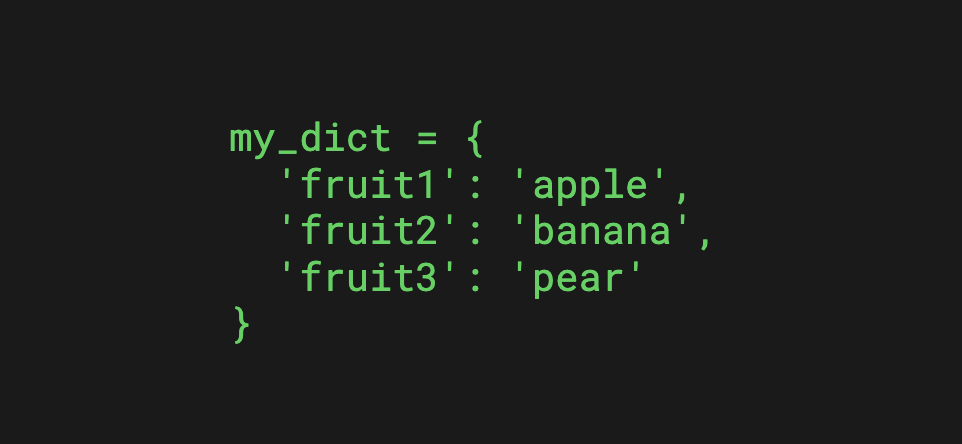
Python dictionaries are a data type that allows for the storage and retrieval of key-value pairs. They are useful because they provide an efficient way to access and modify data, allowing for quick and easy data manipulation. Additionally, dictionaries can be nested and combined with other data types, making them versatile and powerful tools for data analysis and manipulation.
For some beginners, Python dictionaries may be challenging to understand at first because they involve using keys and values instead of indices like lists do. However, with practice and guidance, most beginners can learn to use Python dictionaries effectively.
This is a comprehensive guide to Python dictionaries.
You will learn how to create and maintain dictionaries with operations and built-in methods. Besides, you will see countless examples to support your understanding of dictionaries and their use.
Let’s jump into it!
How to Define a Dictionary in Python
A Python dictionary is a collection of key-value pairs. It is defined using curly braces {} in which you place key-value pairs such that each key-value pair is separated by a comma.
The best way to understand this is by having a look at some examples.
Example 1
Let’s create a dictionary called my_dict
and place some data in it:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'}
In this example, ‘name’, ‘age’, and ‘city’ are the keys, and ‘John’, 25, and ‘New York’ are their respective values.
Example 2
Let’s create another Python dictionary for demonstration’s sake.
my_dict = {'fruit1': 'apple', 'fruit2': 'banana', 'fruit3': 'pear'}
In this example, the keys are ‘fruit1’, ‘fruit2’, and ‘fruit3’ and their corresponding values are ‘apple’, ‘banana’, and ‘pear’.
Example 3
You can also create an empty dictionary in Python and start placing values in it with the square-bracket operator.
my_dict = {} my_dict['name'] = 'John' my_dict['age'] = 25 my_dict['city'] = 'New York' print(my_dict)
Output:
{'name': 'John', 'age': 25, 'city': 'New York'}
In this example, an empty dictionary is first defined and then key-value pairs are added to it using the square bracket notation. The name placed inside the square brackets specifies a new key and the assignment operator adds a new value to it.
Now that you’ve seen a bunch of examples of creating dictionaries in Python, let’s talk about how you can easily read values from a dictionary—the very reason that makes dictionaries so handy.
How to Access Dictionary Values
To access dictionary values in Python, you can use the square bracket notation and specify the key associated with the value you want to access.
For example, let’s say you have a dictionary called fruits
that has the following keys and values:
fruits = {"apple": 5,"banana": 3,"orange": 4,"grapes": 6}
To access the value associated with the key “apple”, you can use the following code:
value = fruits["apple"]
This retrieves the value 5 from the dictionary and assigns it to a variable called value
.
You can also use a for loop to iterate over all the keys and values in the dictionary and access each value individually:
for key, value in fruits.items(): print(key, ":", value)
This will print out each key and its associated value, separated by a colon.
Output:
apple: 5 banana: 3 orange: 4 grapes: 6
More about the dict.items()
method later on.
Dictionaries Use Keys Instead of Indexes
The key difference between a list and a dictionary in Python is that a list uses an index to access elements. On the other hand, a dictionary uses keys to access values.
Here’s a code example that illustrates the usage of keys instead of indices in Python dictionaries:
my_dict = { "name": "John Doe", "age": 30, "city": "New York" } # Access values using keys print(my_dict["name"]) # Output: John Doe print(my_dict["age"]) # Output: 30 print(my_dict["city"]) # Output: New York # Add new key-value pair my_dict["profession"] = "Software Developer" # Update existing key-value pair my_dict["city"] = "Boston" # Delete key-value pair del my_dict["age"] # Check if a key exists in the dictionary if "name" in my_dict: print("Key 'name' exists in the dictionary.")
As you can see, we access the values in a dictionary using keys, instead of using an index like in a list. We can also add, update, and delete key-value pairs using the keys, and check if a key exists in the dictionary using the in
keyword. This makes the dictionary a much more extensible and multi-purpose data structure than a list.
Dictionary Key Restrictions
Even though a Python dictionary is a flexible data type, there are some restrictions on the keys. For example, no two keys can be called the same.
Let’s take a closer look at the key restrictions in Python dictionaries.
