To find all files with a particular extension in Python, you can use the os.walk
function to traverse the directory structure, and check if each file has the desired extension using the file.endswith()
method.
For example, let’s find all the .txt
files:
import os for root, dirs, files in os.walk('/path/to/directory'): for file in files: if file.endswith('.txt'): print(os.path.join(root, file))
This will print the full path of all files with the .txt
extension in the specified directory. You can replace the.txt
with any other desired file extension.
If you’re looking for a quick answer, I’m sure the above will do!
But if you’re looking to improve your Python skills and want to learn how to recursively find files in a directory with subdirectories, keep on reading. This guide introduces you to 3 different methods you can use to find files of a particular type in Python.
Let’s jump into it!
3 Ways to Find All Files by Extension in Python
There are three main ways to find all the files by extension in Python.
- The ‘glob’ Module
- The ‘os.listdir’ Function
- The ‘os.walk’ Function
Let’s take a closer look at how each of these approaches works.
1. The ‘glob’ Module
You can use the glob module in Python to find all files with the .txt extension using the following code:
import glob # Find all files with the .txt extension in the current directory txt_files = glob.glob('*.txt') # Print the names of the files found print(txt_files)
The glob.glob()
function searches for all files in the current directory that match the specified pattern, which in this case is '*.txt'
. This tells the function to find all files with a name that ends in .txt
. The result is a list of the names of the matching files.
You can also specify a different directory to search in by passing the path to the directory as the first argument to the glob.glob()
function. For example, if you want to search for all .txt
files in the /path/to/directory
directory, run the following:
import glob # Find all files with the .txt extension in the specified directory txt_files = glob.glob('/path/to/directory/*.txt') # Print the names of the files found print(txt_files)
Example
Here’s an example project I have:
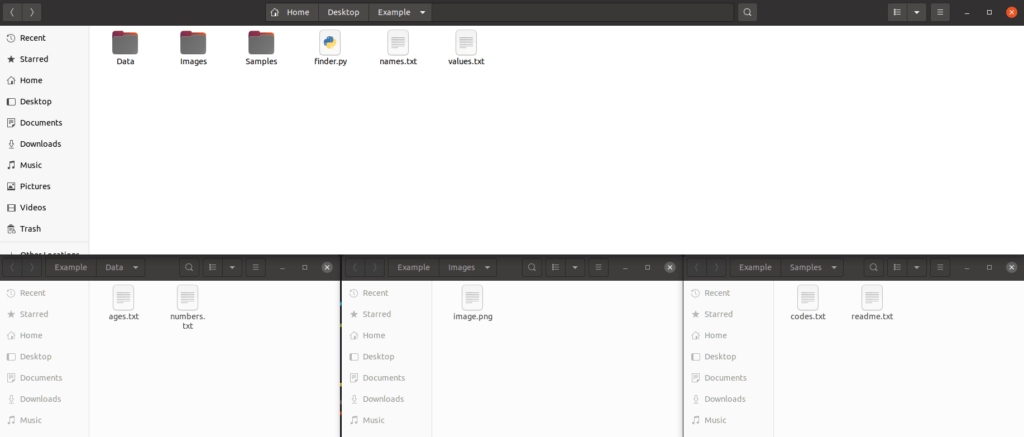
The above image shows the folder structure, but in case you need clarity, here’s what it looks like:
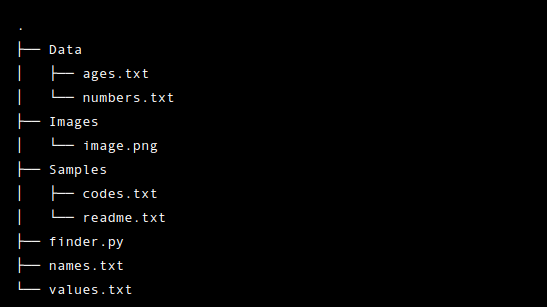
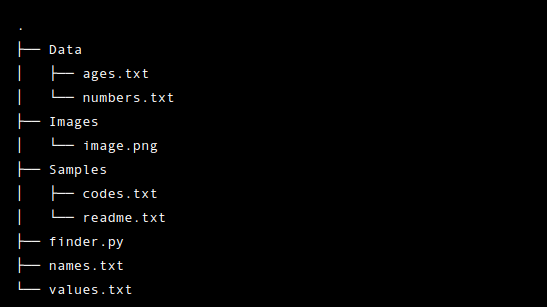
Let’s find all the text files (ending with ‘.txt’) in the folder.
