Learn basic geometry with Python, such as, how to calculate the volume of a triangular prism.
This guide teaches you maths while teaching you how to convert your simple math problems into Python code.
Geometric Volumes with Python
In this article, you find a cheat sheet of volumes of the most common geometric shapes.
For each shape, I’ve written down a Python program to calculate the volume.
1. Cylinder
A cylinder is a shape that consists of two parallel circular bases connected together by a curved surface.
Here is an illustration:
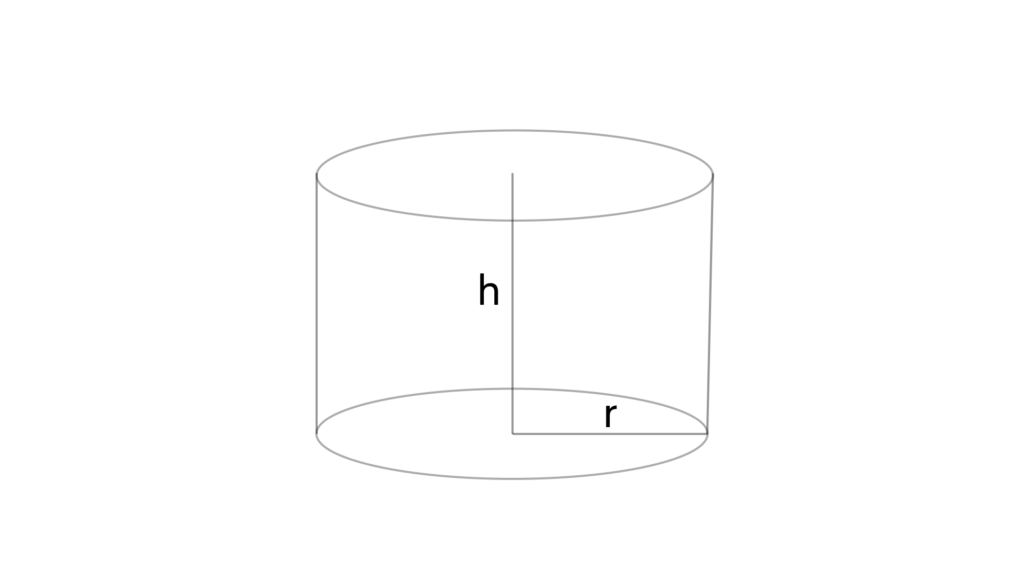
To figure out the volume of a cylinder, you need to know the radius of the base and the height of the connecting surface.
Here is the formula:
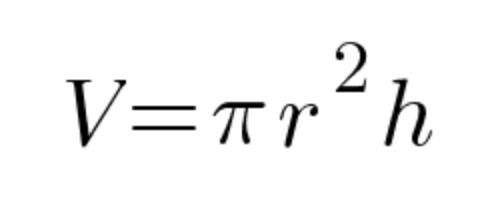
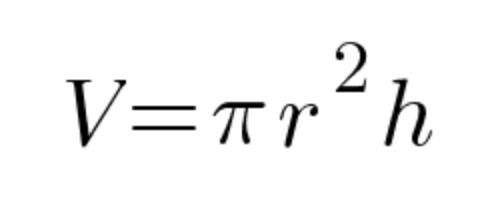
Here is a Python function that calculates the volume of a cylinder given radius and height:
import math def v_cylinder(r, h): return math.pi * r ** 2 * h
2. Sphere
A sphere is a round and symmetrical shape in three dimensions. Each surface point of the sphere is equally far from the center.
To find the volume of a sphere, all you need to know is the radius of the sphere.