1. Unique Keys
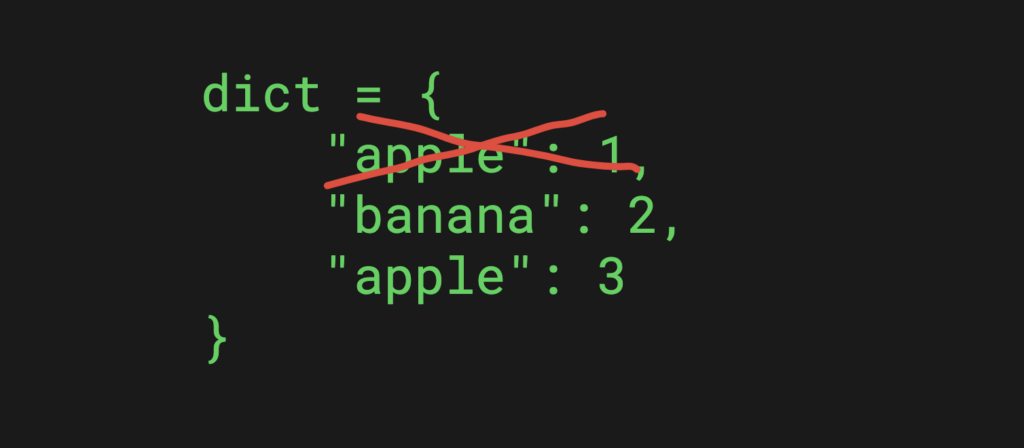
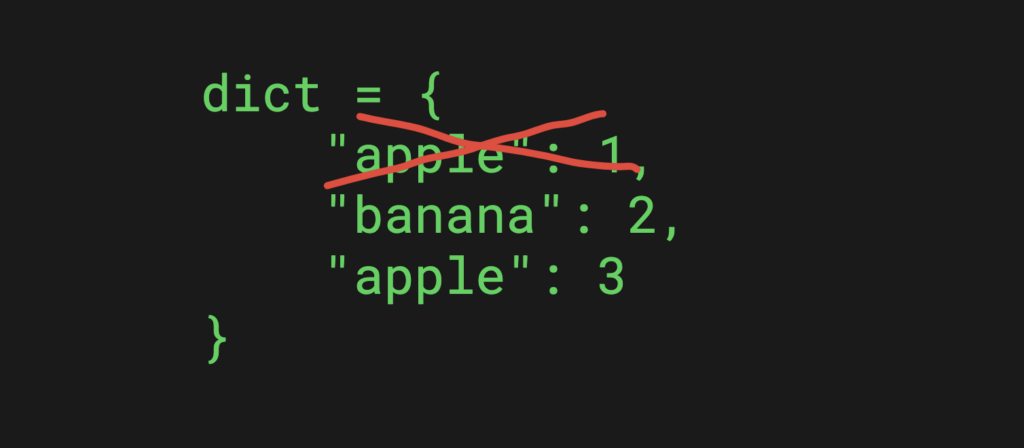
Keys must be unique within a dictionary. This means that no two keys can have the same value.
For example:
dict = { "apple": 1, "banana": 2, # This won't work because "apple" already exists as a key "apple": 3 }
This piece of code doesn’t throw an error. Instead, the second “apple” key-value pair is going to override the first key-value pair. You can see this by printing the dictionary:
print(dict)
Output:
{'apple': 3, 'banana': 2}
2. Immutable Keys
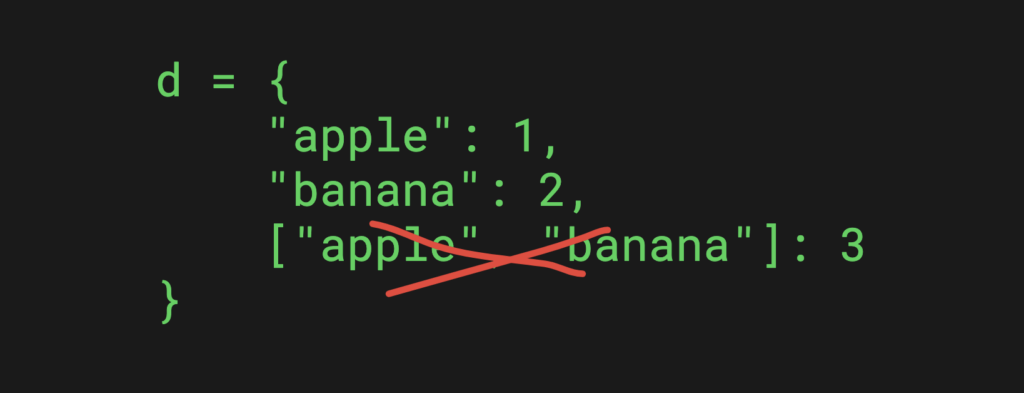
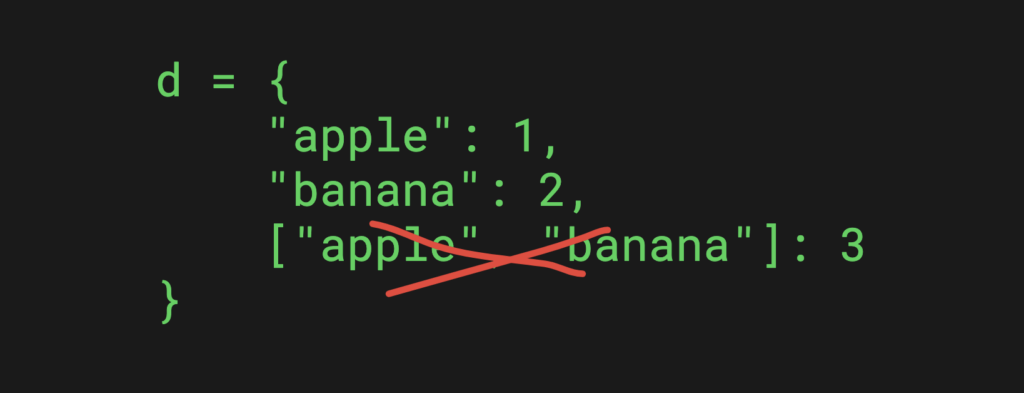
Dictionary keys must be immutable.
This means that the keys cannot be changed or modified once they are added to the dictionary.
For example:
d = { "apple": 1, "banana": 2, # This will throw an error because a list is not an immutable type ["apple", "banana"]: 3 }
Dictionary Value Restrictions
Python dictionary values have no restrictions. As long as the value is valid in Python, it’s ok to have one in a dictionary!
For example, you can assign a class, method, integer, string, or anything as a dictionary value in Python.
Python Dictionary Operators and Functions
One thing that makes Python dictionaries such a useful and extensible data type in Python is that you can do all sorts of operations on them.
Let’s take a closer look at some of the handiest operations you can perform on a Python dictionary.
The “[]” Operator
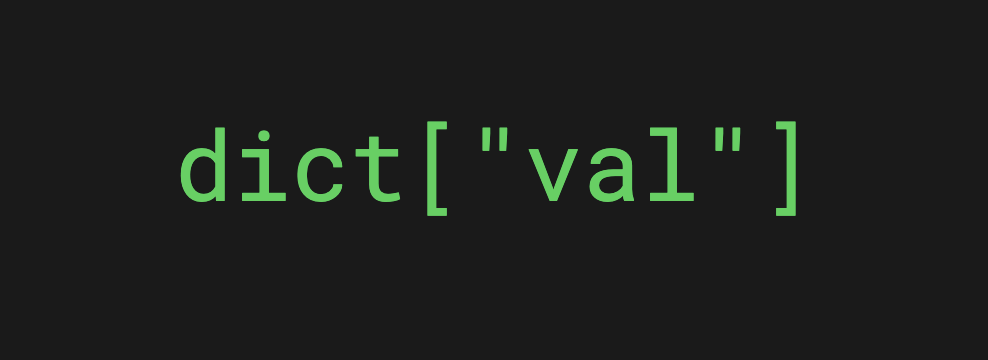
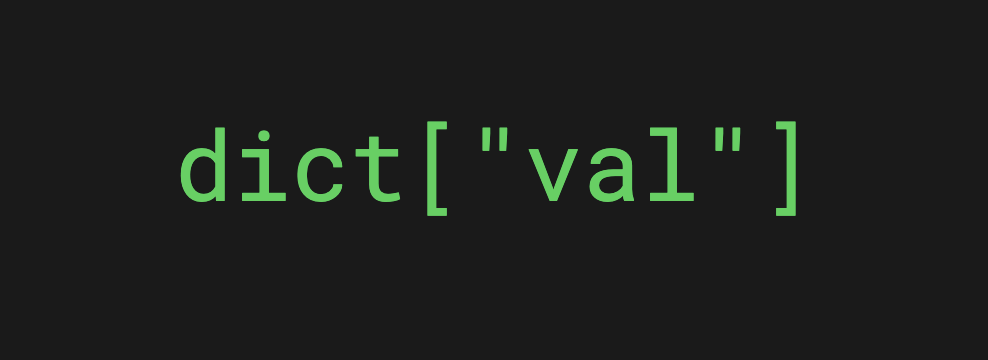
In Python, the “[]” operator gives access to the value of a given key in a dictionary.