To do this, let’s run the code from the previous section to see what happens:
import glob # Find all files with the .txt extension in the current directory txt_files = glob.glob('*.txt') # Print the names of the files found print(txt_files)
Output:
['names.txt', 'values.txt']
It returns the ‘names.txt
‘ and ‘values.txt
‘ files but not the text files in the subfolders. To list the files with a particular extension in the subfolders, you need to take a recursive approach.
2. Recursive Search with ‘os.listdir()’
As you saw in the earlier example, the glob.glob()
function only searches for files in the specified directory and does not search in any of its subdirectories.
To search for files with a particular extension in the specified directory and all its subdirectories, you can use the os.listdir()
function in a recursive manner.
Here’s an example implementation:
import os def find_files(dir_path, extension): # Check if the given path is a valid directory if not os.path.isdir(dir_path): return # Get a list of all the files and directories in the given directory files_and_dirs = os.listdir(dir_path) # Iterate over the list of files and directories for file_or_dir in files_and_dirs: # Construct the full path of the file or directory full_path = os.path.join(dir_path, file_or_dir) # If the full path is a directory, recursively call the function to find the files in that directory if os.path.isdir(full_path): find_files(full_path, extension) else: # If the full path is a file that ends with extension, print its path if full_path.endswith(extension): print(full_path)
This code defines a recursive function called find_files()
that takes a directory path as its argument and searches for files in that directory and all its subdirectories.
It uses the os.listdir()
function to get the names of all files and directories in the specified directory, and then uses the os.path.isdir()
function to check if a given file or directory is a directory.
- If it is a directory, the function calls itself recursively to search for files with a specific extension in that directory.
- If it is a file, the function checks the extension and prints the file name out if it ends with the target extension.
Let’s call this function to see it in action.
Example
Let’s continue with the Example project folder with the following structure:
. ├── Data │ └── ages.txt │ └── numbers.txt ├── Images │ └── image.png ├── Samples │ └── codes.txt │ └── readme.txt ├── finder.py ├── names.txt └── values.txt
Our goal is to find all the .txt
files in the entire folder (including the subfolders) by running a script in the finder.py
file.
To do this, let’s copy the find_files
function from the previous section to the finder.py
file.
Let’s call the function by setting the current directory and the .txt
as parameters.
find_files('.', '.txt')
Output:
./Data/numbers.txt ./Data/ages.txt ./Samples/readme.txt ./Samples/codes.txt ./names.txt ./values.txt
Awesome! This time the function not only found the text files at the same level as the python file but also the text files in the subfolders.
Even though this example offers great practice with recursion in Python, there’s an easier alternative for finding the files.
3. The ‘os.walk’ Function
The easiest way to recursively find all the files with a particular extension in a folder and its subfolders is by using the os.walk
function.
Here’s what calling it might look like
import os for root, dirs, files in os.walk('/path/to/directory'): for file in files: if file.endswith('.someextension'): print(os.path.join(root, file))
This code imports the os
module, which provides functions for interacting with the operating system. It then uses the os.walk()
function to recursively iterate through all the directories and subdirectories within the specified directory (‘/path/to/director
y’).
For each directory in the tree, it sets three variables: root, dirs, and files. The root
variable contains the path to the current directory, dirs
is a list of subdirectories within that directory, and files
is a list of files in the current directory.
It then loops through the list of files in the current directory and checks if each file ends with the specified extension. If it does, it prints the full path to that file using the os.path.join()
function. This allows it to find all the files with the specified extension within the entire directory tree, not just the current directory.
This is a convenient replacement for the rather lengthy recursion approach from the earlier section.
Example
Let’s continue with the project structure you’ve already seen in this guide.
. ├── Data │ └── ages.txt │ └── numbers.txt ├── Images │ └── image.png ├── Samples │ └── codes.txt │ └── readme.txt ├── finder.py ├── names.txt └── values.txt
In particular, let’s find all the .txt files from the entire folder and its subfolders.
To do this, let’s modify the previously introduced code to target the current folder and to only show files with .txt
extension:
import os for root, dirs, files in os.walk('.'): for file in files: if file.endswith('.txt'): print(os.path.join(root, file))
Running finder.py
with the above code shows all the files with .txt
extension:
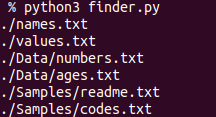
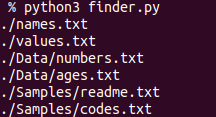
Summary
Today you learned how to find files with a specific extension in Python.
To take home, use the os.walk()
function to recursively walk through folders and subfolders to find all the files with a particular extension.
Thanks for reading. Happy coding!