If you need anything more complex than the basic arithmetic operators in Python, there’s a built-in library called Math
you can use.
With this module, you can calculate square roots, medians, distances, and such.
This guide teaches you how to start using the math module in Python.
To start using the module in your project, you need to import it first.
import math
Common Math Module Operations in Python
Some common math
module operations you are going to see a lot are:
- Square root with
sqrt
. - Rounding down with
floor
. - Rounding up with
ceil
.
Let’s go through each of these.
How to Find the Square Root of a Number in Python
Square root answers the question: “What number squared gives this number?”.
In Python, you can find the square root of a number using the math
module’s sqrt()
function.
For example:
number = 16 square_rooted = math.sqrt(number) print(square_rooted)
Output:
4.0
How to Round Numbers in Python
To round numbers in Python, use the built-in round()
function. (This is not part of the math
module, but it fits well here, because of the following sections.)
The round
function takes two arguments:
- The decimal number to be rounded.
- The number of desired decimal places.
For example, let’s round a decimal to two decimal places:
x = round(5.76543, 2) print(x)
Output:
5.77
How to Round Numbers Down in Python
To round a number down to its nearest integer value, use the math
module’s floor()
function.
For example:
number = 3.9 floored = math.floor(number) print(floored)
Output:
3
How to Round Numbers Up in Python
To round a number up to its nearest integer value, use the math
module’s ceil()
function.
For example:
number = 3.1 ceiled = math.ceil(number) print(ceiled)
Output:
4
Trigonometry with the Math Module in Python
The math
module is useful because in addition to the basic operations you saw above, you can do basic trigonometry with it. These involve dealing with angles and distances.
Pi Constant in Python
Pi is probably the most famous number in mathematics. It is the ratio of the circumference of any circle to the diameter of that circle.
You can access the value of π via the math
module:
pi = math.pi print(pi)
Output:
3.141592653589793
How to Calculate Distance in Python
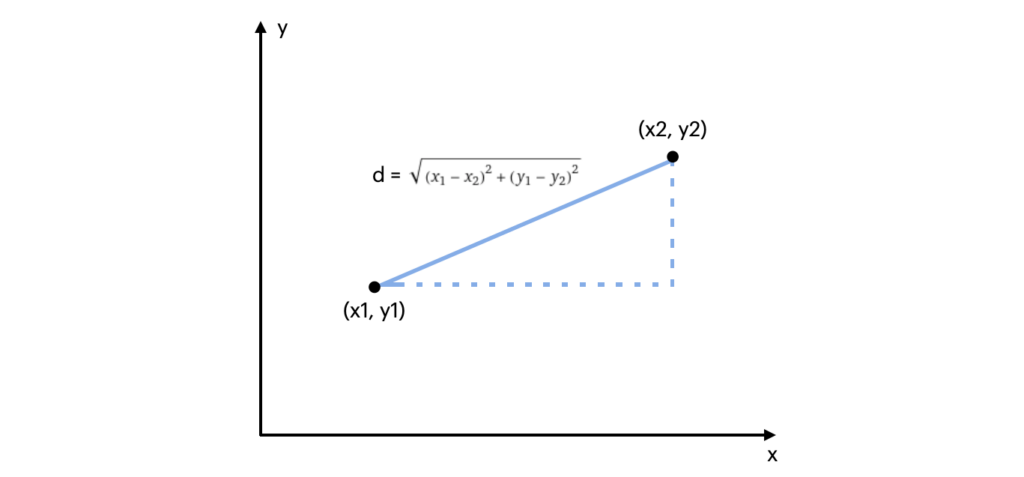
To calculate the (Euclidian) distance between two points, use the math.dist()
function.
For example, let’s calculate the distance between origin and point (1, 1)
in a 2D coordinate system:
origin = (0, 0) p1 = (1, 1) distance = math.dist(origin, p1) print(distance)
Output:
1.4142135623730951
Notice you can compute the distance in any other dimension too.
How to Convert Degrees to Radians in Python
To convert degrees to radians, use the math.degrees()
function.
For example, let’s convert 2π radians to degrees:
rads = 2 * math.pi degs = math.degrees(rads) print(degs)
Output:
360.0
How to Convert Radians to Degrees in Python
To convert radians to degrees, use the math.radians()
function.
For example, let’s convert 180 degrees to radians:
degs = 180.0 rads = math.radians(degs) print(rads)
Output:
3.141592653589793
(Which is equal to π.)