For example, let’s read the “name” in an example dictionary:
my_dict = {"name": "John", "age": 20} print(my_dict["name"]) # Output: "John"
The “in” Operator
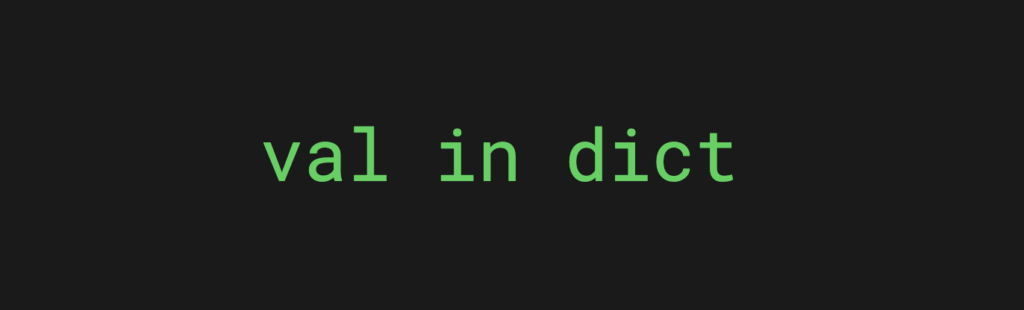
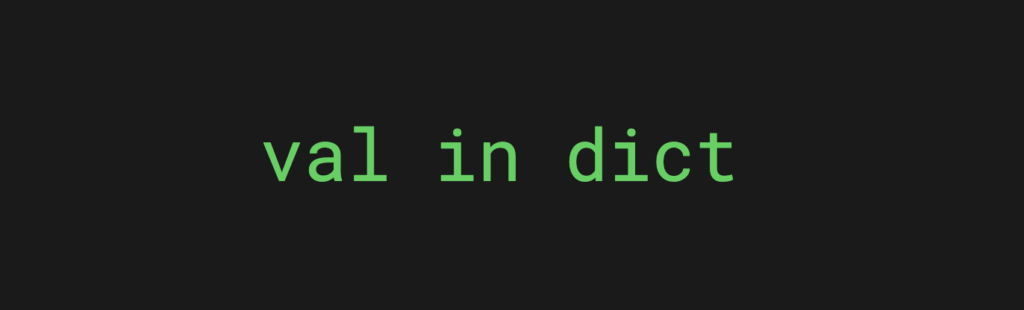
You can call the “in” operator on a Python dictionary. It checks if a given key exists in a dictionary
For example, let’s check if the “name” key exists in my_dict
:
my_dict = {"name": "John", "age": 20} if "name" in my_dict: print("Key 'name' exists in my_dict")
The “not in” Operator
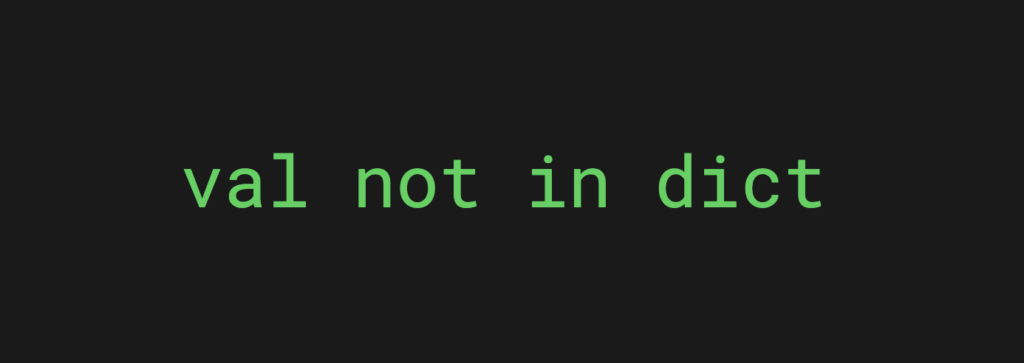
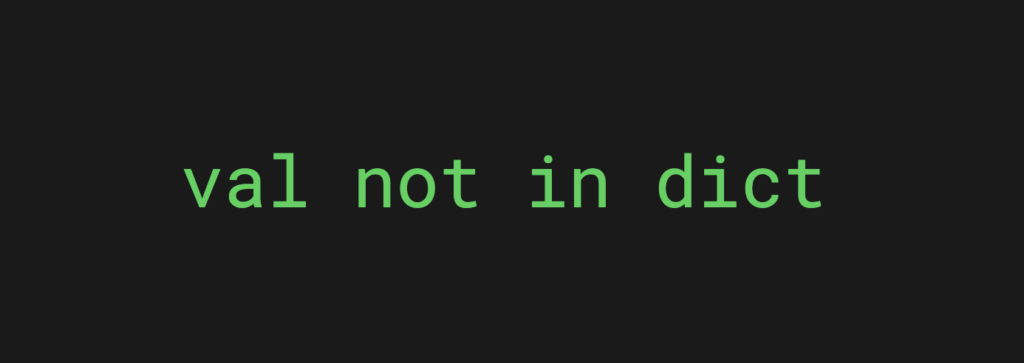
The “not in” operator is the opposite of the “in” operator. You can use it to check if a key does not exist in a dictionary.
