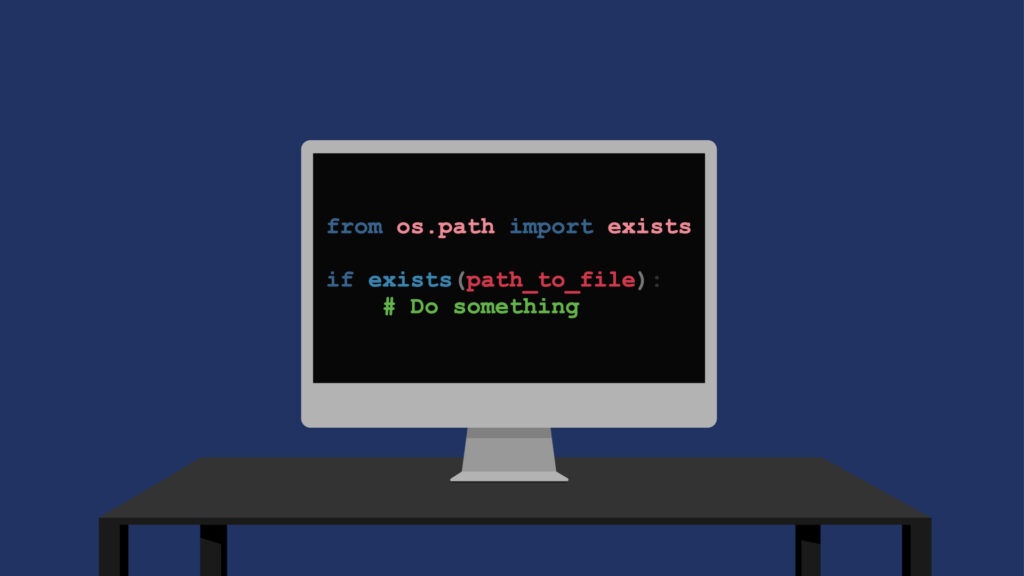
To check if a file exists using Python, use the exists()
function from the os.path
module:
from os.path import exists if exists(path_to_file): # Do something
Here is a short illustration of checking if a file exists in the same folder:
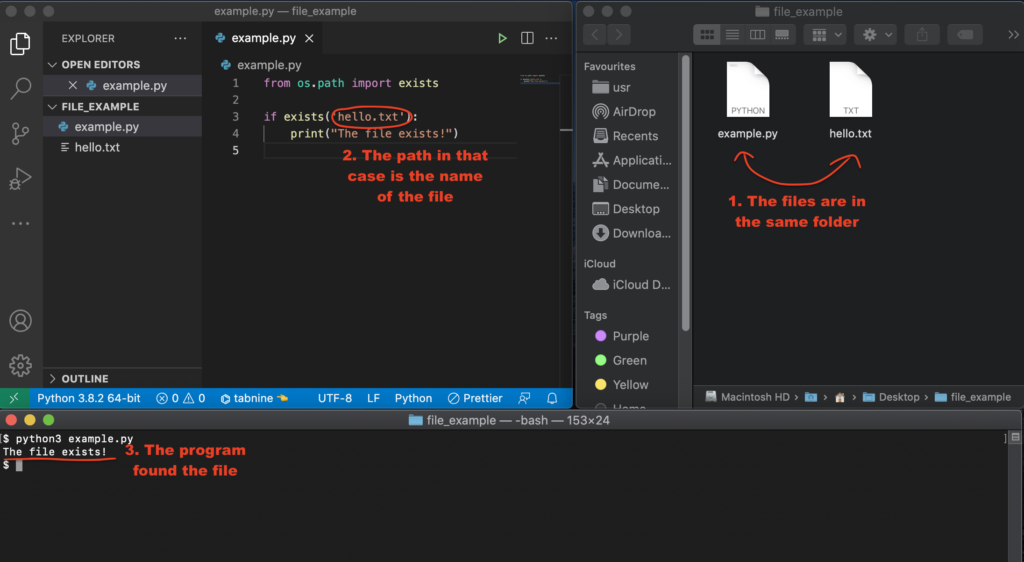
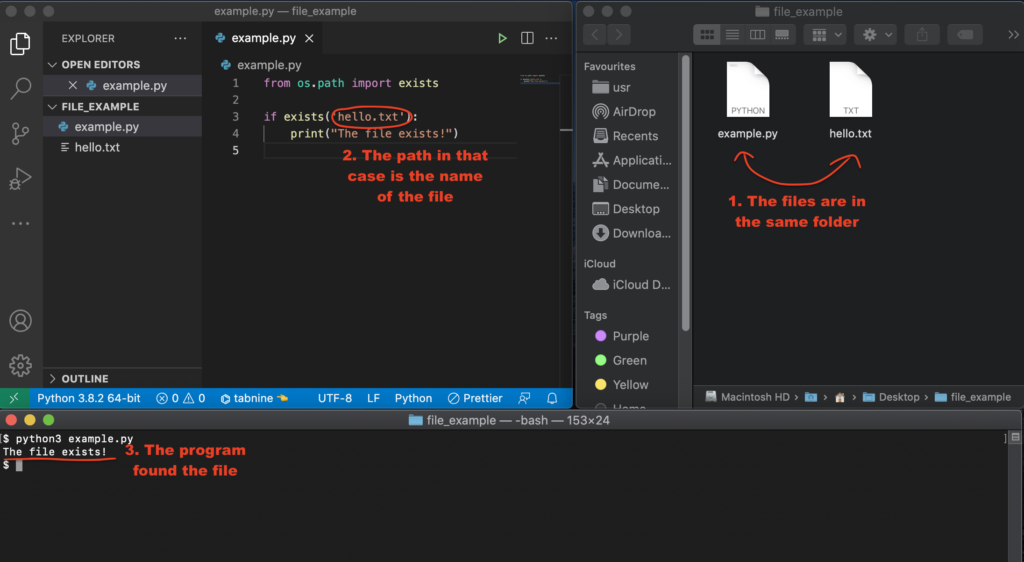
Alternatively, you can use the is_file()
function from the Path
class from the pathlib
module:
from pathlib import Path path = Path(path_to_file) if path.is_file(): # Do something
Where path_to_file
is relative to the Python file that checks the existence of the file.
For example, if there’s a file called hello.txt in the same folder as this Python program, the path_to_file
is the name of the file.
Check If a File Exists—A Step-by-Step Guide
Before you start working with a file, you may want to check if one exists. To do this you can use the exists()
function from os.path
module.
Step 1. Include the os.path Module
The os.path
module is is useful when processing files from different places in the file system of your program.
Make sure you import the os.path
module before trying to use it:
import os.path
Step 2. Call os.path.exists() Function
After including the os.path module, call the exists()
function. Remember to pass it the name or path of the file as an argument:
os.path.exists(path_to_file)
Where path_to_file
is the path to the file you are seeking (relative to the Python file’s path). If the file is in the same folder as your Python program, the path is just the name of the file.
If the file exists, the exists()
function returns True
. Otherwise, it returns False
.