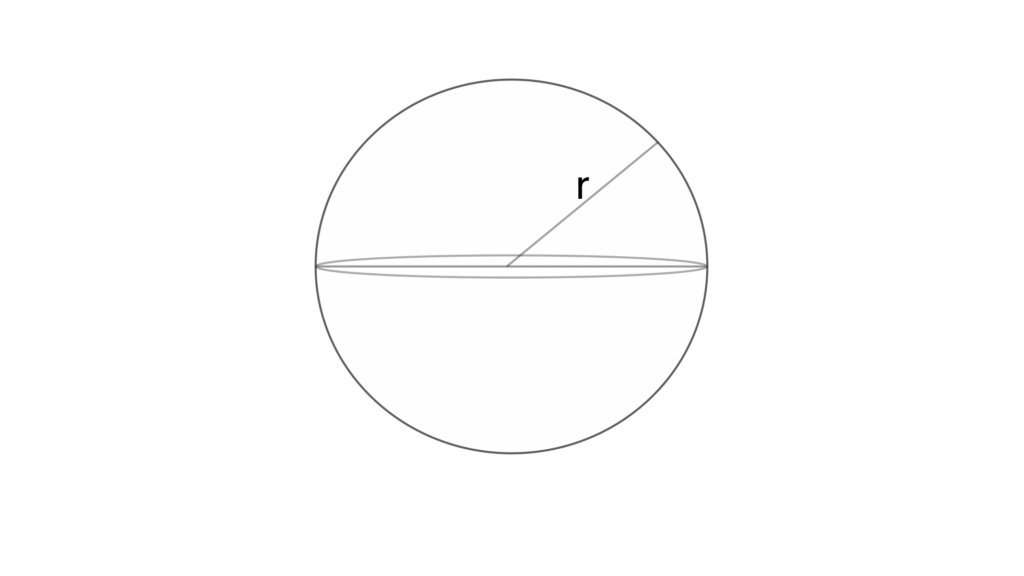
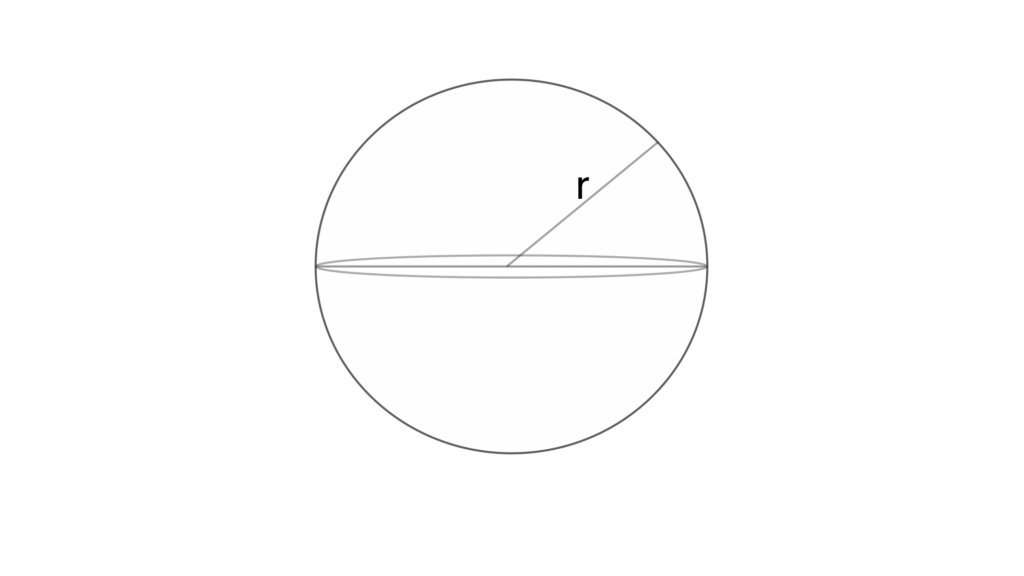
Here is the formula for the volume of the sphere:
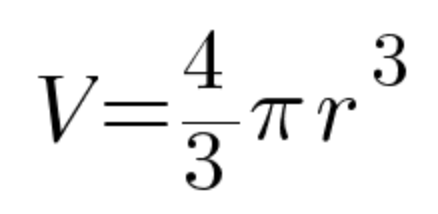
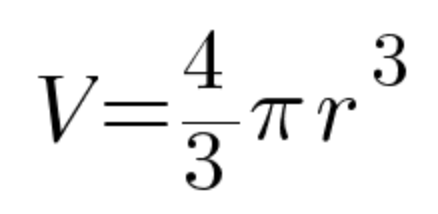
Here is a Python function that calculates the volume of a sphere given the radius of the sphere:
import math def v_sphere(r): return (4/3) * math.pi * r ** 3
3. Cube
A cube is a 3D object that is bounded by six faces. Each face of the cube has equal side lengths.