For example, let’s check if the “email” field is not present in my_dict
:
my_dict = {"name": "John", "age": 20} if "email" not in my_dict: print("Key 'email' does not exist in my_dict")
The “del” Operator
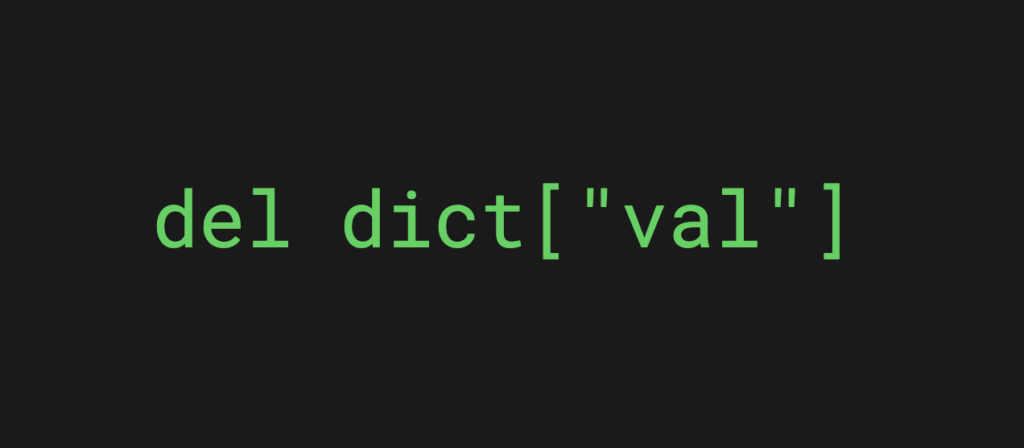
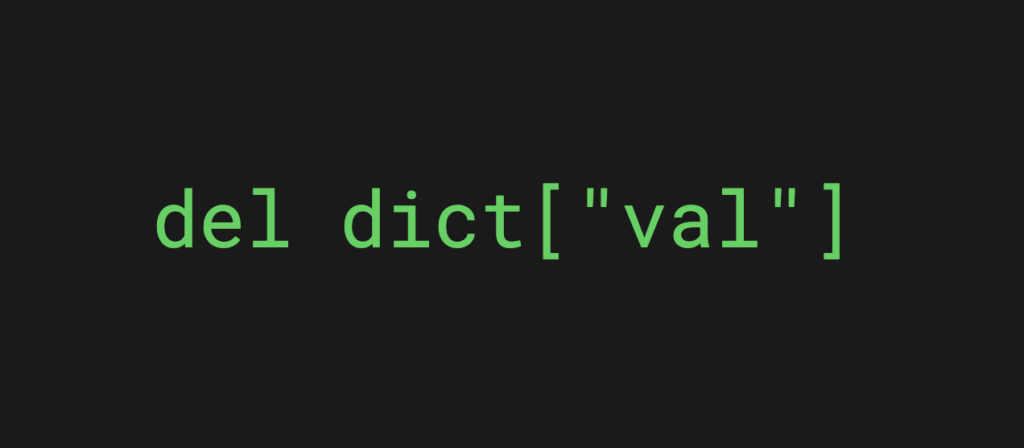
The “del” operator deletes a key-value pair from a dictionary.
For example, let’s remove the key-value pair with the key “name”:
my_dict = {"name": "John", "age": 20} del my_dict["name"] print(my_dict) # Output: {"age": 20}
The “+” Operator
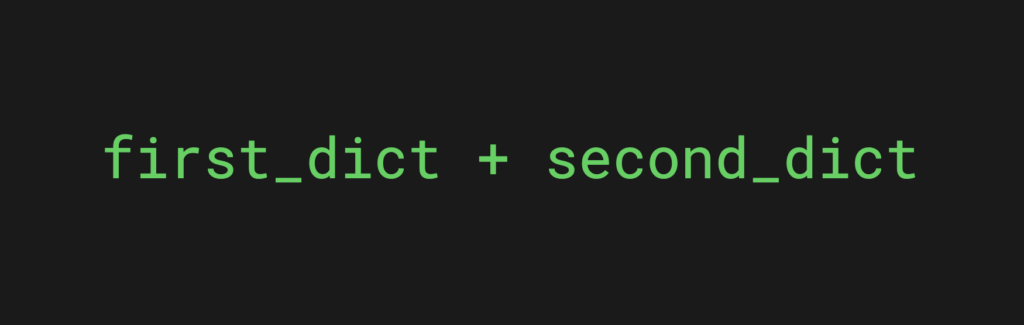
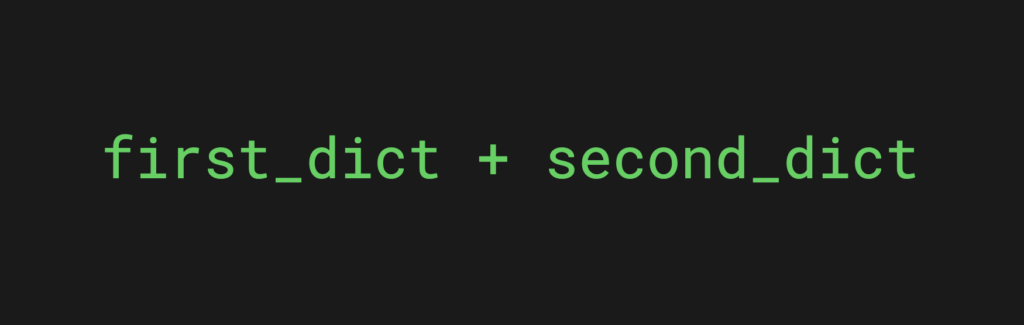
You can use the “+” operator to merge two dictionaries into a new dictionary.
For instance:
dict1 = {"name": "John", "age": 20} dict2 = {"email": "john@gmail.com", "phone": "555-555-5555"} dict3 = dict1 + dict2 print(dict3) # Output: {"name": "John", "age": 20, "email": "john@gmail.com", "phone": "555-555-5555"}
Now that you know about the most notable operations of dictionaries, let’s talk about the perhaps even more useful dictionary methods.
Dictionary Methods in Python
The dictionary data type comes with a whole bunch of useful built-in methods. With these methods, you can apply the most common operations on dictionaries without reinventing the logic yourself.
For example, you can safely access a dictionary value with the .get() method such that the program doesn’t crash if the key doesn’t exist.
This is just one example of the many useful methods there are.
Let’s take a closer look at some of the most useful dictionary methods in Python.