Sin in Python
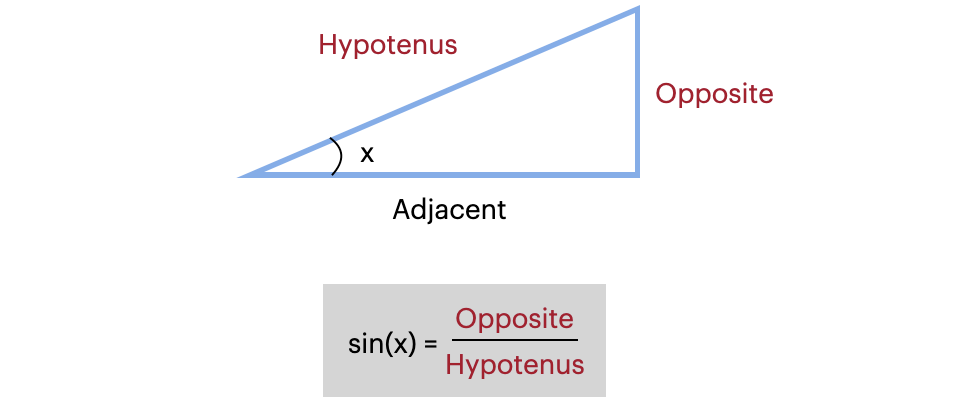
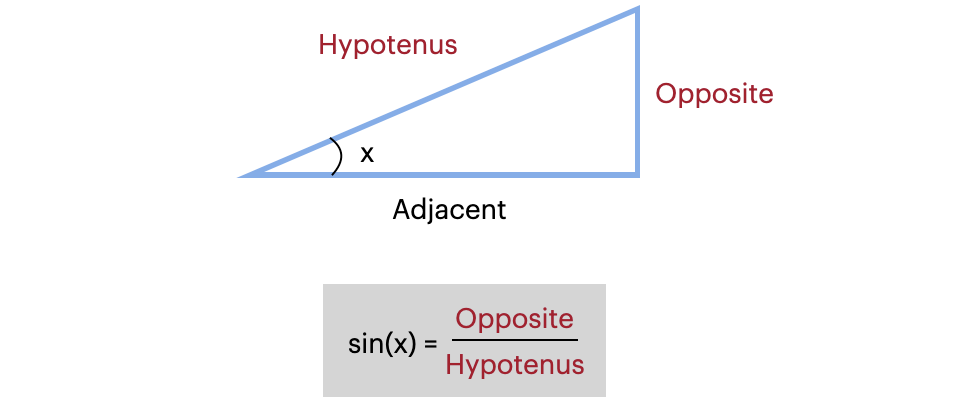
To calculate the sine of an angle, use the math.sin()
function. Notice this function assumes your angle is in radians. If you’re using degrees, remember to convert the degrees to radians with math.radians()
function.
For example:
degs = 45.0 rads = math.radians(degs) print(math.sin(rads))
Output:
0.7071067811865475
Arc Sin in Python
To get an angle given a fraction, you can use the math.asin()
function.
For example:
ratio = 0.7071067811865475 rads = math.asin(ratio) degrees = math.degrees(rads) print(degrees)
Output:
44.99999999999
This value should be exactly 45, but due to floating-point accuracy, you might see a value really close to that.
Cos in Python
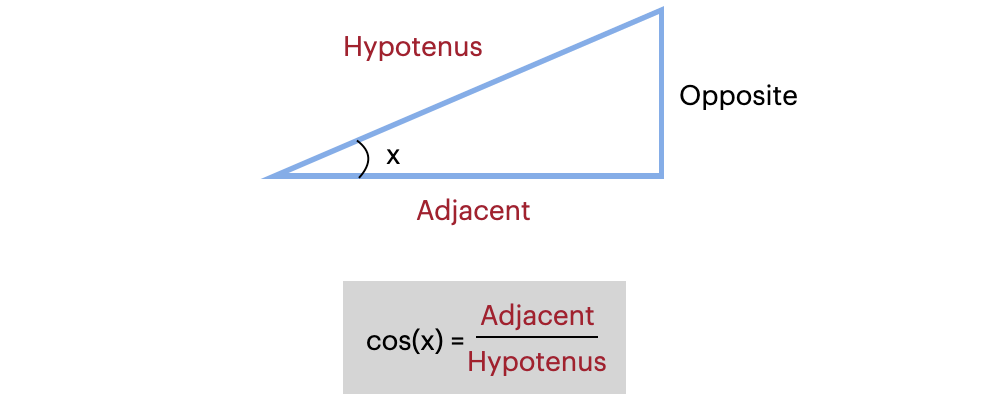
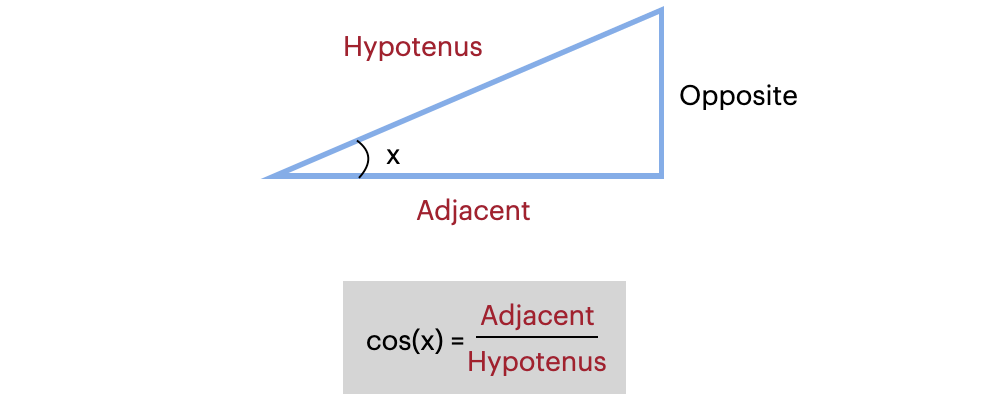
To calculate the cosine of an angle, use the math.cos()
function.