The following example program uses the exists()
function to check if the hello.txt
file exists:
import os.path file_found = os.path.exists('hello.txt') print(file_found)
If the hello.txt
file exists, you’ll see the following output:
True
Step 3. (Optional) Make the Call Shorter
To make the call to the exists()
function shorter, import the function from the module:
from os.path import exists exists('hello.txt')
And here is an example where the file is in another folder called some_files
:
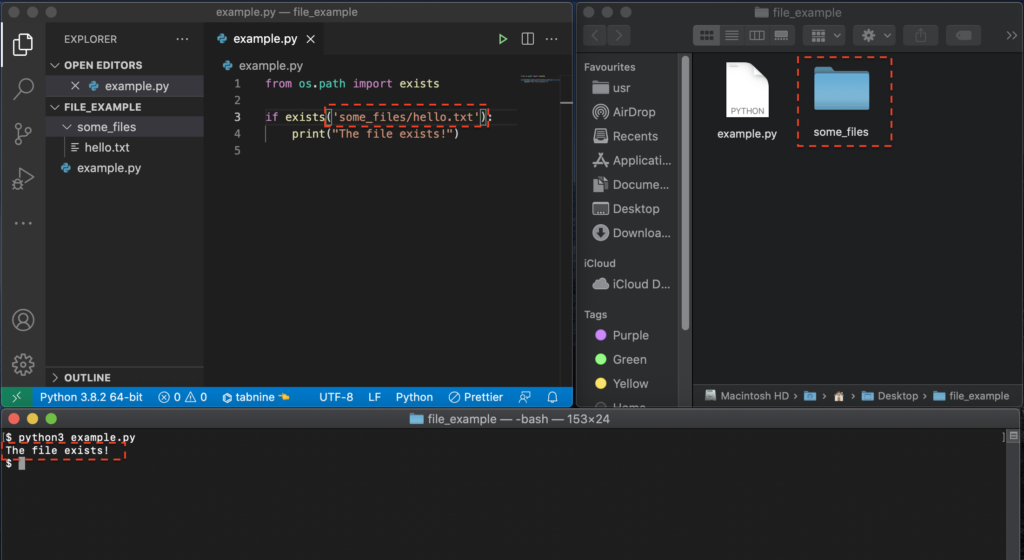
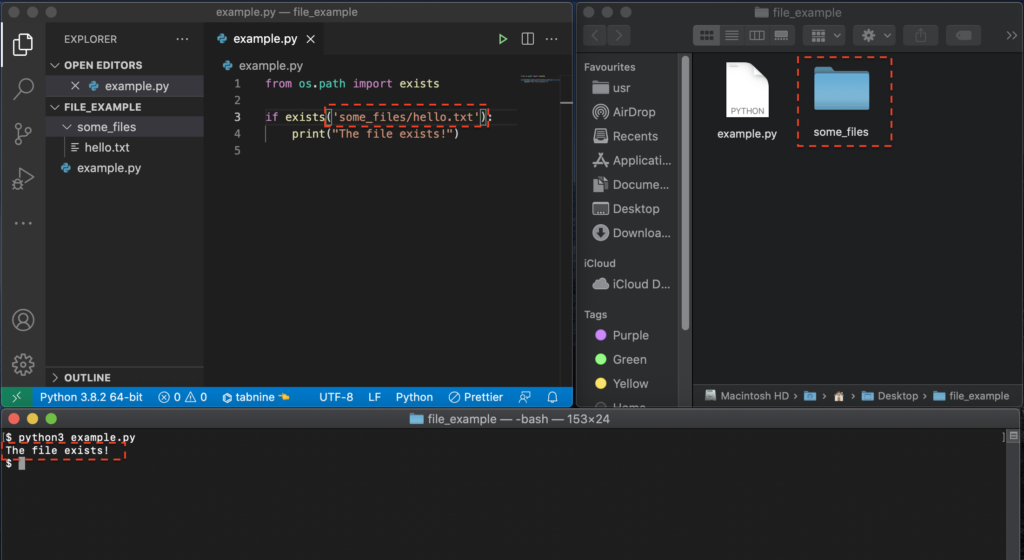
Here you need to provide the path to the file to find out if it exists. Because the folder where the file is, is in the same folder as the Python program, its path is:
some_files/hello.txt
Now, this is how you can use the os module to check if a file exists in Python. But there’s an alternative approach by using the relatively new pathlib module.
Check If a File Exists Using ‘Pathlib’
The pathlib
module lets you manipulate files and folders.
To use it, import the Path
class from the pathlib
module:
from pathlib import Path
Then, create a Path
object by initializing it with the file path whose existence is the point of interest:
path = Path(path_to_file)
Now, check if the file exists using the is_file()
method. This essentially tests if the object you created is a valid file.
path.is_file()
For instance, let’s use this approach to see if the hello.txt
file exists:
from pathlib import Path path_to_file = 'hello.txt' path = Path(path_to_file) if path.is_file(): print(f'{path_to_file} exists') else: print(f'{path_to_file} does not exist')
If the hello.txt
file exists, you’ll see the following output:
hello.txt exists
Conclusion
Today you learned how to check if a file exists in Python.
To take home, you can use the exists() method from the os.path library. To use this method, all you need to do is import the exists() method from os.path module and call the method by passing a file path as an argument.
Thanks for reading!