dict.clear()
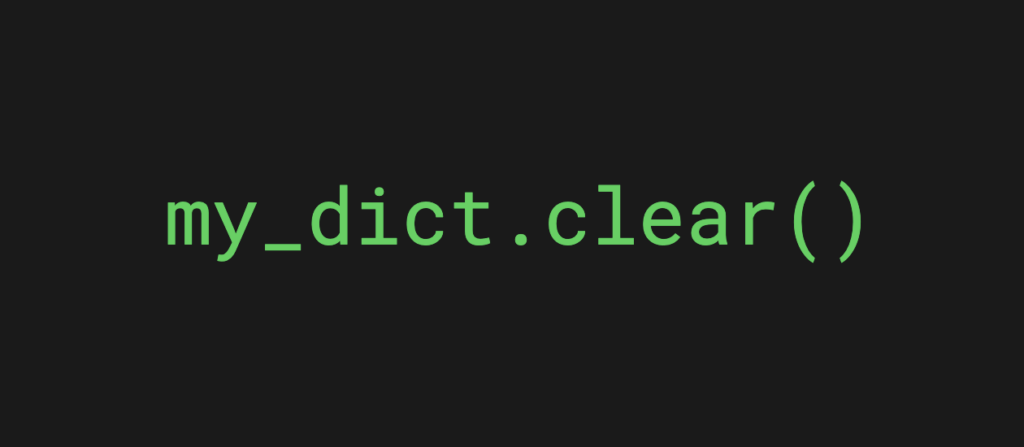
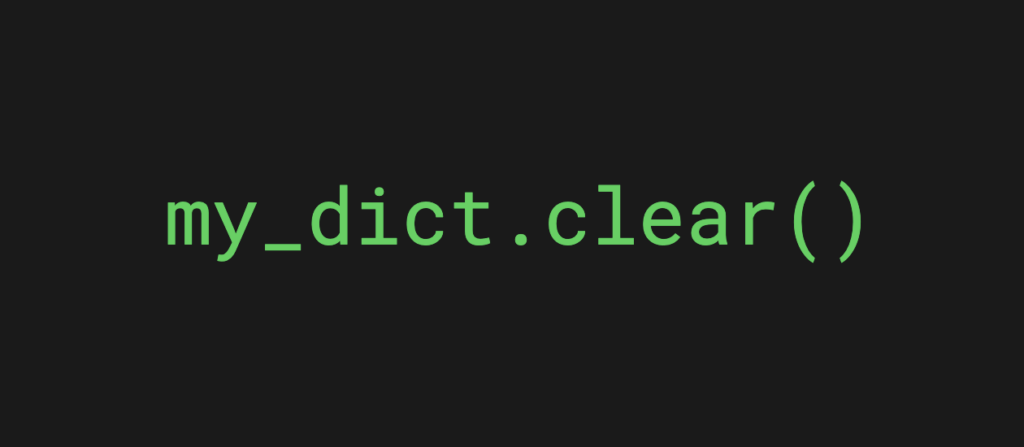
The dictionary.clear()
method in Python is a built-in method used to remove all items from a dictionary. This method does not take any arguments and returns nothing.
Here is an example of how to use the dictionary.clear()
method:
my_dict = {"name": "John", "age": 20} my_dict.clear() print(my_dict) # Output: {}
In the above example, we have created a dictionary called my_dict
with two key-value pairs. Then, we used the dictionary.clear()
method to remove all items from the dictionary. Finally, we printed the dictionary to see that it is now empty.
dict.get()
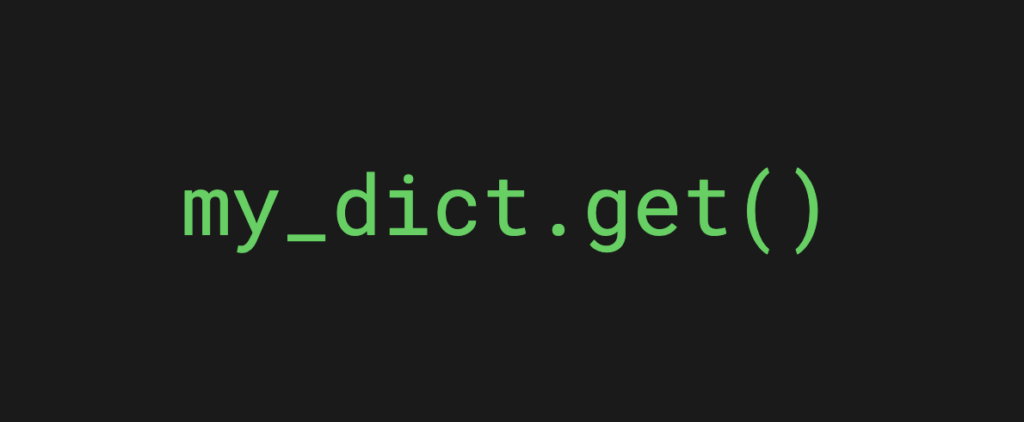
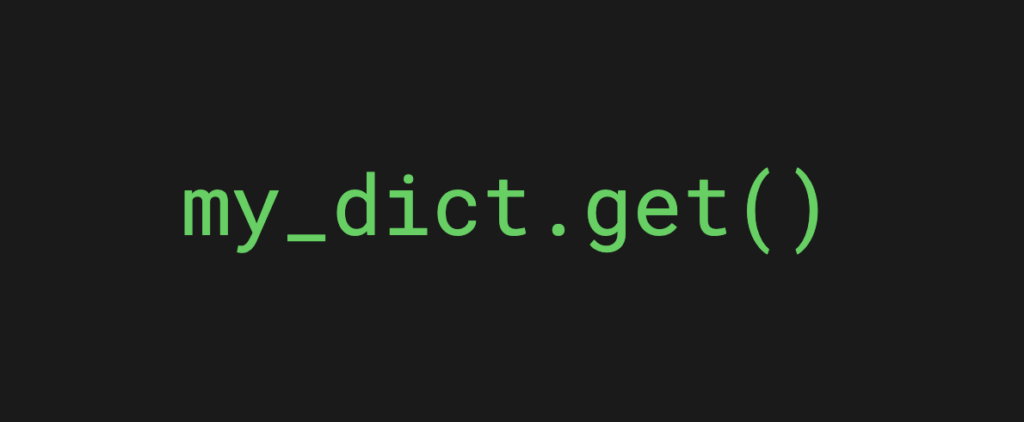
In Python, the get()
method allows you to retrieve the value for a given key from a dictionary.
This method takes two arguments:
- The key of the value you want to retrieve.
- Default value to return if the key is not found in the dictionary.
Here’s an example:
my_dict = {'name': 'John Doe', 'age': 30} # Get the value for the 'name' key name = my_dict.get('name') # This will return 'John Doe' # Get the value for the 'age' key age = my_dict.get('age') # This will return 30 # Try to get the value for a key that doesn't exist in the dictionary favorite_color = my_dict.get('favorite_color', 'unknown') # This will return 'unknown', because the 'favorite_color' key is not in the dictionary
If the key you specify in the get()
method exists in the dictionary, the method will return the corresponding value. If the key does not exist, the method will return the default value you specified.