This function assumes your angle is in radians. If you’re using degrees, remember to convert the degrees to radians with math.radians()
function.
For example:
degs = 30.0 rads = math.radians(degs) print(math.cos(rads))
Output:
0.8660254037844387
Arc Cos in Python
To get an angle given a fraction, you can use the math.acos()
function.
For example:
ratio = 0.8660254037844387 rads = math.acos(ratio) degrees = math.degrees(rads) print(degrees)
Output:
29.999999999999996
This value should be exactly 30, but due to floating-point accuracy, you might see a value really close to that.
Tan in Python
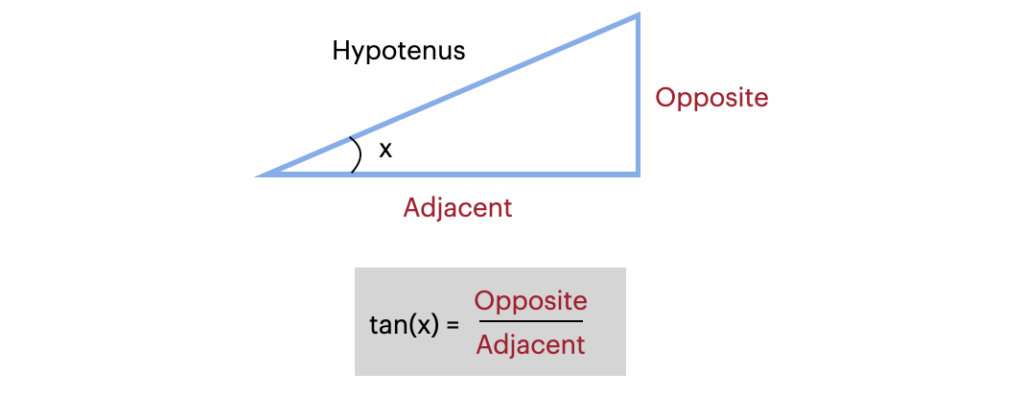
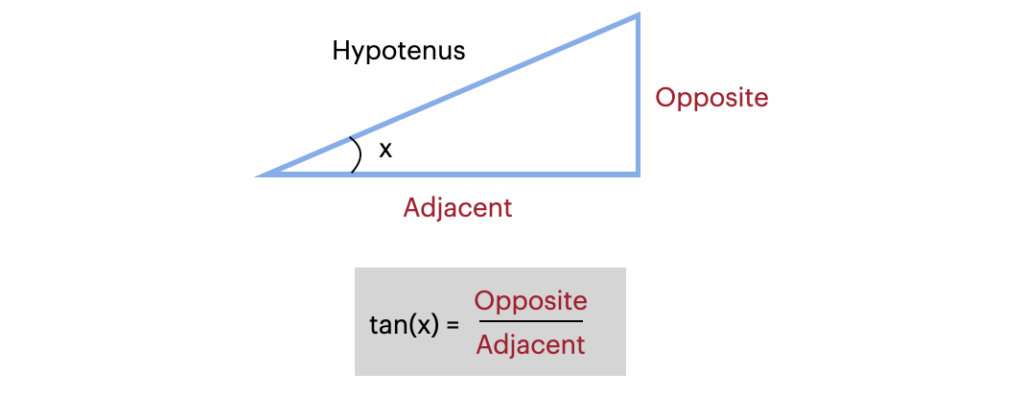
To calculate the tangent of an angle, use the math.tan()
function.
This function assumes your angle is in radians. If you’re using degrees, remember to convert the degrees to radians with math.radians()
function.
For example:
degs = 75.0 rads = math.radians(degs) print(math.tan(rads))
Output:
3.7320508075688776
Arc Tan in Python
To get an angle given a fraction, you can use the math.atan()
function.
For example:
ratio = 3.7320508075688776 rads = math.atan(ratio) degrees = math.degrees(rads) print(degrees)
Output:
75.0
Conclusion
Now you know the basics of maths with Python.
Python natively supports 7 arithmetic operations: addition, subtraction, multiplication, division, floor division, exponentiation, and modulus.
To perform more common maths operations in Python, use the math
module by importing it to your project.
Thanks for reading. I hope you find it useful.
Happy coding.