Drawing a Christmas tree with asterisks (*) in Python isn’t probably the most impressive Christmas present, but it offers a nice challenge that tests your understanding of loops.
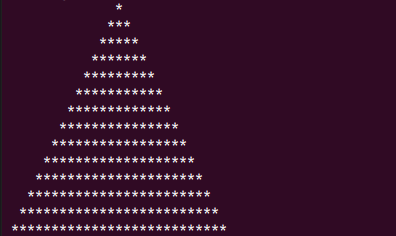
This guide teaches you how to draw Christmas trees in Python in three different ways that incorporate loops and recursion.
Let’s jump into it!
Drawing Christmas Trees in Python
There are three main ways to draw Christmas trees in Python:
- For loop
- While Loop
- Recursion
Let’s take a closer look at how each of these approaches works. In each example, the code has comments you can read to follow along.
1. For Loop Approach
To draw a Christmas tree using asterisks (*) in Python, you can use a for loop to print the asterisks in the shape of a tree.
Here is a code example of how to do this:
# Function to draw a Christmas tree with a given height def draw_tree(height): # Loop through each row of the tree for i in range(1, height + 1): # Print the spaces before the asterisks on each row for j in range(height - i): print(" ", end="") # Print the asterisks on each row for j in range(2 * i - 1): print("*", end="") # Move to the next line print() # Call the function to draw a tree with a height of 5 draw_tree(5)
This piece of code defines a draw_tree()
function. It takes a height as an argument and uses two nested for loops to print the asterisks in the shape of a Christmas tree.
- The outer for loop iterates through each row of the tree.
- The inner for loop prints the asterisks on each row.
The number of spaces before the asterisks and the number of asterisks on each row is calculated based on the height of the tree.
When you call the draw_tree()
function and pass it a height, it will print a Christmas tree with the given height.
For instance, if you call draw_tree(5)
, it prints a Christmas tree like follows:
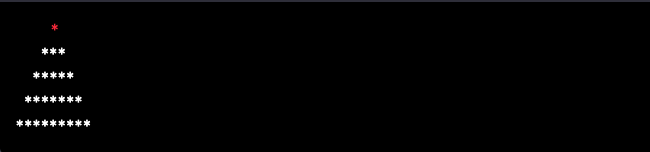
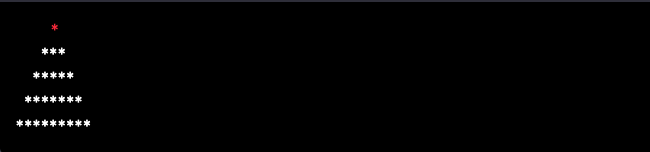
2. While Loop Approach
The for-loop approach is the simplest one to generate a Christmas tree in Python.
But it doesn’t mean you couldn’t do it with a while loop. More importantly, if you’re new to loops, you should definitely try reproducing the tree in the previous example using a while loop.
To draw a Christmas tree in Python, let’s use a while loop to print the asterisks in the shape of a tree.
Here’s what it looks like in the code:
# Function to draw a Christmas tree with a given height def draw_tree(height): # Set the initial values for the while loop i = 1 j = 1 # Loop while the row number is less than or equal to the height of the tree while i <= height: # Print the spaces before the asterisks on each row while j <= height - i: print(" ", end="") j += 1 # Reset the value of j j = 1 # Print the asterisks on each row while j <= 2 * i - 1: print("*", end="") j += 1 # Reset the value of j j = 1 # Move to the next line print() # Increment the value of i i += 1 # Call the function to draw a tree with a height of 5 draw_tree(15)
This piece of code defines a draw_tree()
function that takes a height as an argument and uses two nested while loops to print the asterisks in the shape of a Christmas tree.
Once again, the outer loop iterates through each row of the tree, and the inner loop prints the asterisks on each row.
The number of spaces before the asterisks and the number of asterisks on each row is calculated based on the height of the tree.
When you call the draw_tree()
function and pass it a height, it will print a Christmas tree with that height using asterisks (*).
For example, if you call draw_tree(15)
, it will print a Christmas tree with a height of 15:
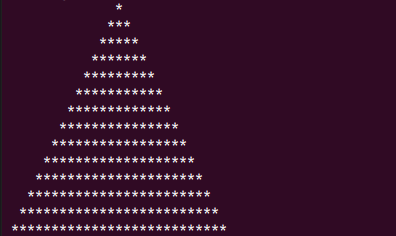
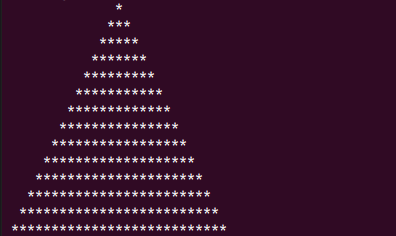
3. Recursion Approach
Last but not least, let’s use a recursive approach for printing the Christmas tree.
