To log colored text to the JavaScript console, call the console.log
method and include special formatting code %c
to colorize the log and pass the colorizing CSS code as the second argument.
For example, to log text in red, you can use the %c
formatting code, followed by the desired text and a CSS color
property setting the text color to red:
console.log('%cThis text is red!', 'color: red');
This is what it looks like in the JS console:
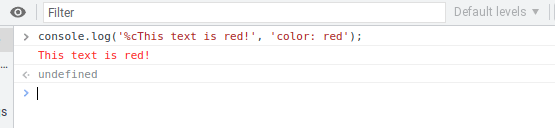
You can also use the %c
formatting code to set other CSS properties, such as the font size or font family:
console.log('%cThis text is red and has a larger font!', 'color: red; font-size: larger');
Output:
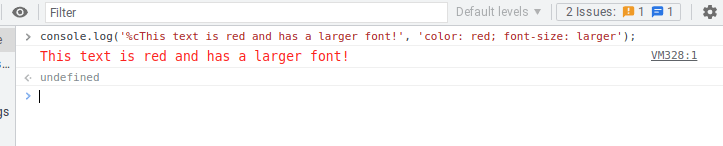
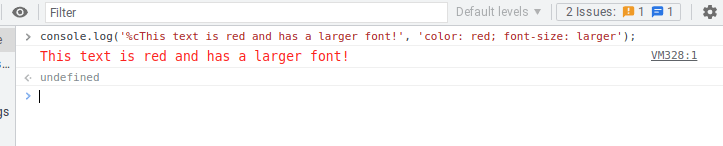
Remember that this formatting is only supported in modern browsers such as Chrome.
More console.log Color Tricks
Now that you understand how you can use CSS to format your console log function calls, let’s see other cool examples you can implement.
Change the Background of the Logged Message
To change the background color of the text logged to the JavaScript console, you can use the %c
formatting code, followed by the desired text and a CSS background-color
property setting the background color to the desired value:
console.log('%cThis text has a yellow background!', 'background-color: yellow');
Output:
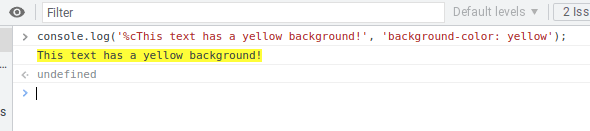
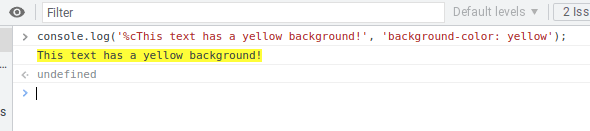
You can also set other CSS properties using the %c
formatting code, such as the font size or font family:
console.log('%cThis text has a yellow background and a larger font!', 'background-color: yellow; font-size: larger');
Output:


Keep in mind that this formatting is only supported in modern browsers. If you need to support older browsers, you may need to use a different approach.
How to Add Multiple Colors on the Same Line
To add multiple colors to the same line when logging a message to the JavaScript console, you can use multiple instances of the %c
formatting code and specify the CSS properties for each segment of text.
For example, the following code will log a message with red text followed by green text:
console.log('%cThis text is red%cThis text is green', 'color: red', 'color: green');
Output:
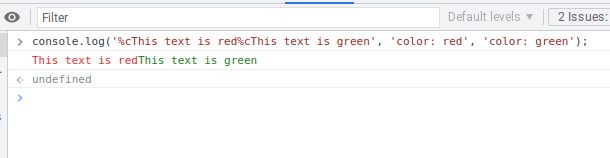
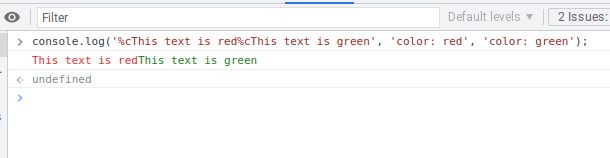
How to Add a Shadow to Console Log
Thus far you’ve seen how to add colors to console logs in JavaScript. But why stop there? You can do a whole bunch of other things with CSS, so let’s play with it a bit more.
For example, let’s create a shadow for the output:
var css = "text-shadow: 1px 1px 2px black, 0 0 1em blue, 0 0 0.2em blue; font-size: 40px;" console.log("%cHello world", css);
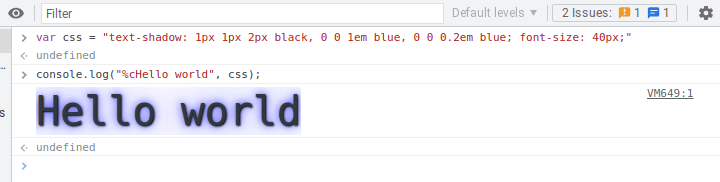
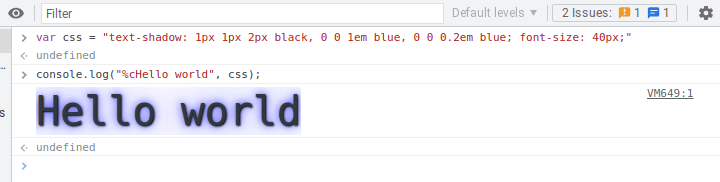
Thanks for reading. Happy coding!