This is useful for avoiding errors when trying to retrieve values from a dictionary.
dict.items()
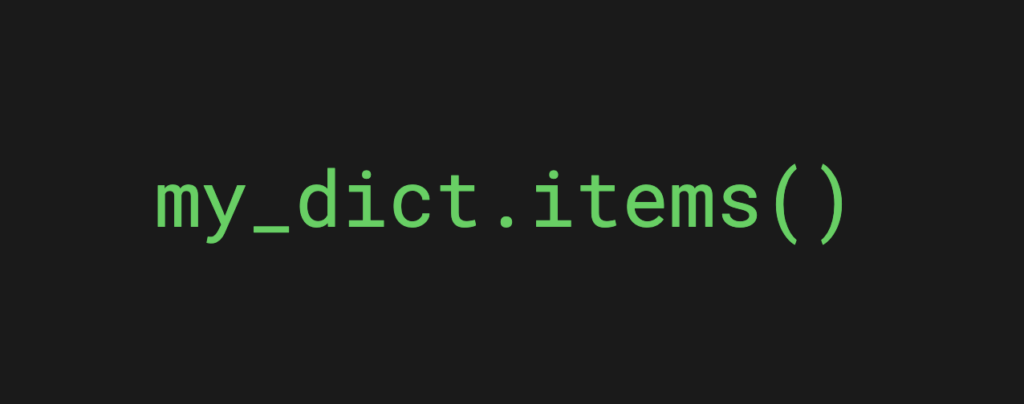
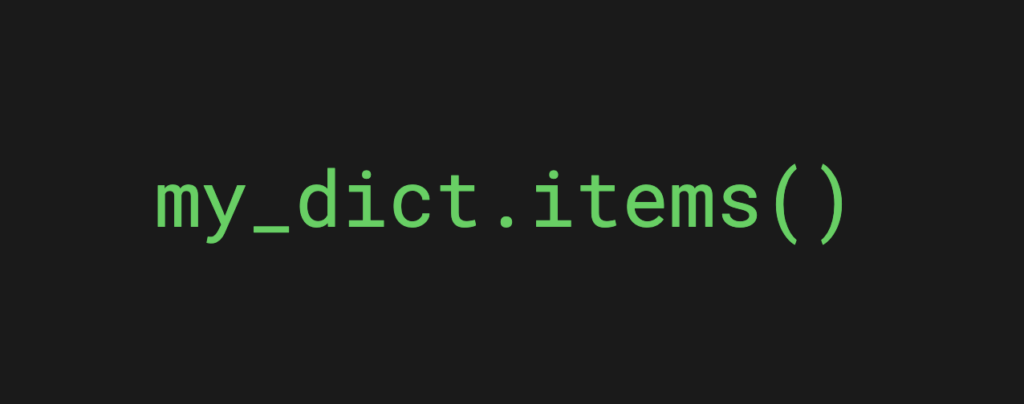
The items()
method in Python dictionaries returns a view object that contains a list of pairs (key, value) for each key-value pair in the dictionary. This method allows the user to access and iterate through the keys and values in the dictionary simultaneously.
For example, let’s loop through the keys and values of a sample dictionary:
my_dict = {'name': 'John Doe', 'age': 30} for key, value in my_dict.items(): print(f"{key}: {value}")
Output:
name: John Doe age: 30
dict.keys()
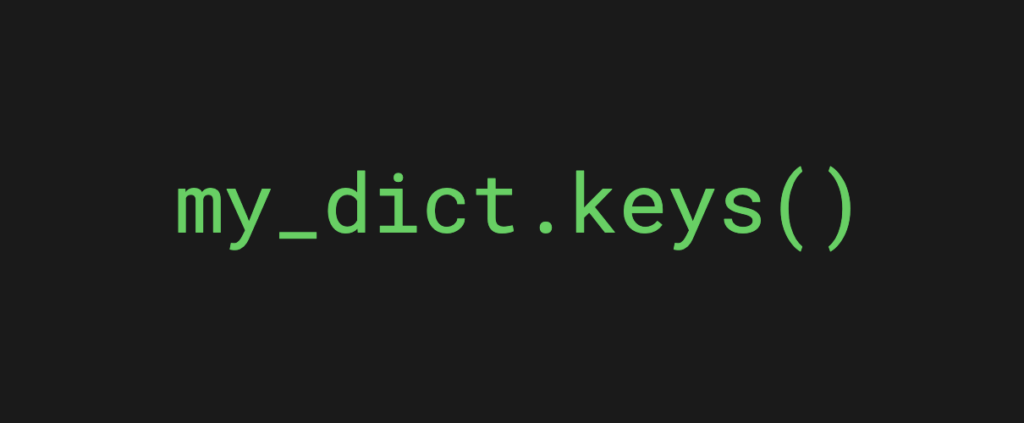
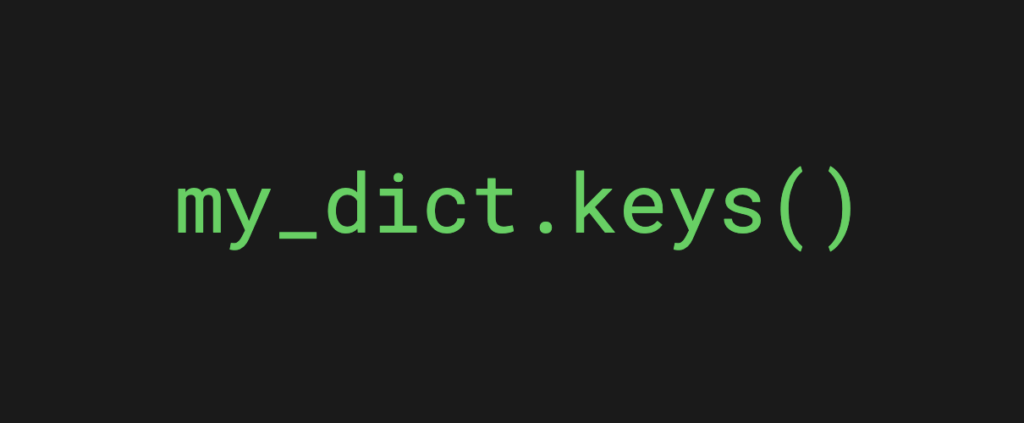
The dictionary’s keys()
method returns a view object that contains the keys of the dictionary.
The keys can then be accessed and used in various ways, such as looping through the keys to access the corresponding values or using the keys to check if a specific key exists in the dictionary.
For example:
my_dict = {'apple': 3, 'banana': 5, 'orange': 7} keys = my_dict.keys() print(keys) # Output: dict_keys(['apple', 'banana', 'orange'])
You can use the keys()
method to loop through the keys and print the corresponding values.
