To create a diamond pattern in Python using a for loop, use this simple piece of code:
h = eval(input("Enter diamond's height: ")) for x in range(h): print(" " * (h - x), "*" * (2*x + 1)) for x in range(h - 2, -1, -1): print(" " * (h - x), "*" * (2*x + 1))
Then run the program. For example, here is a diamond output of height 7
:
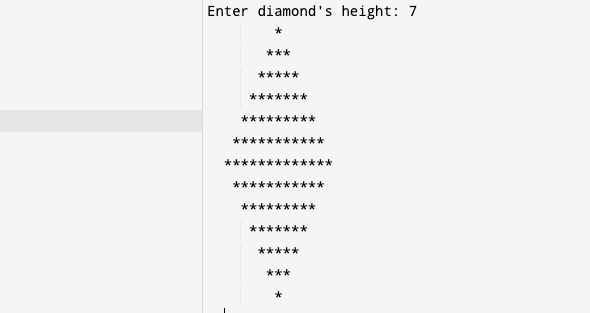
How Does It Work
The diamond pattern using for loop in Python is a common beginner question in Python courses. Make sure you understand how it works.
Two Loops Construct the Diamond
Then there are two for loops that construct the diamond.
The first loop prints the “upper half” of the diamond.
For example, if the height of the diamond is 7, the upper loop prints the lines 1,2,3,4,5,6,7
read from the top:
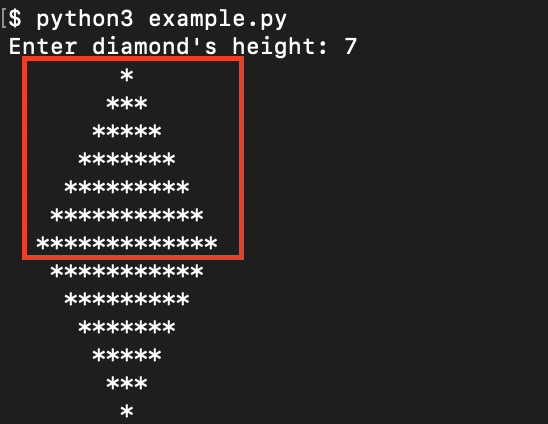
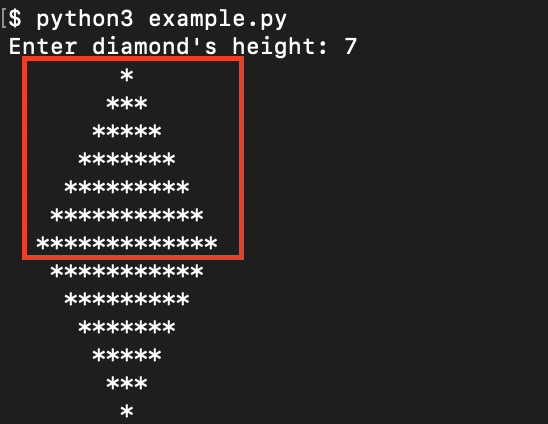
The second for loop prints the “lower half” of the diamond.
For example, if the height of the diamond is 7
, the lower loop prints the lines 6,5,4,3,2,1
starting from the end of the top half of the diamond:
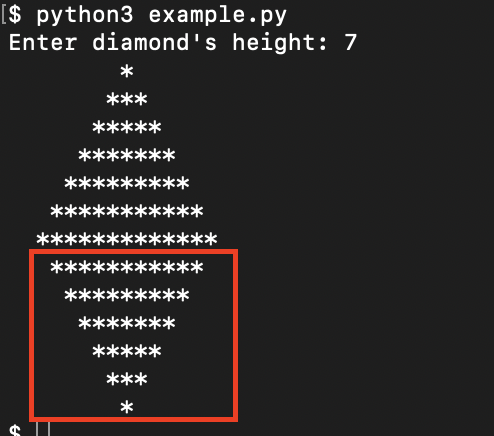
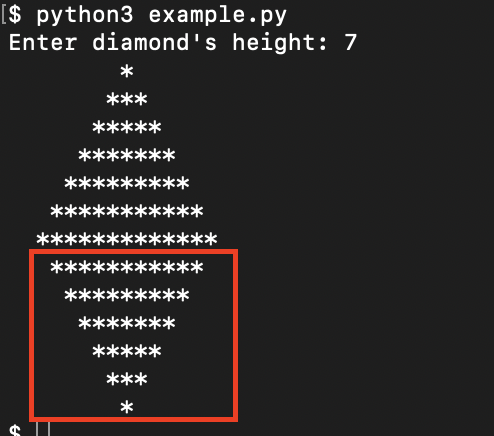
The Number of Asterisks and White Spaces
Now that you understand why there are two separate for loops in the code, let’s take a look at what they do.
The problem to solve is to get the number of asterisks (*) and white spaces right for each line.
The Upper Half of the Diamond
If you look at the diamond, in the upper half, the number of asterisks grows by 1, 3, 5, 7...
So if x
is the row number of the diamond starting from the top, 2x+1
is the number of asterisks in that row.
To compensate for the increase in asterisks, the number of blank spaces on the left must decrease by one the further we go. Thus the number of white spaces is h-x
. (No white spaces are printed on the right!)
Thus, for each row, you want to print h-x
white spaces and 2x+1
asterisks, where x is the height that goes from 0 to height – 1.
for x in range(h): print(" " * (h - x), "*" * (2*x + 1))
The Lower Half of the Diamond
In the lower half, the number of asterisks decreases by ..., 7, 5, 3, 1
In other words, if x is the row number of the diamond starting from the middle of the diamond, 2x+1 is the number of asterisks in that row.
To compensate for the decrease in asterisks, the number of blank spaces on the left must increase by one the lower we go. So the number of white spaces is h-x
.
Thus, for each row, you want to print h-x
white spaces and 2x+1
asterisks, where x is the height that goes from height – 2 to 0.
for x in range(h - 2, -1, -1): print(" " * (h - x), "*" * (2*x + 1))
Here the range(h-2, -1, -1)
is a reversed range.
- The first parameter h – 2 is the starting value for the range.
- The second parameter -1 specifies until which number the range continues (stops at 0 when it is -1).
- The third parameter -1 specifies the step size. With -1 the range goes from larger number to smaller.
Conclusion
Today you learned how to write a Python program that displays a diamond of height h in the console.
To recap, you need two loops for the diamond.
- One loop prints the top of the diamond and the other prints the bottom.
- The number of asterisks is
2x+1
, wherex
is the row number. The number of white spaces on the left ish-x
whereh
is the height andx
is the row number.
Thanks for reading. I hope you enjoy it.
Make sure you check out my list of must-have resources for software development.
5+ Useful Tools and Resources for Developers (Updated 2023)
Happy coding!