To find out the volume of a cube, you need to know the side length of a face.
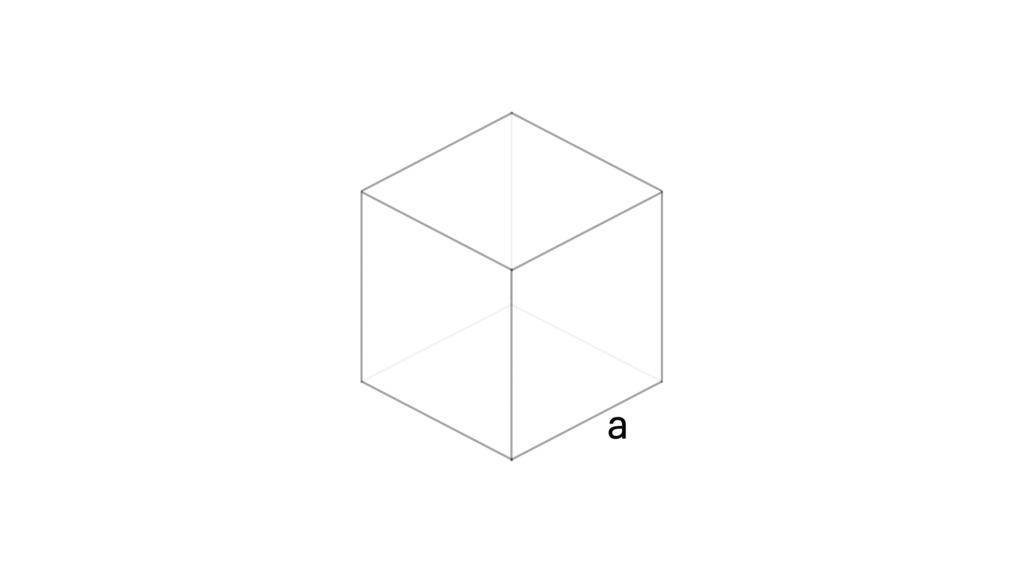
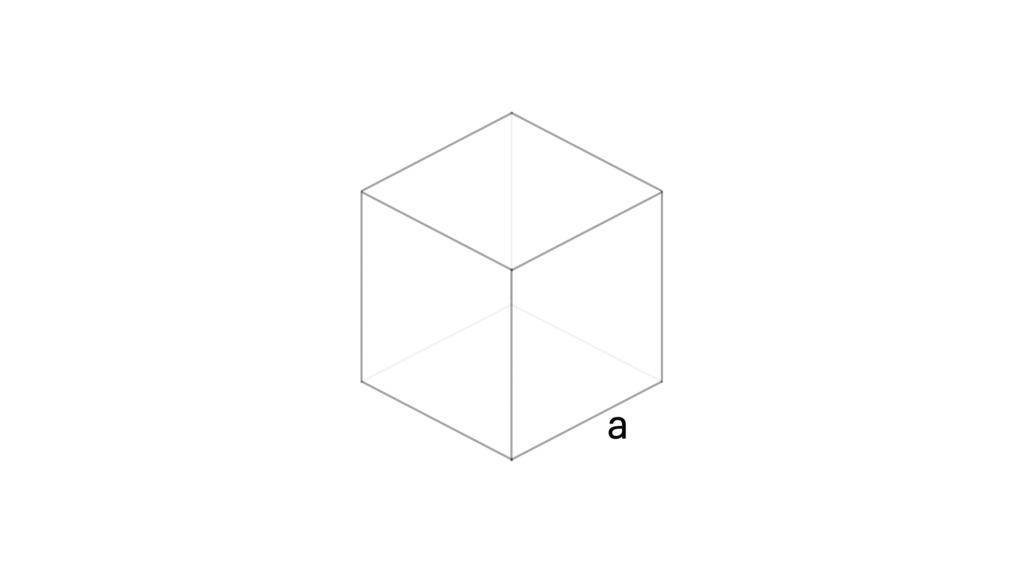
Here is the formula for the volume of a cube.
