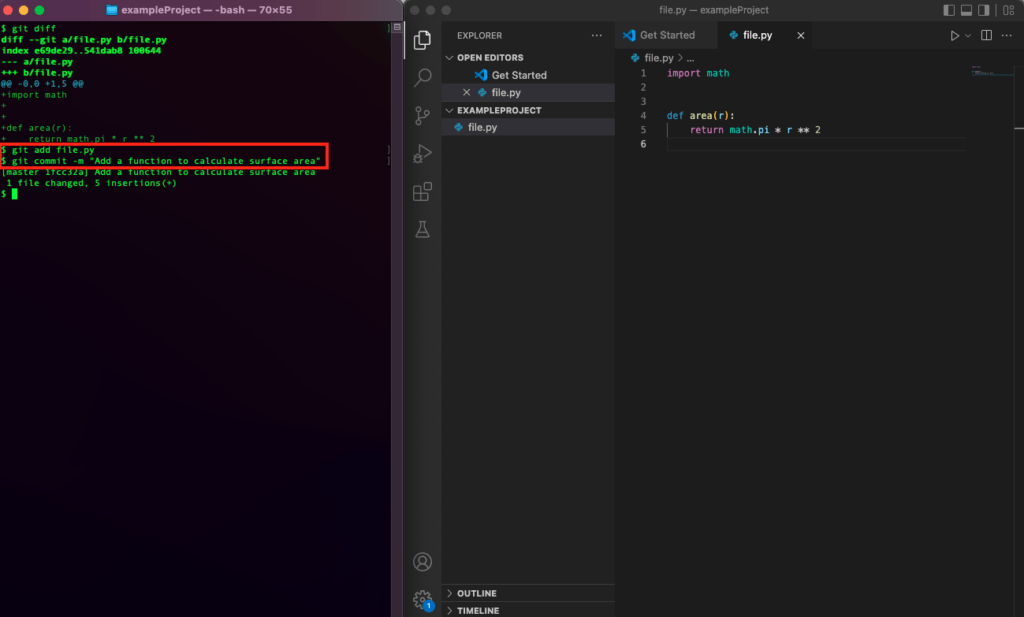
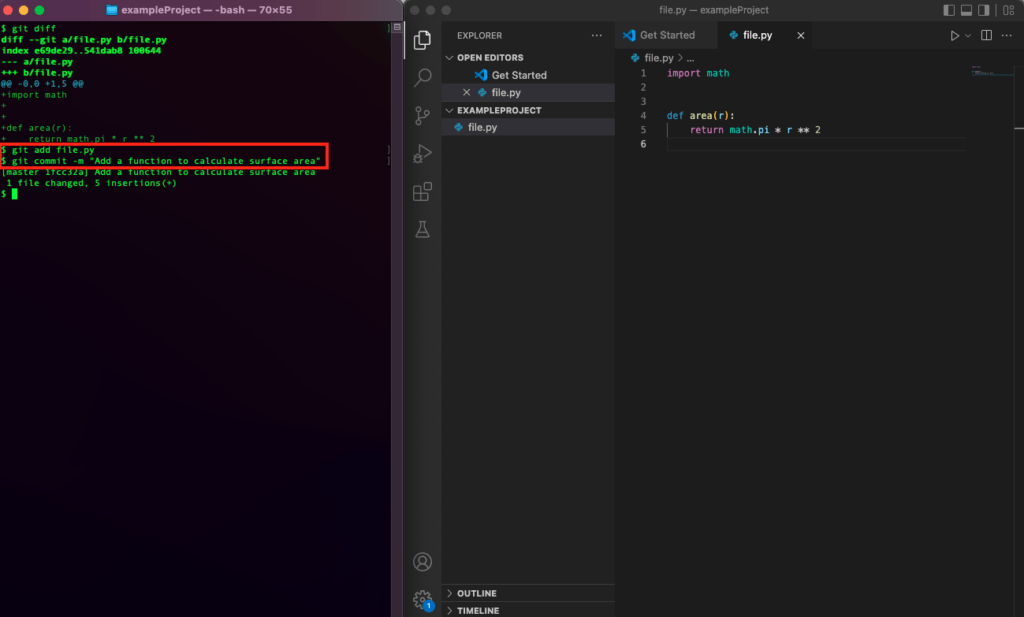
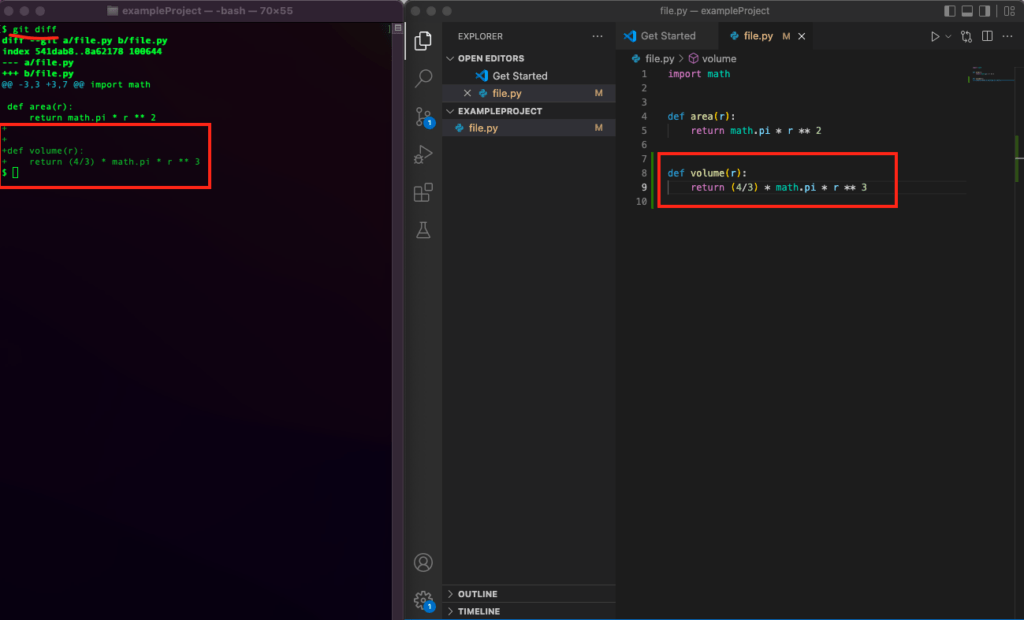
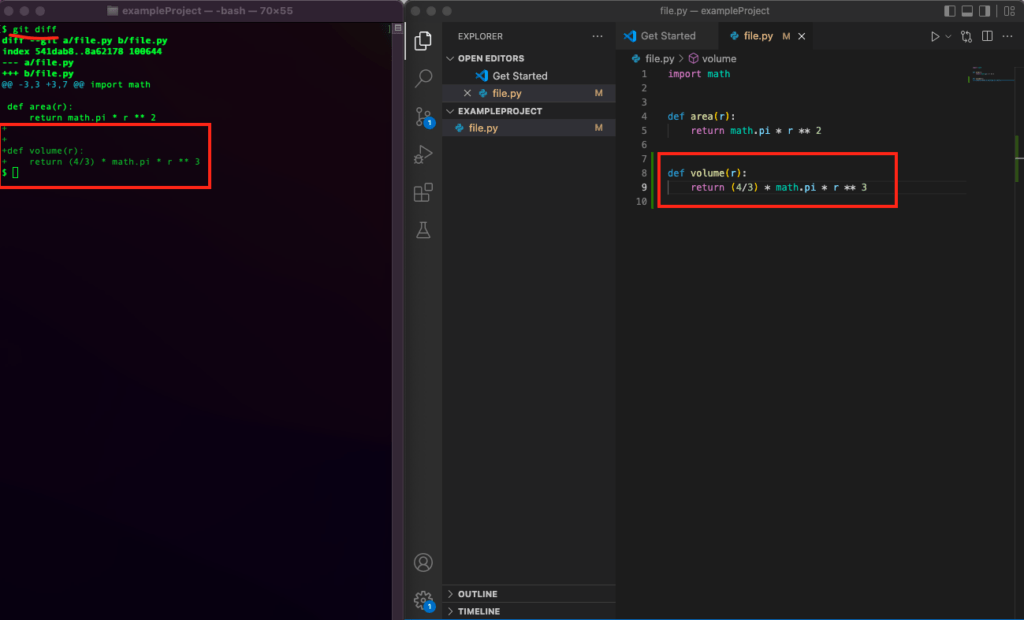
Now the new code lines are stored in the version control. For the sake of demonstration, let’s add a new function to the code file. Then, let’s call git diff again.