For example:
my_dict = {'apple': 3, 'banana': 5, 'orange': 7} for key in my_dict: print(my_dict[key]) # Output: 3 5 7
dict.values()
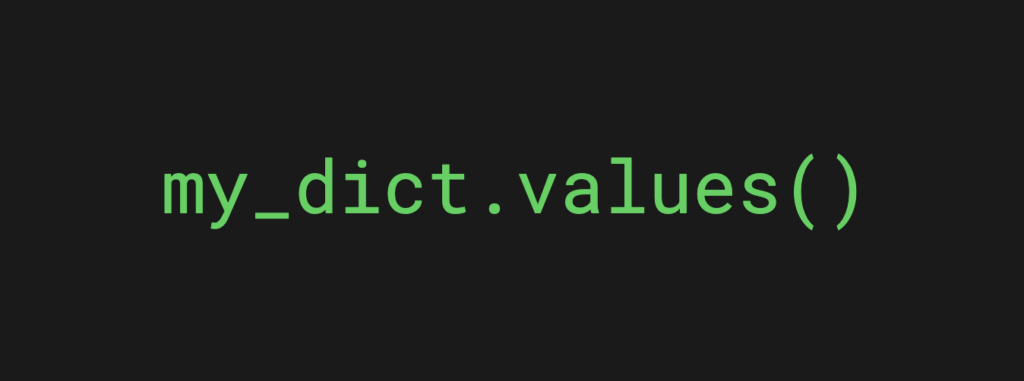
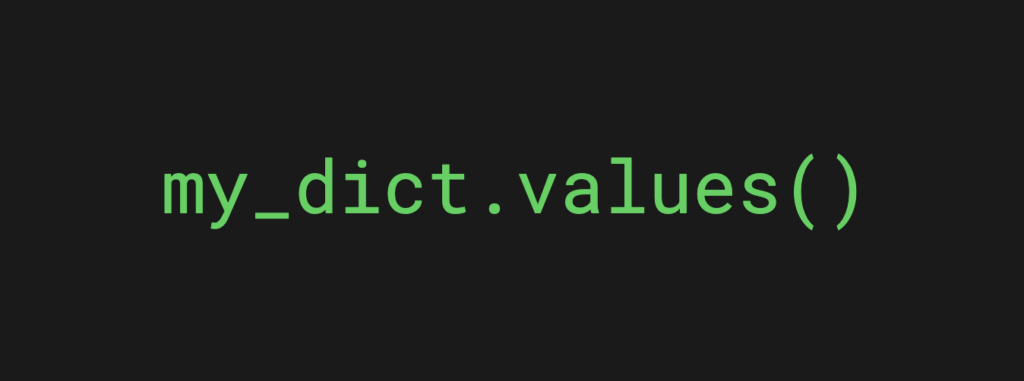
The values()
method in Python dictionaries returns a view object containing the values in the dictionary. This view object is dynamic and updates itself as the dictionary is modified. The values can be accessed by iterating over the view object or by converting it to a list using the list()
function
For example:
my_dict = { "key1": "value1", "key2": "value2", "key3": "value3" } values_view = my_dict.values() # view object containing the dictionary values print(values_view) # outputs: dict_values(['value1', 'value2', 'value3']) values_list = list(values_view) # convert the view object to a list print(values_list) # outputs: ['value1', 'value2', 'value3']
dict.pop()
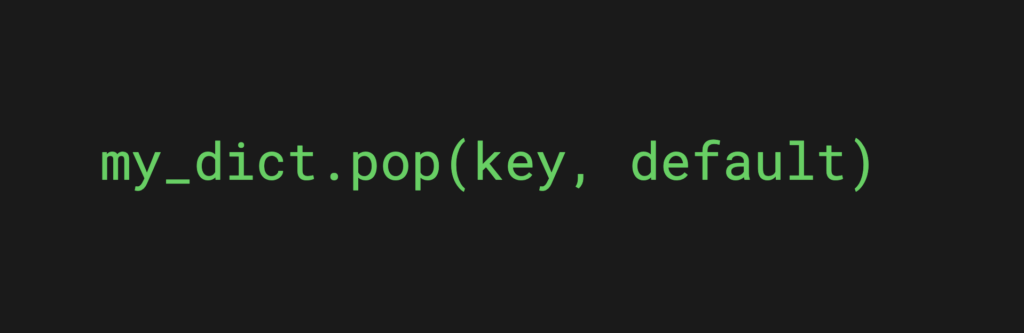
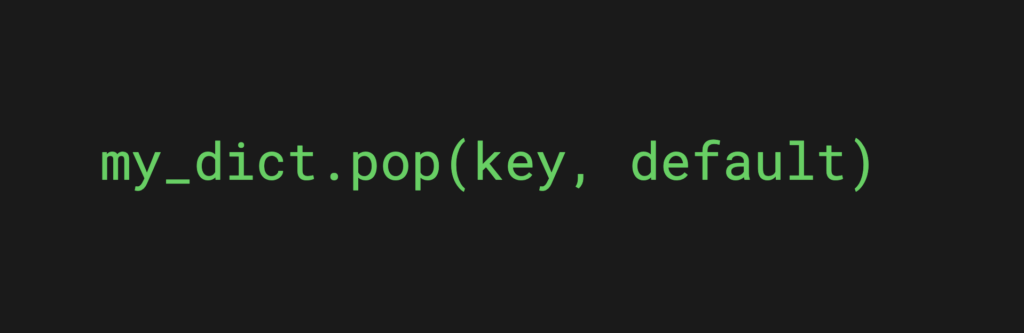
The pop()
method in Python dictionaries is used to remove a specific key-value pair from the dictionary and return the removed value. The syntax for using the pop method is as follows:
dict_name.pop(key, default_value)
Where key
is the key of the key-value pair to be removed, and default_value
is the value to be returned if the key does not exist in the dictionary.
For example, consider the following dictionary:
my_dict = { "name": "John", "age": 20, "gender": "Male" }
To remove the key-value pair with the key “age” using the pop method, we can use the following code:
my_dict.pop("age")
This will remove the key-value pair with the key “age” from the dictionary and return the value 20. The updated dictionary will be:
my_dict = { "name": "John", "gender": "Male" }
If you try to use the pop()
method to remove a key that does not exist in the dictionary, it will raise a KeyError
exception. To avoid this, we can specify a default value to be returned if the key does not exist in the dictionary, as shown below:
age = my_dict.pop("age", None)
In this example, if the key “age” does not exist in the dictionary, the value None
will be returned and no error will be raised.
dict.popitem()


The popitem()
method in Python dictionaries works by removing and returning a randomly chosen key-value pair from the dictionary.