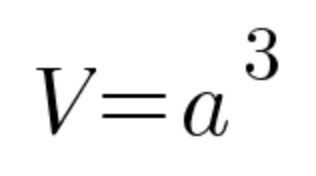
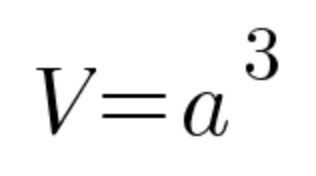
Here is a Python function that calculates the volume of a cube given side length:
def v_cube(a): return a ** 3
4. Rectangular Prism/Cuboid
A rectangular prism, also known as a cuboid, is a 3D shape with six rectangular surfaces. Each side is rectangular. A cuboid has length, width, and height.
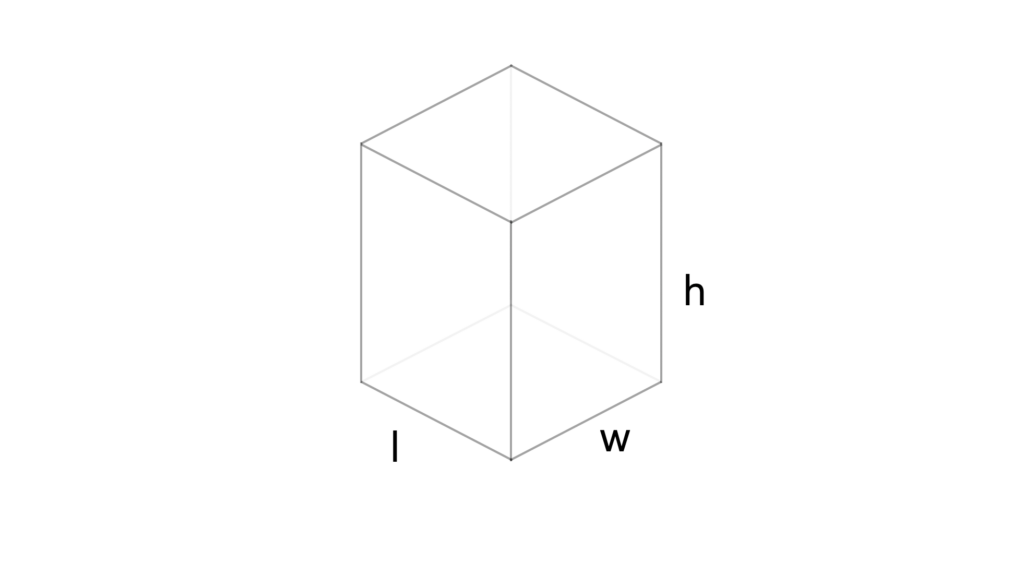
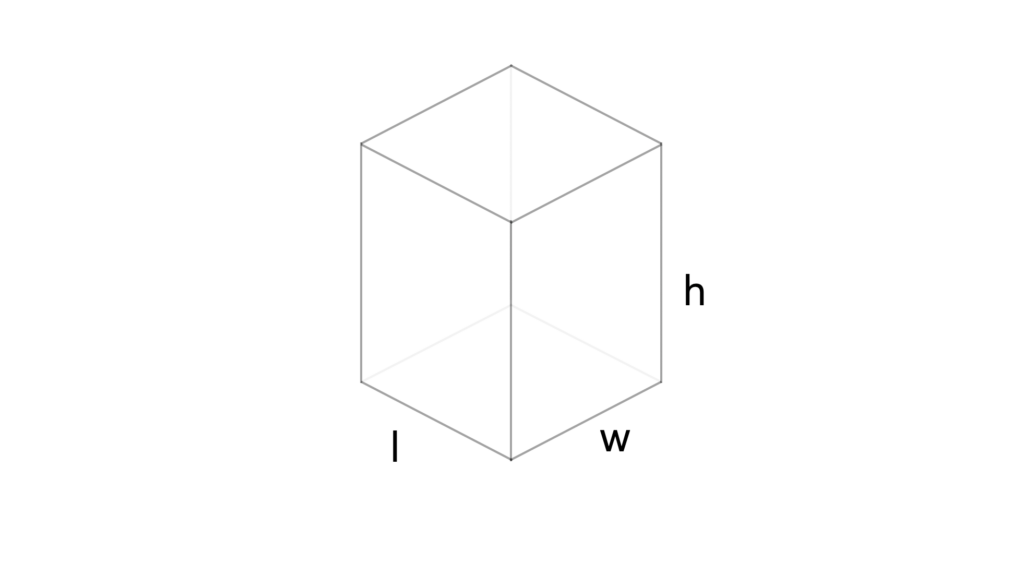
To calculate the volume of a cuboid, you need to know the width, height, and length of the cuboid.