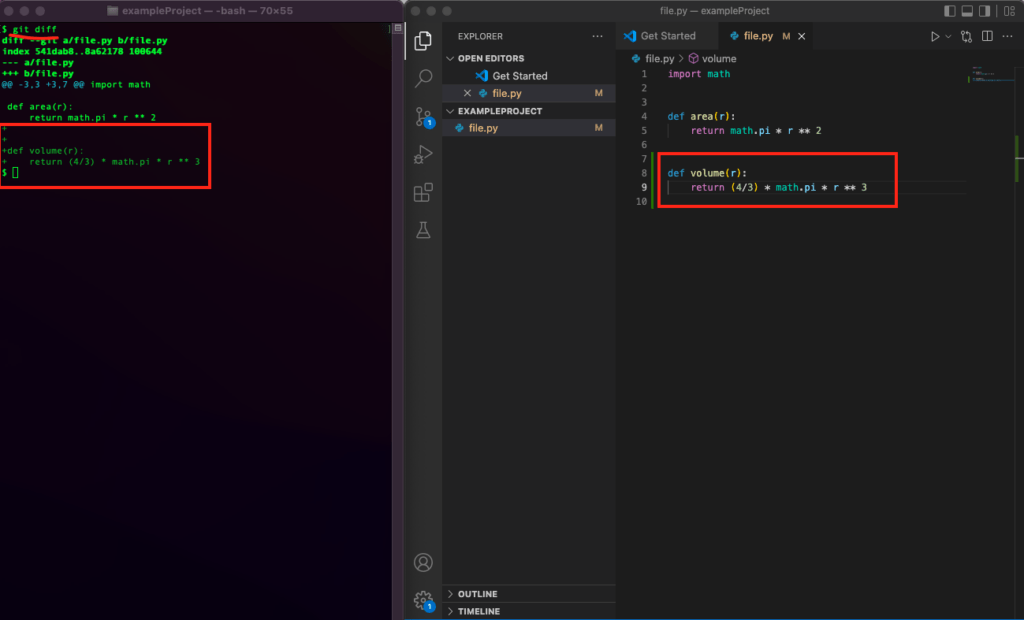
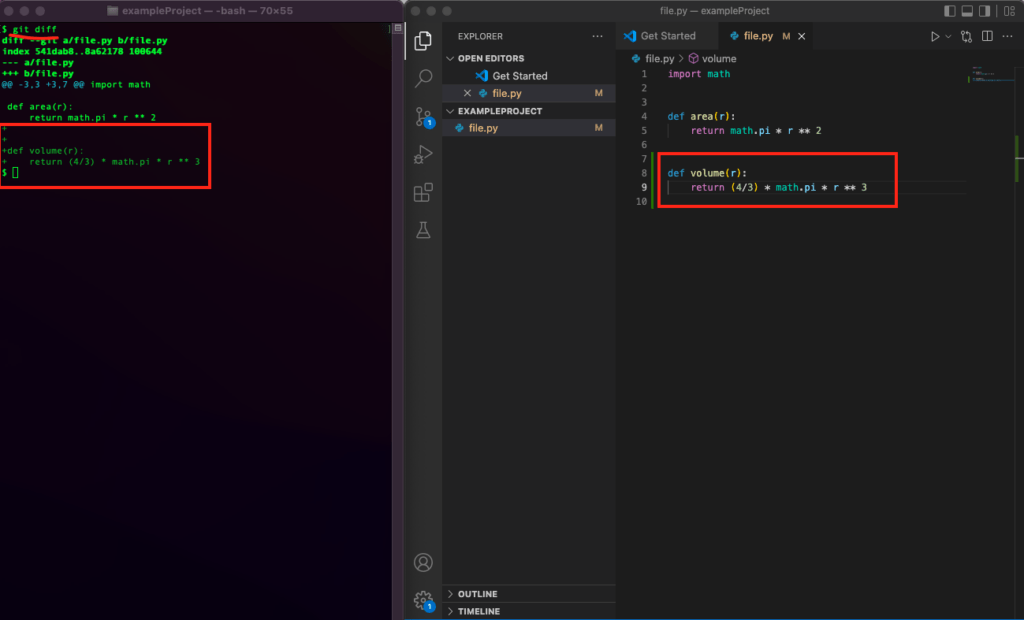
Git shows the changes in the file on the terminal window again. The new lines are marked with a + sign. Let’s commit these changes again:
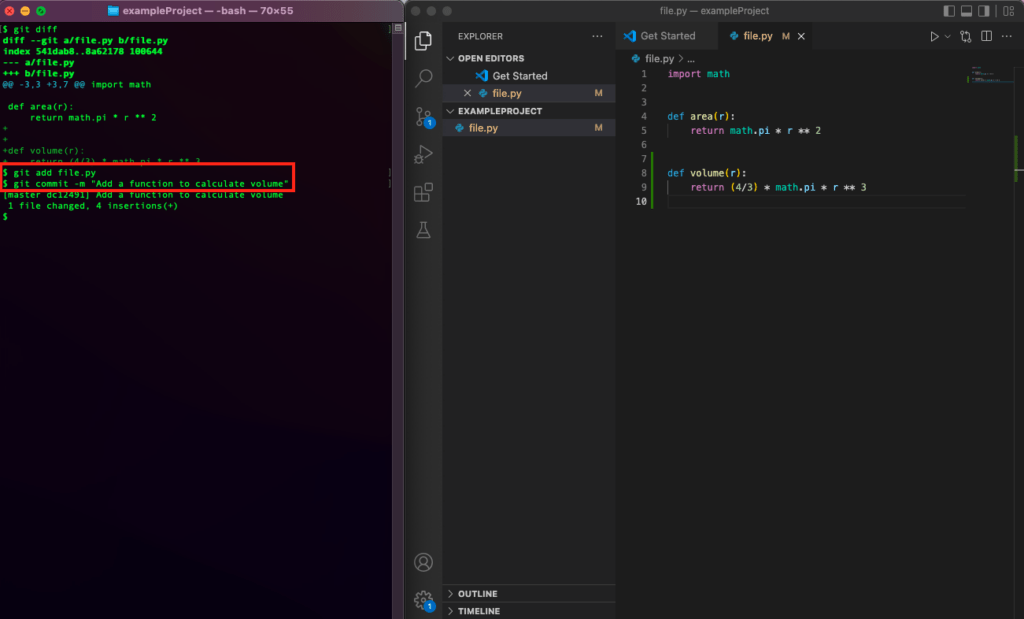