Here is an example of how the popitem()
method can be used in a Python dictionary:
# Create a dictionary with some key-value pairs my_dict = { "apple": "red", "banana": "yellow", "carrot": "orange" } # Use the popitem method to remove a random key-value pair from the dictionary random_pair = my_dict.popitem() # Print the removed key-value pair print(random_pair) # Print the updated dictionary print(my_dict)
Output:
('carrot', 'orange') {'apple': 'red', 'banana': 'yellow'}
In the above example, the popitem()
method removes a random key-value pair from the dictionary and stores it in the random_pair
variable. The updated dictionary is then printed, which shows that the removed key-value pair is no longer present in the dictionary.
dict.update()
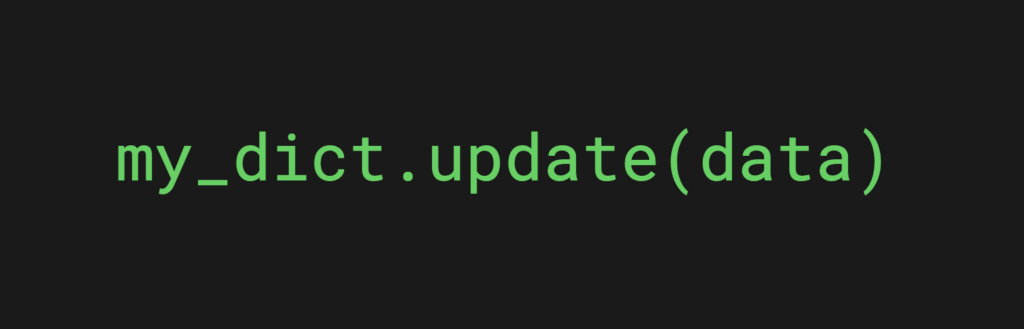
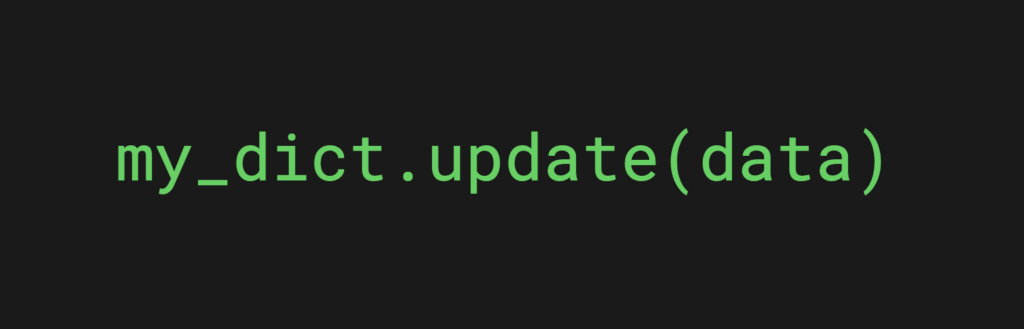
The update()
method in Python dictionaries is used to add or update key-value pairs in a dictionary. It takes a dictionary or an iterable object (such as a list or a set) as an argument and adds the key-value pairs from that object to the original dictionary.
For example, let’s first create a dictionary called my_dict
:
my_dict = {'fruit': 'apple', 'vegetable': 'carrot'}
Then let’s see a couple of examples of using the update()
method.
1. Add a new key-value pair to the dictionary
You can update the dictionary by passing in a new key-value pair:
my_dict.update({'meat': 'chicken'})
Let’s print the dictionary to see how it changed:
print(my_dict)
Output:
{'fruit': 'apple', 'vegetable': 'carrot', 'meat': 'chicken'}
Update the value of an existing key in the dictionary
You can also use the update() method to update the value of an existing key.
my_dict.update({'fruit': 'banana'}) print(my_dict)
Output:
{'fruit': 'banana', 'vegetable': 'carrot', 'meat': 'chicken'}
Note: If the same key exists in both the original dictionary and the object passed to the update method, the value in the object passed to the update method will replace the value in the original dictionary.
How to Loop Through Dictionaries in Python?
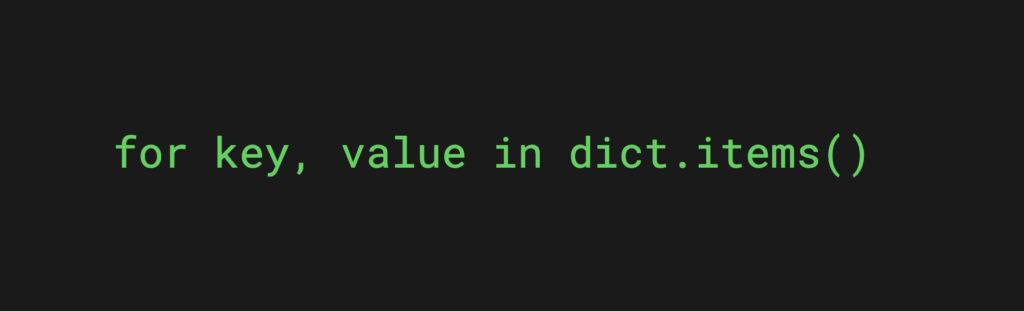
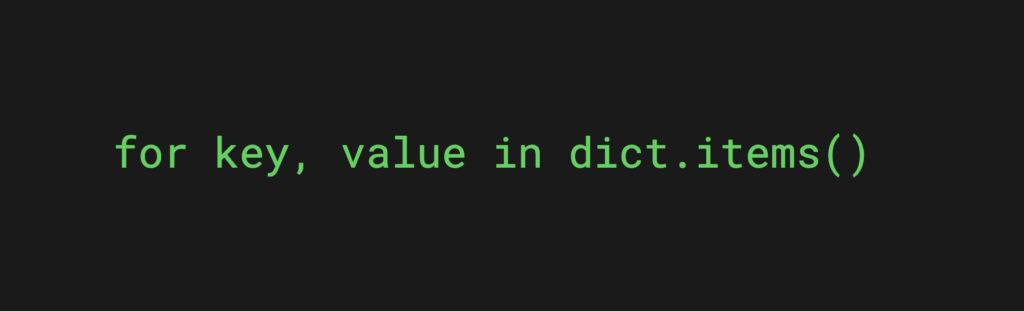
To loop through a dictionary in Python, use the for loop and the items()
method, which returns the dictionary’s keys and values.
You actually saw an example of this earlier in this guide. But because looping through a dictionary is such a common operation, it’s worthwhile to go through it in a bit more detail here.
For example:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} for key, value in my_dict.items(): print(key + ": " + value)
This prints each key-value pair on a separate line:
key1: value1 key2: value2 key3: value3
To loop through the keys only, use the for loop combined with the keys()
method.
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} for key in my_dict.keys(): print(key)
This will print each key on a separate line, like this:
key1 key2 key3
To loop through the values only, use the values()
method. It returns a list of the dictionary’s values.
For example:
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} for value in my_dict.values(): print(value)
This prints each value on a separate line, like this:
value1 value2 value3
Summary
Today you learned what is a dictionary in Python.
The main use case for Python dictionaries is to store and retrieve data in a key-value format. In Python, dictionaries are used to represent data structures that are similar to associative arrays or hash tables in other programming languages.
Dictionaries are often used to store data in a more structured and efficient way than other data types, such as lists or tuples. This is because dictionaries allow you to access and retrieve the data using keys instead of indexes. This makes it easier to organize and manipulate the data in your code, especially when working with large datasets.
Additionally, dictionaries are often used to implement more complex data structures, such as graphs and trees, because they allow you to store and access data in a hierarchical or nested format.
Overall, the main advantage of using dictionaries in Python is that they provide an efficient and flexible way to store, retrieve, and manipulate data in your code.
Thanks for reading. Happy coding!