Here is the formula:
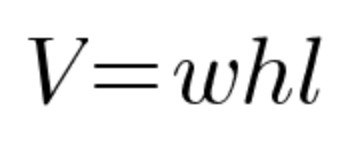
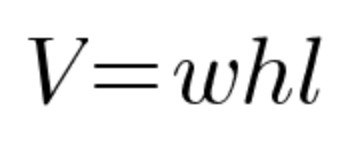
Here is a Python function for calculating the volume of a rectangular prism given width, height, and length:
def v_cuboid(w, h, l): return w * h * l
5. Triangular Prism
A triangular prism is a 3D shape. It consists of two triangular bases and three rectangular sides.
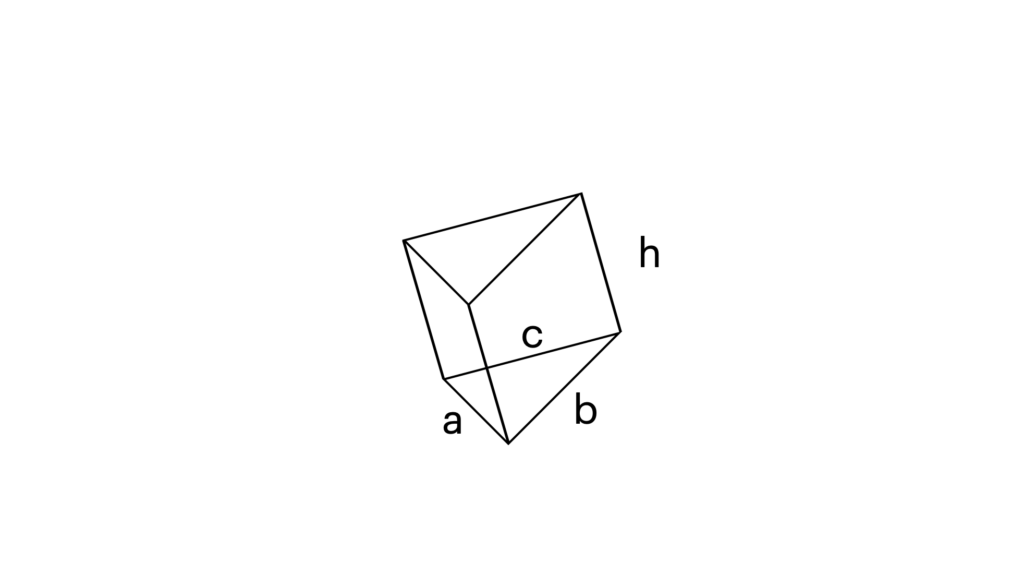
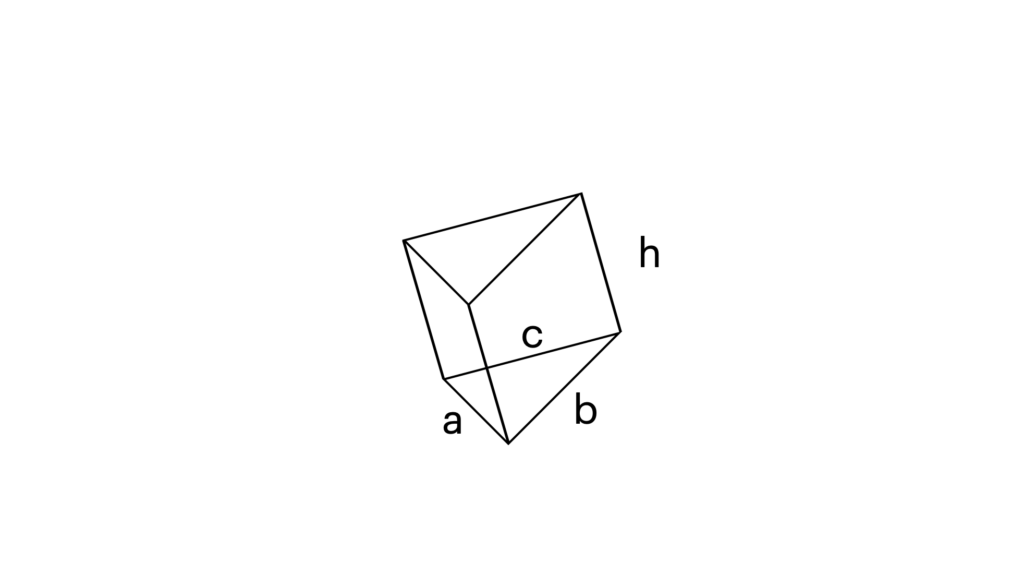
To figure out the volume of a triangular prism, you need to know the basis triangle’s side lengths and the height of the prism.