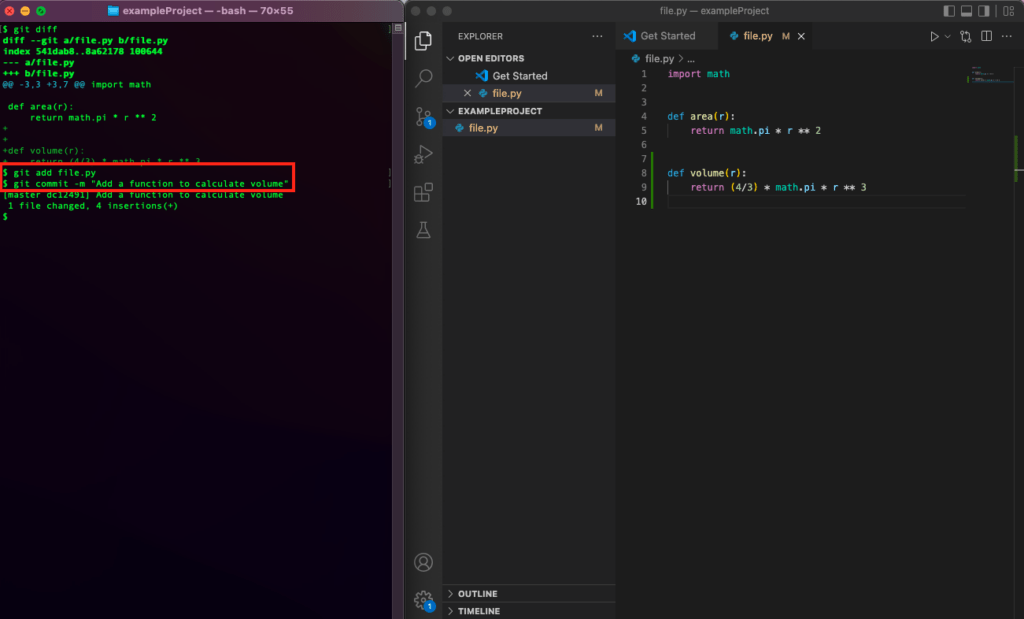
To recap, thus far you have recorded three separate changes to your example project:
- Add an empty Python file.
- Add a function to calculate the area of a circle.
- Add a function to calculate the volume of a circle.