In case you’re unfamiliar with recursion, it means that a function calls itself causing a loop-like code structure. If this is the first time you hear about recursion, you should read a separate article about the topic before proceeding. It takes a moment to wrap your head around recursion.
To draw a Christmas tree using asterisks (*) in Python, you can use a recursive function that takes the height and level of the tree as arguments, and prints the asterisks in the shape of a tree.
Here is an example of how to do this:
# Function to draw a Christmas tree with a given height and level def draw_tree(height, level): # Check if the level is equal to the height of the tree if level == height: # Return if the level is equal to the height return # Print the spaces before the asterisks on each row for j in range(height - level): print(" ", end="") # Print the asterisks on each row for j in range(2 * level - 1): print("*", end="") # Move to the next line print() # Call the function recursively with the next level draw_tree(height, level + 1) # Call the function to draw a tree with a height of 5 draw_tree(5, 1)
This code defines a draw_tree()
function that takes a height and a level as arguments, and uses recursion to print the asterisks in the shape of a Christmas tree.
The function checks if the level is equal to the tree’s height, and returns if it is. If the level is not equal to the height, it prints the asterisks on the current level, then calls itself recursively with the next level.
When you call the draw_tree()
function and pass it a height and a starting level (usually 1), it will print a Christmas tree with that height using asterisks (*).
For example, if you call draw_tree(5, 1)
, it will print a Christmas tree with a height of 5:
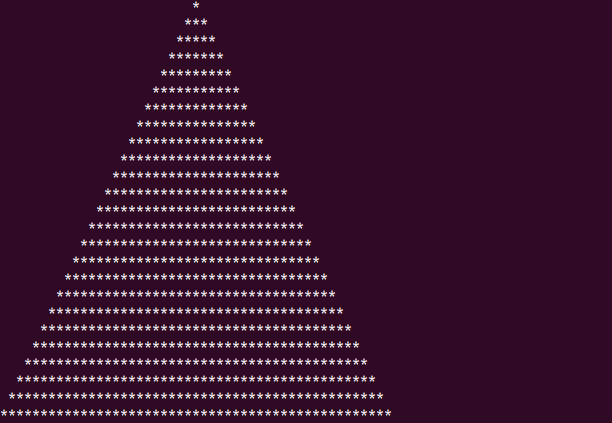
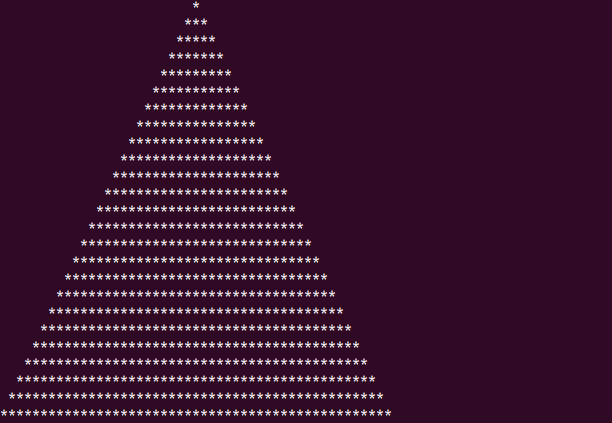
To clarify, if you set the level parameter to something else than 1, you will end up with a truncated Christmas tree. For example, calling draw_tree(15, 3) gives a result like this:
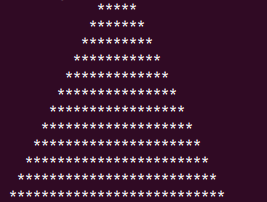
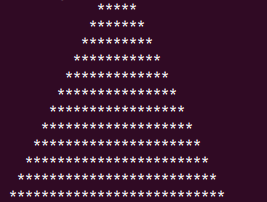
Summary
Today you learned how to draw a Christmas tree in Python by using asterisks (*).
To take home, there are many ways you can draw a Christmas tree. The critical point is it’s all about iteration. You have to create a loop (for, while, or recursive) that increases the number of asterisks (and spaces) the further you go. This gives rise to a shape that resembles a Christmas tree.
Thanks for reading. Happy coding!