Here is a Python function that calculates the volume of a triangular prism given side lengths of the base triangle and the height of the prism.
import math def v_triangular_prism(a, b, c, h): return (1/4) * h * math.sqrt(-a ** 4 + 2 * (a * b) ** 2 + 2 * (a * c) ** 2 - b ** 4 + 2 * (b * c) ** 2 - c ** 4)
6. Cone
A cone is a 3D shape that has a circular base and a straight line as its axis. The cone narrows down the higher you go until it reaches its apex.
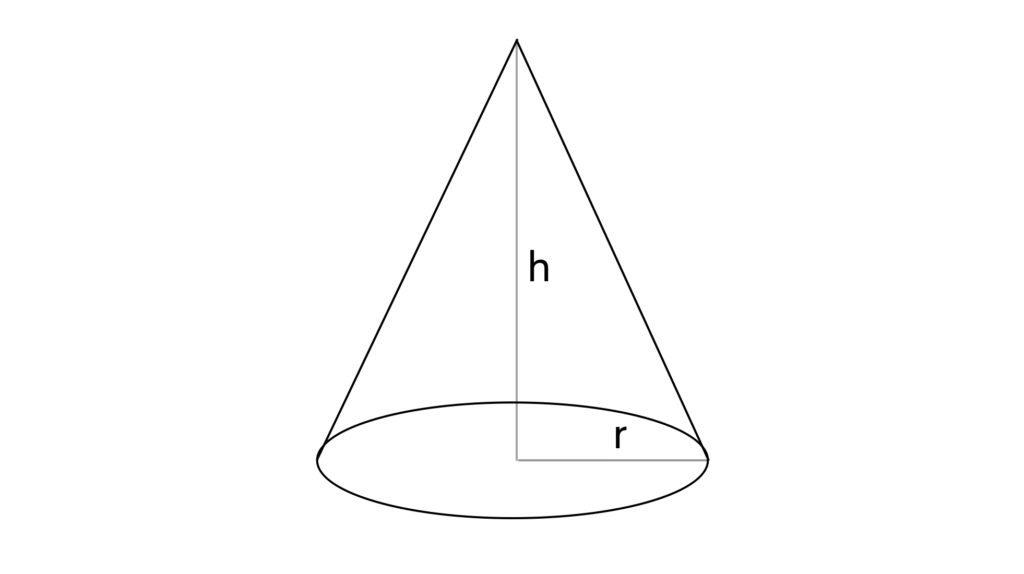
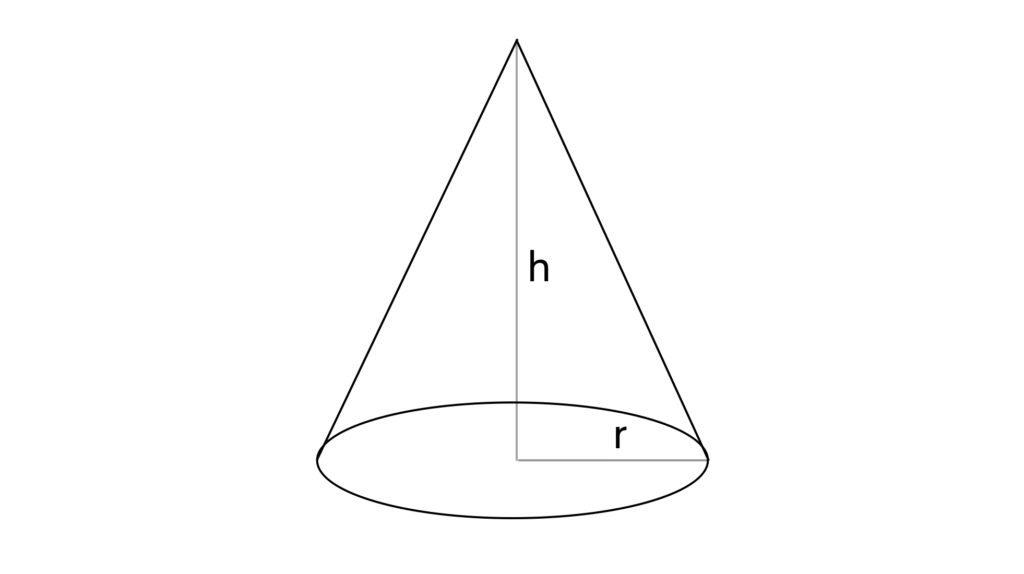
To calculate the volume of a cone, you need to know the radius of the base and the height to the apex.
Here is the formula for calculating the volume of a cone:
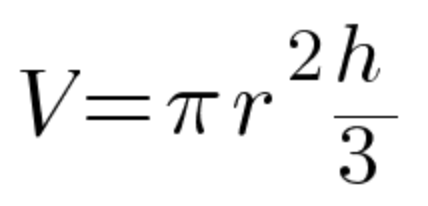
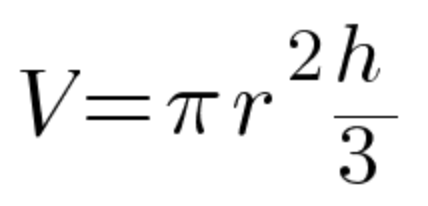
Here is a Python program that calculates the volume of a cone:
import math def v_cone(r, h): return math.pi * r ** 2 * h / 3
7. Pyramid
A pyramid is a 3D shape with a rectangular base. Its sides are triangular. They are tilted such that the pyramid narrows down the higher you go until you reach the apex.