To actually see these documented in the version control, you can call git log:
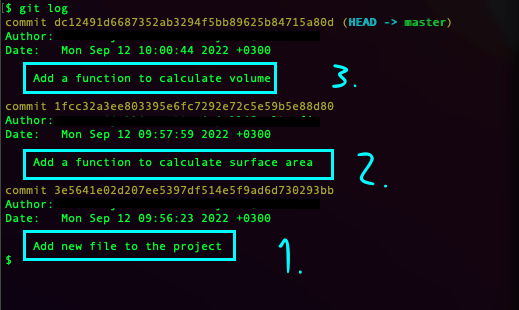
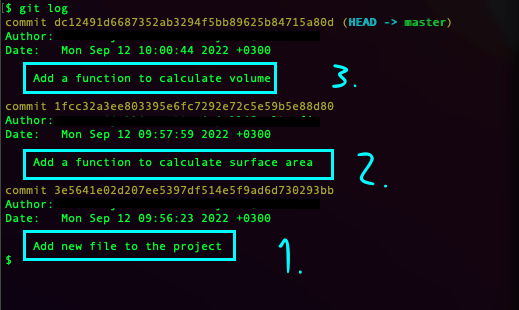
This shows you the three separate changes you recorded (committed) to the project.
Take a look at the above screenshot of the logs. Each commit has a unique hash before it. You can use this hash to travel back in time to see how the code file looked after a particular code change. For example, let’s grab the 2nd commit hash and use git checkout hash_id to travel back in time.
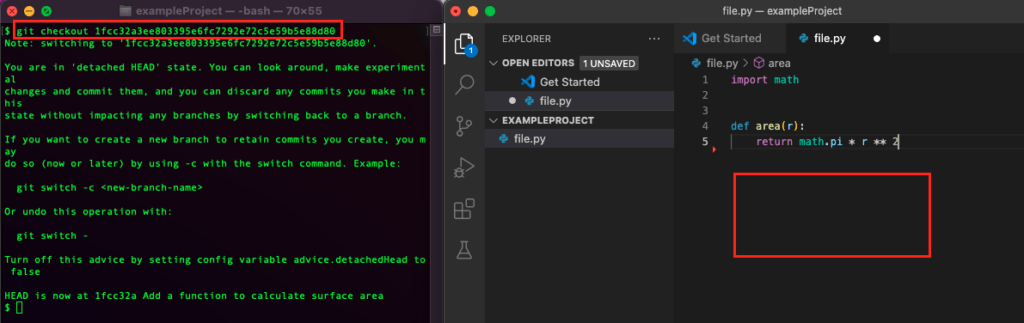
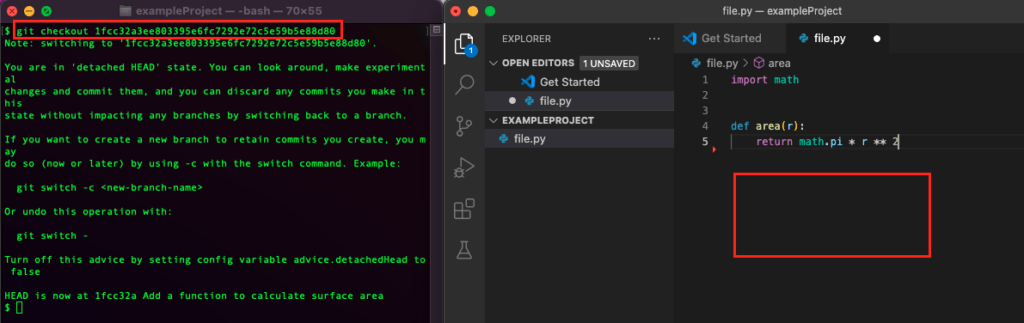
As you can see, the volume function is now gone from the code file. This is because you jumped back in time to see what the file looked like before creating the volume function.
To jump back to the previous commit, you can call git checkout –.
Awesome! So now you saw the very basics of version control. Notice that the above example merely scratches the surface. There are much more useful features that I didn’t cover to keep it in scope. These include:
- Uploading the project into a cloud-based version of Git, that is, GitHub.
- Inviting collaborators.
- Collaborating with the codebase with branching.
- Modifying, removing, and rearranging commits.
- Reverting changes.
- Doing pull requests.
And much more.
Wrap Up
Version control is a system that keeps track of code changes. It’s a tool that enables the development of software at scale. Both individual developers and development teams benefit from version control systems. The main benefits of version control include:
- Access to the full project history
- Branching and merging for improved collaboration
- Integrations with project management tools and such
Besides, the best version control system, Git, is completely free to use.
Thanks for reading! I hope you start learning version control as soon as possible! Just remember to be patient. Don’t expect to master version control overnight.