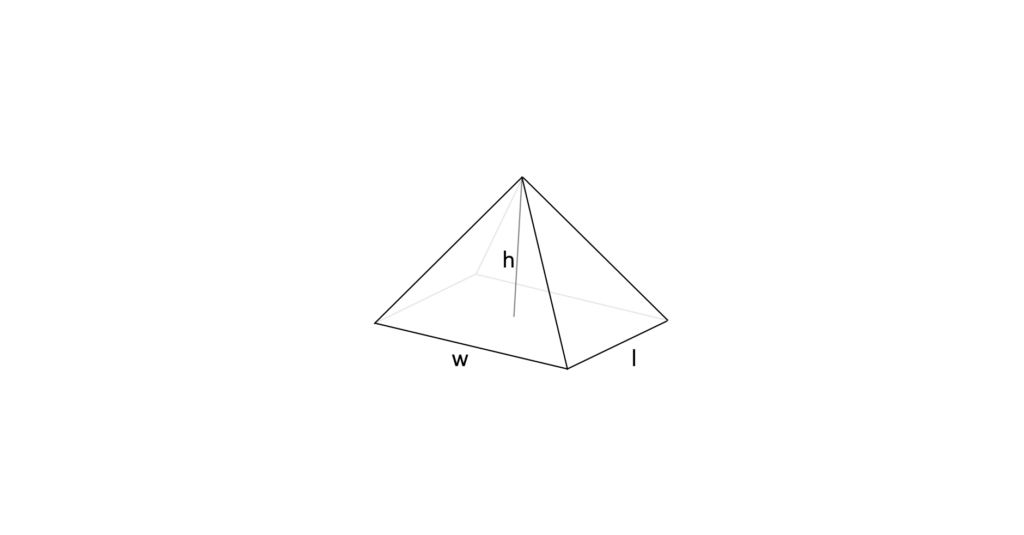
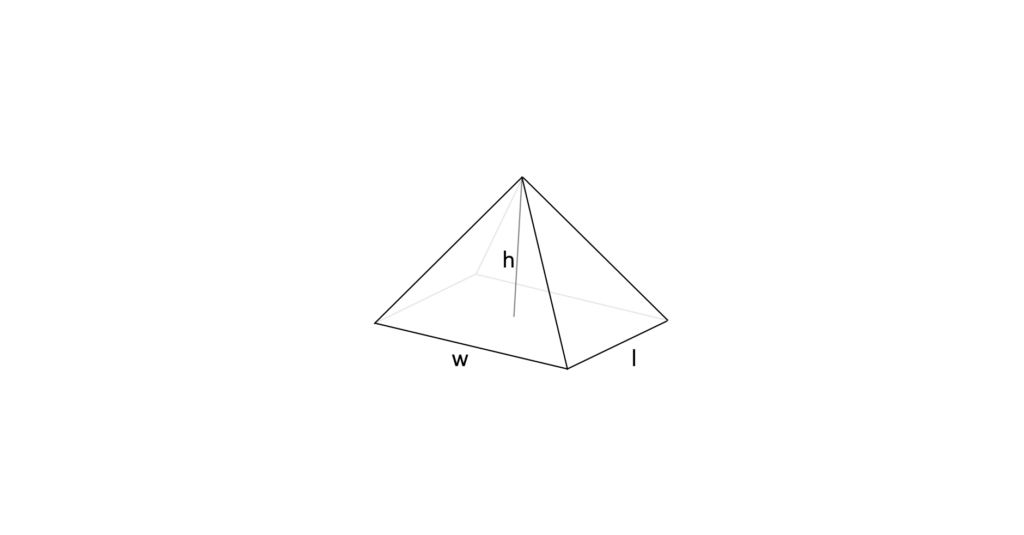
To figure out the volume of a pyramid, you need to know the side lengths of the base rectangle and the height of the apex.
Here is the formula for calculating the volume of a Pyramid:
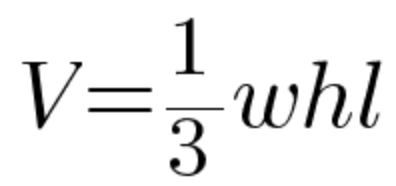
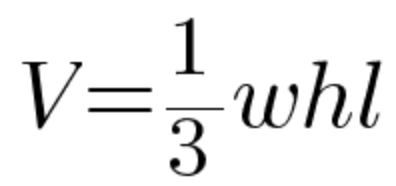
Here is a Python function for calculating the volume of a Pyramid given its width, height, and length:
def v_pyramid(w, h, l): return (1/3) * w * h * l